Mastering Argument Parsing in Java Command Line Apps
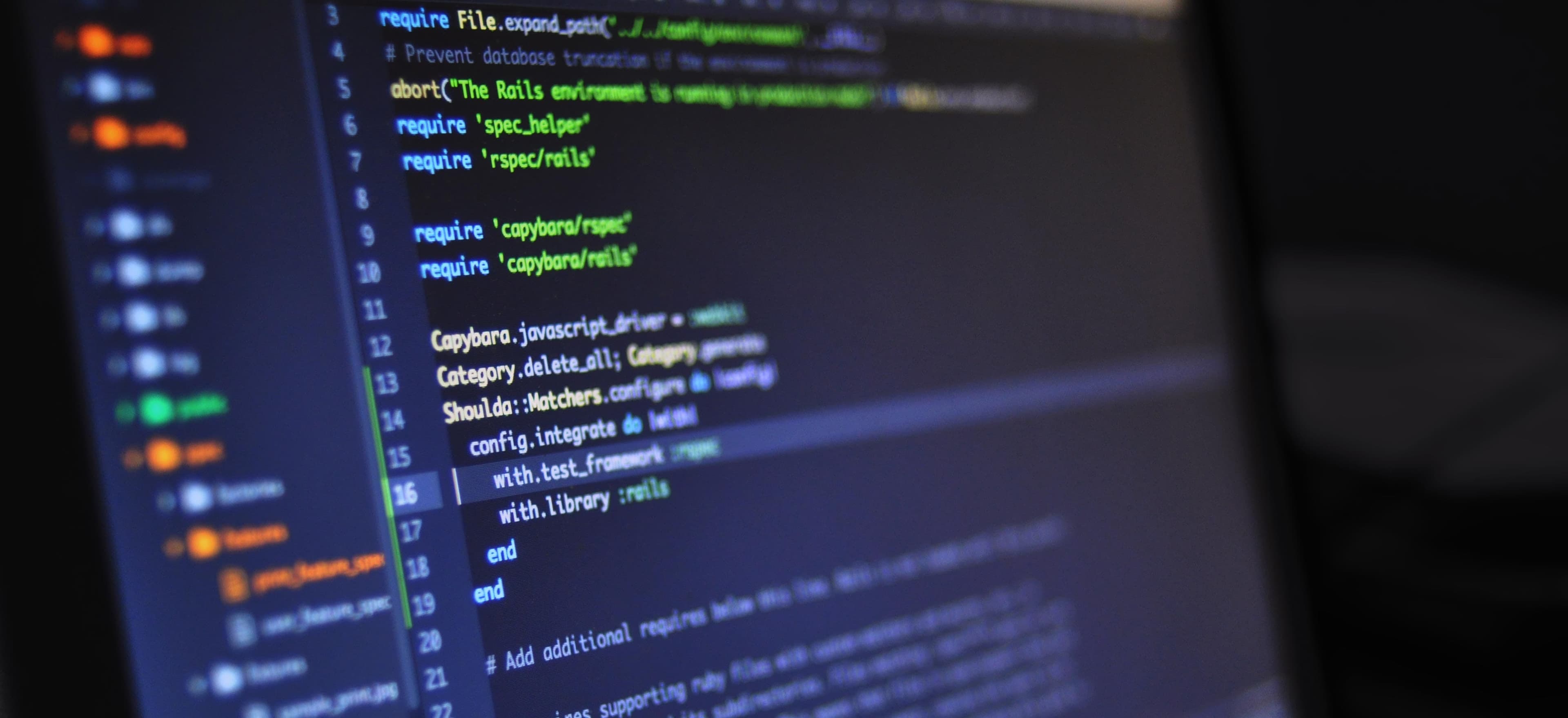
- Published on
Mastering Argument Parsing in Java Command Line Apps
Command line applications are an integral part of software development, offering a simple, straightforward method for users to interact with applications. One of the crucial features of command line applications is argument parsing. Today, we will delve into mastering argument parsing in Java, exploring its importance, various libraries, and offering practical code examples.
Why Argument Parsing Matters
Every command line application requires input, often in the form of command line arguments. Efficient argument parsing is essential for improving the user experience. Properly parsed arguments allow users to:
- Customize how commands operate.
- Input multiple parameters in a limited interface.
- Avoid hardcoding values into applications.
Effective argument parsing also allows applications to handle errors gracefully, which is vital for maintaining user trust.
Java Command Line Basics
Before we dive into argument parsing, let's refresh our understanding of how Java handles command line arguments. The main method in Java is defined as follows:
public static void main(String[] args) {
// Code here...
}
The args
parameter is an array of strings containing the command line arguments passed to the program. For example, executing:
java MyApplication arg1 arg2 arg3
will populate args
with ["arg1", "arg2", "arg3"]
.
Manual Argument Parsing
Although you can manually parse arguments using a simple for
loop, this method can quickly become cumbersome, especially as you add more options and complexity to your application.
Example of Manual Parsing
Here’s a basic example of how you might manually parse arguments in Java:
public class ManualArgumentParsing {
public static void main(String[] args) {
String name = null;
int age = 0;
for (int i = 0; i < args.length; i++) {
switch (args[i]) {
case "--name":
if (i + 1 < args.length) {
name = args[++i]; // Get the next value in the array
}
break;
case "--age":
if (i + 1 < args.length) {
age = Integer.parseInt(args[++i]); // Convert to int
}
break;
default:
System.out.println("Unknown argument: " + args[i]);
break;
}
}
System.out.println("Name: " + name);
System.out.println("Age: " + age);
}
}
Commentary
args[i]
: We iterate over each argument and check for specific flags (e.g.,--name
).- Data validation: This approach checks if a subsequent value exists before accessing it, minimizing exceptions.
- Error handling: If the developer inputs an unknown argument, a message is printed.
While manual parsing suffices for basic applications, it doesn't scale well as complexity grows.
Using Apache Commons CLI
To streamline argument parsing and improve maintainability, using libraries like Apache Commons CLI is advisable. This library allows more robust features such as automatic usage help documentation, parsing validation, and customizable parameter definitions.
Getting Started with Apache Commons CLI
First, add the Maven dependency to your pom.xml
:
<dependency>
<groupId>commons-cli</groupId>
<artifactId>commons-cli</artifactId>
<version>1.4</version>
</dependency>
Example with Apache Commons CLI
Here’s a refined example using Apache Commons CLI to manage command line arguments:
import org.apache.commons.cli.*;
public class ApacheCliExample {
public static void main(String[] args) {
Options options = new Options();
options.addOption("n", "name", true, "User's name");
options.addOption("a", "age", true, "User's age");
CommandLineParser parser = new DefaultParser();
try {
CommandLine cmd = parser.parse(options, args);
String name = cmd.getOptionValue("n");
String ageStr = cmd.getOptionValue("a");
int age = ageStr != null ? Integer.parseInt(ageStr) : 0;
System.out.println("Name: " + name);
System.out.println("Age: " + age);
} catch (ParseException e) {
System.out.println("Error parsing command line: " + e.getMessage());
HelpFormatter formatter = new HelpFormatter();
formatter.printHelp("Command Line Arguments", options);
}
}
}
Commentary
- Options Definition: Options are defined using the
Options
class, making it easy to specify required flags, their data types, and descriptions. - Parsing: The
DefaultParser
interprets the command line arguments, and any parsing errors are handled cleanly. - Help Generation: If parsing fails, you use
HelpFormatter
to display available options, creating a user-friendly command line experience.
Using JCommander
Another powerful library for parsing command line arguments is JCommander. This library uses annotations to simplify argument declarations, reducing boilerplate code significantly.
Getting Started with JCommander
First, add the Maven dependency:
<dependency>
<groupId>com.beust</groupId>
<artifactId>jcommander</artifactId>
<version>1.81</version>
</dependency>
Example with JCommander
Now let's see JCommander in action:
import com.beust.jcommander.JCommander;
import com.beust.jcommander.Parameter;
public class JCommanderExample {
static class CommandLineArgs {
@Parameter(names = "--name", description = "User's name")
private String name;
@Parameter(names = "--age", description = "User's age")
private int age = 0; // Default value
@Parameter(names = "--help", help = true)
private boolean help;
}
public static void main(String[] args) {
CommandLineArgs cliArgs = new CommandLineArgs();
JCommander jCommander = JCommander.newBuilder()
.addObject(cliArgs)
.build();
try {
jCommander.parse(args);
if (cliArgs.help) {
jCommander.usage();
return;
}
System.out.println("Name: " + cliArgs.name);
System.out.println("Age: " + cliArgs.age);
} catch (Exception e) {
System.out.println("Error parsing command line: " + e.getMessage());
jCommander.usage();
}
}
}
Commentary
- Annotations: JCommander uses annotations to define expected parameters, streamlining the process of argument specification.
- Help Option: Adding a help flag is straightforward, and using
usage()
automatically generates a help message based on defined parameters. - Default Values: You can set default values for parameters directly, simplifying your error handling.
My Closing Thoughts on the Matter
Command line argument parsing is critical in Java command line applications. While manual parsing works for basic functionality, leveraging libraries like Apache Commons CLI and JCommander enhances usability, maintainability, and flexibility.
As your command line application grows, consider options that can handle complexity gracefully. The ability to parse arguments effectively allows users to customize their experience, improving both satisfaction and functionality.
For further reading:
By mastering argument parsing in Java, you can enhance your command line applications, ensuring they meet users' needs efficiently while maintaining clean and manageable code. Happy coding!
Checkout our other articles