Eliminating Common Java Code Smells for Cleaner Code
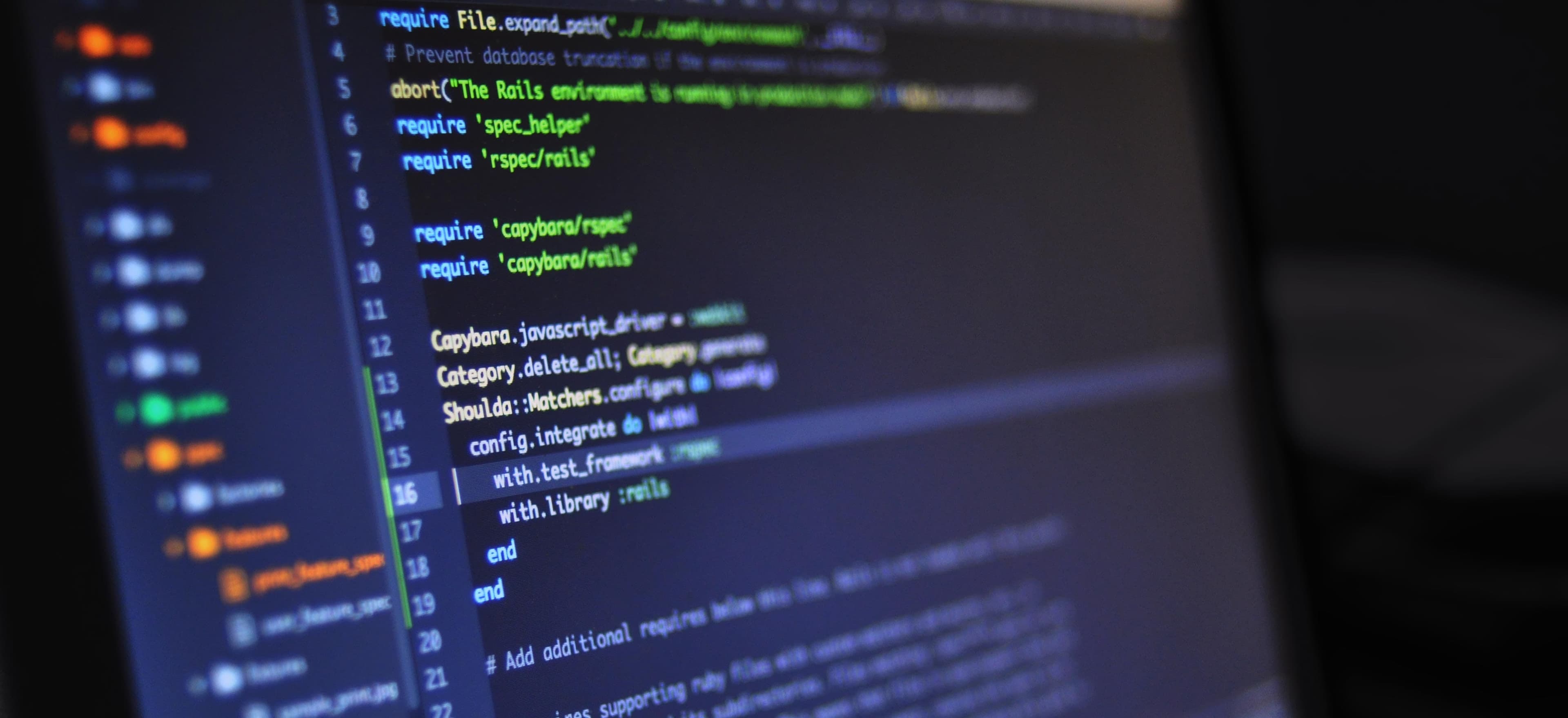
- Published on
Eliminating Common Java Code Smells for Cleaner Code
Java, as a robust object-oriented programming language, offers vast capabilities for building scalable and maintainable software. However, even the most experienced developers can fall into the trap of "Code Smells" — indicators that there might be a deeper problem in your code base. In this blog post, we will explore common Java code smells, their potential pitfalls, and ways to eliminate them for cleaner, more maintainable code.
What Are Code Smells?
Code smells are not bugs but rather indicators of potential issues in your code. The term was coined by Kent Beck and carried forward by Martin Fowler in his book, "Refactoring: Improving the Design of Existing Code". Recognizing and addressing code smells early can save significant time and resources in later stages of development.
Why Pay Attention to Code Smells?
- Maintainability: Code that is clean and free from smells is easier to maintain and update.
- Readability: Code is not just written for the machine; it is also read by humans. Cleaner code is easier to understand.
- Scalability: A well-organized code base accommodates future growth more effectively.
Common Java Code Smells and How to Fix Them
1. Long Methods
Long methods can lead to confusion and reduce readability. They often do too many things at once, violating the Single Responsibility Principle.
Fix: Break long methods into smaller, more cohesive methods. Each method should handle a single task.
public void processOrder(Order order) {
validateOrder(order);
calculateOrderTotal(order);
sendConfirmationEmail(order);
}
private void validateOrder(Order order) {
// Validation logic here
}
private void calculateOrderTotal(Order order) {
// Total calculation logic here
}
private void sendConfirmationEmail(Order order) {
// Email sending logic here
}
In this example, we refactored a lengthy processOrder
method into three distinct methods, enhancing clarity and manageability.
2. Duplicated Code
Duplicated code increases the risk of inconsistent changes and code bloat. It's often referred to as "drifting" code and can make debugging more challenging.
Fix: Extract common functionality into a separate method or class.
public void sendInvoiceEmail(Invoice invoice) {
// Logic for preparing and sending the email
}
public void sendOrderEmail(Order order) {
// Similar logic for sending order emails, but now we extract the common email sending logic
sendInvoiceEmail(order.getInvoice());
}
By creating a single method for sending emails, we eliminate redundancy and consolidate our email-sending logic.
3. Magic Numbers and Strings
Hardcoding values directly in the code can make it challenging to update and understand. These "magic" values can obscure the intent of your code.
Fix: Use constants or enums to make these values clear.
public static final int MAX_CONNECTIONS = 50;
public void addConnection(Connection connection) {
if (currentConnections < MAX_CONNECTIONS) {
// Add connection
}
}
Using constants clarifies what "50" represents, improving readability and maintainability.
4. Unused Parameters
Having parameters that are never used can cause confusion and imply that the method does more than it actually does.
Fix: Remove any unused parameters to simplify the method signature.
public void processPayment(Payment payment) {
// Only payment is used
}
If you have any parameters not used in the method, eliminate them to reduce complexity.
5. Large Classes
A class that tries to do too much can become unwieldy and challenging to maintain.
Fix: Apply the Single Responsibility Principle by splitting large classes into smaller classes, each responsible for a specific task.
public class Order {
// Order-related properties and methods
}
public class Invoice {
// Invoice-related properties and methods
}
Now, the Order
and Invoice
classes each have their clear responsibilities, making the code base cleaner and better organized.
6. Switch Statements
While switch statements can be useful, they often lead to brittle code. Adding new cases can require modifying existing code, increasing the risk of bugs.
Fix: Use polymorphism to handle different behaviors.
public interface OrderType {
void process();
}
public class OnlineOrder implements OrderType {
public void process() {
// Online order processing logic
}
}
public class InStoreOrder implements OrderType {
public void process() {
// In-store order processing logic
}
}
// Usage
OrderType order = new OnlineOrder();
order.process();
This approach not only eliminates the switch statement but also follows the Open/Closed Principle, allowing new functionality to be added without modifying existing code.
7. Overuse of Comments
Comments can often signal that the code is not adequately self-explanatory. If you find yourself writing lengthy comments to explain complex operations, it’s time to rethink your code structure.
Fix: Aim for self-documenting code through meaningful variable names and method signatures.
// Bad
int d = 20; // Diameter
// Good
int diameter = 20;
In the second example, the variable name hints at its purpose, reducing the need for comments.
Wrapping Up
Eliminating common code smells is crucial for maintaining a clean, efficient, and scalable code base. By focusing on principles like DRY (Don't Repeat Yourself), SRP (Single Responsibility Principle), and others, developers can create a robust and manageable code that stands the test of time.
For further reading, you may visit Martin Fowler’s Refactoring for a deeper understanding of the refactoring process and Clean Code by Robert C. Martin for additional insights into writing cleaner code.
As you strive to improve your Java code, remember that the goal is not just to write less code but to write code that is easier to read, maintain, and scale. Happy coding!