Common Pitfalls in Automation Testing Beginners Face
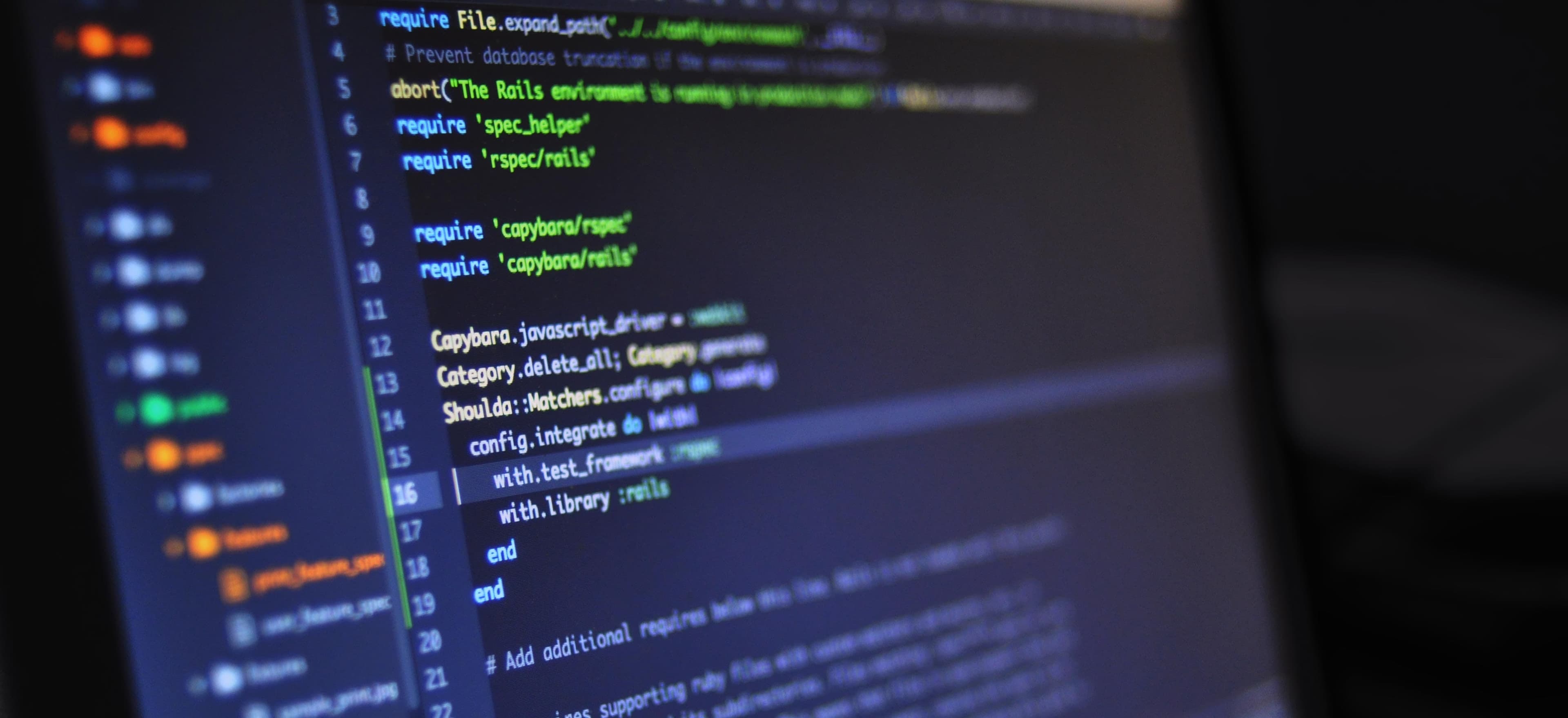
- Published on
Common Pitfalls in Automation Testing Beginners Face
Automation testing is a critical component of the software development lifecycle, offering benefits like increased efficiency, reduced manual errors, and faster feedback loops. However, beginners often encounter several hurdles when they start their journey into automation testing. This blog post discusses these common pitfalls and offers guidance on how to avoid them.
1. Lack of Clear Objectives
One of the primary pitfalls beginners face is not defining clear objectives for automation testing. Without a clear understanding of what you aim to achieve, your efforts can become unfocused.
Why It Matters
When objectives are vague, it's challenging to measure success and effectiveness. Clear goals set the direction for your testing strategy.
Example Objective:
To reduce the regression testing time from two weeks to one week.
Actionable Tip
Before automating tests, spend time discussing and defining your objectives with your team. Make sure that everyone is on the same page regarding what needs to be automated and why.
2. Choosing the Wrong Tools
Another common issue that beginners encounter is choosing the wrong testing tools for their automated testing needs. The plethora of tools available can be overwhelming.
Why It Matters
If you choose an unsuitable tool, it could lead to cumbersome implementations and may not support the technologies you are working with. For instance, a tool that primarily focuses on web applications may not be suitable for a mobile application.
Actionable Tip
Evaluate the tools based on the technology stack, team skill levels, and community support. Some popular options include:
- Selenium - Ideal for browser-based applications.
- Appium - Best for mobile applications.
- JUnit - Great for Java unit testing.
Learn more about selecting the right testing tools here.
3. Ignoring Test Maintenance
Test maintenance is often neglected by beginners, leading to a brittle test suite that breaks frequently and undermines its reliability.
Why It Matters
Code changes often lead to tests becoming obsolete or failing unnecessarily. If you've built a massive library of tests and they begin to fail, it can become a frustrating experience and result in reduced confidence in your automated tests.
Example Code Snippet
// A simple test method in JUnit
@Test
public void testAddition() {
assertEquals("This should return the sum of two numbers", 5, add(2, 3));
}
Commentary
In this code, our test method checks the addition function. But as our application changes, if the add method or its parameters change, our tests may need adjustments too. Always keep tests in sync with code changes.
Actionable Tip
Establish a routine for maintaining your test cases. Dedicate time to revisiting and updating tests following any significant changes to your application.
4. Writing Tests That Are Too Complex
Complex test scripts can cause confusion among team members and increase the maintenance burden.
Why It Matters
When tests are overly complicated, they can be hard to understand and difficult to fix when they fail. This can lead to a situation where no one wants to work on them, which effectively negates your investment in automation.
Actionable Tip
Strive to keep your tests simple and understandable. Use the Arrange-Act-Assert paradigm when writing tests:
@Test
public void testGetUserName() {
// Arrange
User user = new User("JohnDoe");
// Act
String userName = user.getUserName();
// Assert
assertEquals("The username should be JohnDoe", "JohnDoe", userName);
}
Commentary
In this example, the test is straightforward, clearly showing what is being tested and why. Beginners should strive to write tests that anyone on the team can read and understand.
5. Not Involving Stakeholders
Oftentimes, beginners isolate themselves and do not involve enough stakeholders in the automation process.
Why It Matters
Engagement from developers, testers, and product owners yields a more comprehensive testing approach. Failing to include these stakeholders can lead to misaligned expectations concerning test coverage and functionality.
Actionable Tip
Hold regular meetings with all stakeholders to align on priorities and scope for automation. Collecting feedback from diverse roles will ensure that automation efforts adequately cover all aspects of the application.
6. Over-Automating Tests
Automation is fantastic, but overdoing it can lead to an unwieldy suite of tests that are less effective.
Why It Matters
It's essential to automate the tests that provide the most value. Automating every conceivable test, including edge cases or those that rarely run, will introduce unnecessary complexity and maintenance.
Actionable Tip
Focus on automating the following types of tests:
- Regression Tests: Ensure new changes don't break existing functionality.
- Smoke Tests: Verify that the most crucial functions work after a new deployment.
Discover more about the balance of manual and automation testing here.
7. Underestimating the Learning Curve
Many beginners underestimate the time needed to become proficient with automation testing tools and frameworks.
Why It Matters
Not allowing the time to learn can lead to frustration and eventually results in subpar testing. Just like any other skill, automation testing requires practice and ongoing education.
Actionable Tip
Invest in training and self-education. Follow courses, read blogs, and join forums dedicated to automation testing. Consider using platforms such as Udemy or Coursera for structured learning.
8. Failure to Integrate with CI/CD
Continuous Integration (CI) and Continuous Deployment (CD) serve as backbones for modern development practices. Beginners often neglect integrating their tests into these pipelines.
Why It Matters
Without integration, automated tests cannot provide timely feedback, and you may miss defects early in the development process.
Actionable Tip
Explore tools that facilitate integration, such as Jenkins or GitLab CI. Automate build and deployment processes that include running your test suite.
Example CI/CD Script Snippet:
# Sample GitLab CI pipeline
stages:
- build
- test
build_job:
stage: build
script:
- mvn clean install
test_job:
stage: test
script:
- mvn test
Commentary
In this basic CI/CD configuration, we define two stages: build and test. This guarantees that whenever there is a code change, the build and tests will automatically run, promoting robust development practices.
The Bottom Line
While automation testing offers numerous benefits, beginners should be mindful of potential pitfalls. By avoiding common mistakes and following best practices, one can harness the advantages of automated testing effectively.
By focusing on clear objectives, choosing the right tools, maintaining tests, and collaborating with stakeholders, you will build a solid foundation for your automation testing efforts.
Embrace learning, facilitate CI/CD integration, and evolve your methodology as you gain experience. Your understanding and capabilities will expand, positioning you to deliver robust, efficient testing solutions.
For any questions, tips, or shared experiences related to automation testing, feel free to drop your comments below!