Resolving Illegal Start of Expression Errors in Java
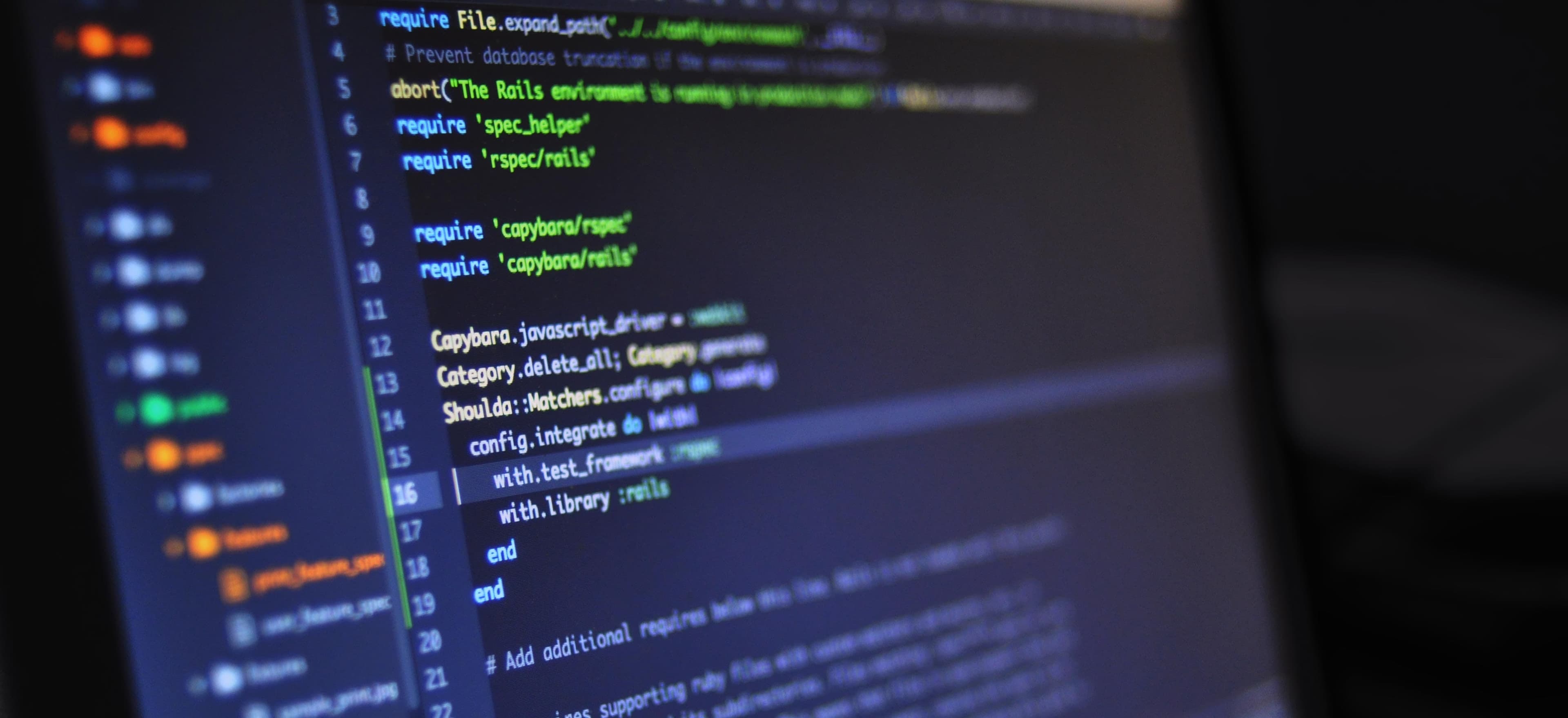
- Published on
Resolving Illegal Start of Expression Errors in Java
When coding in Java, encountering errors is part of the development process. One common error that often frustrates developers, especially those new to the language, is the "Illegal Start of Expression." This error can be perplexing and may appear within a variety of contexts. In this blog post, we will delve into what causes this error, explore how to identify it, and discuss strategies to rectify it—all while providing practical code snippets for clarity.
Understanding the Error
The "Illegal Start of Expression" error indicates a syntax problem within your Java code. Java, being a heavily syntax-driven language, has stringent rules for how code structures should be formed. This error can arise from various mistakes, such as misplacing a keyword, omitting a necessary semicolon, or incorrectly structuring control statements.
Common Causes of the 'Illegal Start of Expression' Error
- Missing or Misplaced Curly Braces: Java uses curly braces to define the scope of methods and classes.
- Incorrect Use of Keywords: Using keywords inappropriately or at the wrong context can lead to this error.
- Syntax Errors in Variable Declarations: Declaring a variable incorrectly.
- Method Definitions: Forgetting to include return types or incorrectly chaining modifiers.
Example of the Error
Let's examine a simple example to demonstrate how this error can manifest in your code.
public class Example {
public static void main(String[] args) {
int x = 5
System.out.println("Value of x: " + x);
}
}
In this code snippet, we can see that there is a missing semicolon at the end of the line where int x = 5
is defined. Thus, Java will respond with an "Illegal Start of Expression" error on the line containing System.out.println
.
How to Identify the Error
Before we jump into solutions, it is essential to know how to identify where this error occurs. When you compile your code, the Java compiler gives you an error message that often points to the line you should investigate. Here's a step-by-step guide to help you track the source of the problem:
- Examine the Line Indicated by the Compiler: The compiler error messages will often point to the line number where it suspects the error lies.
- Check the Surrounding Context: Sometimes, the actual mistake may be on the previous line, as Java reads lines sequentially.
- Look for Missing or Misplaced Syntax Elements: Semicolons, parentheses, and braces are common culprits.
Strategies to Resolve the Error
Identifying the issue is only half the battle—let's discuss some strategies to fix the "Illegal Start of Expression" error.
1. Correcting Syntax Mistakes
Always ensure that your statements end with the appropriate semicolon.
Code Snippet:
public class CorrectionExample {
public static void main(String[] args) {
int x = 5; // Added semicolon to denote end of statement
System.out.println("Value of x: " + x);
}
}
2. Properly Structure Control Statements
Improperly structured control statements such as if
, for
, or while
can also instigate this error. For instance, an if
might be incorrectly formed.
Code Snippet:
public class ControlStatement {
public static void main(String[] args) {
int x = 10;
if (x > 5) // Missing curly brace
System.out.println("x is greater than 5");
else
System.out.println("x is less than or equal to 5");
}
}
In this case, you may want to introduce curly braces to ensure clarity and correct syntax.
public class ControlStatement {
public static void main(String[] args) {
int x = 10;
if (x > 5) {
System.out.println("x is greater than 5");
} else {
System.out.println("x is less than or equal to 5");
}
}
}
3. Ensure Proper Variable Declaration
When declaring variables, ensure that their data types and initializations are accurately placed.
Code Snippet:
public class VariableDeclaration {
public static void main(String[] args) {
int x = 5 // Error: Missing semicolon
String message = "Hello, World!";
System.out.println(message);
}
}
Fixed Code:
public class VariableDeclaration {
public static void main(String[] args) {
int x = 5; // Fixed by adding the missing semicolon
String message = "Hello, World!";
System.out.println(message);
}
}
4. Double-Check Method Definition
Make sure that method definitions include all necessary components, such as return types.
Code Snippet:
public class MethodDefinition {
public static void main(String[] args) {
displayMessage("Hello");
}
void displayMessage(String msg) { // Error: Missing 'public static' on method
System.out.println(msg);
}
}
The corrected definition should look like this:
public class MethodDefinition {
public static void main(String[] args) {
displayMessage("Hello");
}
public static void displayMessage(String msg) { // Correcting method visibility
System.out.println(msg);
}
}
Closing the Chapter
Encountering the "Illegal Start of Expression" error in Java can be frustrating, particularly for newcomers. However, understanding its causes and learning strategies to detect and rectify the issue can significantly enhance your coding experience.
Remember to scrutinize your syntax diligently, pay attention to the compiler messages, and employ best practices in your code structure.
If you want to dive deeper into error handling in Java, consider checking out Java Exception Handling for a broader understanding of managing various errors in Java effectively.
By being vigilant and adhering to syntax rules, you can sail through the challenges presented by Java programming with greater ease. Happy coding!
Checkout our other articles