Common Pitfalls when Composing Java Annotations
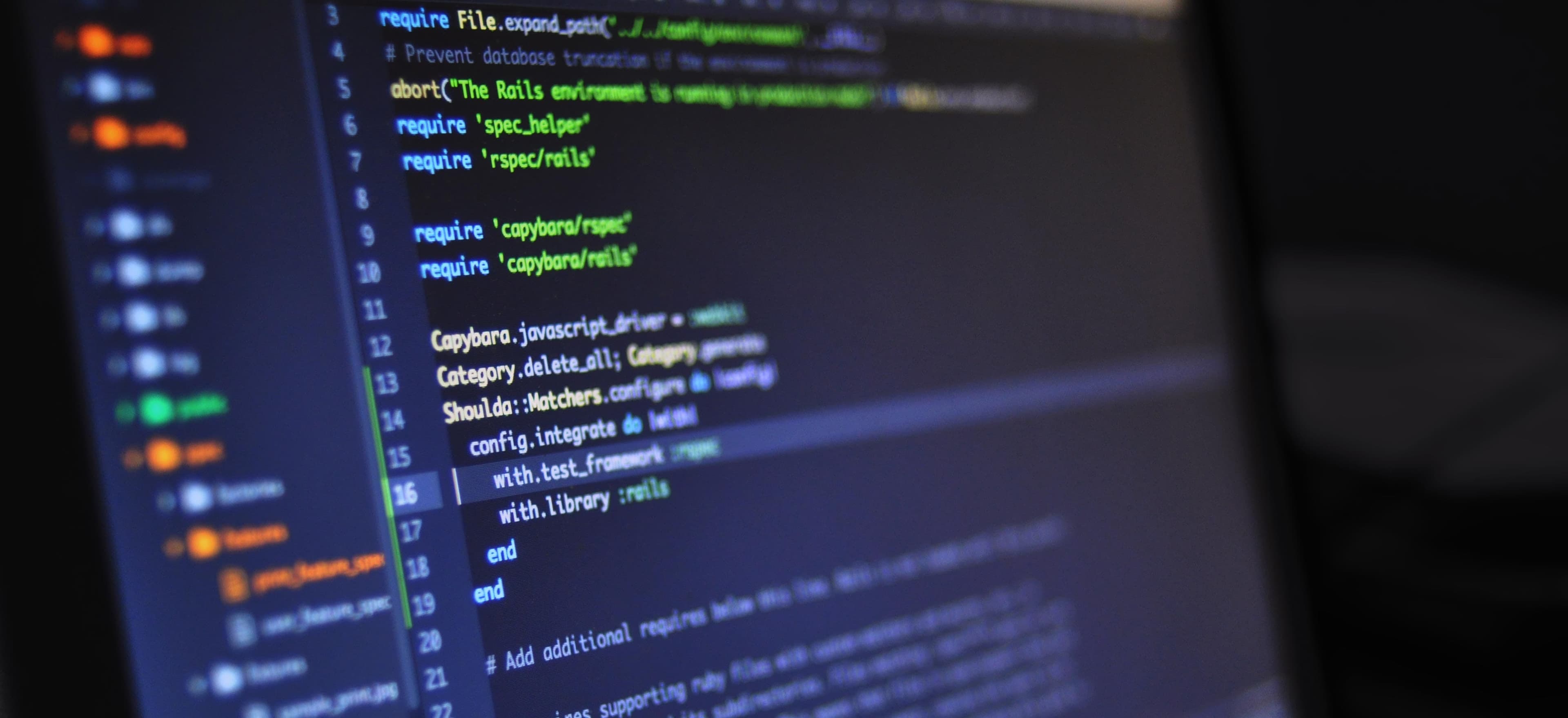
- Published on
Common Pitfalls When Composing Java Annotations
Java annotations are a powerful feature that can add metadata to your code, allowing for more readable, maintainable, and flexible implementations. However, as with any powerful tool, there are common pitfalls that developers encounter when composing these annotations. This post aims to shed light on these pitfalls, aiding you in avoiding them.
What Are Java Annotations?
Java annotations are a form of metadata that provide data about a program but are not part of the program itself. They serve a variety of purposes, from configuration for frameworks (like Spring or Hibernate) to specialized tasks such as documentation generation or even code analysis.
Example annotation syntax:
public @interface MyCustomAnnotation {
String value();
}
This declaration creates an annotation MyCustomAnnotation
that has a single attribute value()
of type String
.
1. Understanding the Annotation Retention Policy
One of the most critical aspects of Java annotations is their retention policy. Annotations can be discarded by the compiler, retained in the class file, or available at runtime. Developers often mistakenly assume that their annotations will be available at runtime when they might not be.
Retention Policies
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
@Retention(RetentionPolicy.RUNTIME) // Available at runtime
public @interface RuntimeAnnotation {
String description();
}
@Retention(RetentionPolicy.SOURCE)
: Discarded by the compiler.@Retention(RetentionPolicy.CLASS)
: Retained in the class file, not available at runtime.@Retention(RetentionPolicy.RUNTIME)
: Available at runtime.
Why This Matters: If you intend to use reflection to read these annotations, setting them to SOURCE
or CLASS
will lead to runtime exceptions when you try to access them.
2. Using Multiple Annotations
Java allows you to create repeatable annotations. However, how you structure these annotations can lead to confusion and complicate your code's readability.
Example of Repeatable Annotations
import java.lang.annotation.ElementType;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Repeatable;
@Repeatable(MyAnnotations.class)
@Retention(RetentionPolicy.RUNTIME)
@Target(ElementType.TYPE)
public @interface MyAnnotation {
String value();
}
@Retention(RetentionPolicy.RUNTIME)
@Target(ElementType.TYPE)
@interface MyAnnotations {
MyAnnotation[] value();
}
Pitfall: Forgetting to specify the enclosing annotation (MyAnnotations
here) can lead to unexpected behavior when you try to retrieve multiple annotations.
Why This Matters: Always ensure that your repeatable annotations are appropriately defined. This saves developers from confusion and keeps the codebase cleaner.
3. Proper Use of Target Annotations
Using the @Target
annotation specifies where an annotation can be applied, such as to methods, classes, or fields. Failing to use this can lead to unintended usage of your annotations.
Example
import java.lang.annotation.ElementType;
import java.lang.annotation.Target;
@Target(ElementType.METHOD) // This annotation can only be applied to methods
public @interface MyMethodAnnotation {
String value();
}
Pitfall: If you omit the @Target
, it defaults to ElementType.TYPE
, leading to possible misuse of your annotation elsewhere in the code.
Why This Matters
Explicitly defining where your annotations can be applied improves code readability and reduces potential errors during compilation or runtime.
4. Annotations vs Interfaces
Sometimes, developers confuse annotations with interfaces. Annotations are not interfaces, and they cannot have method implementations. They are merely collections of attributes.
Example of an Incorrect Usage
public @interface MyInterfaceAnnotation { // Incorrect usage
void doSomething(); // This will cause a compilation error
}
Why This Matters: Recognizing that annotations are not interfaces prevents confusion and compilation errors.
5. Avoiding Annotations That Carry Too Much Responsibility
A common pitfall in designing annotations is giving them too many responsibilities or features. A good annotation should have a single, well-defined purpose.
Overloading an Annotation
public @interface DataValidation {
String message() default "Invalid data!";
boolean isRequired() default false;
String regex() default ""; // Overkill! One purpose, one responsibility
}
Why This Matters: Keeping annotations focused prevents misuse and simplifies the maintenance of your code. Stick to the single responsibility principle.
6. Ignoring the JavaDoc for Annotations
Annotations, like classes, can benefit from good documentation. A common pitfall is neglecting JavaDoc for annotations, making it harder for other developers to understand their use.
Example Annotation with JavaDoc
/**
* This annotation is used to mark methods that require
* data validation before execution.
*/
@Retention(RetentionPolicy.RUNTIME)
public @interface ValidateData {
}
Why This Matters: Good documentation improves the developer experience and helps maintain clarity across your codebase.
7. Serialization Issues with Annotations
When using annotations, you may inadvertently break serialization. serialVersionUID consistency is essential, especially if annotations change over time.
Example of Serialization Pitfall
import java.io.Serializable;
public @interface SerializableAnnotation extends Serializable {
}
Pitfall: If the annotation changes, old serialized objects may cause compatibility issues.
Why This Matters: By understanding serialization, you will avoid problems related to backward compatibility and system architecture.
In Conclusion, Here is What Matters
Java annotations provide a flexible mechanism for adding metadata to our Java programs. However, to make the most out of them, it's essential for developers to avoid common pitfalls such as incorrect retention policies, overloading responsibilities, and overlooking good documentation practices.
By being aware of these issues and implementing best practices, you can ensure that your annotations remain useful and understandable. Additionally, check out the official Java annotation documentation and Effective Java by Joshua Bloch for more insights and tips on annotations in Java.
Remember, as with all features of a programming language, understanding the nuances of annotations will improve the quality of your Java applications. Happy coding!