Why This Common API Technique Can Derail Your Project
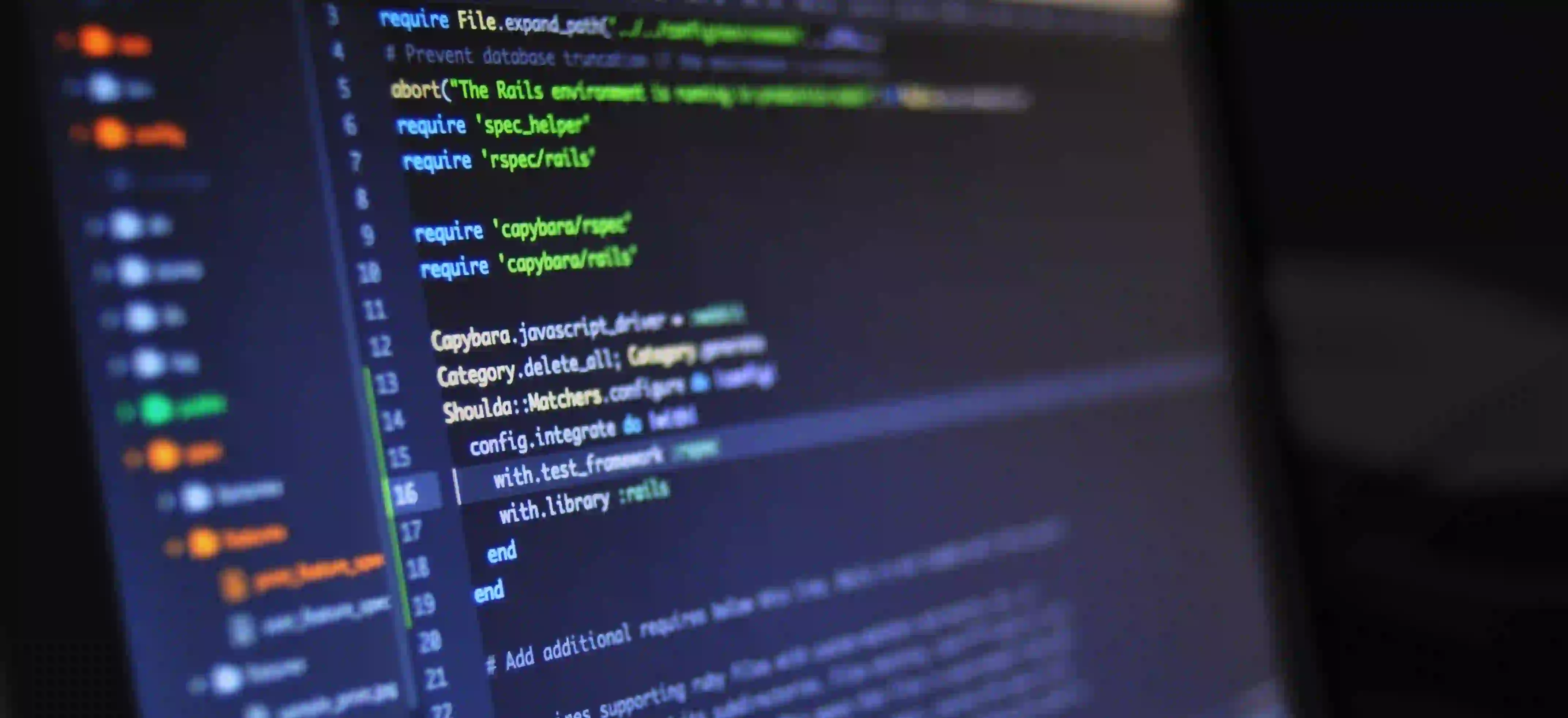
Why This Common API Technique Can Derail Your Project
APIs (Application Programming Interfaces) are the backbone of modern software development. They allow disparate systems to communicate with one another efficiently. However, there are common pitfalls developers encounter when implementing APIs that can derail entire projects. In this blog post, we will explore one of the most prevalent techniques—over-reliance on synchronous API calls—and discuss its implications.
We will delve into the "why" behind the recommendations, demonstrate with code snippets, and provide resourceful links for detailed reading.
Understanding Synchronous API Calls
Synchronous API calls are operations where the client sends a request to a server and waits ("blocks") until the server responds. This is a straightforward approach but often misleadingly convenient.
The Problem with Blocking
Imagine a user trying to log into your application. When they submit their credentials, they expect immediate feedback. If your backend API performs a synchronous call to check the credentials:
- The server takes some time to process the request.
- The client remains idle, waiting for that response.
This blocking mechanism can lead to several issues:
- Poor User Experience: Clients will experience lag and may even perceive the application as unresponsive.
- Increased Server Load: A high volume of synchronous calls can overwhelm server resources, especially if multiple requests from multiple clients are piling up.
- Scaling Concerns: As your user base grows, the limitations of synchronous calls become glaringly evident, often leading to bottlenecks.
Here's a simple Java code snippet demonstrating a synchronous call:
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
public class SynchronousApiCall {
public static void main(String[] args) {
String urlString = "https://api.example.com/login";
try {
URL url = new URL(urlString);
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setRequestMethod("POST");
// Send request
conn.setDoOutput(true);
conn.getOutputStream().write("username=user&password=pass".getBytes());
// Get response
BufferedReader in = new BufferedReader(new InputStreamReader(conn.getInputStream()));
String responseLine;
StringBuilder response = new StringBuilder();
while ((responseLine = in.readLine()) != null) {
response.append(responseLine);
}
in.close();
System.out.println("Response: " + response.toString());
} catch (Exception e) {
e.printStackTrace();
}
}
}
Commentary on the Code
This code sends a POST request to an API to log in a user. While it seems straightforward, the client is blocked until the server responds. The consequences, as we mentioned earlier, are:
- Lag for the User: If the server has any latency, the user will be left waiting.
- Limited Concurrency: Multiple users will cause the server to handle requests serially, potentially leading to longer wait times.
To read more about synchronous vs asynchronous programming concepts, check this comprehensive guide.
The Asynchronous Alternative
Instead of blocking calls, asynchronous API calls allow the application to continue processing while waiting for the server response. This can significantly enhance both speed and user experience.
Benefits of Asynchronous Calls
- Improved Responsiveness: Users can interact with the application even when data is being fetched.
- Better Resource Management: Reduces the number of threads waiting for responses, allowing for more efficient use of server resources.
- Enhanced Scalability: Makes it easier to scale out as operational load changes.
Here’s an example of how to implement an asynchronous API call using Java's CompletableFuture
:
import java.net.HttpURLConnection;
import java.net.URL;
import java.util.concurrent.CompletableFuture;
public class AsynchronousApiCall {
public static void main(String[] args) {
String urlString = "https://api.example.com/login";
CompletableFuture.supplyAsync(() -> {
try {
URL url = new URL(urlString);
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setRequestMethod("POST");
conn.setDoOutput(true);
conn.getOutputStream().write("username=user&password=pass".getBytes());
BufferedReader in = new BufferedReader(new InputStreamReader(conn.getInputStream()));
StringBuilder response = new StringBuilder();
String responseLine;
while ((responseLine = in.readLine()) != null) {
response.append(responseLine);
}
in.close();
return response.toString();
} catch (Exception e) {
throw new RuntimeException(e);
}
}).thenAccept(response -> {
System.out.println("Response: " + response);
});
System.out.println("API call submitted. Continue with other tasks...");
}
}
Why This Works Better
In the above example, the API call is executed in a separate thread using CompletableFuture
. The main thread is free to perform other tasks while waiting for the API response.
- Immediate Feedback: The user receives immediate confirmation that their request was submitted.
- Efficiency: Keeps the application responsive and resource-efficient.
For more about the power of asynchronous programming in Java, explore this in-depth resource.
Pitfalls to Watch Out For
While asynchronous calls can benefit your application profoundly, they are not without challenges. Some common pitfalls include:
- Complexity: Asynchronous programming can lead to complicated code structures, particularly with error handling and state management.
- Debugging Difficulty: Tracing errors through multiple threads can be more challenging than working with synchronous calls.
- Potential for Resource Starvation: Mismanaged asynchronous tasks can lead to excessive resource consumption, particularly when using thread pools.
Best Practices for using Asynchronous Calls
-
Use CompletableFuture Effectively: Taking advantage of Java's
CompletableFuture
can help manage conditional callbacks and exceptions more clearly. -
Implement Timeout and Retry Logic: This ensures that any long-running tasks can signal failures without blocking indefinitely.
-
Monitor and Log: Always log both success and failures in your asynchronous processes for better insight.
The Bottom Line
To wrap up, over-reliance on synchronous API calls can hinder application performance, usability, and scalability. Transitioning to asynchronous API calls can dramatically improve the user experience and optimize resource usage.
It’s crucial to strike a balance when implementing asynchronous flows to avoid complexity and debugging challenges. The transition may seem challenging, but the benefits to your project will undoubtedly outweigh the costs.
As a developer, adopting better strategies around API consumption is vital to ensuring the longevity of your projects. Keep learning, keep coding, and most importantly, keep optimizing.
By focusing on asynchronous programming practices, your application will not only improve in terms of functionality but will also pave the way for a smoother user experience in this fast-paced digital world.
For further reading on API efficiency and best practices, check out REST API Design Rulebook by Mark Masse.
Happy coding! 🎉