Common Pitfalls When Writing Your First Maven Plugin
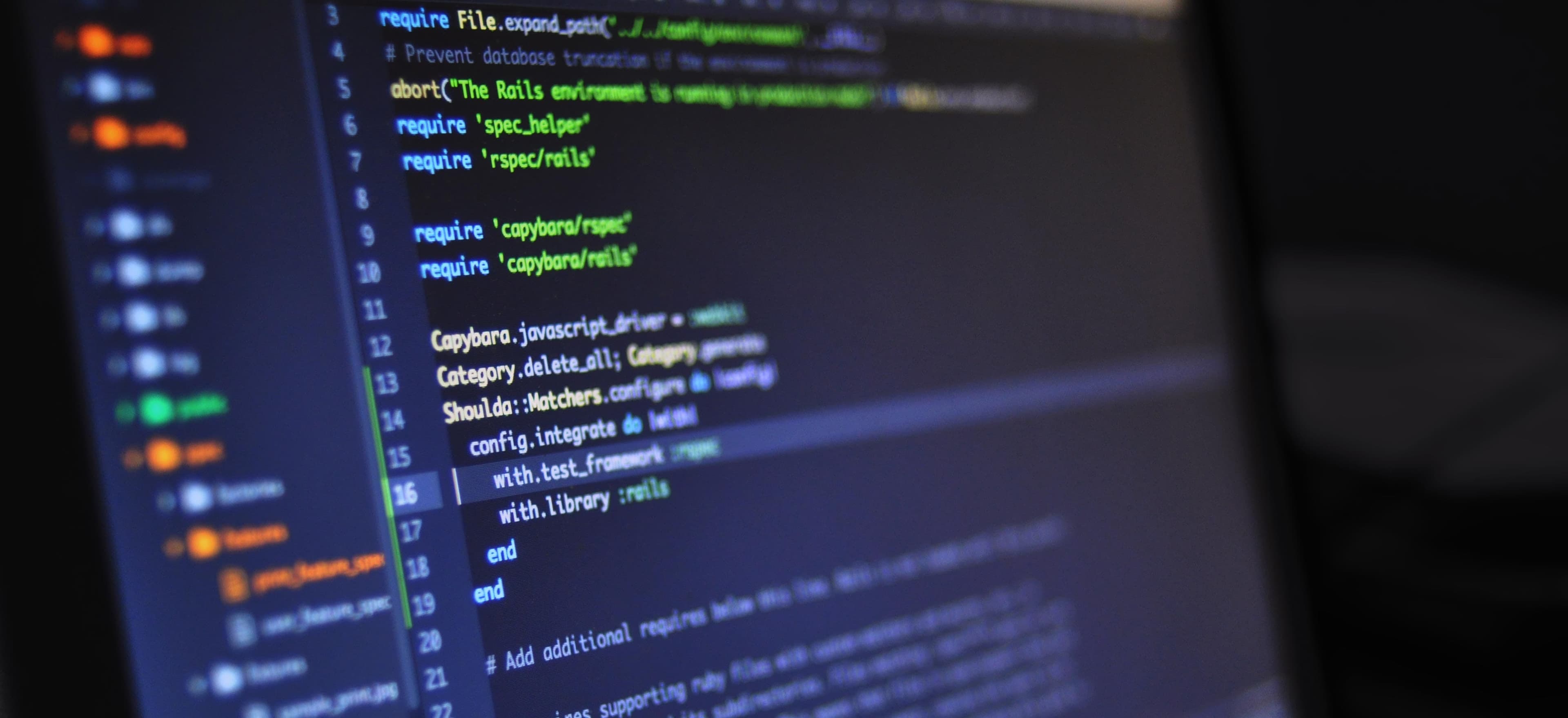
- Published on
Common Pitfalls When Writing Your First Maven Plugin
Creating a custom Maven plugin can seem daunting, especially for newcomers. Maven, a build automation tool used primarily for Java projects, provides an extensible framework for managing builds, dependencies, and project structures. However, developing a Maven plugin requires an understanding of both the Maven lifecycle and the Plugin API. In this blog post, we will explore the common pitfalls faced by developers when writing their first Maven plugin and how to avoid them.
What is a Maven Plugin?
A Maven plugin is essentially a collection of goals that can be added to the Maven build lifecycle. It allows developers to extend Maven's capabilities to meet specific project requirements. These plugins can perform numerous tasks, such as code generation, testing, packaging, and more.
Why Create a Custom Maven Plugin?
Creating a Maven plugin offers several benefits:
- Reusability: You can encapsulate common build processes into a plugin that can be reused across different projects.
- Customization: Tailor builds to fit specific needs not addressed by existing plugins.
- Collaboration: Share your plugins with others in the developer community.
Key Pitfalls When Developing a Maven Plugin
1. Ignoring the Maven Lifecycle
One of the most important aspects of Maven is its build lifecycle, which defines the order in which tasks are executed. A common mistake is to ignore this lifecycle. When designing your plugin, ensure that your goals are appropriately bound to lifecycle phases.
Example
@Mojo(name = "my-goal", defaultPhase = LifecyclePhase.INSTALL)
public class MyGoalMojo extends AbstractMojo {
public void execute() throws MojoExecutionException {
getLog().info("Executing my custom goal...");
}
}
In this code snippet, we define a goal that will be executed during the INSTALL
phase. Binding your goal to an appropriate lifecycle phase ensures it executes within the correct context.
2. Not Defining Configuration Properties
Configuration parameters play a critical role in how your plugin behaves. Failing to define them can lead to hardcoded values that limit flexibility and usability.
Example
@Parameter(defaultValue = "${project.build.directory}", property = "outputDirectory")
private File outputDirectory;
This example shows how to define a configuration property. This allows users of your plugin to specify a different output directory without needing to modify the plugin code directly.
3. Neglecting Dependency Management
Maven manages dependencies through the pom.xml
file. Yet, first-time plugin developers may overlook the necessity of declaring dependencies in the project's pom.xml
.
Example
<dependencies>
<dependency>
<groupId>org.apache.maven</groupId>
<artifactId>maven-plugin-api</artifactId>
<version>${maven.version}</version>
<scope>provided</scope>
</dependency>
</dependencies>
In the above code snippet, we declare the maven-plugin-api
as a dependency. Make sure to specify the correct scope, typically "provided" for Maven plugins, so that it does not get included in the final build artifact.
4. Not Testing the Plugin
Testing is essential for any software development process, constructing a reliable Maven plugin is no exception. Common pitfalls include failing to write unit or integration tests and not validating that the plugin works as intended.
Example
You can use the Maven Plugin Testing Harness to test your plugin:
public class MyGoalMojoTest {
@Test
public void testExecute() throws Exception {
MyGoalMojo mojo = new MyGoalMojo();
mojo.setLog(new Slf4jLogger());
mojo.execute();
// Assert statements go here
}
}
Leveraging testing tools ensures your plugin performs reliably across different Maven versions and circumstances.
5. Hardcoding Values
Hardcoding values makes your plugin inflexible and difficult to maintain. Values like file paths or configurations should be parameterized to enable customization.
Example
@Parameter(defaultValue = "default-output.txt", property = "outputFile")
private String outputFile;
By parameterizing this value, users can easily change the output file name and location without modifying the source code.
6. Poor Error Handling
Handling errors gracefully is crucial for providing a good user experience. Avoiding exceptions can lead to unexpected behavior and a frustrating experience for the users of your plugin.
Example
public void execute() throws MojoExecutionException {
try {
// logic here
} catch (SpecificException e) {
throw new MojoExecutionException("Specific error occurred", e);
}
}
Implementing error handling allows users to understand what went wrong and how to rectify it, improving the overall robustness of your plugin.
7. Not Using Existing Plugins
Finally, one of the biggest pitfalls is not leveraging existing Maven plugins. Often, the functionality you seek is already implemented in another plugin. Investigate repositories like Maven Central before reinventing the wheel.
Best Practices for Writing Maven Plugins
- Follow Convention over Configuration: Stick to Maven's conventions so experienced users will understand your plugin.
- Keep it Simple: Start with a minimal implementation and gradually add features.
- Document Your Plugin: Providing clear documentation helps users understand how to use your plugin and reduces support issues.
- Version Control: Use semantic versioning to maintain backward compatibility and functionality.
- Continuous Integration: Set up a CI/CD pipeline to run tests and validate each change made to the plugin.
Key Takeaways
Writing your first Maven plugin is a rewarding experience that can significantly enhance your projects and those of the community. By avoiding the common pitfalls outlined in this post, you can create a robust, flexible, and user-friendly plugin. Use the right tools and adopt best practices to smooth the learning curve and ensure success.
For more information on writing Maven plugins, consider checking out the Apache Maven Plugin Development Guide for comprehensive resources and tutorials.
As you embark on your journey to create your first Maven plugin, remember that every challenge you face is an opportunity to learn and grow. Happy coding!