Troubleshooting MQTT Connection Issues in IoT Projects
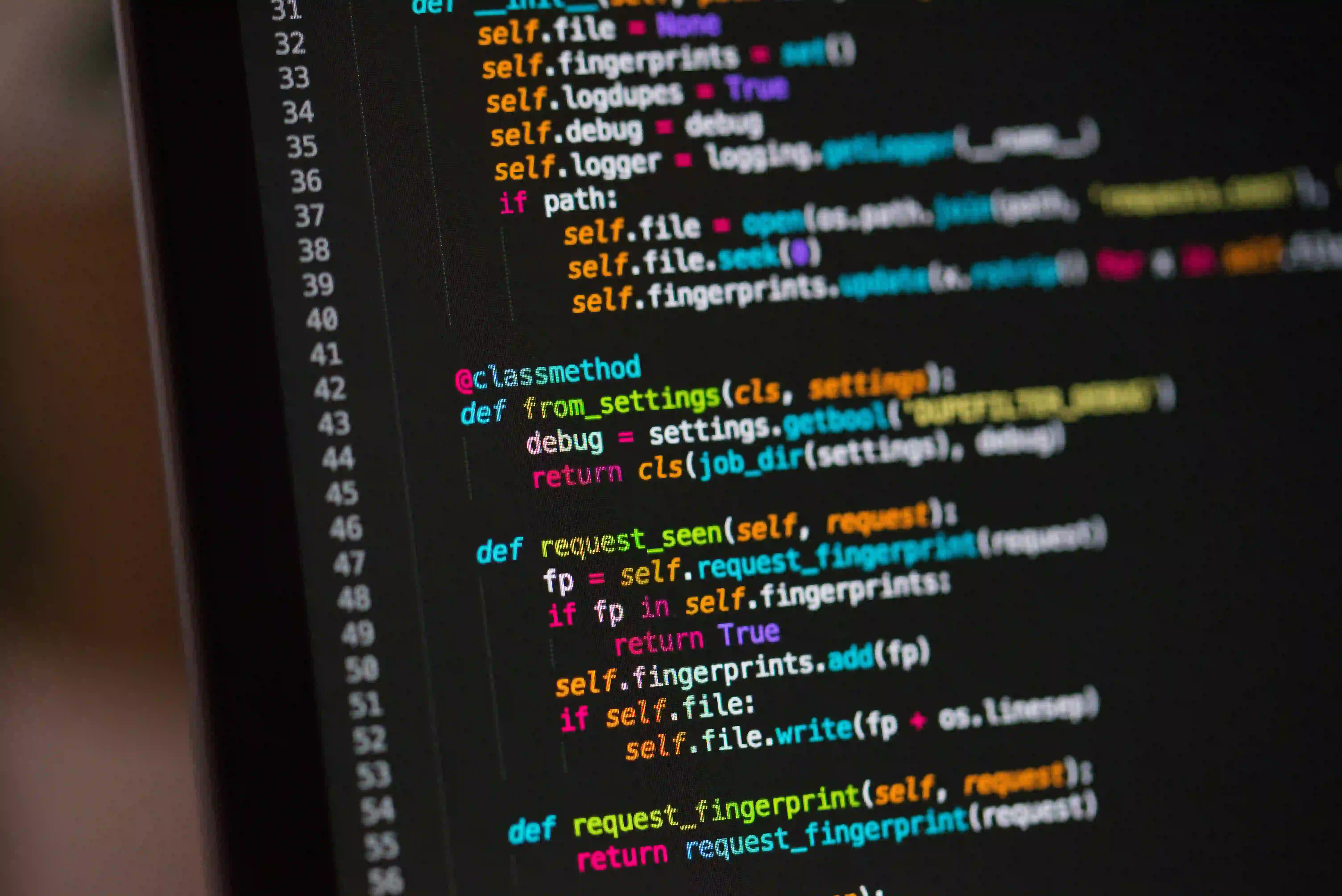
Troubleshooting MQTT Connection Issues in IoT Projects
In the expanding universe of Internet of Things (IoT), MQTT (Message Queuing Telemetry Transport) has emerged as a powerful communication protocol. It’s lightweight, efficient, and perfect for constrained devices and low-bandwidth, high-latency networks. However, like any technology, it can present connection challenges. This article will provide you with a structured approach to troubleshooting MQTT connection issues in your IoT projects.
Understanding MQTT
Before diving into troubleshooting, let's briefly review how MQTT works. It employs a client-server architecture where a broker mediates messages between clients. Devices (clients) publish messages on specific topics while other devices (subscribers) listen for messages on those topics.
Advantages of MQTT
- Low Bandwidth Usage: MQTT messages are small and efficient.
- Quality of Service Levels: MQTT offers three QoS levels, allowing flexibility in message delivery guarantees.
- Last Will Testament: This feature helps to notify other clients when a client disconnects unexpectedly.
For a deeper understanding of the MQTT protocol, the OASIS MQTT Protocol Specification offers extensive documentation.
Common Connection Issues
1. Incorrect Broker Configuration
Why it Matters
The broker is the heart of any MQTT communication setup. If the broker is misconfigured, clients will struggle to connect.
How to Troubleshoot
- Verify that the broker is running and accessible. Try pinging the broker's IP address or hostname.
- Double-check the broker's port number (default is 1883 for MQTT).
- Ensure that the broker configuration allows for the number of allowed clients and does not exceed the set limits.
// Example configuration for an MQTT client in Java
import org.eclipse.paho.client.mqttv3.MqttClient;
import org.eclipse.paho.client.mqttv3.MqttException;
public class MQTTConnection {
public static void main(String[] args) {
String broker = "tcp://broker.hivemq.com:1883"; // Ensure this is correct
String clientId = "JavaSampleClient";
try {
MqttClient client = new MqttClient(broker, clientId);
client.connect(); // This line must not throw an exception
System.out.println("Connected to broker.");
} catch (MqttException e) {
System.err.println("Error connecting to broker: " + e.getMessage()); // Catch connection issues
}
}
}
2. Network Issues
Why it Matters
IoT devices often operate on various networks, which can introduce instability or restrictions.
How to Troubleshoot
- Check Firewall Settings: Ensure that the necessary ports are open for outbound connections.
- Use Network Analysis Tools: Tools like Wireshark can help you analyze traffic and identify if messages are being blocked.
- Verify DNS Resolutions: Ensure that the hostname of your broker resolves correctly to an IP address.
// Network troubleshooting output using Java
if (!InetAddress.getByName("broker.hivemq.com").isReachable(2000)) {
System.err.println("Broker is unreachable! Check your network settings.");
}
3. Authentication and Authorization Failures
Why it Matters
Many MQTT brokers enforce security protocols that require users to authenticate and authorize access.
How to Troubleshoot
- Check Credentials: Make sure you are using the correct username and password.
- Inspect Broker Policies: Review roles and permissions to ensure the client has access to the requested topic.
// Example with username and password for secured MQTT connection
import org.eclipse.paho.client.mqttv3.MqttConnectOptions;
public class SecureMQTTConnection {
public static void main(String[] args) {
String broker = "tcp://broker.hivemq.com:1883";
String clientId = "JavaSecureClient";
String username = "user"; // Use real credentials
String password = "pass"; // Use real credentials
try {
MqttClient client = new MqttClient(broker, clientId);
MqttConnectOptions options = new MqttConnectOptions();
options.setUserName(username);
options.setPassword(password.toCharArray());
client.connect(options);
System.out.println("Securely connected to broker.");
} catch (MqttException e) {
System.err.println("Secure connection error: " + e.getMessage());
}
}
}
4. Quality of Service (QoS) Mismatches
Why it Matters
Understanding your QoS settings is critical as it directly influences message delivery reliability.
How to Troubleshoot
- Make sure that both publishers and subscribers are aligned on QoS levels.
- If lower QoS is being used on a subscriber, messages might get lost.
// Adjusting QoS in Java
try {
client.subscribe("topic/example", 1); // Subscribe with QoS level 1
} catch (MqttException e) {
System.err.println("Subscription error: " + e.getMessage());
}
5. Client Timeout and Heartbeat Intervals
Why it Matters
MQTT clients keep the connection alive using "keep-alive" messages. If timeouts aren’t managed correctly, clients can disconnect unexpectedly.
How to Troubleshoot
- Increase the keep-alive interval in the client settings.
- Monitor the connection status and reconnect as necessary.
// Adjusting keep-alive in Java
MqttConnectOptions options = new MqttConnectOptions();
options.setKeepAliveInterval(60); // Set keep-alive interval to 60 seconds
Summary
Troubleshooting MQTT connection issues can be a challenging yet rewarding aspect of managing IoT projects. By systematically exploring each potential issue—broker configuration, network problems, authentication errors, QoS mismatches, and client timeouts—you can gain invaluable insights into the nuances of MQTT connectivity.
For further reading on advanced MQTT functionality or scaling best practices in IoT implementations, consider checking out the HiveMQ MQTT Essentials series which provides insights into optimizing your applications.
As IoT continues to evolve, understanding and effectively managing MQTT connections will remain a fundamental skill for developers and engineers alike.