Common Pitfalls When Setting Up JNDI with Embedded Jetty
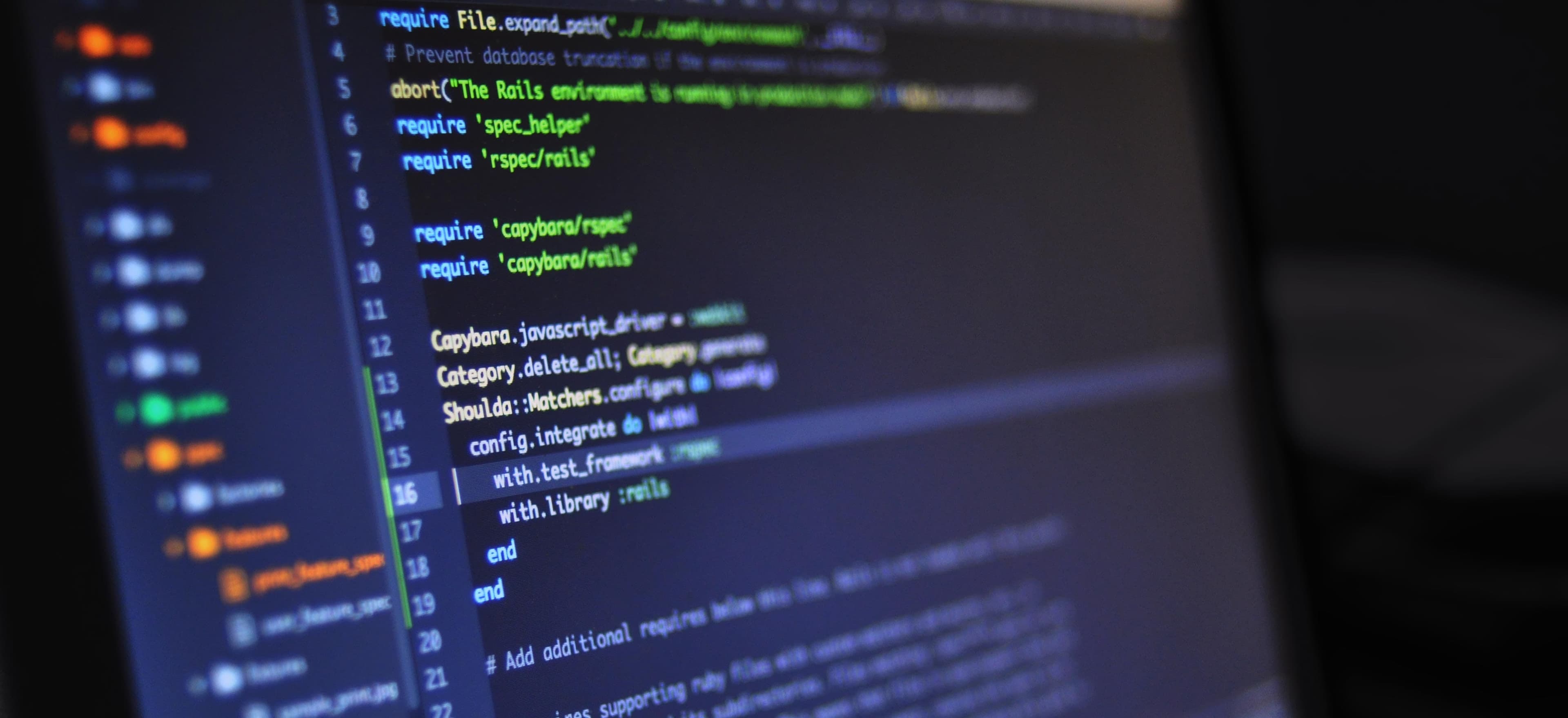
- Published on
Common Pitfalls When Setting Up JNDI with Embedded Jetty
Java Naming and Directory Interface (JNDI) is a powerful API that allows Java applications to access naming and directory services dynamically. When combined with Embedded Jetty, it provides a lightweight and straightforward setup for web applications. However, several common pitfalls can arise when setting up JNDI with Embedded Jetty.
In this blog post, we will explore these pitfalls and provide solutions to avoid them. This will help you streamline your development process and ensure a smooth integration of JNDI with Embedded Jetty.
Understanding JNDI and Embedded Jetty
Before diving into the common pitfalls, let’s take a moment to understand what JNDI and Embedded Jetty are.
-
JNDI is primarily used to look up objects such as data sources, EJBs, and JMS resources in a directory service. It is essential for accessing resources defined in applications.
-
Embedded Jetty is a Java-based HTTP server and servlet container that can be embedded in applications. It simplifies testing and development processes by eliminating the need for a separate application server.
Setting up JNDI in an Embedded Jetty environment enables you to leverage the directory services’ advantages, but improper configuration can lead to various issues.
Common Pitfalls When Setting Up JNDI with Embedded Jetty
1. Incorrect JNDI Resource Naming
One of the most common mistakes developers make is inconsistent or incorrect naming of the JNDI resources. If your application, JNDI config, and the environment do not align, you will face lookup issues.
Example
Context initialContext = new InitialContext();
DataSource ds = (DataSource) initialContext.lookup("java:comp/env/jdbc/myDataSource");
Why? If "jdbc/myDataSource" does not match the JNDI entry defined in the web.xml or other configuration files, this lookup will fail, resulting in a ContextNotFoundException.
Solution
Ensure that the JNDI resource name matches exactly with what your server and the application declare. Maintain a standardized naming convention.
2. Missing Resource Configuration in the Jetty XML
Another pitfall is the absence of resource definitions in your Jetty XML configuration file. Without proper declarations, Jetty has no idea about the resources your application intends to access.
Example
<Configure id="Server" class="org.eclipse.jetty.server.Server">
<Call name="addHandler">
<Arg>
<New class="org.eclipse.jetty.webapp.WebAppContext">
<Set name="contextPath">/myapp</Set>
<Set name="war"><SystemProperty name="jetty.home" default="."/>/myapp.war</Set>
<Set name="JNDI">
<New class="org.eclipse.jetty.jndi.InitialContextFactory">
<Set name="java:comp/env/jdbc/myDataSource">
<New class="org.h2.jdbcx.JdbcDataSource">
<Set name="URL">jdbc:h2:mem:testdb</Set>
<Set name="User">sa</Set>
<Set name="Password"></Set>
</New>
</Set>
</New>
</Set>
</New>
</Arg>
</Call>
</Configure>
Why? If the JNDI context and resource are not defined within Jetty's configuration, data sources or other resources may not be available during runtime, causing NullPointerExceptions or similar failures.
Solution
Always include the necessary resource configurations in your Jetty XML file. Be meticulous when defining your resources to ensure they match your application’s needs.
3. ClassLoader Issues
ClassLoader issues often arise when using JNDI in an embedded setup. If the correct ClassLoader is not provided, the JNDI lookups may fail.
Example
ClassLoader currentClassLoader = Thread.currentThread().getContextClassLoader();
Thread.currentThread().setContextClassLoader(getClass().getClassLoader());
Context context = new InitialContext();
Thread.currentThread().setContextClassLoader(currentClassLoader);
Why? The class loader used to load your application classes may not be the same as the one used for JNDI lookups, leading to ClassNotFoundExceptions.
Solution
Make sure to set the context ClassLoader appropriately, as shown above. This helps in bridging any gaps that arise from differing ClassLoaders.
4. Not Handling Exceptions Properly
Failing to handle JNDI-related exceptions can lead to runtime failures and obscure error reporting.
Example
try {
Context ctx = new InitialContext();
DataSource ds = (DataSource) ctx.lookup("java:comp/env/jdbc/myDataSource");
} catch (NamingException e) {
e.printStackTrace(); // Not the best practice
}
Why? Simply printing the stack trace may not log the error in a way that is easy to understand or diagnose later.
Solution
Implement proper logging strategies that capture more details when exceptions occur. Utilize logging frameworks like SLF4J or Log4j to log exceptions effectively.
5. Using Default Resource Configuration
Embedded Jetty uses default configurations that don't always align with what your application requires. Relying too heavily on defaults can lead to subtle bugs and errors.
Example
Server server = new Server(8080);
By default, Embedded Jetty will configure itself in basic modes that might not cater to your application’s JNDI needs.
Solution
Review and explicitly set configurations to suit your requirements instead of relying on defaults. Customize resources, ports, and handlers to improve performance and reliability.
In Conclusion, Here is What Matters
Setting up JNDI with Embedded Jetty provides a plethora of benefits that can help enhance your Java applications. However, understanding the common pitfalls—like incorrect naming, missed configurations, ClassLoader issues, poor exception handling, and reliance on defaults—will enhance your application’s robustness.
By paying attention to these potential issues and applying the suggested solutions, you can streamline the integration process. Properly configured JNDI resources will create a stable environment for your application, paving the way for better scalability and maintainability.
Additional Resources
For more in-depth guidance on JNDI and Embedded Jetty configurations, consider checking out the following resources:
- Jetty Documentation
- Oracle JNDI Documentation
With careful planning and execution, you can leverage JNDI with Embedded Jetty effectively, avoiding common traps along the way. Happy coding!
Checkout our other articles