Mastering DynamicReports: Overcoming JSF Integration Issues
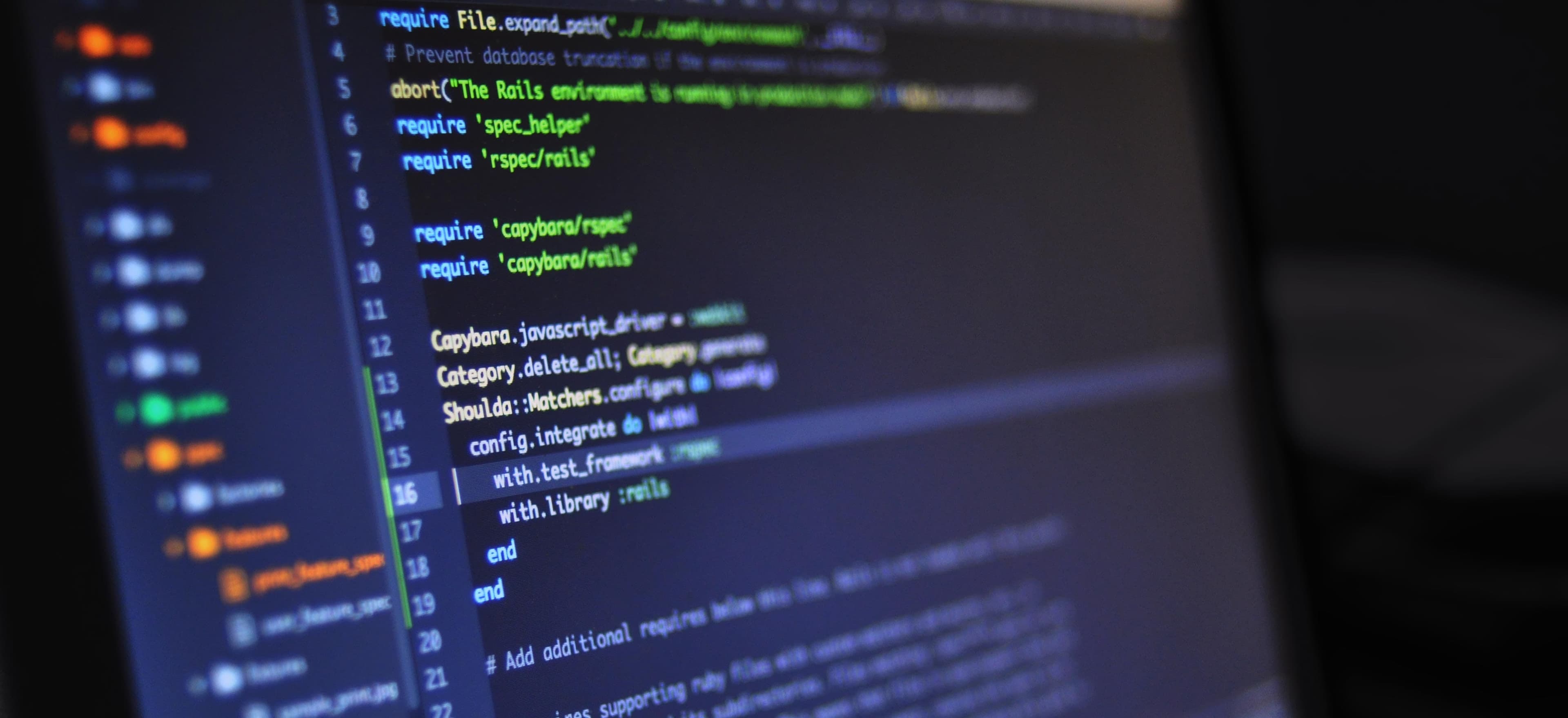
- Published on
Mastering DynamicReports: Overcoming JSF Integration Issues
DynamicReports is a powerful Java reporting library that leverages JasperReports under the hood, providing a simplified API for creating reports. While it offers several advantages, integrating it with JavaServer Faces (JSF) can sometimes pose challenges. In this blog post, we will explore the common issues you might face and detail strategies to overcome them.
Table of Contents
- What is DynamicReports?
- Why Use DynamicReports with JSF?
- Common Integration Issues
- Workaround Strategies
- Example Code Snippets
- Conclusion
What is DynamicReports?
DynamicReports is an open-source reporting library designed to simplify the creation of reports for the Java platform. It allows developers to create complex reports using a fluent API, saving time and reducing boilerplate code. This library supports both simple and advanced reporting needs, making it scalable for various applications.
For more information about DynamicReports, you can check DynamicReports Documentation.
Why Use DynamicReports with JSF?
JavaServer Faces (JSF) is a Java specification for building component-based user interfaces for web applications. It enables developers to create rich user interfaces with less hassle. Combining DynamicReports with JSF provides several benefits:
- Rich Reporting Features: Generate complex reports across diverse formats.
- Easy Integration: Leverage JSF's managed bean capabilities to collect and pass parameters for reports.
- Controller-View Separation: Maintain clean architecture by separating business logic from presentation.
By using both together, developers can harness the strengths of DynamicReports while retaining JSF's structured and component-based architecture.
Common Integration Issues
While DynamicReports and JSF work well in theory, several common integration issues often arise:
- ClassNotFoundException: You might encounter class loading issues due to missing dependencies, especially when working with complex projects.
- PDF Rendering Issues: Sometimes, reports generated in PDF format might not render correctly in the browser, causing unexpected output.
- JSF ViewState Problems: Large data sets can lead to issues with JSF's view state serialization.
Workaround Strategies
Here are effective strategies to resolve integration challenges between DynamicReports and JSF.
1. ClassNotFoundException
Ensure you have all the necessary dependencies in your classpath. Use a build tool like Maven or Gradle to manage these dependencies effectively.
<!-- Maven Dependencies -->
<dependency>
<groupId>net.sf.dynamicreports</groupId>
<artifactId>dynamicreports-core</artifactId>
<version>6.12.0</version>
</dependency>
<dependency>
<groupId>net.sf.jasperreports</groupId>
<artifactId>jasperreports</artifactId>
<version>6.15.0</version>
</dependency>
Why this matters: Using Maven allows you to easily update libraries, and it helps ensure that all necessary libraries are present.
2. PDF Rendering Issues
Sometimes, generated PDF reports may not be displayed correctly. This can be a configuration issue. Ensure that the appropriate configuration settings in the application’s web.xml file are set up for content types.
<servlet>
<servlet-name>reportServlet</servlet-name>
<servlet-class>net.sf.jasperreports.engine.JasperExportServle</servlet-class>
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>reportServlet</servlet-name>
<url-pattern>/reports/*</url-pattern>
</servlet-mapping>
Why this matters: Proper servlet mapping ensures that the requests for generating reports are handled correctly, reducing the chances of errors.
3. JSF ViewState Problems
For large datasets, consider using dynamic paging. This allows you to load data in chunks, which can help mitigate issues with JSF’s view state.
A simple example on how to manage pagination:
@ManagedBean
@ViewScoped
public class ReportBean {
private List<MyData> dataList;
private int pageSize = 50;
public void loadData(int pageIndex) {
int start = pageIndex * pageSize;
dataList = loadDataFromDatabase(start, pageSize); // Implement pagination in this method
}
}
Why this matters: It helps control the volume of data loaded at any one time, which directly impacts JSF view state performance.
Example Code Snippets
Now that we've covered potential issues and workarounds, let's look at a more comprehensive example of using DynamicReports with JSF.
Basic Report Generation
Here is a simple snippet for generating a PDF report using a JSF managed bean:
import net.sf.dynamicreports.report.builder.DynamicReports;
import net.sf.dynamicreports.report.exception.DynamicReportsException;
@ManagedBean
@ViewScoped
public class ReportBean {
public void generateReport() {
try {
JasperReportBuilder report = DynamicReports.report();
report.columns(
col.column("Column 1", "column1", type.stringType()),
col.column("Column 2", "column2", type.integerType())
)
.title("My Report Title")
.pageHeader(cmp.text("Report Header"))
.setDataSource(createDataSource());
// Export to PDF
exportToPdf(report);
} catch (DynamicReportsException e) {
e.printStackTrace();
}
}
private JRDataSource createDataSource() {
// Create and return your data source
return new JRBeanCollectionDataSource(getDataFromDatabase());
}
private void exportToPdf(JasperReportBuilder report) {
// Your PDF export logic here
}
}
Why this matters: The code demonstrates how to build a report using DynamicReports and how to integrate it within a JSF managed bean structure seamlessly.
Closing Remarks
Integrating DynamicReports with JSF can present challenges, but with the right strategies and understanding of the libraries, you can overcome these hurdles effectively. By addressing class loading issues, ensuring proper PDF rendering, and utilizing dynamic paging for large datasets, developers can enjoy the best of both worlds: powerful reporting capabilities and a responsive user interface.
As you delve deeper into DynamicReports and JSF integration, do not hesitate to explore additional resources such as JasperReports Integration for advanced configurations and features.
By mastering these skills, you can produce elegant, high-quality reports that enhance the functionality and user experience of your Java web applications. Happy coding!
Checkout our other articles