How to Use Constants for Enum Annotations in Java
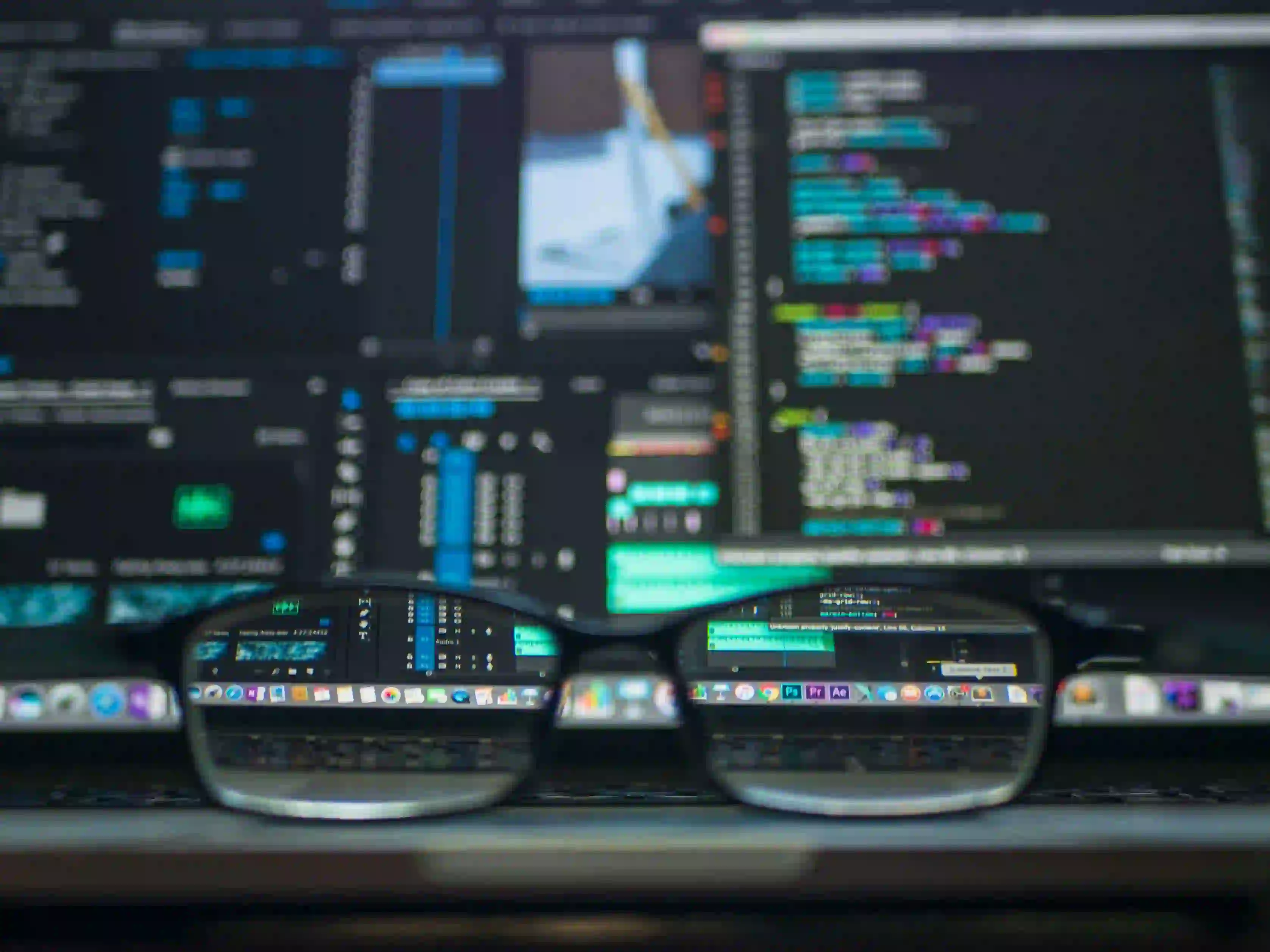
How to Use Constants for Enum Annotations in Java
Enums in Java are much more than just simple constants; they can hold fields, methods, and even annotations. Utilizing constants for enum annotations can enhance your codebase by making it more readable, maintainable, and less error-prone. In this blog post, we will discuss how to use constants for enum annotations, why it's beneficial, and share some code snippets to clarify the concepts.
What are Enums?
First, let's clarify what an enum is. An enum (short for enumeration) is a special Java type used to define collections of constants. Enums are more powerful than simple enums, as they can have fields, methods, and constructors.
Here’s a simple example of an enum:
public enum Day {
SUNDAY, MONDAY, TUESDAY, WEDNESDAY, THURSDAY, FRIDAY, SATURDAY;
}
In the above code, Day
is an enum that lists the days of the week.
Why Use Annotations with Enums?
Annotations provide information about the program but have no effect on the operation of the code. They can serve various purposes, from providing metadata that is processed by tools to validating the values of fields.
Using constants for enum annotations can be beneficial in several ways:
- Readability: Constants improve readability, as you convey meaning behind the number or string.
- Maintainability: If you need to change the value, you only have to do it in one place.
- No Magic Numbers: Avoid using "magic numbers" or hardcoded strings in your code.
How to Annotate Enums
You can provide enums with annotations by defining them as constant metadata. Consider the scenario where you have different types of users in your application (e.g., Admin, User, Moderator) and you want to associate roles with each enum type.
@Retention(RetentionPolicy.RUNTIME)
@Target(ElementType.FIELD)
public @interface Role {
String value();
}
In this snippet, we define an annotation called Role
, which can be assigned to fields and represents a string value.
Adding Annotations to Enum Constants
Next, let's create an enum with annotated constants indicating user roles.
public enum UserRole {
@Role("Admin")
ADMIN,
@Role("General User")
USER,
@Role("Content Moderator")
MODERATOR;
}
In this case, each constant in the UserRole
enum has an associated @Role
annotation.
How to Access the Annotations
To extract the annotation value at runtime, you can use reflection. Below is a method demonstrating how to retrieve the role associated with the enum constants:
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Target;
import java.lang.annotation.ElementType;
import java.lang.reflect.Field;
public class EnumAnnotationExample {
public static void main(String[] args) {
for (UserRole role : UserRole.values()) {
Role annotation = getRoleAnnotation(role);
if (annotation != null) {
System.out.println(role + ": " + annotation.value());
}
}
}
private static Role getRoleAnnotation(UserRole role) {
try {
Field field = role.getDeclaringClass().getField(role.name());
return field.getAnnotation(Role.class);
} catch (NoSuchFieldException e) {
e.printStackTrace();
return null;
}
}
}
Explanation
- Reflection: We utilize Java Reflection to access the fields of our enum.
- getRoleAnnotation(Role): This method fetches the
Role
annotation for eachUserRole
.
Advantages of Using Enum Annotations
- Centralized Control: The roles are defined in a central location and can easily be managed.
- Type Safety: Enums provide compile-time type safety, which reduces errors.
- Clearer APIs: When using these enums in methods, the intention is clear.
Handling Different Value Types
While we used strings for our Role
annotation, Java annotations can also be parameterized with other types, such as enums or even arrays.
Example of Parameterized Annotations
Consider extending our previous annotation to accept multiple roles:
@Retention(RetentionPolicy.RUNTIME)
@Target(ElementType.FIELD)
public @interface Roles {
String[] value();
}
Then, your enum can look like this:
public enum UserRole {
@Roles({"Admin", "User"})
ADMIN,
@Roles({"User"})
USER,
@Roles({"Moderator"})
MODERATOR;
}
Accessing Parameterized Annotations
You can modify the getRoleAnnotation
method to fetch multiple roles from the annotation:
private static Roles getRolesAnnotation(UserRole role) {
try {
Field field = role.getDeclaringClass().getField(role.name());
return field.getAnnotation(Roles.class);
} catch (NoSuchFieldException e) {
e.printStackTrace();
return null;
}
}
// Output method showcasing the roles for each user.
for (UserRole role : UserRole.values()) {
Roles annotation = getRolesAnnotation(role);
if (annotation != null) {
System.out.println(role + ": " + Arrays.toString(annotation.value()));
}
}
Real-World Scenario
In a real-world application, you might manage permissions within roles. For example, using a Permission
enum with an annotation can streamline permission management:
@Retention(RetentionPolicy.RUNTIME)
@Target(ElementType.FIELD)
public @interface Permission {
String value();
}
public enum UserPermissions {
@Permission("READ_PRIVILEGES")
READ,
@Permission("WRITE_PRIVILEGES")
WRITE,
@Permission("DELETE_PRIVILEGES")
DELETE;
}
Summary
Using constants for enum annotations in Java is a powerful technique that brings numerous benefits, including better readability, maintainability, and type safety. By creatively employing annotations within enums, we can create versatile and expressive code.
To deepen your understanding of Java enums and annotations, you may find these resources useful:
Incorporating constants for enum annotations could transform your codebase into a more robust, error-resistant architecture. Start applying these concepts today, and see the difference firsthand!
This Java-focused blog post should serve as a comprehensive guide for developers looking to leverage the power of constants within enum annotations to write cleaner and more maintainable code.