Maximizing Cloud Bigtable Write Efficiency: Common Pitfalls
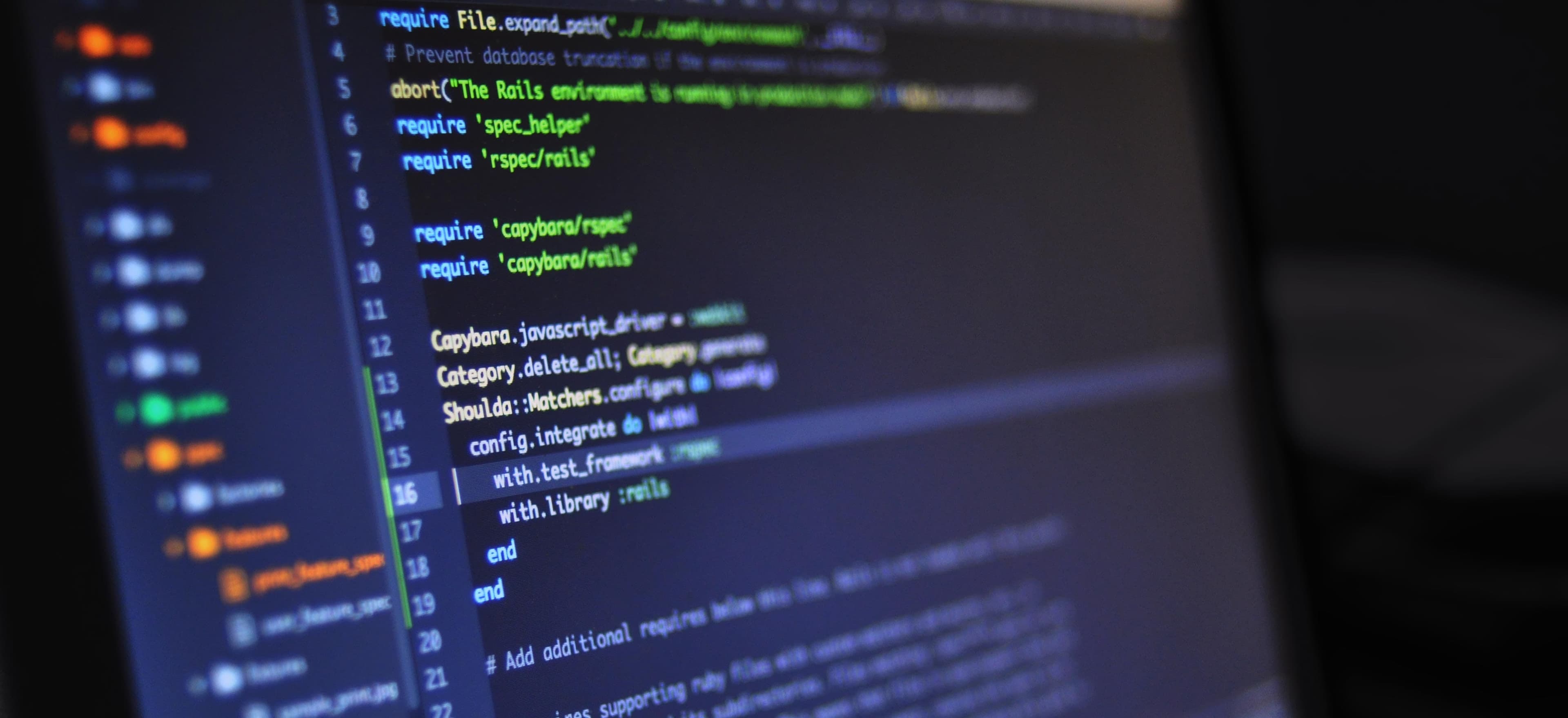
- Published on
Maximizing Cloud Bigtable Write Efficiency: Common Pitfalls
Google Cloud Bigtable is a powerful, fully managed, petabyte-scale NoSQL database designed for large analytical and operational workloads. Its performance relies heavily on how data is written to the table. While Bigtable is engineered to handle high write loads, there are common pitfalls that developers often encounter that can impede write efficiency. This blog post will delve into these pitfalls, offering actionable solutions to enhance write efficiency.
Understanding Cloud Bigtable Architecture
Before diving into the pitfalls, it’s essential to understand the fundamental architecture of Bigtable. Bigtable uses a sparse, distributed, multi-dimensional sorted map. The dimensions of this map are your rows, clusters, and columns, and the data is stored in tablets that are split across multiple servers. This architecture allows Bigtable to efficiently scale horizontally, but it also means that inefficient write paths can lead to significant performance degradation.
Common Pitfalls and Their Solutions
1. Poor Row Key Design
The Issue: The row key is the primary identifier for data in Bigtable. Its design directly affects performance. Choosing a monotonically increasing key (e.g., timestamps) leads to "hotspots" where writes get concentrated in a single tablet, causing throttled writes and increased latency.
The Solution: Instead, opt for a more distributed row key scheme. One popular approach is to add a random prefix to your row identifier.
Example Code Snippet:
import java.util.Random;
public class RowKeyGenerator {
private static final Random random = new Random();
public static String generateRowKey(String id) {
// Prepend a random number to distribute writes
int randomNum = random.nextInt(10000);
return randomNum + "_" + id;
}
}
Why This Works: By introducing randomness in the row key, the writes are spread across more tablets, preventing hotspots and improving write throughput.
2. Large Write Batches
The Issue: Sending data in large write batches can seem efficient, but if poorly sized, it can overwhelm Bigtable. Very large batches increase the risk of timeouts and throttled operations.
The Solution: Implement a strategy to send fixed-size batches, optimizing the batch size based on your workload’s characteristics.
Example Code Snippet:
import com.google.cloud.bigtable.data.v2.BigtableDataClient;
import com.google.cloud.bigtable.data.v2.models.RowMutation;
import java.util.ArrayList;
import java.util.List;
public class BatchWriter {
private final BigtableDataClient client;
private static final int BATCH_SIZE = 100;
public BatchWriter(BigtableDataClient client) {
this.client = client;
}
public void writeData(List<RowMutation> mutations) {
List<RowMutation> batch = new ArrayList<>();
for (RowMutation mutation : mutations) {
batch.add(mutation);
if (batch.size() >= BATCH_SIZE) {
client.mutateRows(batch);
batch.clear();
}
}
// Commit any remaining mutations
if (!batch.isEmpty()) {
client.mutateRows(batch);
}
}
}
Why This Works: This method helps ensure that each batch sent to Bigtable is optimal in size, minimizing the likelihood of experiencing write latency spikes due to overwhelming loads.
3. Excessive Small Writes
The Issue: While frequent writes might seem pragmatic, excessively small writes can lead to performance issues. Each write operation incurs overhead, so if you are writing very small pieces of data, this can turn into a bottleneck.
The Solution: Instead of writing small records independently, consolidate multiple write operations into a single batch.
When to Use: If you find yourself writing each piece of data individually (e.g., logging events), aggregate those events before writing.
Example Code Snippet:
import com.google.cloud.bigtable.data.v2.models.RowMutation;
public class EventLogger {
public void logEvent(List<Event> events) {
RowMutation mutation = new RowMutation("combined_logs");
for (Event event : events) {
// Assume Event has a method to convert to a string
mutation.setCell("events", event.getId(), event.toString());
}
// Now, write a single operation for all events
client.mutateRow(mutation);
}
}
Why This Works: This method minimizes the number of write operations, optimizing overall throughput by aggregating data writes.
4. Ignoring Write Throughput Capacity
The Issue: Each Cloud Bigtable instance has throughput limitations. Writing data at a rate that exceeds the provisioned throughput can lead to throttling and increased write latency.
The Solution: Monitor your write throughput and adjust your design to stay within limits, scaling horizontally by adding more nodes if necessary.
Usage of Monitoring Tools: Consider integrating Stackdriver Monitoring or your preferred analytics to visualize your write throughput.
Read more about monitoring and scaling Bigtable here.
5. Not Taking Advantage of Bulk Loading
The Issue: When ingesting large datasets, certain developers overlook the option of bulk loading, which can significantly reduce write times compared to standard write operations.
The Solution: Use Cloud Bigtable's bulk loading feature to upload larger datasets efficiently.
Implementation Slant: While this approach might require initial setup or preprocessing of data files (e.g., exporting data from other systems), it pays off in the long run regarding write speeds.
In Conclusion, Here is What Matters
Maximizing write efficiency in Cloud Bigtable is vital for scalable and performant applications. By being aware of common pitfalls—like row key design, batch sizes, and excessive small writes—you can improve your system's throughput, minimize latency, and enhance user experiences.
Building a well-architected data write strategy is fundamental. Whether you consolidate writes, design row keys wisely, or make use of bulk loaders, these practices will save you time, resources, and headaches in the long run. By addressing these pitfalls strategically, you'll be positioned to leverage Cloud Bigtable to its full potential.
To further enhance your productivity, consider utilizing tools and resources provided by Google Cloud. Monitoring your writing patterns can provide invaluable insights that will help refine your approaches as you scale your operations.
With these insights in hand, developers can confidently navigate the challenges associated with Bigtable's write operations and harness its full potential! Happy coding!
Checkout our other articles