Transitioning from XML to JavaConfig in Spring Batch Jobs
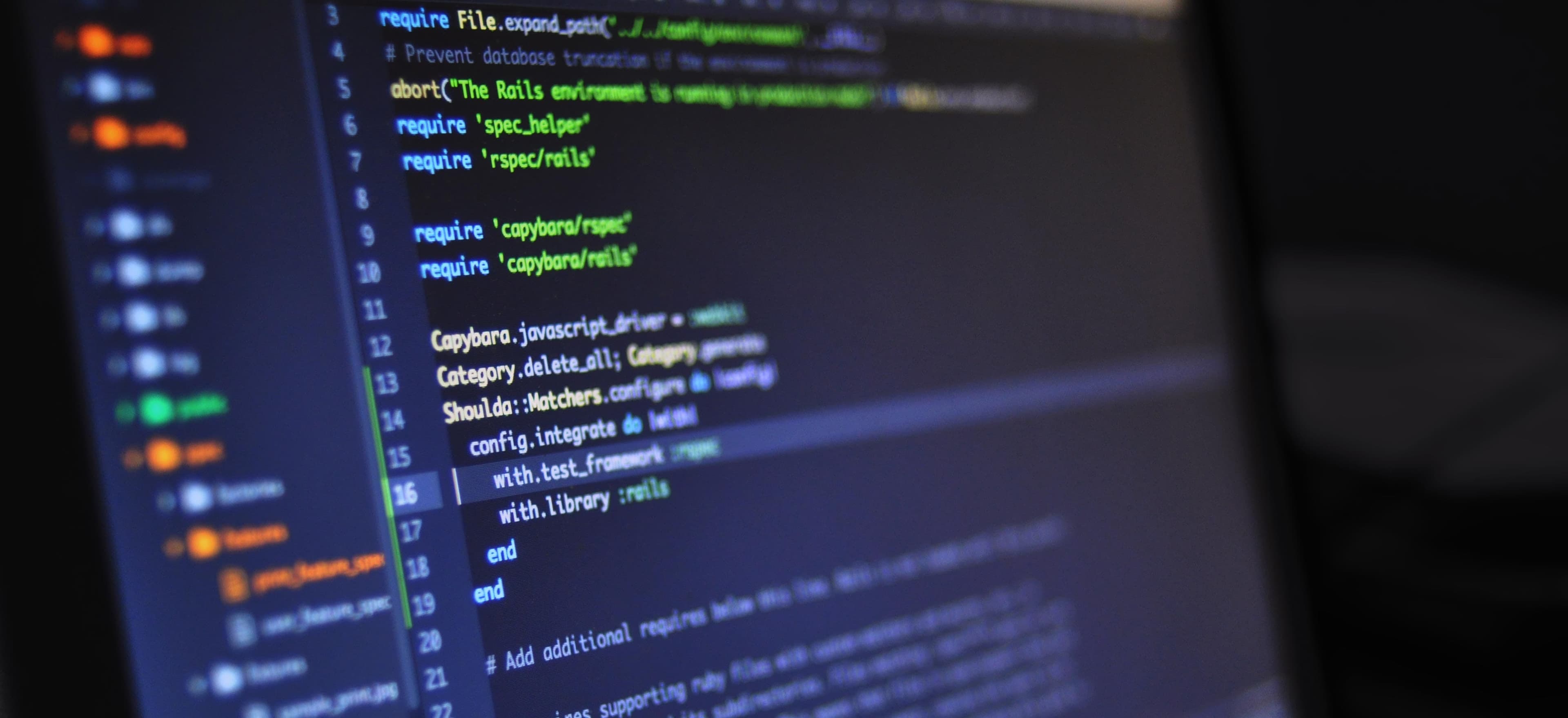
- Published on
Transitioning from XML to JavaConfig in Spring Batch Jobs
Spring Batch is a powerful framework for batch processing in Java. Traditionally, configuration was done using XML files, but with the advent of JavaConfig, developers can leverage type-safe configuration, reduced boilerplate, and better integration with Java applications. In this blog post, we will explore how to transition from XML to JavaConfig in Spring Batch jobs, enhancing both readability and maintainability.
Why Transition from XML to JavaConfig?
- Type Safety: JavaConfig provides compile-time checks, reducing runtime errors related to misconfigurations.
- IDE Support: Refactoring and code navigation benefits from your IDE become available.
- Consistency: Having configurations in Java means consistency with the rest of your Java-based applications.
- Readability: JavaConfig can lead to more readable configurations, making it easier for developers to understand how jobs are set up.
Overview of a Spring Batch Job Configuration
Before we dive into the specifics of moving from XML to JavaConfig, let’s outline what a Spring Batch job typically consists of:
- Job: The overarching task to be performed.
- Step: A phase of the job that processes data.
- ItemReader: Reads data from a source.
- ItemProcessor: Processes the data.
- ItemWriter: Writes the processed data to a destination.
Example XML Configuration
Here’s a simple XML configuration for a Spring Batch job:
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd">
<job id="importJob">
<step id="step1">
<tasklet>
<chunk reader="itemReader" processor="itemProcessor" writer="itemWriter" commit-interval="10"/>
</tasklet>
</step>
</job>
<bean id="itemReader" class="org.springframework.batch.item.file.FlatFileItemReader">
<property name="resource" value="classpath:input.csv"/>
<property name="lineMapper">
<bean class="org.springframework.batch.item.file.mapping.DefaultLineMapper">
<property name="fieldSetMapper">
<bean class="com.example.MyItemMapper"/>
</property>
</bean>
</property>
</bean>
<bean id="itemProcessor" class="com.example.MyItemProcessor"/>
<bean id="itemWriter" class="org.springframework.batch.item.file.FlatFileItemWriter">
<property name="resource" value="classpath:output.csv"/>
<property name="lineAggregator">
<bean class="org.springframework.batch.item.file.transform.PassThroughLineAggregator"/>
</property>
</bean>
</beans>
Transitioning to JavaConfig
Now, let’s translate this XML configuration into JavaConfig.
Step 1: Create a Configuration Class
Create a configuration class annotated with @Configuration
. This class will hold all your Spring Batch configurations.
import org.springframework.batch.core.Job;
import org.springframework.batch.core.Step;
import org.springframework.batch.core.configuration.annotation.EnableBatchProcessing;
import org.springframework.batch.core.configuration.annotation.JobBuilderFactory;
import org.springframework.batch.core.configuration.annotation.StepBuilderFactory;
import org.springframework.batch.item.ItemProcessor;
import org.springframework.batch.item.ItemReader;
import org.springframework.batch.item.ItemWriter;
import org.springframework.batch.item.file.FlatFileItemReader;
import org.springframework.batch.item.file.FlatFileItemWriter;
import org.springframework.batch.item.file.mapping.DefaultLineMapper;
import org.springframework.batch.item.file.transform.PassThroughLineAggregator;
import org.springframework.batch.item.file.transform.LineAggregator;
import org.springframework.batch.item.file.transform.FieldSetMapper;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.core.io.ClassPathResource;
@Configuration
@EnableBatchProcessing
public class BatchConfig {
@Autowired
public JobBuilderFactory jobBuilderFactory;
@Autowired
public StepBuilderFactory stepBuilderFactory;
@Bean
public Job importJob() {
return jobBuilderFactory.get("importJob")
.incrementer(new RunIdIncrementer())
.flow(step1())
.end()
.build();
}
@Bean
public Step step1() {
return stepBuilderFactory.get("step1")
.<InputType, OutputType>chunk(10)
.reader(itemReader())
.processor(itemProcessor())
.writer(itemWriter())
.build();
}
@Bean
public FlatFileItemReader<InputType> itemReader() {
FlatFileItemReader<InputType> reader = new FlatFileItemReader<>();
reader.setResource(new ClassPathResource("input.csv"));
reader.setLineMapper(lineMapper());
return reader;
}
@Bean
public DefaultLineMapper<InputType> lineMapper() {
DefaultLineMapper<InputType> lineMapper = new DefaultLineMapper<>();
lineMapper.setFieldSetMapper(myItemMapper());
// Add additional configuration if needed
return lineMapper;
}
@Bean
public FieldSetMapper<InputType> myItemMapper() {
return new MyItemMapper();
}
@Bean
public ItemProcessor<InputType, OutputType> itemProcessor() {
return new MyItemProcessor();
}
@Bean
public FlatFileItemWriter<OutputType> itemWriter() {
FlatFileItemWriter<OutputType> writer = new FlatFileItemWriter<>();
writer.setResource(new ClassPathResource("output.csv"));
writer.setLineAggregator(lineAggregator());
return writer;
}
@Bean
public LineAggregator<OutputType> lineAggregator() {
return new PassThroughLineAggregator<>();
}
}
Breakdown of the Code
- Job Configuration: The job is defined with
.incrementer(new RunIdIncrementer())
to ensure each execution is unique. - Step Definition: Each step is defined with type parameters
<InputType, OutputType>
, facilitating type safety. - ItemReader Configuration: The
FlatFileItemReader
is set up withClassPathResource
, which is preferable to hardcoded paths. - Line Mapper: The
DefaultLineMapper
utilizes our customFieldSetMapper
, which you need to implement to define how each line of input is converted into an object.
Advantages of Using JavaConfig
Using JavaConfig eliminates issues common in XML such as the lack of compile-time checks. Also, code completion and IDE features significantly enhance the development experience.
Testing the JavaConfig
Once you have your JavaConfig set up, you can run your Spring Batch job just like before, but now with more robust, maintainable configuration.
Here’s a simple test case to run your job:
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.test.context.ActiveProfiles;
@SpringBootTest
@ActiveProfiles("test")
public class BatchJobTest {
@Autowired
private JobLauncherTestUtils jobLauncherTestUtils;
@Test
public void testJob() throws Exception {
JobExecution jobExecution = jobLauncherTestUtils.launchJob();
assertEquals(BatchStatus.COMPLETED, jobExecution.getStatus());
}
}
The Last Word
Transitioning from XML to JavaConfig in Spring Batch Jobs fosters a more modern, maintainable, and type-safe approach to job configuration. The example presented serves as a template. You can adapt it to fit your specific requirements, ensuring you enhance both your development workflow and the quality of your batch jobs.
For more in-depth insights on Spring Batch and JavaConfig, feel free to check the Spring Batch Documentation for additional configuration options and best practices.
Happy coding!