Common Pitfalls When Using RESTful API Clients
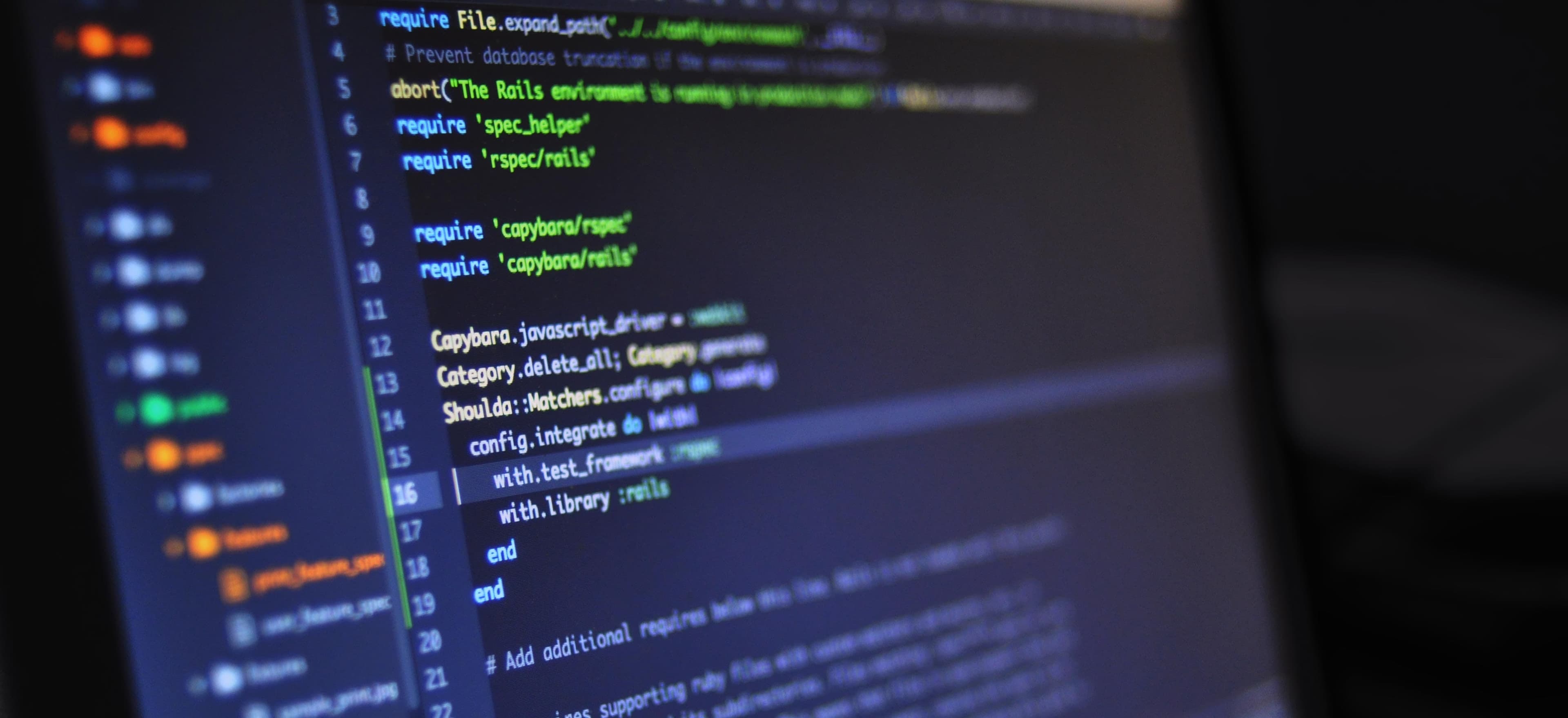
- Published on
Common Pitfalls When Using RESTful API Clients
In the world of software development, RESTful APIs have become the backbone for many applications. They provide a flexible, scalable way to communicate across different services. However, working with RESTful API clients can bring some challenges. In this blog post, we will explore common pitfalls when using RESTful APIs, particularly focusing on Java-based clients.
Understanding RESTful APIs
Before diving into the pitfalls, let's clarify what we mean by RESTful APIs. REST (Representational State Transfer) is an architectural style that defines a set of constraints for building web services. It is built around resources, which can be accessed using standard HTTP methods such as GET, POST, PUT, and DELETE.
Using a RESTful API in Java typically involves an HTTP client to make requests to the server and handle responses. Popular libraries for this purpose include Apache HttpClient, OkHttp, and Spring RestTemplate.
Benefits of RESTful APIs
- Stateless: Each API request contains all the information needed to process it, allowing for scalability.
- Language-agnostic: REST can be implemented by any programming language, making it versatile.
- Standardized: Following HTTP standards leads to predictable behavior.
Despite these benefits, developers can run into various pitfalls. Let’s discuss some of the most common issues and how to avoid them.
1. Poor Error Handling
A common mistake is not adequately handling errors that may arise from API calls. APIs may return different response codes that denote success (2xx) or various levels of failure (4xx, 5xx).
Example:
HttpResponse response = httpClient.execute(httpGet);
if (response.getStatusLine().getStatusCode() != 200) {
throw new RuntimeException("Failed : HTTP error code : " + response.getStatusLine().getStatusCode());
}
Why this matters:
Ignoring harmless responses like 404 Not Found or 403 Forbidden can lead to misleading application behavior. Proper error handling ensures that you account for these discrepancies and respond appropriately in your application.
Solution:
Use a centralized error handling mechanism. This can help categorize errors into client-side, server-side, or network issues.
2. Not Using Timeouts
Network calls can fail for various reasons: the server might go down, or there could be a network interruption. Not setting timeouts can cause your application to hang indefinitely.
Example:
RequestConfig requestConfig = RequestConfig.custom()
.setSocketTimeout(5000) // 5 seconds
.setConnectTimeout(5000)
.build();
CloseableHttpClient client = HttpClients.custom()
.setDefaultRequestConfig(requestConfig)
.build();
Why this matters:
Implementing timeouts can prevent resource exhaustion and maintain responsiveness in your application.
Solution:
Always set connection and socket timeouts, as shown above, to ensure your application can gracefully handle unresponsive services.
3. Not Handling Pagination
APIs that return large datasets will often paginate results. Failing to account for pagination can lead to incomplete data retrieval.
Example:
int currentPage = 1;
while (true) {
HttpResponse response = httpClient.execute(new HttpGet("https://api.example.com/data?page=" + currentPage));
// Process response...
if (noMoreData) break;
currentPage++;
}
Why this matters:
If your application assumes that a single API call will return all relevant data, it may not work as expected. This can lead to inconsistent states or errors during data processing.
Solution:
Check API documentation for pagination details and implement logic to request additional pages of data as necessary.
4. Assuming Responses Are in JSON
RESTful APIs promoted the exclusive use of JSON for data exchange. However, some APIs may return XML or other formats. Assuming a specific format can lead to runtime errors.
Example:
String responseBody = EntityUtils.toString(response.getEntity());
// If the response is XML instead of JSON, this can lead to parsing errors
JSONObject jsonResponse = new JSONObject(responseBody);
Why this matters:
If your code assumes a certain response format, any deviation could crash your application.
Solution:
Always validate the Content-Type of the response header before attempting to parse it, and implement logic to handle different formats as necessary.
5. Lack of Rate Limiting
Many APIs impose limits on how frequently you can make requests. Ignoring these limits can result in your application getting throttled or banned.
Example:
for (int i = 0; i < 100; i++) {
// Make API request
// ...
Thread.sleep(100); // Ensuring requests are spaced out (if applicable)
}
Why this matters:
Hitting the API rate limit can lead to downtime or degraded functionality for your application.
Solution:
Refer to the API documentation for rate limiting guidelines and implement logic to handle retries or back off requests as necessary.
6. Not Caching Responses
Fetching the same data repeatedly from an API can be inefficient. Not implementing caching can lead to increased latency and unnecessary load on the server.
Example:
Map<String, String> cache = new HashMap<>();
String url = "https://api.example.com/data";
String response;
if (cache.containsKey(url)) {
response = cache.get(url);
} else {
HttpResponse httpResponse = httpClient.execute(new HttpGet(url));
response = EntityUtils.toString(httpResponse.getEntity());
cache.put(url, response); // Cache the response
}
Why this matters:
Caching speeds up response times for frequently accessed data, improving the overall user experience.
Solution:
Consider using in-memory databases like Redis or simple in-memory caching to store responses.
Closing the Chapter
Navigating the world of RESTful APIs can come with its challenges. By being aware of common pitfalls such as poor error handling, not using timeouts, failing to manage pagination, and other issues discussed in this blog, developers can create more robust applications.
For further reading, consider checking out these resources:
- REST API Documentation Best Practices
- Java HTTP Client Overview
- Best Practices for HTTP API
By avoiding these pitfalls, you can enhance the reliability and efficiency of your applications. Keep coding smartly, and until next time, happy coding!
Checkout our other articles