Are You Ready for Java 9? Avoid These Jigsaw Pitfalls!
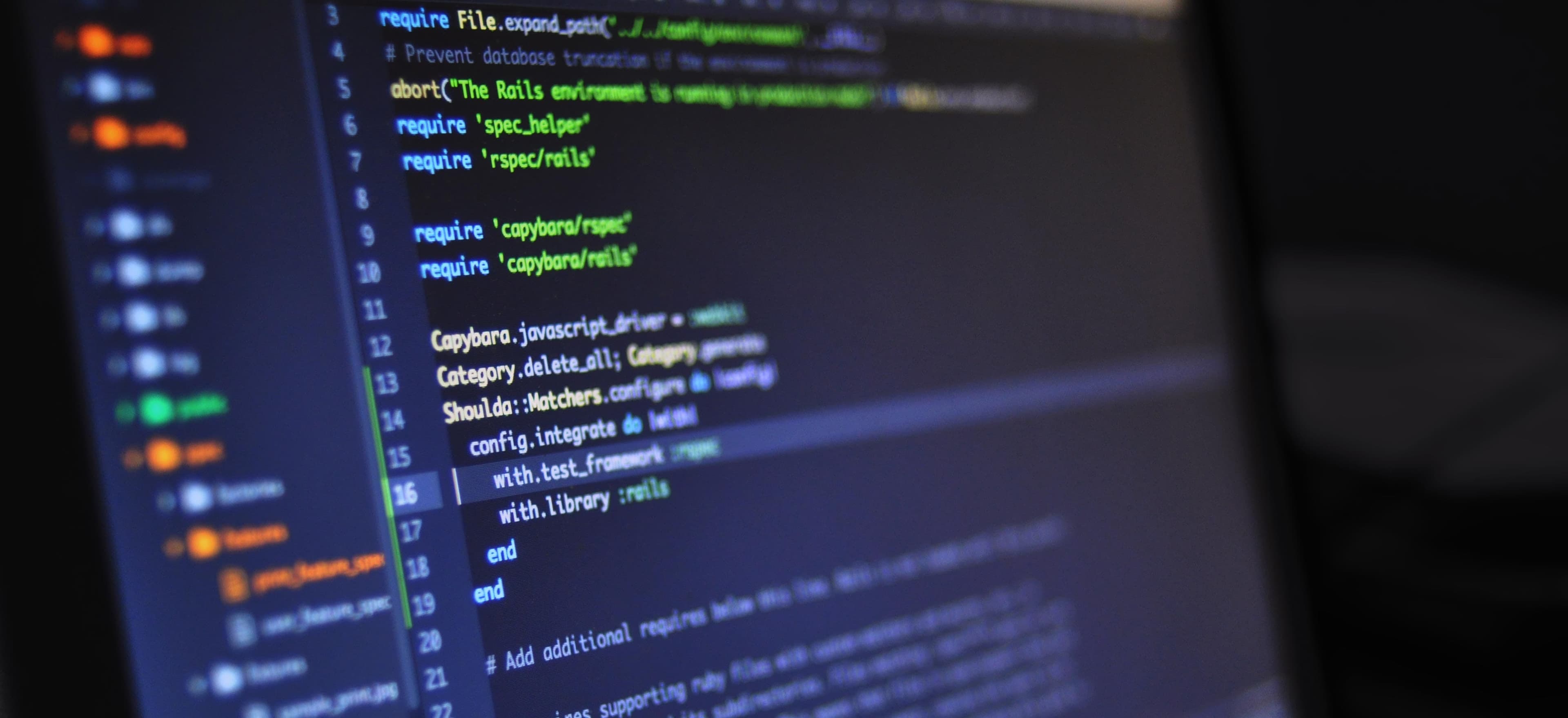
- Published on
Are You Ready for Java 9? Avoid These Jigsaw Pitfalls!
Java 9 brought with it several exciting features and enhancements, chief among them being the Java Platform Module System, commonly referred to as Jigsaw. Jigsaw aims to improve the modularization of Java applications, making them more maintainable, scalable, and understandable. However, transitioning to this new module system can pose challenges. In this post, we'll delve into some common pitfalls developers might encounter while adapting to Java 9's Jigsaw and how to sidestep them.
Why Jigsaw?
Java has long been criticized for its monolithic architecture. With Jigsaw, the goal is to break the Java ecosystem into more manageable modules. This has several advantages:
- Improved Performance: By allowing developers to load only the required modules, applications can start faster and consume less memory.
- Better Encapsulation: Jigsaw promotes the notion of hiding implementation details, leading to cleaner APIs.
- Easier Maintenance: With modularity, codebases become more organized and manageable.
For those looking to delve deeper into the specifications of Java 9 and modularity, you can check the official documentation here.
Common Pitfalls When Migrating to Java 9
1. Inadequate Understanding of Modules
One of the biggest hurdles developers face is the concept of modules themselves. A module is a set of packages designed for reuse. Every module has a module-info.java
file that declares its dependencies and the packages it exports.
Example of a module-info.java:
module com.example.myapp {
exports com.example.myapp.api;
requires com.example.dependency;
}
Why This Matters:
- The
exports
clause allows other modules to use the specified packages. - The
requires
clause specifies dependencies on other modules.
Failing to correctly define your modules could lead to class not found exceptions or unexpected behavior.
2. Mismanagement of Dependencies
Under the new system, dependencies must be clearly defined and managed. Traditional JAR files are not enough. You must ensure that all modules are compatible and without cyclic dependencies.
Tip: Utilize tools like Maven or Gradle, which now support module metadata, to track and manage dependencies. This can save you a great deal of guesswork and overhead.
3. Ignoring Readability and Maintenance
With modularization comes the promise of better readability. However, lean heavily into discipline. Make sure every module serves a single purpose and that code is well-documented.
Here's a simple example of a modularized codebase structure:
MyApp/
│
├── module1/
│ ├── src/
│ │ └── com/example/module1/
│ │ ├── Module1Class.java
│ │ └── module-info.java
│ └── build.gradle
│
└── module2/
├── src/
│ └── com/example/module2/
│ ├── Module2Class.java
│ └── module-info.java
└── build.gradle
Why Structure Matters: A well-structured project can simplify collaboration among teams, streamline onboarding, and enhance maintainability.
4. Avoiding the --add-modules
Flag
When running modularized Java applications, you might encounter issues with modules not found during execution. A common method to bypass this is by using the --add-modules
flag.
Example:
java --module-path mods -m com.example.myapp/com.example.myapp.Main
Using the --add-modules
flag ensures that required modules are included in the runtime.
Why This is Important: Not using this flag can lead to runtime exceptions, which can derail your application's launch.
5. Trying to Force Compatibility Mode
Many developers mistakenly try to run legacy code in a modularized environment without adjusting it. However, most JDK libraries are unsealed and can make the transition hiccup-prone. Tools like jlink
allow you to create a custom runtime with only the necessary modules.
JLink Example:
jlink --module-path $JAVA_HOME/jmods:myapp.jar --add-modules com.example.myapp \
--output custom-runtime
Why Customize Your Runtime? This reduces your application's size and enhances performance since only essential modules are included.
6. Not Testing Thoroughly
With modularization comes an increased complexity. It is paramount to implement a robust testing strategy. Unit tests, integration tests, and end-to-end tests should all be revisited.
Why Testing Matters:
- Unit tests can identify problems early in development.
- Integration tests ensure that modules interact as expected.
- End-to-end tests validate the application’s behavior in a production-like setting.
To learn more about testing in Java 9, consider checking out JUnit 5 documentation.
7. Underestimating the Migration Cost
Finally, transitioning a large codebase into modules isn't a trivial task. Factor in time for refactoring, rewriting documentation, and training your team.
Tip: Start by breaking down the transition into manageable chunks. Prioritize critical modules first, and gradually refactor the rest.
Key Takeaways
Java 9's Jigsaw module system presents an incredible opportunity for developers to modernize their applications. By understanding these pitfalls and adopting a strategic approach to migration, you can leverage the power of modules effectively.
Embrace modularization by correctly defining your modules, managing dependencies, ensuring a comprehensive testing strategy, and structuring your projects well. Your future self (and your fellow developers) will thank you.
For those eager to dive deeper into Java's rich feature set, consider exploring Oracle's comprehensive tutorials on Java Platform Module System.
Happy coding!
Checkout our other articles