Troubleshooting SSEEmitter: Common RXJava Pitfalls
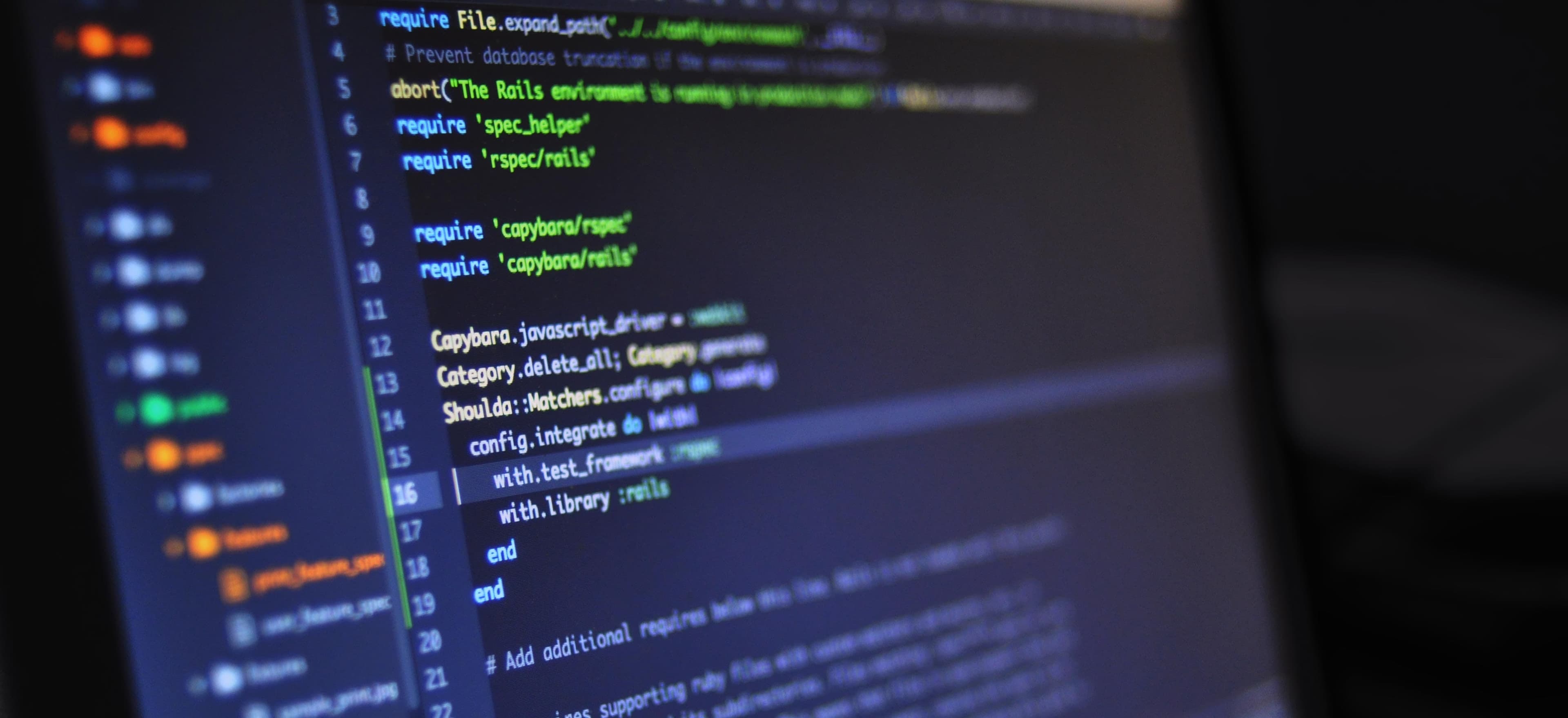
- Published on
Troubleshooting SSEEmitter: Common RXJava Pitfalls
Server-Sent Events (SSE) offer a powerful way to push updates from the server to the client in real-time. In the Java ecosystem, combining SSE with RXJava allows for reactive programming and simplifies the manipulation of asynchronous data streams. However, developers often encounter pitfalls when using SSEEmitter
in a Spring project with RXJava. In this blog post, we will explore common issues and solutions, while providing practical examples and best practices.
What is SSEEmitter?
The SSEEmitter
is a component of Spring Framework that allows developers to send SSE to clients. It can be incredibly useful for applications requiring real-time notifications, such as chat applications or live dashboards.
How SSEEmitter Works
When using SSEEmitter
, the server keeps a connection open with the client and streams events as they occur. The client listens for these events and processes them in real-time.
Here is a basic example of using SSEEmitter
:
@RestController
public class EventController {
@GetMapping("/events")
public SSEEmitter streamEvents() {
SSEEmitter emitter = new SSEEmitter();
Executors.newSingleThreadExecutor().submit(() -> {
try {
for (int i = 0; i < 10; i++) {
emitter.send("Event " + i);
Thread.sleep(1000);
}
emitter.complete();
} catch (Exception e) {
emitter.completeWithError(e);
}
});
return emitter;
}
}
Commentary:
In this example, an EventController
streams events to the client every second. The emitter.send()
method pushes the message, and the connection is maintained until the loop completes, at which point the call to emitter.complete()
closes the connection gracefully.
Common RXJava Pitfalls with SSEEmitter
While using SSEEmitter
with RXJava can simplify event management, several common pitfalls may arise during development:
1. Blocking the Main Thread
A common mistake when working with SSEEmitter
is blocking the main thread with long-running or synchronous operations. This can lead to timeouts and unhandled exceptions.
Solution: Use Background Threads
Always offload intensive operations to a separate thread. As shown in the previous example, using an ExecutorService
allows tasks to run asynchronously, preventing the main thread from being blocked.
2. Mismanaging Streams
It's crucial to manage the RXJava streams properly. Creating potentially infinite loops without control can lead to memory leaks and performance degradation.
Example of Event Stream Management:
Observable<String> eventStream = Observable.create(emitter -> {
for (int i = 0; i < 10; i++) {
emitter.onNext("Event " + i);
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
emitter.onError(e);
}
}
emitter.onComplete();
});
Commentary:
Here, an RXJava Observable
is created that pushes events every second. By using onNext()
to send data and onComplete()
to finish the stream, the code maintains control over the lifetime of the stream.
3. Not Handling Errors Gracefully
Ignoring error handling can lead to unexpected crashes. RXJava provides operators like onError
to handle exceptions.
Improved Error Handling Example:
eventStream
.subscribe(
event -> emitter.send(event),
error -> emitter.completeWithError(error),
() -> emitter.complete()
);
Commentary:
By subscribing to the eventStream
, you can define what happens on data (event
), handle errors (error
), and signify completion with a graceful exit (() -> emitter.complete()
).
4. Not Closing the Emitter
Failing to close the emitter can lead to hanging connections, resulting in resource leaks over time. Always ensure the emitter closes properly.
Properly Closing Emitter:
emitter.complete();
Call this method to signal that you are done sending data. It's a good practice to implement this within a finally
block or relevant error handling logic.
5. Overwhelming Clients with Events
If you are sending events too frequently, clients might become overwhelmed, leading to dropped messages or browser/browser tab crashes.
Solution: Throttling Events
Use operators such as debounce
or throttleFirst
from RXJava to limit how often events are sent.
eventStream
.debounce(1, TimeUnit.SECONDS)
.subscribe(emitter::send, emitter::completeWithError, emitter::complete);
This approach buffers events and sends the last event only after a specified duration of silence.
The Closing Argument
The SSEEmitter
combined with RXJava offers a robust solution for real-time applications, but developers must approach it with caution. By understanding and avoiding common pitfalls such as blocking the main thread, mismanaging streams, neglecting error handling, forgetting to close emitters, and flooding clients with events, you can create a stable and effective architecture.
Additional Resources
To learn more about reactive programming in Java with RXJava, check out these resources:
By following these guidelines and best practices, you can harness the full potential of SSEEmitter
and RXJava in your applications. Happy coding!
Checkout our other articles