Maximizing Direct Memory Size for Default Hotspot Configuration
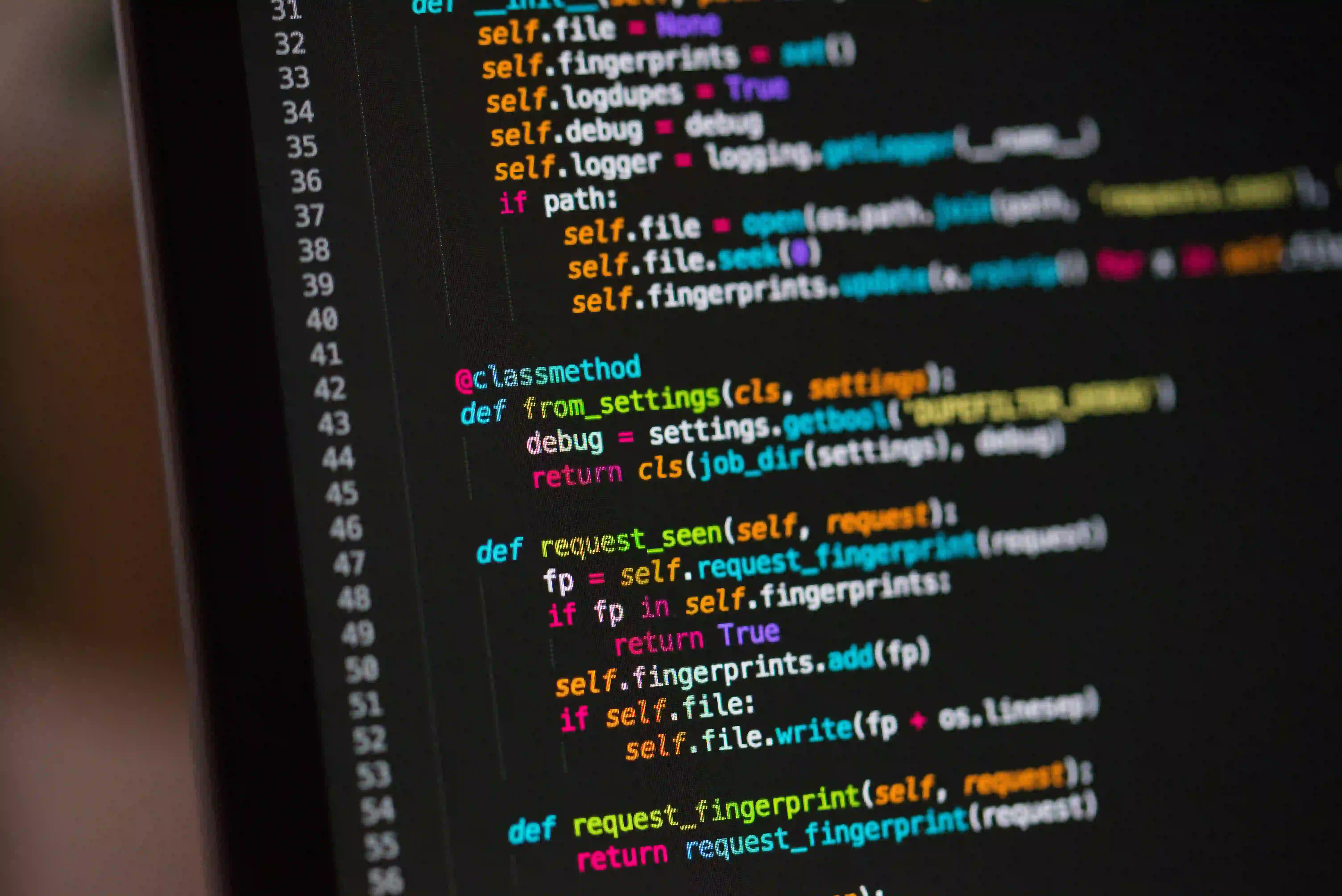
Maximizing Direct Memory Size for Default HotSpot Configuration in Java
When dealing with Java applications, particularly those requiring high performance and low latency, optimizing memory usage becomes critical. One of the key areas developers should pay attention to is Direct Memory. In this blog post, we will explore how to maximize Direct Memory Size for the default HotSpot configuration, understand the implications, and provide some practical coding examples to illustrate these concepts.
Understanding Direct Memory in Java
Direct Memory in Java refers to the memory areas allocated outside the Java heap. This memory is managed by the Operating System and Java provides the java.nio
package which allows for efficient memory management. Direct memory access is particularly useful in applications that require high throughput and low latency, such as database clients and high-performance networking applications.
Benefits of Using Direct Memory
- Performance: Direct memory access eliminates the need for Java’s garbage collection, reducing latency.
- Less Overhead: Allows for the management of large buffers without the overhead of the heap.
- Native Buffering: Accessing memory in a native way can lead to better performance compared to heap-based memory.
Configuring Direct Memory in HotSpot
Java's HotSpot JVM has a default limit on the amount of direct memory that can be allocated. By default, this limit is set to a percentage of the maximum heap size, often making it less than ideal for memory-intensive applications. However, it's relatively simple to configure the maximum size of Direct Memory using JVM options.
JVM Options for Direct Memory
To specify the maximum size of Direct Memory, you can use the -XX:MaxDirectMemorySize
flag. The default value is set to the same size as the heap, but for systems demanding higher throughput, this can be adjusted.
java -XX:MaxDirectMemorySize=2g -jar your-app.jar
In this example, we allocate 2GB of Direct Memory. The optimal size will depend on the specific requirements and workload of your application.
Key Considerations
When increasing Direct Memory, keep the following in mind:
- Analyze the memory requirements of your application beforehand.
- Continuously monitor application performance; adjusting these values can have unexpected effects.
- Ensure your system can manage the increased memory allocation both in terms of hardware capabilities and operating system configurations.
Practical Example: Using DirectByteBuffer
To illustrate how to utilize Direct Memory in Java, we can create a simple example involving DirectByteBuffer
.
Step 1: Allocating Direct Memory
Allocating a DirectByteBuffer
involves the following code:
import java.nio.ByteBuffer;
public class DirectMemoryExample {
public static void main(String[] args) {
int size = 1024; // Size of buffer
ByteBuffer directBuffer = ByteBuffer.allocateDirect(size);
// Fill the buffer with sample data
for (int i = 0; i < size; i++) {
directBuffer.put((byte) i);
}
// Read the data back into an array
directBuffer.flip(); // Preparing buffer for reading
byte[] array = new byte[size];
directBuffer.get(array);
printBuffer(array);
}
private static void printBuffer(byte[] array) {
for (byte b : array) {
System.out.print(b + " ");
}
}
}
Why Use DirectByteBuffer
?
- Non-blocking I/O: This buffer is particularly useful in NIO (New I/O) operations which need non-blocking behavior and greater efficiency.
- Memory Management: It allocates memory outside of the garbage-collected heap, thus preventing frequent GC pauses which may happen during critical operations.
Step 2: Handling Memory Efficiently
While using direct buffers, never forget to manage memory. Unlike heap memory, direct byte buffers are not subject to garbage collection.
To ensure efficient memory usage, you should always clear or release the DirectByteBuffer
when it is no longer needed using clean()
method, although it requires reflection for direct access since it has no public method.
Example Code for Cleanup:
import java.lang.reflect.Method;
import java.nio.ByteBuffer;
public class MemoryCleanupExample {
public static void clean(ByteBuffer buffer) throws Exception {
// Access the cleaner of the buffer and invoke it
Method cleanerMethod = buffer.getClass().getMethod("cleaner");
cleanerMethod.setAccessible(true);
Object cleaner = cleanerMethod.invoke(buffer);
Method cleanMethod = cleaner.getClass().getMethod("clean");
cleanMethod.invoke(cleaner);
}
public static void main(String[] args) throws Exception {
ByteBuffer directBuffer = ByteBuffer.allocateDirect(1024);
// Use the buffer
clean(directBuffer); // Clean up memory explicitly
}
}
Monitoring Memory Usage
Monitoring the memory usage of direct buffers can be achieved through various profiling tools. Some popular JVM profilers include:
- VisualVM: A free tool that provides insight into memory and CPU performance.
- Java Mission Control: A tool for monitoring and analyzing performance in Java.
For more advanced applications, consider integrating Java Flight Recorder which is built into Oracle JDK.
Key Takeaways
Maximizing the Direct Memory Size is a crucial step for developers looking to boost the performance of their Java applications. Utilizing the -XX:MaxDirectMemorySize
flag along with proper usage of DirectByteBuffer
can lead to significant improvements in throughput and responsiveness.
Always consider your application's specific needs and configurations when tweaking these parameters. With careful planning and monitoring, you will optimize your application for performance, reducing the risk of latency and improving user experience.
For further reading on memory management in Java and advanced topics on Direct Memory, you can check out Java SE Documentation.
Happy coding!