Unlocking Quarkus Dev Mode for Non-Quarkus Projects
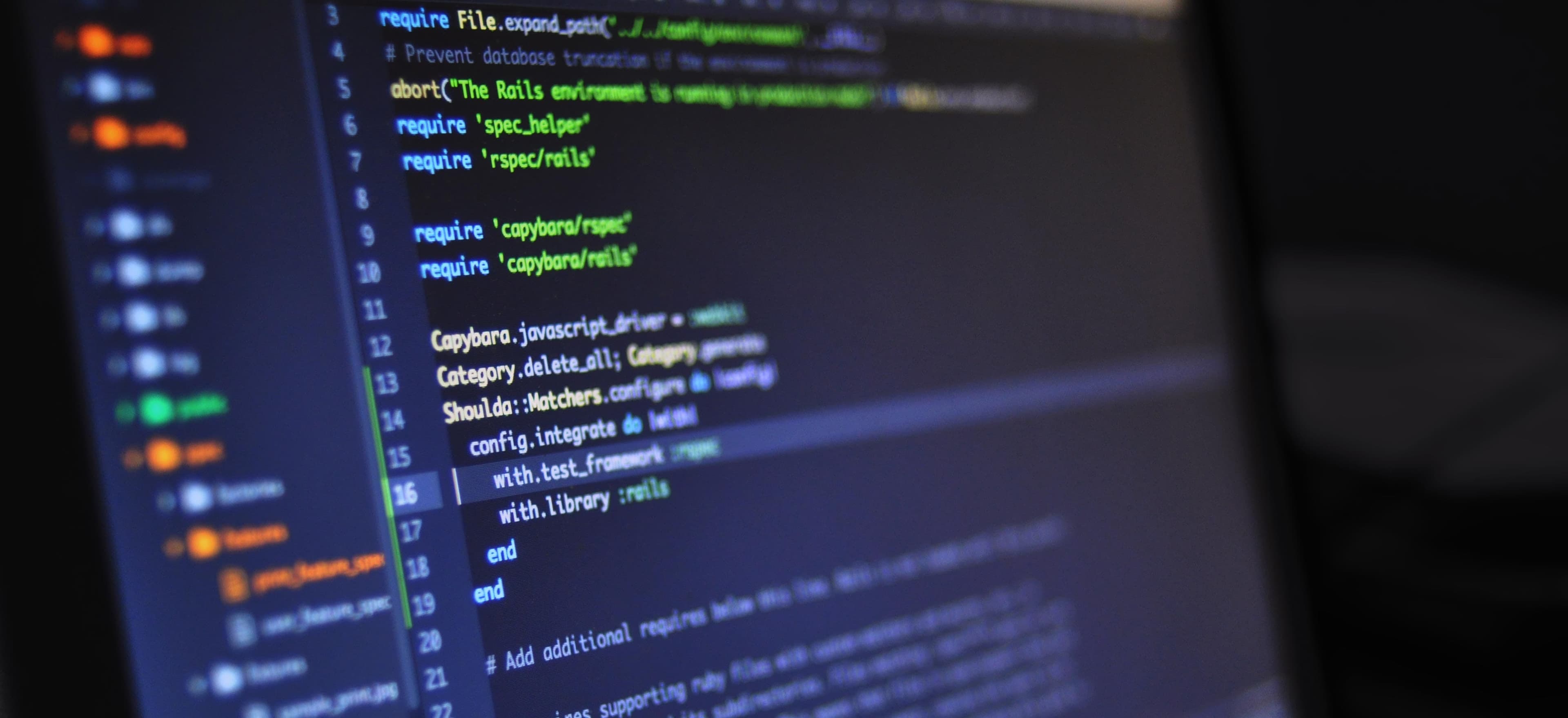
- Published on
Unlocking Quarkus Dev Mode for Non-Quarkus Projects
Quarkus is often celebrated as the "Supersonic Subatomic Java" framework designed specifically for Kubernetes and cloud-native environments. One of its most compelling features is its Dev Mode, which enhances developer productivity by providing crucial hot-reload capabilities and in-the-flow debugging. However, what if you want to leverage Quarkus Dev Mode outside of a typical Quarkus project? This blog post will walk you through integrating Quarkus Dev Mode with non-Quarkus projects, enabling you to maximize your productivity while adhering to Quarkus principles.
What is Quarkus Dev Mode?
Before delving into the integration process, let's establish what Quarkus Dev Mode is. In a nutshell, Quarkus Dev Mode allows developers to write code and see immediate effects without restart delays.
Here’s why you should consider using Dev Mode:
- Hot Reloading: Code changes can be reflected instantly without restarting the application.
- Simplified Testing: With the ability to debug instantaneously, testing becomes not only quicker but also more effective.
- Enhanced Developer Experience: It’s built for productivity—just what you need to keep up with the fast-paced world of software development.
For more information on Quarkus features, you can check out the official Quarkus documentation.
The Prerequisites
Before you can use Quarkus Dev Mode in a non-Quarkus environment, you’ll need to meet several prerequisites:
- Java JDK 11 or newer: Quarkus requires modern Java versions to take full advantage of its capabilities.
- Maven or Gradle: You need a build tool that integrates with the Quarkus ecosystem.
- Familiarity with Quarkus concepts: Having a basic understanding of Quarkus Hibernates, REST services, and dependency injection will speed up the process.
- Existing Java project: Ensure you have a Java project to integrate with, regardless of its framework (Spring, Micronaut, etc.).
Setting up a Non-Quarkus Project with Quarkus Dev Mode
Let’s assume you have a standard Maven-based Java project. Below are the steps to set up Quarkus Dev Mode.
Step 1: Add Quarkus Dependencies
To start, you need to integrate Quarkus into your existing Maven project. Open your pom.xml
file and add the following dependency inside the <dependencies>
tag:
<dependency>
<groupId>io.quarkus</groupId>
<artifactId>quarkus-resteasy</artifactId>
</dependency>
Why? The quarkus-resteasy
dependency will allow you to build RESTful services, a common requirement in modern applications.
Then, include the Quarkus plugin in your pom.xml
:
<build>
<plugins>
<plugin>
<groupId>io.quarkus</groupId>
<artifactId>quarkus-maven-plugin</artifactId>
<version>${quarkus.version}</version>
<executions>
<execution>
<goals>
<goal>build</goal>
<goal>dev</goal>
</goals>
</execution>
</executions>
</plugin>
</plugins>
</build>
Why? This plugin is responsible for managing your Quarkus application lifecycle, with built-in capabilities for development mode.
Step 2: Initializing Quarkus in your Project
Once your dependencies are in place, you need to set up Quarkus configurations. Create a new configuration file named application.properties
in your src/main/resources
directory.
quarkus.http.port=8080
Why? This sets the HTTP server port for your application, which can be customized as per your preferences.
Step 3: Creating a REST Endpoint
Next, let’s create a simple REST resource. Create a new Java class named HelloWorldResource
in your src/main/java
directory:
import javax.ws.rs.GET;
import javax.ws.rs.Path;
@Path("/hello")
public class HelloWorldResource {
@GET
public String hello() {
return "Hello, World!";
}
}
Why? This REST endpoint serves to demonstrate the hot-reload capability of Quarkus Dev Mode.
Step 4: Running Quarkus Dev Mode
Open your terminal and navigate to the root directory of your project. Run the following command to start Quarkus in Dev Mode:
./mvnw quarkus:dev
Why? This command initiates the Quarkus Dev Mode, watching for any changes you make to the source files and reloading the application as needed.
Step 5: Testing your Endpoint
Once Quarkus Dev Mode is running, navigate to http://localhost:8080/hello
in your web browser. You should see:
Hello, World!
Now, modify the hello
method:
public String hello() {
return "Hello, Dev Mode!";
}
Save the file, and without restarting, revisit your endpoint — you should see the updated message!
Advanced Features of Quarkus Dev Mode
Beyond simple REST services, Quarkus offers a suite of advanced features that enhance developer productivity:
Profile-based Configuration
Quarkus supports profile-based configurations that allow you to customize the application for different environments (development, production, etc.). Modify your application.properties
to enable this:
%dev.quarkus.http.port=8081
Why? This allows your application to run on a different port in development mode, preventing conflicts with other applications.
Integration with Other Frameworks
Quarkus can also integrate with other Java frameworks seamlessly. If your non-Quarkus project uses Hibernate, you can add compatibility to load your entities and repositories alongside Quarkus functionality.
Add Hibernate dependencies to your pom.xml
:
<dependency>
<groupId>io.quarkus</groupId>
<artifactId>quarkus-hibernate-orm</artifactId>
</dependency>
Integrating Quarkus with Hibernate allows you to benefit from Native Queries and ORM capabilities while using Dev Mode, simplifying your development cycle.
Using Quarkus Extensions
Extensions extend Quarkus’ capabilities. You can add a variety of extensions ranging from database clients to messaging systems to enhance your project's functionality.
To add an extension, use the following command in your terminal:
./mvnw quarkus:add-extension -Dextensions="quarkus-jdbc-postgresql"
Why? This allows you to connect your application to a PostgreSQL database, demonstrating how easily you can integrate external services into your development cycle.
The Last Word
Integrating Quarkus Dev Mode into your non-Quarkus Java project is not just feasible but also straightforward. By following the steps outlined in this blog post, you can improve your development experience significantly. With features like hot reloading, profile-based configurations, and rich ecosystem extensions, Quarkus presents compelling utilities for any Java developer.
You can explore more about Quarkus features and its entire ecosystem here.
Feel free to share your experiences with Quarkus Dev Mode in the comments below, and happy coding!