Common Pitfalls in JSON API Processing and How to Fix Them
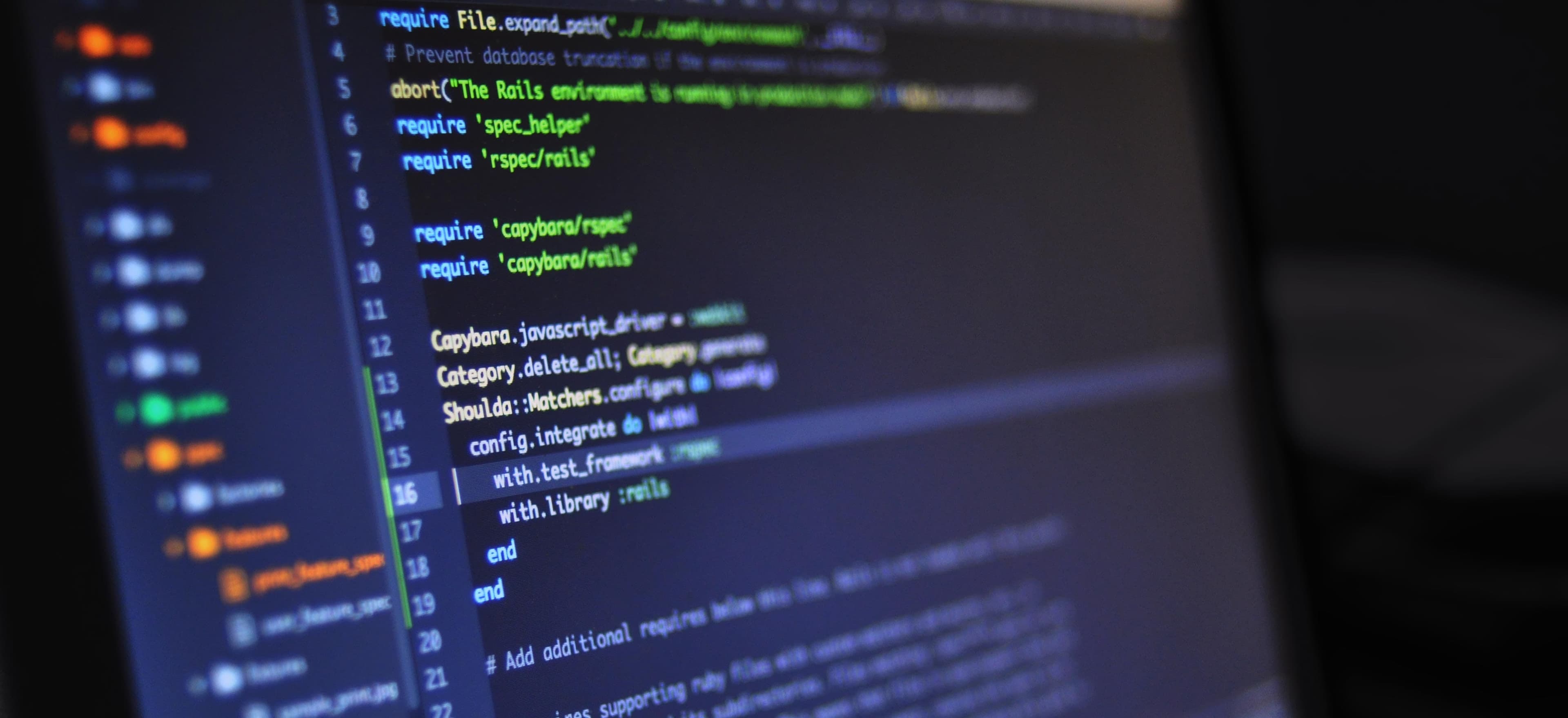
- Published on
Common Pitfalls in JSON API Processing and How to Fix Them
As the prevalence of APIs continues to grow, the JSON format has emerged as the preferred method for data exchange. Lightweight and easy to read, JSON is an ideal choice for web services in various applications. However, despite its simplicity, developers often encounter several pitfalls when processing JSON APIs. This post discusses these common pitfalls and provides practical solutions to enhance your API processing efficiency.
Understanding JSON and Its Importance
JSON (JavaScript Object Notation) is a lightweight data-interchange format that is easy for humans to read and write. Its key-value pair structure allows for a clear hierarchy of data, which is essential when sending and receiving information between servers and clients.
Using JSON APIs has become the norm in modern web development because of:
- Platform Agnosticism: JSON can be used across different programming languages.
- Ease of Use: Its simple format makes it easy to understand and implement.
- Data Structure: JSON supports nested structures, which allows for complex data representation.
Understanding the common hurdles encountered when processing JSON APIs is critical to building robust applications. Let’s delve into those issues and how to mitigate them.
Pitfall 1: Improper Error Handling
Problem: When dealing with API calls, developers often overlook error handling. An API might return an error code that signifies an issue, but if your application does not account for this, it can lead to unhandled exceptions or unwanted behaviors.
Solution: Always validate the response status of your API calls and implement robust error handling routines. Below is an example in Java using OkHttp for an API request:
import okhttp3.*;
import java.io.IOException;
public class ApiService {
private final OkHttpClient client = new OkHttpClient();
public void fetchData(String url) {
Request request = new Request.Builder()
.url(url)
.build();
client.newCall(request).enqueue(new Callback() {
@Override
public void onFailure(Call call, IOException e) {
// Handle network errors gracefully
System.out.println("Network error: " + e.getMessage());
}
@Override
public void onResponse(Call call, Response response) throws IOException {
if (!response.isSuccessful()) {
// Handle possible API errors
System.out.println("API Error: " + response.code() + " - " + response.message());
return;
}
// Process the successful response
String responseData = response.body().string();
System.out.println("Response: " + responseData);
}
});
}
}
Why This Matters: Handling errors gracefully allows developers to provide informative feedback to users instead of letting unexpected exceptions crash the application. Always consider both HTTP error responses and exceptions.
Pitfall 2: Overlooking Data Validation
Problem: Not validating or sanitizing the data received from an API can lead to security vulnerabilities, such as SQL injection or XSS attacks.
Solution: Implement robust data validation techniques for any input. Use libraries that can handle JSON parsing and validation. For example, using Jackson for processing JSON can be very effective:
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.ObjectMapper;
public class JsonProcessor {
private final ObjectMapper objectMapper = new ObjectMapper();
public void processJson(String jsonString) {
try {
JsonNode jsonNode = objectMapper.readTree(jsonString);
// Validate required fields
if (jsonNode.has("name")) {
String name = jsonNode.get("name").asText();
// Process name safely
} else {
throw new IllegalArgumentException("Missing required field: name");
}
} catch (Exception e) {
System.out.println("Error processing JSON: " + e.getMessage());
}
}
}
Why This Matters: Validating data ensures that only correct and expected information is processed, reducing the risk of attacks and maintaining the integrity of your application.
Pitfall 3: Failing to Handle Nested JSON
Problem: Nested JSON structures can lead to confusion if not handled properly. Developers may assume flat data structures are returned, leading to errors when trying to access data.
Solution: Take time to analyze the structure of the JSON data and implement recursive or iterative techniques to traverse this hierarchy effectively. Here is an example:
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.ObjectMapper;
public class NestedJsonHandler {
private final ObjectMapper objectMapper = new ObjectMapper();
public void handleNestedJson(String jsonData) {
try {
JsonNode rootNode = objectMapper.readTree(jsonData);
JsonNode addressNode = rootNode.path("address");
// Safely access nested values
String city = addressNode.path("city").asText();
String zip = addressNode.path("zip").asText();
System.out.println("City: " + city + ", Zip: " + zip);
} catch (Exception e) {
System.out.println("Error parsing nested JSON: " + e.getMessage());
}
}
}
Why This Matters: Understanding the structure of JSON allows developers to access and manipulate data accurately. By leveraging libraries that can navigate the JSON tree, such as Jackson, developers can make their code cleaner and more maintainable.
Pitfall 4: Ignoring Asynchronous Processing
Problem: Synchronous API calls can lead to suboptimal performance, especially if waiting for the server response blocks execution. This can severely affect user experience.
Solution: Utilize asynchronous processing techniques. Libraries like Retrofit or native Java's CompletableFuture can help manage asynchronous calls. Here’s an example:
import java.util.concurrent.CompletableFuture;
public class AsyncApiService {
private final OkHttpClient client = new OkHttpClient();
public CompletableFuture<String> fetchAsyncData(String url) {
return CompletableFuture.supplyAsync(() -> {
Request request = new Request.Builder()
.url(url)
.build();
try (Response response = client.newCall(request).execute()) {
if (!response.isSuccessful()) {
throw new IOException("Unexpected code " + response);
}
return response.body().string();
} catch (IOException e) {
throw new RuntimeException("Failed to fetch data", e);
}
});
}
}
// Usage
AsyncApiService service = new AsyncApiService();
service.fetchAsyncData("https://api.example.com/data")
.thenAccept(System.out::println)
.exceptionally(e -> { System.out.println(e.getMessage()); return null; });
Why This Matters: Asynchronous processing ensures that your application remains responsive, improving the overall user experience. The code remains clean and allows for better resource management.
Wrapping Up
Processing JSON APIs effectively remains a fundamental skill for developers. By being aware of common pitfalls—including error handling, data validation, handling of nested structures, and employing asynchronous processing—you can create applications that are not only robust but also efficient and secure.
For further reading on API best practices, check out RESTful API Design and visit JSON.org for more on JSON specifications.
Avoiding these pitfalls will enhance your development process, reduce maintenance overhead, and improve user satisfaction. By implementing the strategies outlined in this post, you are sure to navigate the complexities of JSON API processing with confidence.