Common Pitfalls in DMN Runtime with Drools Explained
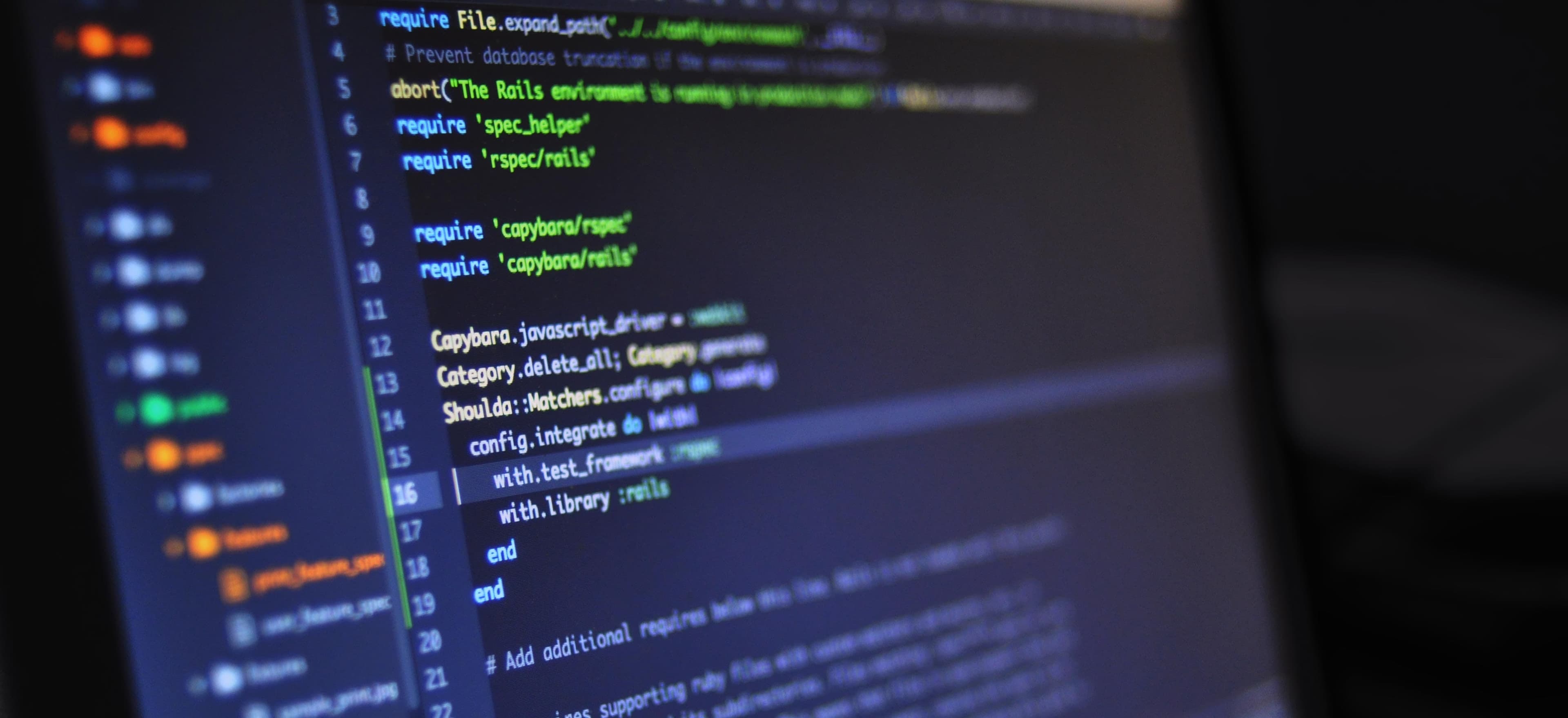
- Published on
Common Pitfalls in DMN Runtime with Drools Explained
Decision Model and Notation (DMN) is a powerful tool that provides a standardized way to model decisions. Combined with Drools, a business rules management system (BRMS), it offers a flexible environment for executing decision models. However, working with DMN in Drools can present some challenges. This blog post highlights common pitfalls developers encounter while using DMN runtime in Drools and offers solutions to avoid them.
Understanding DMN and Drools
Before delving into the common pitfalls, it’s essential to understand what DMN and Drools are:
- DMN: A standardized notation for modeling decision-making processes, which allows business and technical stakeholders to easily articulate and modify their decisions.
- Drools: A robust BRMS that enables you to define business rules and policies using DMN. Drools allows for complex event processing, chaining rules, and executing decisions based on data inputs.
Common Pitfalls
1. Misunderstanding DMN Hierarchy and Structure
The Pitfall:
One of the most frustrating issues developers face is misinterpreting DMN models' hierarchy. Each DMN model can consist of multiple decision tables, ctx (contexts), and decision requirements. New users often overlook the relationship between these entities.
Solution:
Always visualize your DMN model before implementation. Make a clear diagram of how decisions are interconnected. Use DMN modeling tools like Camunda Modeler or KIE Workbench to facilitate this process. This will help in understanding dependencies and responsibilities.
2. Incompletely Defined Inputs
The Pitfall:
Often, developers assume that all required input data for the DMN model will always be available. Missing or incomplete inputs can cause the execution to fail or produce incorrect results.
Solution:
Implement validation checks before executing the DMN model. Here’s a simple Java snippet that ensures required inputs are checked:
public boolean validateInputs(Map<String, Object> inputs) {
List<String> requiredInputs = Arrays.asList("Input1", "Input2", "Input3");
for (String input : requiredInputs) {
if (!inputs.containsKey(input)) {
throw new IllegalArgumentException("Missing required input: " + input);
}
}
return true;
}
Why this is important: This validation guarantees that you have all needed data before proceeding, preventing unexpected errors or miscalculations.
3. Ignoring Version Control in DMN Models
The Pitfall:
Neglecting to version-control DMN files creates confusion, particularly when multiple team members are involved. Changes made by one member can inadvertently conflict with changes made by another, causing unexpected behaviors.
Solution:
Employ a proper versioning system for your DMN files. Use Git or other version control tools to track changes. Here’s how you can add a version comment directly in your DMN model:
<dmn:definitions xmlns:dmn="http://www.omg.org/spec/DMN/20191111/MODEL/" xmlns:feel="http://www.omg.org/spec/FEEL/20141201">
<dmn:decision id="decision1" name="Decision Example">
<!-- Version 1.0 -->
</dmn:decision>
</dmn:definitions>
Why this matters: Proper version control allows teams to revert to previous versions when issues arise and understand the history of changes made to decision models.
4. Performance Bottlenecks
The Pitfall:
As decision models grow in complexity, developers might experience performance issues during execution, particularly with large data sets. A poorly optimized DMN model can lead to slow execution times.
Solution:
Optimize your decision tables by using the "hit policy" efficiently and reducing the number of rows. Also, ensure that computationally intense expressions are minimized. Here is an example of optimizing expressions:
<dmn:decisionTable hitPolicy="FIRST">
<dmn:input id="Input1">
<dmn:inputExpression typeRef="string">Input Data</dmn:inputExpression>
</dmn:input>
<dmn:output id="Output" label="Result" typeRef="string"/>
<dmn:rule>
<dmn:inputEntry>
<dmn:text>value1</dmn:text>
</dmn:inputEntry>
<dmn:outputEntry>
<dmn:text>result1</dmn:text>
</dmn:outputEntry>
</dmn:rule>
<!-- Add more rules as needed. Avoid redundant calculations. -->
</dmn:decisionTable>
Why this is essential: Efficient decision tables can drastically improve the performance of your DMN engine, allowing for faster decision processing and robust applications.
5. Lack of Testing and Debugging Procedures
The Pitfall:
Some developers dive into implementing a DMN model without dedicated testing, leaving several edge cases unaddressed. This can lead to flawed decision outcomes after deployment.
Solution:
Establish a robust testing framework for your DMN models using JUnit, ensuring that all rules are covered and behave as expected. Here’s how to set this up:
import static org.junit.jupiter.api.Assertions.*;
import org.junit.jupiter.api.Test;
import org.kie.api.runtime.KieContainer;
import org.kie.api.runtime.KieSession;
public class DMNTest {
@Test
public void testDecisionLogic() {
KieContainer kieContainer = ... // Initialize your KieContainer
KieSession kieSession = kieContainer.newKieSession("ksession-dmn");
// Example Input Data
Map<String, Object> inputs = new HashMap<>();
inputs.put("Input1", "value1");
inputs.put("Input2", "value2");
kieSession.execute(new DMNCommand(inputs));
// Assert the expected output
Object result = ...; // Get the result from the session
assertEquals("expectedResult", result);
}
}
Why testing is crucial: Comprehensive testing uncovers hidden bugs early, ensuring that your decision model is reliable and functions well under various inputs.
Key Takeaways
The DMN runtime in Drools provides powerful capabilities for executing decision models, but it is not without its pitfalls. By understanding common issues and implementing solutions proactively, developers can create robust, performant DMN applications. As with any programming technology, consistency in best practices, along with clear collaboration among team members, drives success.
For more insights into utilizing Drools effectively, check the official documentation on Drools Documentation. Additionally, the DMN Specification provides valuable resources to understand decision modeling comprehensively.
By being aware of these common pitfalls and employing the suggested solutions, you can save yourself countless headaches and streamline your development process. Happy coding!