How to Spot and Fix Memory Leaks in Java Applications
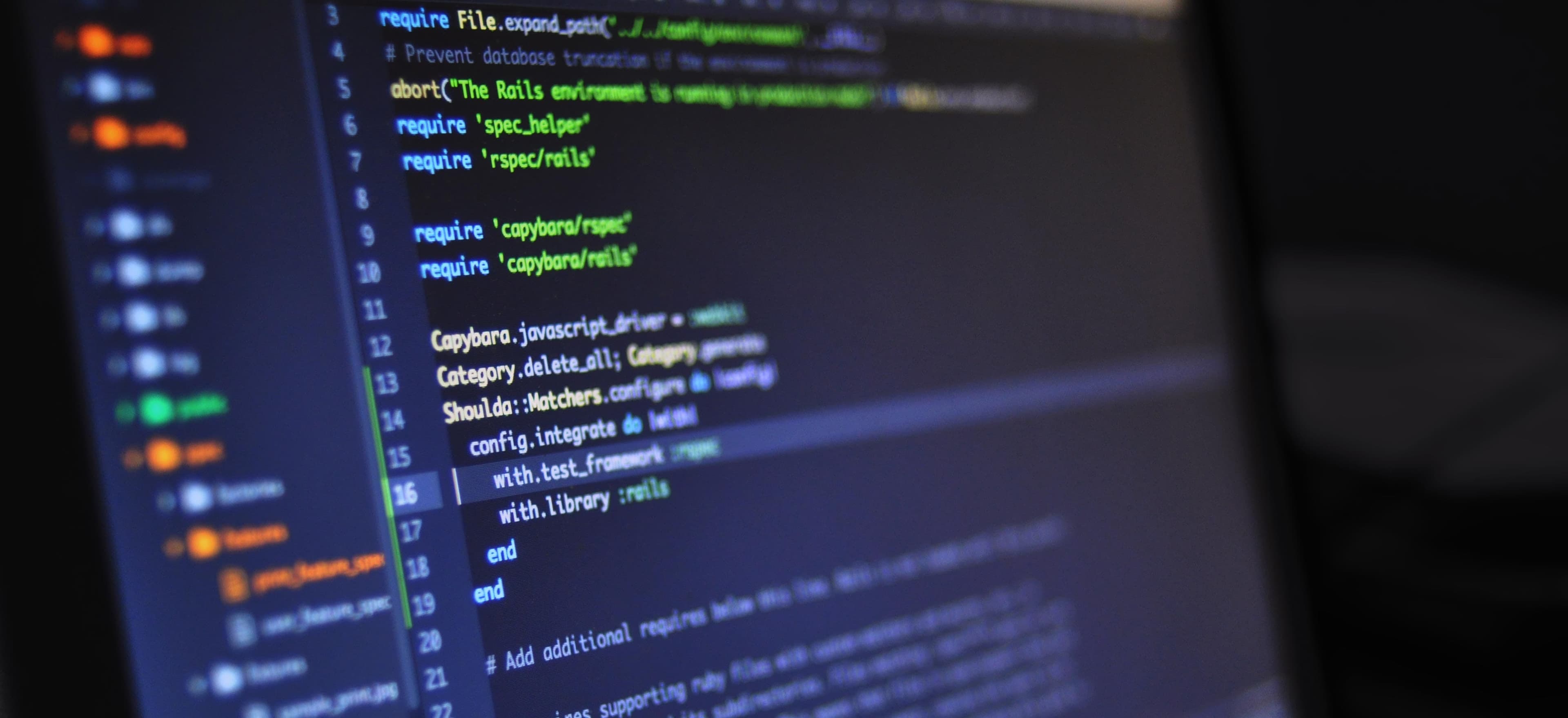
- Published on
How to Spot and Fix Memory Leaks in Java Applications
Memory leaks are a common yet insidious problem in Java applications. The elegance of Java's automatic garbage collection can create a false sense of security. However, improper handling of object references can lead to situations where memory is not being released, resulting in degraded application performance and, in worst cases, application crashes due to OutOfMemoryError.
In this comprehensive guide, we will explore:
- What memory leaks are
- How to identify them
- Tools to help detect and fix memory leaks
- Best practices for preventing memory leaks
What is a Memory Leak?
In the context of programming, a memory leak occurs when an application allocates memory but fails to release it back to the operating system. In Java, this typically happens when objects remain referenced after their use, preventing the garbage collector from reclaiming that memory.
Examples of scenarios that can lead to memory leaks in Java include:
- Unintentional retention of object references (e.g., static data structures, Listener patterns)
- Caches that grow indefinitely
- External resources that are not closed properly
How to Identify Memory Leaks
Identifying memory leaks requires a combination of monitoring your application and analyzing its memory usage. Here are critical steps you can take:
1. Monitoring Memory Usage
Java provides powerful tools, including:
- VisualVM: A monitoring tool that provides detailed statistics about memory consumption and allows you to analyze heap dumps.
- Java Mission Control (JMC): An advanced tool that gives real-time insights into JVM performance without adding overhead.
To monitor memory usage, follow these steps:
-
Install VisualVM: Download it from visualvm.github.io.
-
Collect Heap Dumps: Capture heap dumps when your application is under load.
Example using jcmd to get a heap dump:
jcmd <pid> GC.heap_dump <file-path>
Replace <pid>
with your Java process ID and <file-path>
with where you want to save the heap dump.
2. Analyzing Heap Dumps
Once you have the heap dump, load it into VisualVM or JMC. Look for suspiciously high memory usage or a significant number of objects in memory without apparent need. Pay close attention to collections or caches that grow arbitrarily.
Example Analysis
If you find a collection that holds onto more elements than expected, it might indicate that items are not being removed after use or that they're being referenced in an unintended manner.
Tools for Memory Leak Detection
Apart from the built-in tools, there are third-party tools you might find useful:
- Eclipse Memory Analyzer (MAT): A powerful tool to analyze heap dumps and detect memory leaks.
- YourKit Java Profiler: A commercial profiler that can help track memory usage.
Using Eclipse MAT to Identify Memory Leaks
- Open Eclipse MAT and load your heap dump.
- Navigate to "Leak Suspects" report.
- It will highlight possible memory leaks, providing insights on what objects are retaining memory and their references.
Code Example: Identifying a Memory Leak
Suppose you have a list that retains references to objects. Here's a simple illustration:
import java.util.ArrayList;
import java.util.List;
public class MemoryLeakExample {
private static List<Object> leakedObjects = new ArrayList<>();
public static void createLeak() {
// Leak: Objects are stored indefinitely
for (int i = 0; i < 100000; i++) {
leakedObjects.add(new Object()); // Each object reference will be retained
}
}
public static void main(String[] args) {
createLeak(); // This method creates a memory leak.
}
}
Why is This a Memory Leak?
In the above example, the static list leakedObjects
retains references to an ever-growing number of objects. Unless this list is cleared or managed, it will continue to consume memory, leading to performance degradation.
How to Fix Memory Leaks
Once you have identified a memory leak, it's crucial to implement a fix. Here’s how you can resolve the most common causes:
1. Remove Unused References
In our previous example, we can modify the createLeak
method to clear the list regularly (e.g., after processing).
public static void createLeak() {
// Process objects then clear the list
for (int i = 0; i < 100000; i++) {
leakedObjects.add(new Object());
}
// Clear the list to release references
leakedObjects.clear(); // Fix to prevent memory leak
}
2. Use Weak References
Weak references allow the garbage collector to reclaim memory if the object is only weakly reachable. For example:
import java.lang.ref.WeakReference;
import java.util.HashMap;
public class Cache {
private HashMap<String, WeakReference<Object>> cache = new HashMap<>();
public void addToCache(String key, Object value) {
cache.put(key, new WeakReference<>(value));
}
public Object getFromCache(String key) {
WeakReference<Object> ref = cache.get(key);
return ref != null ? ref.get() : null; // Returns null if the object has been collected
}
}
3. Employ Proper Resource Management
Always ensure that resources such as file handles, database connections, and other external resources are closed properly after use. Use try-with-resources wherever applicable.
public void readData() {
try (BufferedReader br = new BufferedReader(new FileReader("file.txt"))) {
String line;
while ((line = br.readLine()) != null) {
// Process the line...
}
} catch (IOException e) {
e.printStackTrace();
}
}
Best Practices to Prevent Memory Leaks
While fixing existing memory leaks is critical, preventing them from occurring in the first place is even better. Here are some best practices:
- Minimize Static References: Avoid static collections that can grow indefinitely.
- Clear Collections: Keep track of what you store in collections and ensure they are cleared or reused.
- Scope Variables Properly: Limit the scope of variables to what is essential.
- Use Profiling Tools Regularly: Incorporate memory profiling tools to catch leaks early.
- Follow Design Patterns: Patterns like Singleton or Listener should be applied carefully to avoid unintended references.
In Conclusion, Here is What Matters
Memory leaks in Java can lead to significant performance issues and application crashes. By implementing monitoring and analysis strategies, utilizing the right tools, and adhering to best practices, you can effectively spot, fix, and prevent memory leaks in your applications.
For further reading on preventing memory leaks in Java applications, consider exploring Java Performance Tuning to delve deeper into optimizing JVM behavior.
Stay vigilant! Regular monitoring and good programming practices will ensure a robust and efficient Java application.