Simplifying Amazon S3 Mock Testing for Java Developers
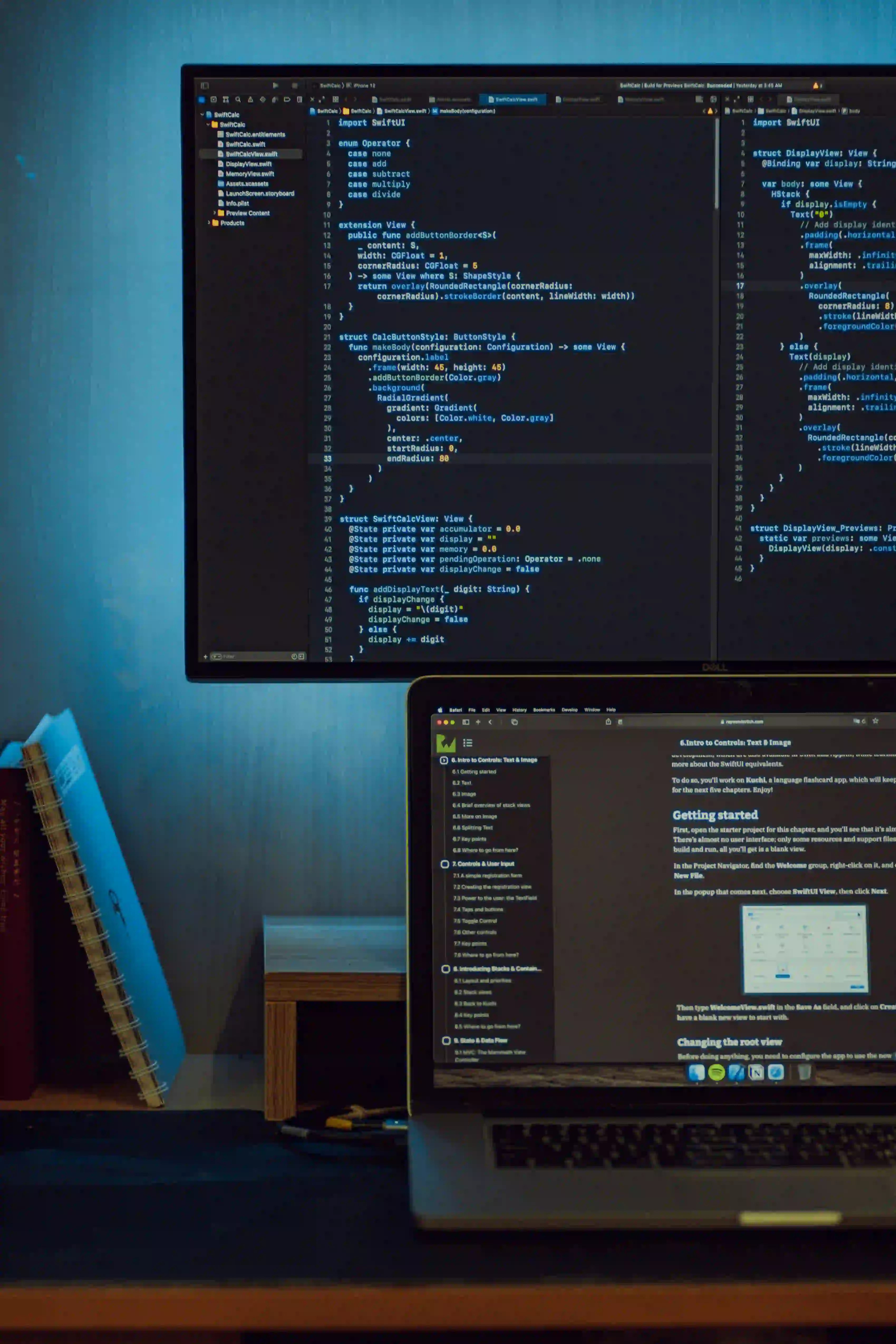
Simplifying Amazon S3 Mock Testing for Java Developers
Testing is an integral part of software development, ensuring that our applications run smoothly and efficiently. When it comes to applications that interact with external services like Amazon S3 (Simple Storage Service), testing can be challenging. However, leveraging mock services makes it easier. This blog post delves into simplifying Amazon S3 mock testing for Java developers, providing you with valuable code snippets and insights along the way.
Understanding Amazon S3
Amazon S3 is a cloud-based object storage service used for storing and retrieving data. It's widely used due to its scalability, durability, and security. When you're developing applications that need to upload, download, or manage files, S3 often becomes a go-to solution.
However, integrating S3 in your application poses challenges during testing, particularly if you want to avoid unnecessary costs and dependencies on internet connectivity. This is where mock testing comes into play.
Why Mock Testing?
Mock testing allows you to simulate the behavior of an external system. Here are some key reasons why mock testing is essential:
- Cost-Effective: Avoid unnecessary expenses when performing tests that involve external services.
- Reliable: Simulate various scenarios, including error conditions, without relying on the actual external system.
- Fast Feedback: Because no network calls are made, mock tests run much faster.
Setting Up Mock Testing for Amazon S3
One popular library for mocking AWS services in Java is LocalStack. LocalStack is a fully functional local AWS cloud stack, which supports various AWS services including S3. You can also use the AWS SDK for Java to interact with S3 programmatically.
Step 1: Add Dependencies
To mock S3 in Java, you'll need to add dependencies for LocalStack and the AWS SDK in your project. If you're using Maven, add the following to your pom.xml
.
<dependencies>
<dependency>
<groupId>cloud.localstack</groupId>
<artifactId>localstack-utils</artifactId>
<version>0.2.11</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>com.amazonaws</groupId>
<artifactId>aws-java-sdk-s3</artifactId>
<version>1.12.300</version>
</dependency>
</dependencies>
Step 2: Start LocalStack
Before running your tests, you need LocalStack to simulate S3. You can do this easily with Docker:
docker run --rm -d -p 4566:4566 -p 4510-4559:4510-4559 localstack/localstack
Step 3: Writing Mock Tests
Now, let’s write a simple unit test using JUnit. We'll create a mock service to upload and retrieve objects from S3.
Code Snippet: Uploading an Object
@Test
public void testUploadObject() {
// Create an S3 client for LocalStack
AmazonS3 s3Client = AmazonS3ClientBuilder.standard()
.withEndpointConfiguration(new AwsClientBuilder.EndpointConfiguration("http://localhost:4566", "us-east-1"))
.withCredentials(new AWSStaticCredentialsProvider(new BasicAWSCredentials("access", "secret")))
.withPathStyleAccessEnabled(true) // Required for LocalStack
.build();
String bucketName = "test-bucket";
String objectKey = "test-file.txt";
String content = "Hello, S3!";
// Create bucket
s3Client.createBucket(bucketName);
// Upload object
s3Client.putObject(new PutObjectRequest(bucketName, objectKey, content));
// Assert that the object exists
Assert.assertTrue(s3Client.doesObjectExist(bucketName, objectKey));
}
Commentary: Why This Works
-
AmazonS3 Client: We create the S3 client pointing to the LocalStack endpoint. The configuration includes credentials and enables path-style access, which LocalStack requires.
-
Bucket Creation: The test first creates a bucket to hold the files. This is a necessary step because you cannot upload files to a non-existent bucket.
-
Object Upload: The
putObject
method is used to upload a string as a file. Here, it’s simplified to just a string, but it could easily be a file or a stream. -
Assertions: Finally, we check if the object exists, which assures us that the upload worked.
Step 4: Cleaning Up After Tests
It's best practice to clean up resources after your tests run. You can add a teardown method to remove test buckets in your test class.
@After
public void tearDown() {
String bucketName = "test-bucket";
s3Client.deleteBucket(bucketName);
}
Handling More Complex Scenarios
Dealing with Exceptions
One significant aspect of writing tests is handling various exceptions that might be thrown by S3 operations. Here’s how to test S3 exception scenarios.
Code Snippet: Testing Exception Handling
@Test(expected = AmazonS3Exception.class)
public void testUploadToNonExistentBucket() {
AmazonS3 s3Client = AmazonS3ClientBuilder.standard()
.withEndpointConfiguration(new AwsClientBuilder.EndpointConfiguration("http://localhost:4566", "us-east-1"))
.withCredentials(new AWSStaticCredentialsProvider(new BasicAWSCredentials("access", "secret")))
.withPathStyleAccessEnabled(true)
.build();
String nonExistentBucket = "non-existent-bucket";
String objectKey = "test-file.txt";
String content = "Hello, S3!";
// Attempt to upload to a non-existent bucket
s3Client.putObject(new PutObjectRequest(nonExistentBucket, objectKey, content));
}
Commentary: Why This Works
-
Exception Testing: The test method is annotated with
@Test(expected = AmazonS3Exception.class)
to expect an exception when trying to upload an object to a non-existent bucket. -
Failing Gracefully: This way, you can ensure that your application handles errors gracefully and that the logic in your application is robust against unexpected S3 states.
The Last Word
Mocking Amazon S3 during unit tests can drastically enhance your development speed and reliability. By utilizing LocalStack and the AWS SDK for Java, you can create effective test suites while keeping your costs low and your tests fast and isolated.
For more detailed information on LocalStack, please visit LocalStack Documentation. For more on the AWS SDK for Java, check the AWS SDK for Java.
As we continue developing more complex applications, embracing mock testing for external services like Amazon S3 will become increasingly important. Start integrating these practices into your workflow and witness significant improvements in your testing processes. Happy coding!