Common Pitfalls in Java's Iterator Design Pattern
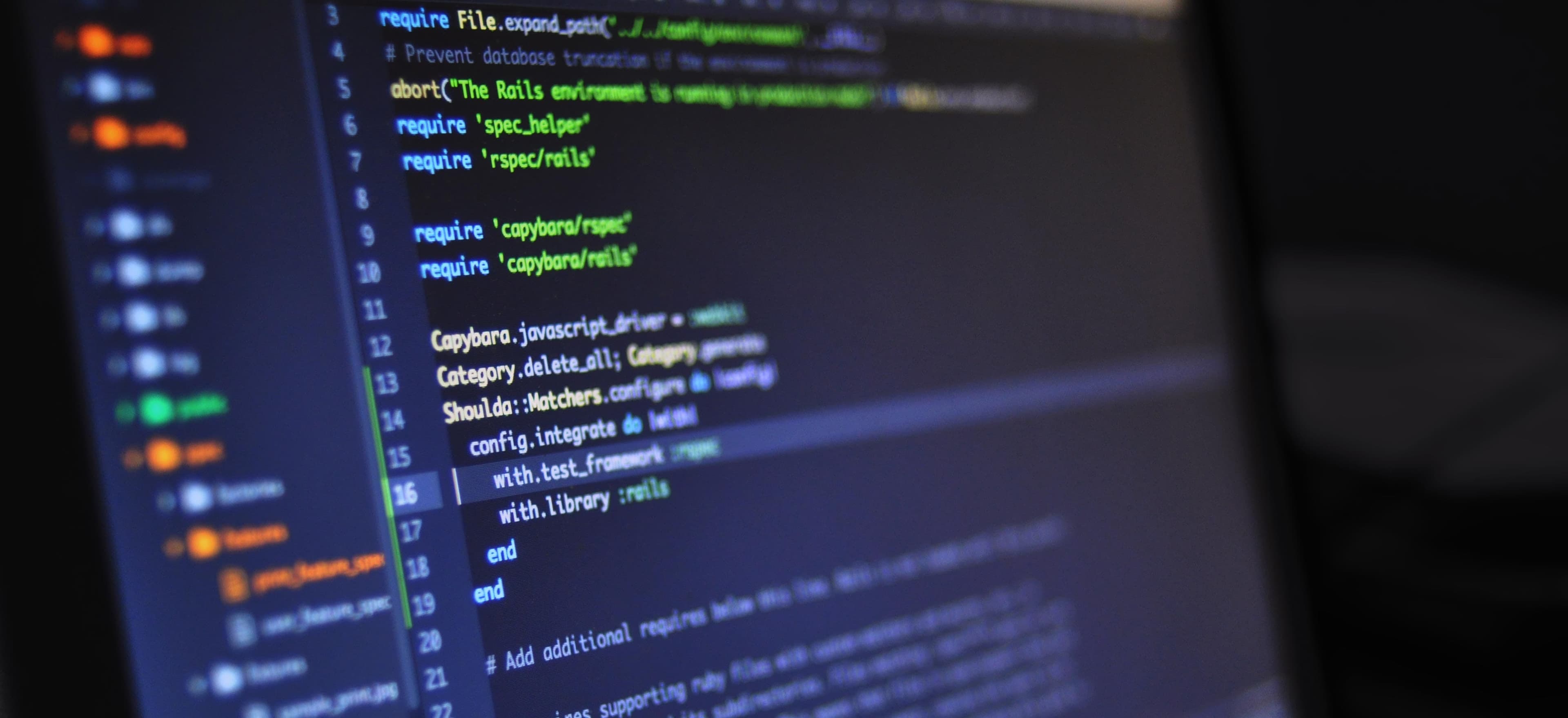
- Published on
Common Pitfalls in Java's Iterator Design Pattern
Java's Iterator Design Pattern is a powerful tool for navigating through collections without exposing their underlying structure. Whether you're dealing with lists, sets, or maps, the Iterator simplifies traversal. However, while its implementation may seem straightforward, several common pitfalls can lead to issues in your code. In this blog post, we'll explore these pitfalls and how to avoid them, coupled with practical examples.
What is the Iterator Design Pattern?
Before diving into the pitfalls, let’s briefly clarify the Iterator design pattern.
The Iterator pattern provides a way to access the elements of an aggregate object sequentially without exposing its underlying representation. In Java, the Iterator interface offers a standard way to enable this behavior. The primary methods of the Iterator interface include:
- hasNext(): Returns
true
if the iteration has more elements. - next(): Returns the next element in the iteration.
- remove(): Removes the last element returned by this iterator.
The Iterator is widely used because it supports the encapsulation of collection details and promotes the decoupling of collection manipulation logic from data structure specifics.
1. Failing to Use Iterator Properly
One of the most common pitfalls is using an iterator incorrectly, such as modifying the collection while iterating over it. This often results in a ConcurrentModificationException
.
Example:
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
public class ConcurrentModificationExample {
public static void main(String[] args) {
List<String> list = new ArrayList<>();
list.add("A");
list.add("B");
list.add("C");
Iterator<String> iterator = list.iterator();
while (iterator.hasNext()) {
String value = iterator.next();
if ("B".equals(value)) {
// Attempting to modify the collection directly
list.remove(value); // This will throw ConcurrentModificationException
}
}
}
}
Why This is a Problem:
Modifying the collection while iterating causes inconsistency since iterators have internal state management that tracks the current position. Proper practice would be to use the iterator’s remove()
method.
Correct Way:
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
public class SafeModificationExample {
public static void main(String[] args) {
List<String> list = new ArrayList<>();
list.add("A");
list.add("B");
list.add("C");
Iterator<String> iterator = list.iterator();
while (iterator.hasNext()) {
String value = iterator.next();
if ("B".equals(value)) {
iterator.remove(); // Safe removal
}
}
System.out.println(list); // [A, C]
}
}
2. Invalidating Iterator State
Another significant pitfall is iterating over a collection that gets modified through non-iterative means. This leads to situations where the iterator’s state gets invalidated.
Example:
import java.util.HashSet;
import java.util.Iterator;
import java.util.Set;
public class InvalidateIteratorStateExample {
public static void main(String[] args) {
Set<String> set = new HashSet<>();
set.add("A");
set.add("B");
set.add("C");
Iterator<String> iterator = set.iterator();
set.add("D"); // Modifying the original collection
while (iterator.hasNext()) {
System.out.println(iterator.next()); // Might throw ConcurrentModificationException
}
}
}
Why This Happens:
When the original collection is changed, it disrupts the current state of the iterator, no longer syncing correctly with the collection.
Good Practice:
Ensure you do not modify the collection directly while an iterator is active. Only use the iterator’s methods.
3. Not Handling the End of Iteration
Another issue arises when dealing with the end of an iteration. Failing to check for the presence of elements before calling next()
can lead to a NoSuchElementException
.
Example:
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
public class EndOfIterationExample {
public static void main(String[] args) {
List<String> list = new ArrayList<>();
list.add("A");
Iterator<String> iterator = list.iterator();
// Incorrect usage of next()
while (true) {
System.out.println(iterator.next()); // This leads to NoSuchElementException when list is empty
}
}
}
Correct Approach:
You should always check hasNext()
before calling next()
.
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
public class SafeIterationExample {
public static void main(String[] args) {
List<String> list = new ArrayList<>();
Iterator<String> iterator = list.iterator();
while (iterator.hasNext()) {
System.out.println(iterator.next()); // Safe since we checked for presence
}
}
}
4. Iterator Not Reusable
Another common misunderstanding is treating an iterator as reusable. Once an iterator is traversed, it cannot be reused to re-tread the collection.
Example:
import java.util.List;
import java.util.ArrayList;
import java.util.Iterator;
public class IteratorReuseExample {
public static void main(String[] args) {
List<String> list = new ArrayList<>();
list.add("A");
list.add("B");
Iterator<String> iterator = list.iterator();
while (iterator.hasNext()) {
System.out.println(iterator.next());
}
// Attempting to reuse the iterator
while (iterator.hasNext()) { // This will not print anything
System.out.println(iterator.next());
}
}
}
Best Practice:
Always create a new iterator instance if you need to iterate over the collection again.
import java.util.List;
import java.util.ArrayList;
public class SafeReuseExample {
public static void main(String[] args) {
List<String> list = new ArrayList<>();
list.add("A");
list.add("B");
// First iteration
Iterator<String> firstIterator = list.iterator();
while (firstIterator.hasNext()) {
System.out.println(firstIterator.next());
}
// New Iterator for second iteration
Iterator<String> secondIterator = list.iterator();
while (secondIterator.hasNext()) {
System.out.println(secondIterator.next());
}
}
}
Closing Remarks
Understanding the common pitfalls associated with the Iterator design pattern in Java is crucial for writing robust and error-free code. From managing concurrent modifications to ensuring safe iteration practices, each of these points highlights the importance of careful collection management.
The Iterator pattern is a powerful abstraction, but like any tool, it requires thoughtful implementation to maximize its benefits. By avoiding these pitfalls, you can improve the reliability and maintainability of your Java applications.
For further reading on design patterns in Java, consider exploring the Design Patterns: Elements of Reusable Object-Oriented Software or checking out the Java documentation for more insights into iterators.
Learning from these examples and refining your approach to using iterators will equip you with a stronger grasp of Java's collection framework. Stay sharp, code smart, and happy coding!
Checkout our other articles