Overcoming Common Challenges in Automated Agile Testing
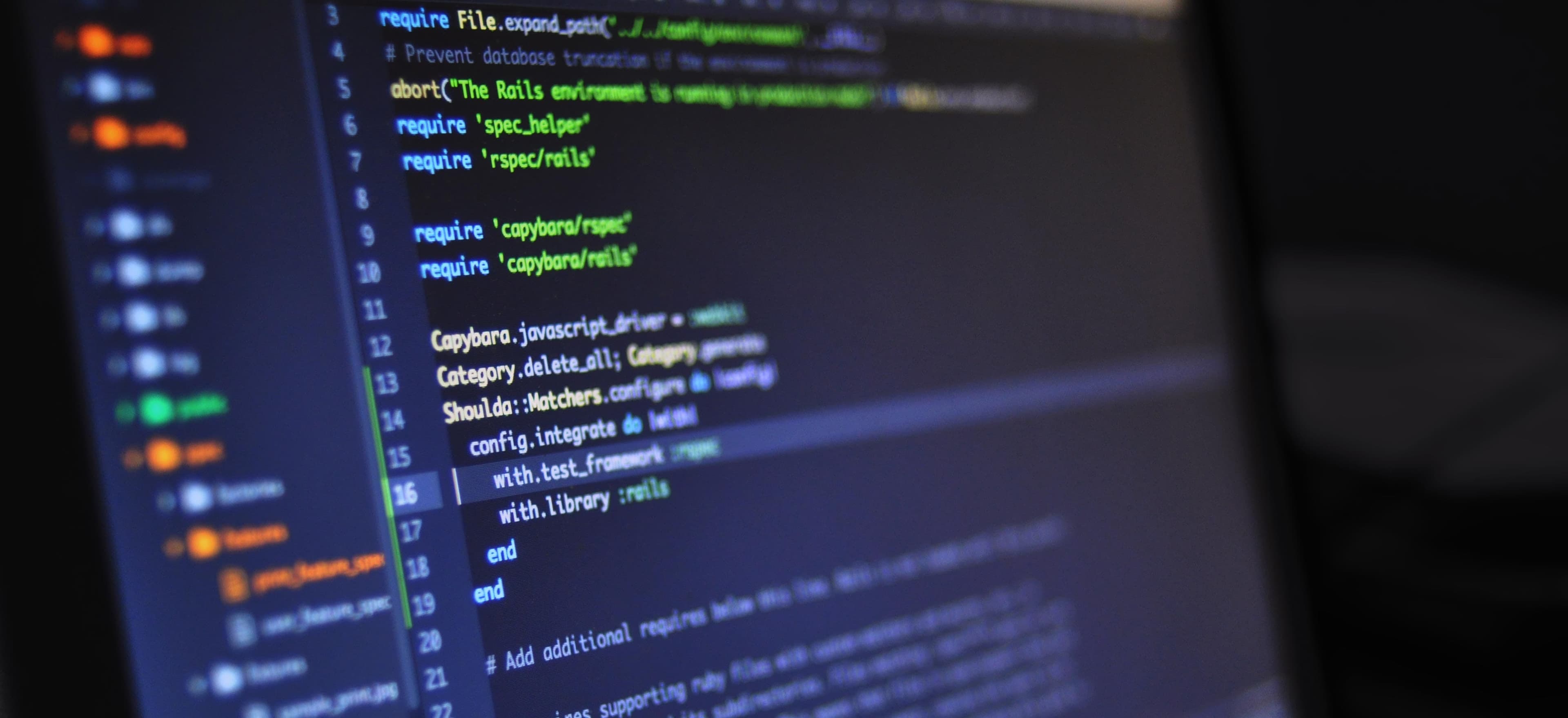
- Published on
Overcoming Common Challenges in Automated Agile Testing
In the fast-paced world of software development, Agile practices have redefined how teams deliver high-quality products. As organizations embrace Agile methodologies, automated testing has become a pillar of software quality assurance. However, it’s not all smooth sailing. Let's explore the most common challenges encountered in automated Agile testing and how to overcome them.
Understanding Automated Agile Testing
Automated Agile testing utilizes automated tools and scripts to validate the functionality, performance, and security of software applications throughout their development cycle. This approach complements Agile values by ensuring faster feedback loops and continuous integration.
Benefits of Automated Agile Testing
- Speed: Automated tests can run much faster than manual tests, allowing for quicker release cycles.
- Consistency: Automated tests produce consistent and repeatable results.
- Efficiency: Test cases can be reused across different test cycles.
- Early Bug Detection: Automated testing allows teams to identify bugs early in the development process.
Common Challenges in Automated Agile Testing
While the benefits are clear, there are several challenges that teams face when implementing automated testing in an Agile environment:
1. Incomplete Test Coverage
The Challenge
One of the primary hurdles is achieving comprehensive test coverage. Agile environments are dynamic, with frequent changes to user stories and requirements. This often leads to essential test cases being overlooked.
The Solution
Establish a clear mapping between user stories and test cases. Regularly review your test suite against new features and changes to maintain alignment. Utilize tools like Cucumber for behavior-driven development (BDD) to ensure that your tests are focused on the desired behavior of the application.
// Sample Cucumber test in Java
@Given("the user is on the homepage")
public void userOnHomepage() {
driver.get("http://example.com");
}
@When("the user clicks the login button")
public void userClicksLogin() {
WebElement loginButton = driver.findElement(By.id("login"));
loginButton.click();
}
@Then("the user should see the login form")
public void userSeesLoginForm() {
assertTrue(driver.findElement(By.id("loginForm")).isDisplayed());
}
2. Tool Selection
The Challenge
Choosing the right testing tool can be daunting given the plethora of options available. Each tool comes with its strengths and weaknesses, and the wrong choice could derail the entire testing process.
The Solution
Evaluate the tools based on your project requirements, team skillset, and integration capabilities with existing systems. Popular options include Selenium for browser automation, TestNG for managing automated tests, and JUnit for unit testing.
3. Maintenance of Test Scripts
The Challenge
As the application evolves, so do the test scripts. Test maintenance can become burdensome, especially when automated tests frequently fail due to minor UI changes.
The Solution
Employ best practices for writing maintainable test scripts:
- Use Page Object Model (POM): Separating page logic from test scripts enhances readability and maintainability.
public class LoginPage {
private WebDriver driver;
public LoginPage(WebDriver driver) {
this.driver = driver;
}
public void clickLogin() {
driver.findElement(By.id("login")).click();
}
public boolean isLoginFormVisible() {
return driver.findElement(By.id("loginForm")).isDisplayed();
}
}
- Implement Regular Refactoring: Schedule periodic reviews of your test scripts to refactor them as needed.
4. Lack of Skilled Resources
The Challenge
Automated testing requires a specific skill set that may be lacking in your team. Testing frameworks often necessitate knowledge of programming and best practices in automation.
The Solution
Invest in training and development for your team. Online platforms like Udemy and Coursera offer courses on automated testing. Pair less experienced members with knowledgeable team members for mentorship.
5. Ineffective Communication Within Teams
The Challenge
In Agile, collaboration among team members is critical. Poor communication can lead to misunderstanding requirements and subsequently inadequate test coverage.
The Solution
Adopt tools for improved collaboration. Platforms like Jira help in tracking issues and testing progress. Establish daily stand-ups to ensure everyone is aligned on testing efforts.
6. Continuous Feedback Loop
The Challenge
Automated tests need to be integrated into the continuous integration (CI) pipeline, which can be cumbersome for teams not familiar with CI/CD practices.
The Solution
Utilize CI tools like Jenkins or CircleCI to integrate automated testing seamlessly. Ensure that your test suite runs on every code check-in, providing immediate feedback to developers.
pipeline {
agent any
stages {
stage('Build') {
steps {
sh 'mvn clean package'
}
}
stage('Test') {
steps {
sh 'mvn test'
}
}
}
}
7. Handling Flaky Tests
The Challenge
Flaky tests often result from environmental issues or timing problems, leading to unreliable results that can significantly hinder progress.
The Solution
Implement stability measures:
- Utilize explicit waits to handle dynamic elements.
- Group tests logically to reduce dependencies between them.
Lessons Learned: Embracing Automated Agile Testing
Automated Agile testing is not without its obstacles, but with a strategic approach, these challenges can be effectively navigated. Emphasizing collaboration, communication, and continuous improvement is essential in this journey.
Further Reading
- For more insights into the Agile testing landscape, you might find Agile Testing by Lisa Crispin and Janet Gregory enlightening.
- Keep up with the latest trends in testing tools through Ministry of Testing.
Establishing a robust automated testing framework is a significant step towards delivering top-tier software products. Address these challenges head-on, and elevate your Agile testing practices to the next level. Happy testing!