Troubleshooting Common MapStruct Mapping Issues
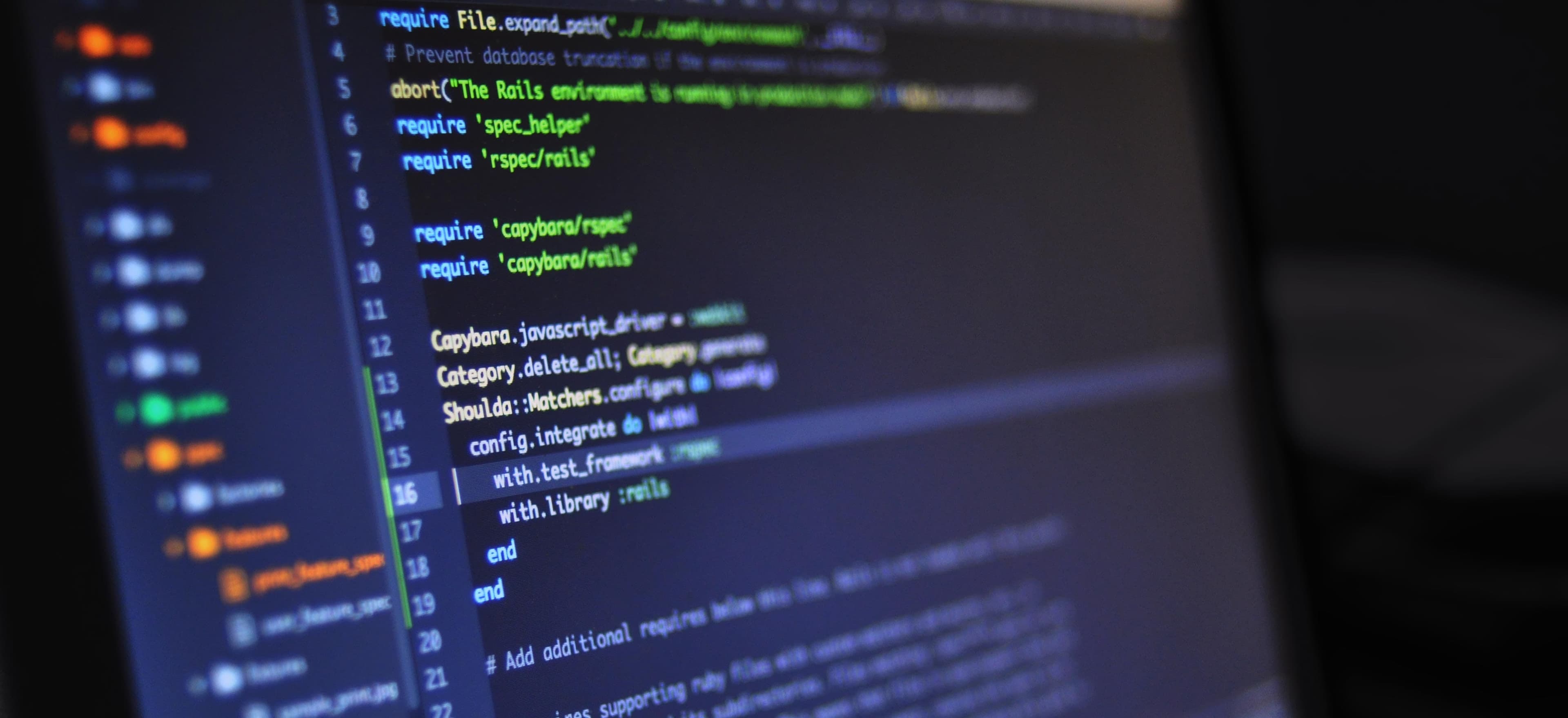
- Published on
Troubleshooting Common MapStruct Mapping Issues
MapStruct is a powerful code generator that simplifies the process of mapping data between Java bean types. It reduces boilerplate code, helps improve performance, and enhances maintainability. Nevertheless, developers often encounter issues while using MapStruct. In this post, we will explore common mapping issues and how to troubleshoot them effectively.
What is MapStruct?
MapStruct is an annotation processor that generates type-safe mappings between Java Beans at compile time. The mappings eliminate the burden of writing tedious conversion code. Its approach is based on using Java annotations to define the mappings between source and target objects.
Before diving into troubleshooting, let’s look at some essential concepts.
Key Concepts
Mappings
Mappings are defined using annotations such as @Mapping
to specify how properties map from source to target.
Mapper Interface
Defining a mapper interface allows MapStruct to generate the implementation at compile time.
Type Conversion
MapStruct handles many primitive and complex types but sometimes requires custom methods for unique conversions.
Setting Up MapStruct
To begin with MapStruct in your project, you must include the following dependencies in your pom.xml
if you are using Maven or the equivalent in Gradle.
<dependency>
<groupId>org.mapstruct</groupId>
<artifactId>mapstruct</artifactId>
<version>1.5.3.Final</version>
</dependency>
<dependency>
<groupId>org.mapstruct</groupId>
<artifactId>mapstruct-processor</artifactId>
<version>1.5.3.Final</version>
<scope>provided</scope>
</dependency>
Basic Example
Let's say we have two Java classes: Person
and PersonDTO
. The PersonDTO
class is used for data transfer operations.
public class Person {
private String name;
private int age;
// Getters and Setters
}
public class PersonDTO {
private String fullName;
private int age;
// Getters and Setters
}
To map between these two:
import org.mapstruct.Mapper;
import org.mapstruct.Mapping;
@Mapper
public interface PersonMapper {
@Mapping(source = "name", target = "fullName")
PersonDTO personToPersonDTO(Person person);
}
Code Explanation
In the code above, we specify that the name
property from the Person
source object should map to the fullName
property in the PersonDTO
target object. This is a simple mapping, but issues can arise with more complex scenarios.
Common Issues and Troubleshooting
Let's explore some common issues developers face and how to troubleshoot them.
1. Mapper Implementation Not Generated
Issue: The most common issue is when the mapper implementation is not generated.
Cause: This usually happens if the annotation processor is not set up correctly.
Solution: Ensure that your IDE recognizes and processes annotations. For Maven, include the maven-compiler-plugin
:
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<version>3.8.1</version>
<configuration>
<source>1.8</source>
<target>1.8</target>
<annotationProcessorPaths>
<path>
<groupId>org.mapstruct</groupId>
<artifactId>mapstruct-processor</artifactId>
<version>1.5.3.Final</version>
</path>
</annotationProcessorPaths>
</configuration>
</plugin>
After modifying, perform a clean build.
2. Mapping Errors for Nested Objects
Issue: If your source or target classes contain nested objects, you may encounter mapping errors.
Cause: By default, MapStruct does not handle nested mappings without explicit instructions.
Solution: You need to define mappings for nested classes as well.
public class Address {
private String city;
private String country;
// Getters and Setters
}
public class PersonDTO {
private String fullName;
private int age;
private AddressDTO address;
// Getters and Setters
}
public class AddressDTO {
private String city;
private String country;
// Getters and Setters
}
@Mapper
public interface PersonMapper {
@Mapping(source = "name", target = "fullName")
@Mapping(source = "address", target = "address")
PersonDTO personToPersonDTO(Person person);
AddressDTO addressToAddressDTO(Address address);
}
In this case, we have provided a mapping for the Address
class as well.
3. Discrepancies in Property Names
Issue: Property names may differ between source and target objects.
Cause: Mismatched property names could lead to mapping failure.
Solution: Use the @Mapping
annotation proactively to define every discrepancy.
@Mapper
public interface PersonMapper {
@Mapping(source = "name", target = "fullName")
@Mapping(source = "age", target = "age")
PersonDTO personToPersonDTO(Person person);
}
For differing names, specify both source
and target
.
4. Null Handling and Default Values
Issue: If you pass null values, the default behavior might cause issues.
Cause: MapStruct may not handle nulls as expected.
Solution: You can customize the null value behavior using @Mapping
with nullValuePropertyMappingStrategy
.
@Mapper(nullValuePropertyMappingStrategy = NullValuePropertyMappingStrategy.SET_TO_NULL)
public interface PersonMapper {
// Mapping methods
}
5. Complex Custom Mappings
Issue: Complex data transformations require more than simple field copying.
Cause: If your source or target requires special transformations, MapStruct may not recognize this.
Solution: You can write custom methods to handle such cases.
@Mapper
public interface PersonMapper {
@Mapping(source = "name", target = "fullName")
@Mapping(source = "age", target = "age")
PersonDTO personToPersonDTO(Person person);
default String mapName(String name) {
return name != null ? name.toUpperCase() : null; // Custom transformation
}
}
In the above code snippet, we transformed the name
before mapping it.
6. Build Time Errors
Issue: Sometimes, MapStruct can cause build time errors that are cryptic.
Cause: These may stem from incompatible types or annotations.
Solution: Check for specific errors in the build log. Ensure you're using compatible versions between MapStruct and its processor.
Closing Remarks
MapStruct is a lightweight yet powerful framework that can simplify the mapping process significantly. However, as we have seen, various issues can arise, particularly around the setup and configuration.
By understanding the common pitfalls and resolutions discussed above, you can leverage MapStruct effectively in your Java projects.
For more information, consider visiting the official MapStruct documentation for guidance on advanced mapping techniques and best practices.
Implementing the right practices will lead you to a smoother development experience and better maintainability for your applications. Happy coding!