Integrating Microprofile 2.2 BOM: Common Pitfalls to Avoid
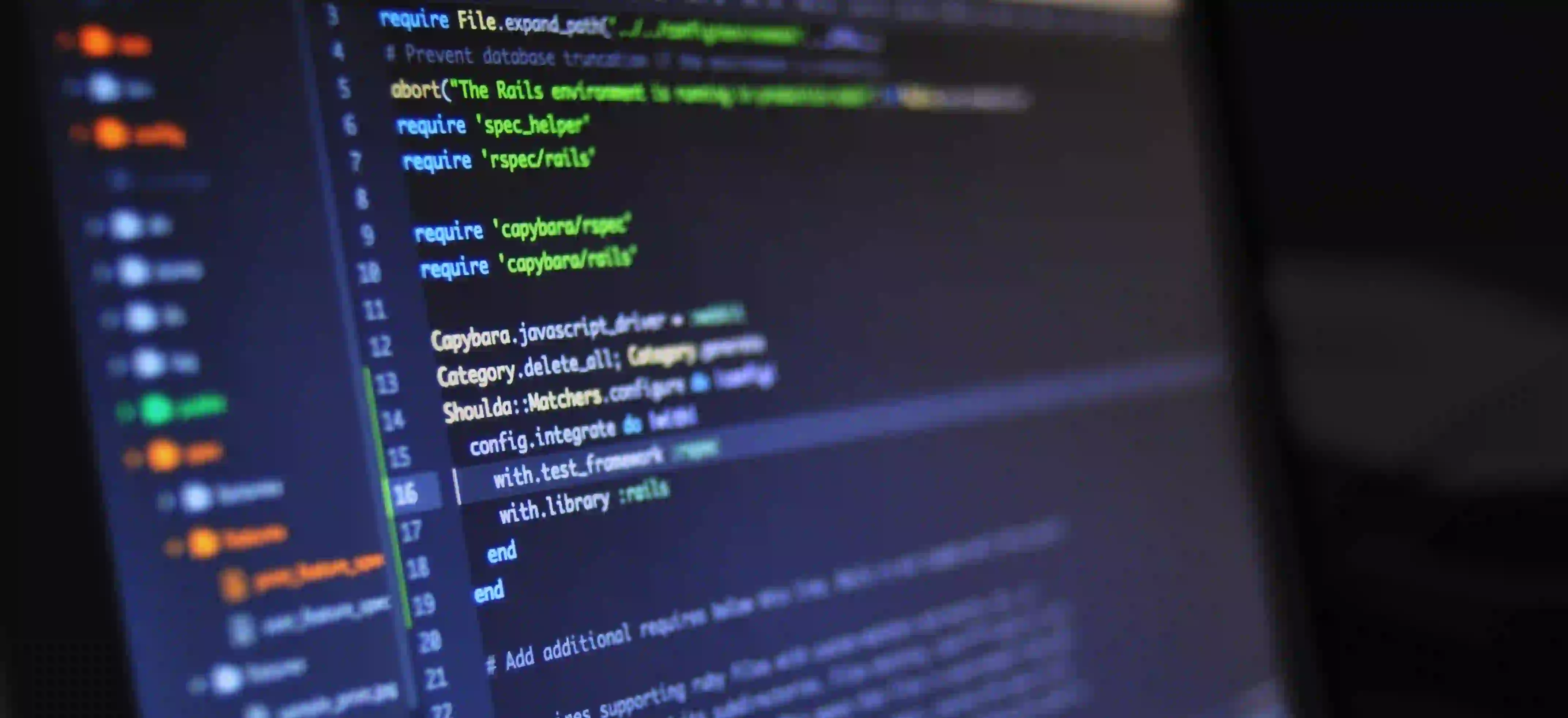
Integrating MicroProfile 2.2 BOM: Common Pitfalls to Avoid
MicroProfile provides a set of specifications that optimize Enterprise Java for microservices architecture. When working with MicroProfile, version management can become a tricky affair, especially when using Bill of Materials (BOM) to ensure compatibility among various dependencies. This blog post will explore the integration of MicroProfile 2.2 BOM into your project, highlighting common pitfalls and best practices to avoid them.
What is MicroProfile BOM?
A Bill of Materials (BOM) is a special POM file in Maven that contains a list of dependencies and versions. By using a BOM, developers can ensure that their project uses compatible versions of various MicroProfile specifications. For example, MicroProfile 2.2 introduces several features such as Fault Tolerance, JWT Propagation, and OpenAPI enhancements, and aligning your project with these specifications can enable better resilience and adaptability.
Why MicroProfile 2.2?
MicroProfile 2.2 brings numerous enhancements, including improved performance and streamlined features. Utilizing the BOM for this version can simplify dependency management. However, the integration process can lead to several pitfalls. Here’s how to seamlessly integrate MicroProfile 2.2 BOM while avoiding common mistakes.
Getting Started with MicroProfile 2.2 BOM
Before diving into code examples, let’s first set up a minimal Maven project that uses MicroProfile 2.2 BOM.
Here is a basic pom.xml configuration:
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.example</groupId>
<artifactId>microprofile-example</artifactId>
<version>1.0-SNAPSHOT</version>
<packaging>jar</packaging>
<dependencyManagement>
<dependencies>
<dependency>
<groupId>org.eclipse.microprofile</groupId>
<artifactId>microprofile-bom</artifactId>
<version>2.2</version>
<type>pom</type>
<scope>import</scope>
</dependency>
</dependencies>
</dependencyManagement>
<dependencies>
<dependency>
<groupId>org.eclipse.microprofile</groupId>
<artifactId>microprofile-fault-tolerance-api</artifactId>
</dependency>
<dependency>
<groupId>org.eclipse.microprofile</groupId>
<artifactId>microprofile-openapi-api</artifactId>
</dependency>
<dependency>
<groupId>org.eclipse.microprofile</groupId>
<artifactId>microprofile-jwt-auth-api</artifactId>
</dependency>
</dependencies>
</project>
Common Pitfalls When Integrating MicroProfile 2.2 BOM
Now, let’s discuss some of the common pitfalls you might encounter and how to address them.
1. Ignoring the Dependency Management Feature
One of the key advantages of using BOM is central management of dependency versions. Failing to leverage this feature can lead to unexpected behavior and conflicts due to mismatched versions.
Solution
Make sure to define your dependencies under the dependencyManagement
section. This avoids specifying versions repeatedly in your dependencies
block.
2. Missing Key Dependencies
When integrating MicroProfile, developers sometimes overlook specific APIs needed for their use cases. For instance, while you might be focused on Fault Tolerance, you should also not neglect APIs like Configuration and Metrics.
Solution
Regularly check the MicroProfile Specification for key APIs relevant to your project needs. For example, if you are building microservices for cloud-native applications, consider including:
<dependency>
<groupId>org.eclipse.microprofile</groupId>
<artifactId>microprofile-metrics-api</artifactId>
</dependency>
3. Incompatible Server Version
Sometimes the issue arises from using a MicroProfile version that is not supported by your runtime server. For instance, if you're using an older server version, it may not support all MicroProfile 2.2 features.
Solution
Consult your runtime documentation to ensure compatibility. If using Payara or Open Liberty, for example, confirm the versions and make sure they've adopted the necessary MicroProfile features.
4. Not Testing in Isolation
When working on larger applications, it's easy to forget to isolate unit tests for MicroProfile features. Failing to do so can lead to cascading failures that are tough to debug.
Solution
Utilize tools like Arquillian to create isolated tests for the MicroProfile aspects of your application, ensuring that changes to one part of the application do not inadvertently break others.
@MicroProfileTest
public class MyServiceTest {
@Inject
MyService myService;
@Test
public void testServiceMethod() {
String response = myService.callExternalService();
assertEquals("Expected Response", response);
}
}
5. Dependency Conflicts Due to Transitive Dependencies
Another common issue arises from the transitive dependencies pulled in with certain MicroProfile APIs. They may introduce versions of libraries that lead to conflicts, potentially breaking your application.
Solution
When using Maven, consult the effective POM using the command:
mvn help:effective-pom
Check for conflicting versions and override them in your POM as necessary.
<dependency>
<groupId>org.eclipse.microprofile</groupId>
<artifactId>microprofile-config-api</artifactId>
<exclusions>
<exclusion>
<groupId>some.group</groupId>
<artifactId>conflicting-artifact</artifactId>
</exclusion>
</exclusions>
</dependency>
6. Lack of Proper Configuration
The configuration capabilities of MicroProfile can easily be underutilized. If you hardcode configurations, you miss out on the flexibility that configuration features offer.
Solution
Utilize the MicroProfile Config API to manage application settings through configuration files, environment variables, or system properties.
Here’s an example that loads a configuration property:
import org.eclipse.microprofile.config.inject.ConfigProperty;
public class GreetingService {
@Inject
@ConfigProperty(name = "greeting.message", defaultValue = "Hello")
String greetingMessage;
public String greet() {
return greetingMessage;
}
}
Ensure the configuration property is set in your appropriate configuration source, such as an application.properties
file.
My Closing Thoughts on the Matter
Integrating MicroProfile 2.2 BOM into your project provides a robust framework for building modern microservices. By recognizing common pitfalls such as ignoring dependency management, missing key dependencies, and encountering version conflicts, you can successfully navigate the integration process.
Make sure to keep up with the MicroProfile specifications for the latest features and best practices. Armed with this knowledge, you'll enhance the resilience and performance of your Java microservices while avoiding common integration issues.
Happy coding!