Overcoming Actor Congestion in Akka Systems: A Guide
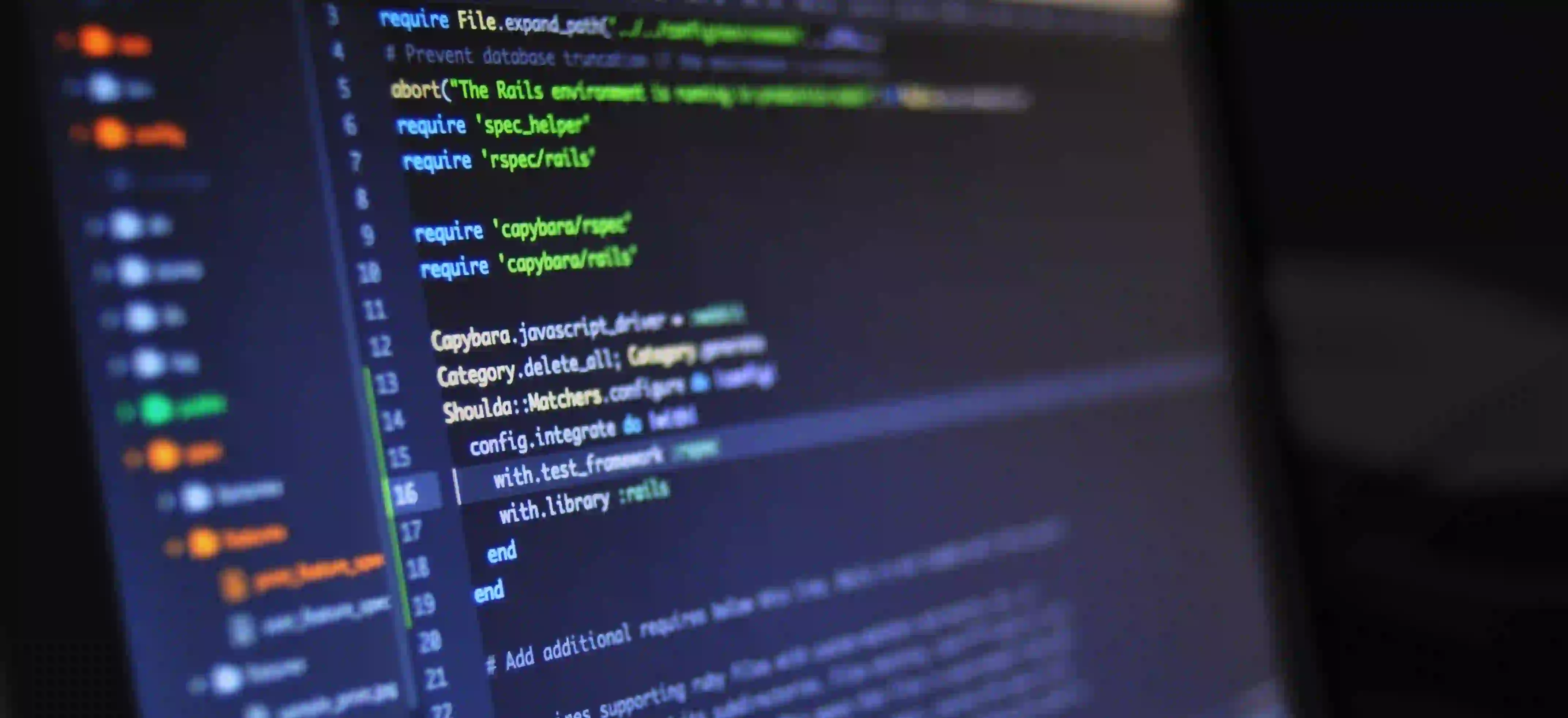
Overcoming Actor Congestion in Akka Systems: A Guide
Akka, the popular toolkit for building concurrent and distributed applications on the JVM, uses the Actor model, promoting an easy way to handle concurrency. However, developers may face a critical issue known as actor congestion. This phenomenon can lead to performance degradation, affecting your entire application. Here, we will delve into understanding actor congestion, its implications, and various strategies to overcome it.
What is Actor Congestion?
Actor congestion refers to a scenario where the processing rate of actors is slowed down due to high message traffic. When one actor receives messages faster than it can process them, it may lead to:
- Increased latency in message processing.
- Memory bloat, as messages queue up.
- Potential actor failures due to overwhelming load.
How Actor Congestion Occurs
To understand actor congestion, it is essential to recognize how the Akka system manages messages. Each actor has a mailbox where incoming messages wait to be processed. If a steady influx of messages exceeds the actor's processing speed, these mailboxes can overflow, contributing to congestion.
Identifying Congestion
Before diving into solutions, you must diagnose whether your Akka application is suffering from actor congestion. Here are some markers to watch for:
- Slow response times in your application’s API endpoints.
- High CPU usage with little to no throughput.
- Large unprocessed message queues in the actor system.
Monitoring Tools
Utilizing monitoring tools can help to observe the performance of your actors and identify bottlenecks. Tools like Akka Monitoring can provide insight into message queues and actor system health.
Strategies to Overcome Actor Congestion
1. Increase Actor Capacity
Increasing the throughput of your actor could be one of the simplest solutions. You can create more instances of the same actor, distributing the load among them.
Example Code Snippet
import akka.actor.{Actor, ActorSystem, Props}
// Define the actor
class Worker extends Actor {
def receive: Receive = {
case msg: String => process(msg)
}
private def process(msg: String): Unit = {
// Simulate processing
Thread.sleep(50)
println(s"Processed message: $msg")
}
}
// Create more workers
val system = ActorSystem("MySystem")
val workers = (1 to 10).map(_ => system.actorOf(Props[Worker]()))
workers.foreach { worker =>
worker ! "Hello, Actor!"
}
Why this Works: By creating multiple instances of the worker actor, you horizontally scale your system, allowing concurrent processing of messages, effectively reducing congestion.
2. Use Router Patterns
The Router pattern can also be an effective approach to distribute messages among actors efficiently. Akka provides built-in routers which front an actor pool and balance the load among them.
Example Code Snippet
import akka.actor.{ActorSystem, Props}
import akka.routing.{RoundRobinPool}
// Define a worker actor
class Worker extends Actor {
def receive: Receive = {
case msg =>
// Processing logic
println(s"Processing message: $msg")
}
}
// Create a router with multiple workers
val system = ActorSystem("RouterSystem")
val workerRouter = system.actorOf(RoundRobinPool(5).props(Props[Worker]))
for (i <- 1 to 50) {
workerRouter ! s"Message $i"
}
Why this Works: The Round Robin router distributes messages evenly across multiple worker actors, alleviating congestion in high-load scenarios.
3. Batched Processing
Processing messages in batches can improve throughput by reducing the number of context switches and enhancing cache locality.
Example Code Snippet
class BatchProcessor extends Actor {
private var messages: List[String] = List()
def receive: Receive = {
case msg: String =>
messages = msg :: messages
if (messages.size >= 10) {
processBatch(messages)
messages = List() // Clear the batch after processing
}
}
private def processBatch(batch: List[String]): Unit = {
// Process messages in batch
println(s"Processing batch: $batch")
}
}
Why this Works: Batching reduces the overhead of repeatedly entering and exiting the actor context for each message, increasing efficiency dramatically.
4. Backpressure Mechanism
Implementing a backpressure mechanism allows you to signal upstream components to slow down the message production rate when the consumer actor is overwhelmed. Akka Streams provides a backpressure mechanism that can be utilized to mitigate actor congestion.
Example Code Snippet
import akka.stream.scaladsl.{Flow, Sink, Source}
// Define a source generating messages
val source = Source(1 to 1000)
val workerFlow = Flow[Int].map { number =>
// Simulate long processing time
Thread.sleep(10)
s"Processed number: $number"
}
// Add backpressure with a sink that limits the flow
val sink = Sink.foreach[String](println)
source.via(workerFlow).to(sink).run()
Why this Works: By managing the flow of messages with backpressure, you can prevent overwhelming your actors, ensuring steady processing without congestion.
5. Actor Separation and Decoupling
If an actor is responsible for too many tasks, consider separating those responsibilities into dedicated actors. This decouples function and can lead to a more manageable flow.
Example Code Snippet
class RequestHandler extends Actor {
val processor1 = context.actorOf(Props[Processor1])
val processor2 = context.actorOf(Props[Processor2])
def receive: Receive = {
case request: Request =>
// Logic to decide which processor handles it
processor1 ! request
}
}
class Processor1 extends Actor {
def receive: Receive = {
case request: Request =>
// Processing logic
}
}
class Processor2 extends Actor {
def receive: Receive = {
case request: Request =>
// Processing logic
}
}
Why this Works: By splitting responsibilities, different actors can focus on specific tasks, reducing the message processing load per actor, and thus helping alleviate congestion.
My Closing Thoughts on the Matter
Actor congestion is a pressing issue for many developers utilizing the Akka framework, but with the right strategies, it can be effectively mitigated. By increasing actor capacity, employing router patterns, batching messages, implementing backpressure, and decoupling responsibilities, you can sustain a high-performing Akka system.
The techniques discussed in this guide are just the beginning. For further exploration of these strategies, consider reading more about Akka’s routing capabilities or backpressure in Akka Streams.
Happy coding, and may your Akka systems always run smoothly without congestion!