Troubleshooting Database Connectivity in Podman for Spring Boot
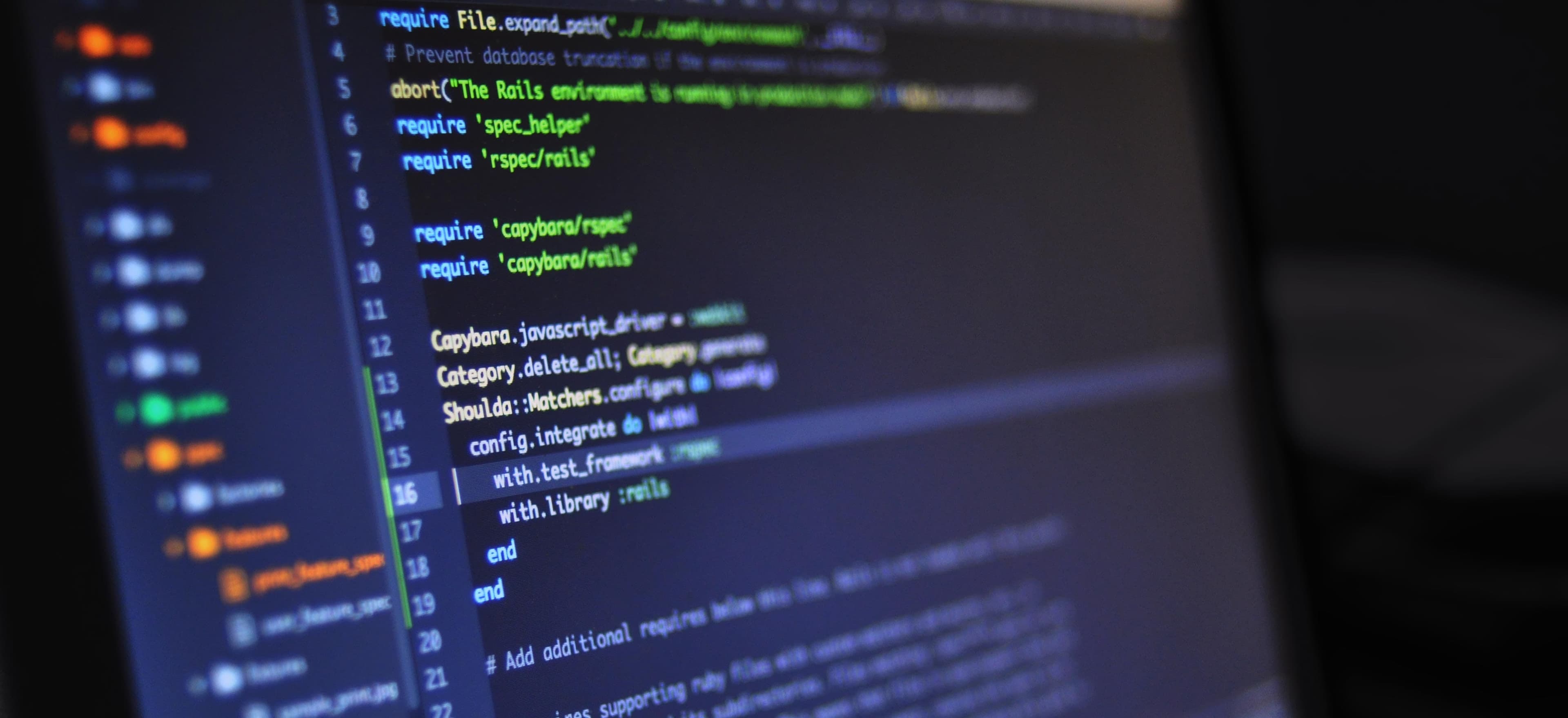
- Published on
Troubleshooting Database Connectivity in Podman for Spring Boot
Spring Boot has revolutionized the way Java applications are developed, making it easier to create stand-alone, production-grade applications. However, when you're running these applications in a containerized environment like Podman, you might encounter some database connectivity issues. In this blog post, we will explore common problems related to database connectivity in Spring Boot applications running on Podman, and how to troubleshoot them effectively.
Understanding Podman and Spring Boot
What is Podman?
Podman is a container management tool that allows you to run containers and manage images without the need for a daemon, making it lightweight and simple to use. It supports a variety of container images, including those for databases and web applications. Unlike Docker, Podman allows you to run containers as non-root users, enhancing security.
What is Spring Boot?
Spring Boot is a popular framework used in the Java ecosystem for building web applications. It simplifies the process by providing pre-configured templates, allowing developers to focus on writing business logic rather than dealing with the complexities of configuration.
Common Database Connectivity Issues
When you're running a Spring Boot application in a Podman container, you may run into several common database connectivity issues. Below are some scenarios you might encounter:
- Container Network Mismatch
- Database Service Not Running
- Incorrect Database Configuration
- Firewall Restrictions
- Driver Class Not Found
- Connection Pool Issues
Let's drill down into each of these issues.
1. Container Network Mismatch
When connecting to a database, your Spring Boot application should be able to reach the database service. Check if your database is running on the same network as your application container.
Quick Check
Run the following command to see active networks in Podman:
podman network ls
Ensure both your application and database containers are connected to the same network.
2. Database Service Not Running
This may seem trivial, but confirm that the database service is up and running. You can check the status of your Podman containers with:
podman ps
3. Incorrect Database Configuration
In your application.properties
or application.yml
file, make sure the database connection settings are correct. A simple misconfiguration can lead to connectivity issues.
spring.datasource.url=jdbc:mysql://db-server:3306/mydb
spring.datasource.username=myuser
spring.datasource.password=mypass
Why It Matters
Each property serves a specific purpose. The database URL should include the correct server and port number. If you're using a different database (like PostgreSQL), ensure you have the correct JDBC dependency in your pom.xml
.
4. Firewall Restrictions
Firewalls can block the database’s port, preventing access from your Spring Boot application. Check your firewall settings and ensure that the necessary ports are open.
Troubleshooting Steps
Use tools like telnet
to test connectivity from your application container to the database:
telnet db-server 3306
5. Driver Class Not Found
Ensure you have the correct JDBC driver dependency in your Maven or Gradle configuration. For instance, if you're using MySQL, add the following dependency:
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.27</version>
</dependency>
6. Connection Pool Issues
Spring Boot uses a connection pool to manage database connections, which can fail due to incorrect configurations. Check your connection pool settings in application.properties
.
spring.datasource.hikari.maximum-pool-size=10
This setting allows a maximum of 10 connections to the database.
Debugging Techniques
Now that we've gone over common issues, let's discuss how to debug problems effectively. Below are some integral debugging techniques.
1. Enable Detailed Logging
Spring Boot allows you to change log levels in application.properties
. Set the logging level for SQL statements to DEBUG:
logging.level.org.springframework.jdbc.core=DEBUG
logging.level.org.hibernate.SQL=DEBUG
2. Inspect Application Startup Logs
Upon starting your application with Podman, closely review the logs for any exceptions related to database connectivity. Addressing the root cause listed in the logs often leads you to a simple fix.
3. Use Podman Exec
You can enter your running container to debug directly. This allows you to use command-line tools from within your application container.
podman exec -it <container_id> /bin/bash
Once inside, test the database connection if a client is available.
Sample Code Snippet
Here is an example of a Spring Boot configuration for a MySQL database:
@Configuration
@EnableTransactionManagement
public class DataSourceConfig {
@Bean
public DataSource dataSource() {
HikariConfig hikariConfig = new HikariConfig();
hikariConfig.setJdbcUrl("jdbc:mysql://db-server:3306/mydb");
hikariConfig.setUsername("myuser");
hikariConfig.setPassword("mypass");
return new HikariDataSource(hikariConfig);
}
}
Why This Configuration Matters
Using HikariCP as a DataSource provides fast connection pooling. The JDBC URL points to the right database server, while the username and password provide necessary credentials for access. This ensures smooth communication between your application and the database.
A Final Look
Troubleshooting database connectivity in Spring Boot applications running on Podman requires a systematic approach. From checking network configurations to verifying dependencies and connection pools, each step is crucial in isolating the problem.
By implementing thorough logging, using debugging techniques, and ensuring all configurations are correct, you can resolve most issues effectively. For a deeper dive into Spring Boot and Podman practices, visit the official Spring documentation and the Podman user guide.
By being prepared and equipped with the right knowledge, you can ensure that your Spring Boot application communicates smoothly with its database, regardless of the environment in which it runs.