Solving Concourse Caching Issues in Java Builds
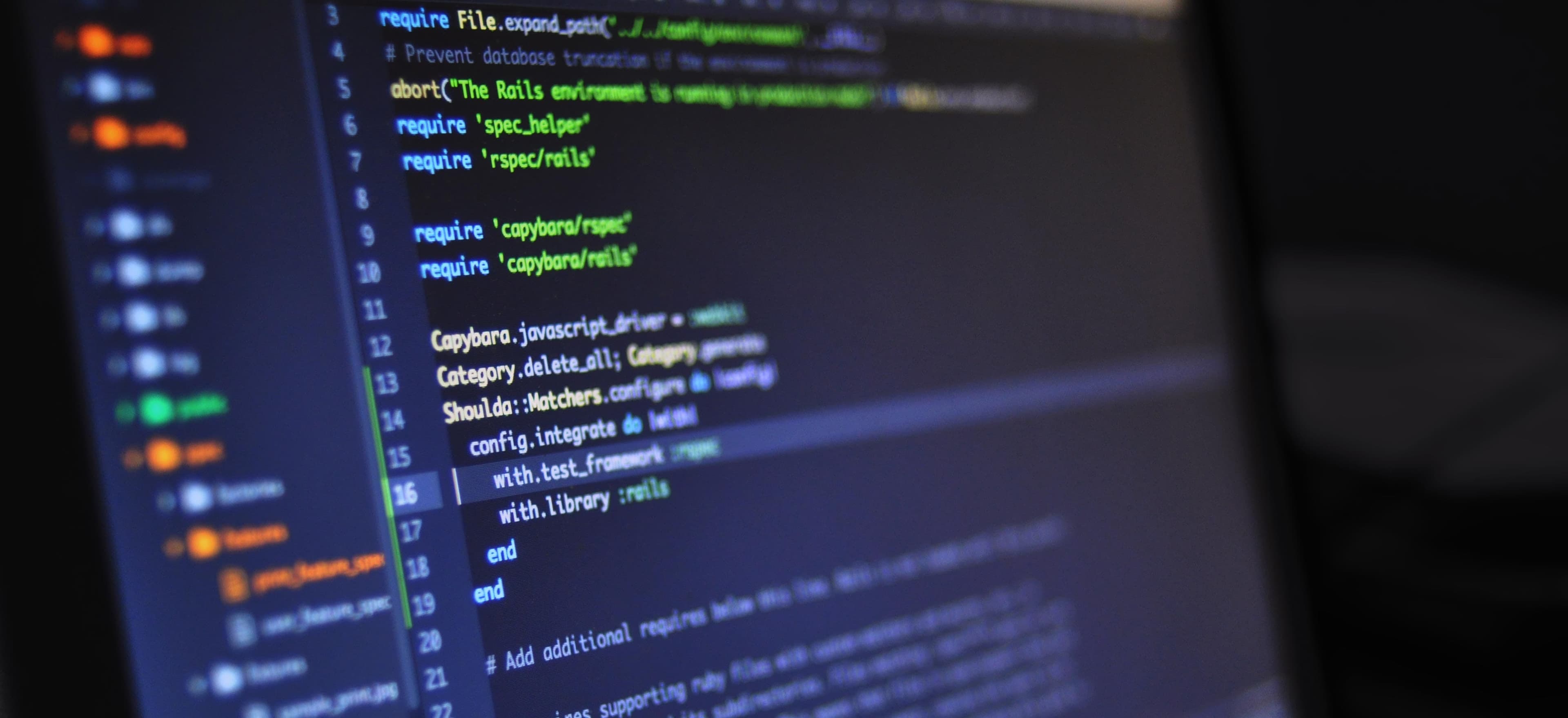
- Published on
Solving Concourse Caching Issues in Java Builds
In the world of Continuous Integration and Continuous Deployment (CI/CD), caching is an integral aspect that can substantially speed up your build process. Concourse CI, known for its simplicity and efficiency, relies heavily on caching to improve build times. However, navigating caching issues can be a daunting task for developers, especially in Java builds.
This blog post will delve into the common caching issues encountered in Concourse CI when building Java applications. We will discuss strategies for addressing those problems effectively, while providing clear examples and code snippets to ensure a smooth execution of your builds.
Understanding Concourse and Caching
Concourse CI operates on a model of pipelines, jobs, and resources. Caching in Concourse can significantly enhance build times by storing tools, libraries, and dependencies. When used correctly, it allows subsequent builds to leverage pre-fetched resources instead of redownloading them. Java builds often require extensive libraries, making caching particularly important.
Why Caching is Crucial in Java Projects
Java projects rely on several dependencies which are specified in build configurations, such as Maven's pom.xml
or Gradle's build.gradle
. Without effective caching strategies, every build could entail downloading these dependencies fresh, leading to an increase in build times and consuming bandwidth unnecessarily.
Let's explore how you can optimize caching in Concourse CI for your Java applications.
Common Caching Issues in Java Builds
1. Ineffective Dependency Caching
One common issue arises when dependencies are not cached properly. This can happen due to misconfiguration in your pipeline.yml
files or tags causing unnecessary cache invalidations.
Example
resources:
- name: maven-repo
type: s3
source:
bucket: my-bucket
access_key_id: ((aws_access_key_id))
secret_access_key: ((aws_secret_access_key))
In the above example, you're using S3 as a caching mechanism for Maven dependencies. However, if the paths or versioning are incorrectly specified, builds will fail to retrieve cached dependencies.
Solution
Ensure that your versioning strategy is consistent. Stick to fixed versions in your configuration files, and update the cache keys carefully to reflect only necessary changes.
2. Inconsistent Build Environments
Another caching issue crops up when different workers are used for builds, leading to variability in the build environment. This can cause cached dependencies to be incompatible with the build requirements.
Example
Using different JDK versions across workers can lead to conflicts:
plan:
- get: maven-repo
params: {version: "my-custom-version"}
- task: build
image: openjdk:8-jdk
run:
path: mvn
args: ["clean", "install"]
In this scenario, switching to a different worker with a different JDK version may result in build failings.
Solution
Use the same Docker image for all your workers wherever possible. Define your images in a consistent manner to alleviate any discrepancies between builds.
3. Cache Invalidation Issues
Cache invalidation is tricky. If resources change frequently without proper versioning, it can lead to cache being obsolete, resulting in failed builds.
Implementation
A proper naming convention for your cache resource can help manage cache invalidation:
resources:
- name: build-cache
type: s3
source:
bucket: my-cache-bucket
access_key_id: ((aws_access_key_id))
secret_access_key: ((aws_secret_access_key))
cache_key: "java-build-cache-v1"
By having a cache_key, you control when to invalidate a cache by updating the ‘v1’ part of the cache_key
.
4. Manual Cache Management
It can be effectively time-consuming when having to manage caches manually. This often leads to forgotten deletions of obsolete caches, taking unnecessary space.
Solution: Automated Garbage Collection
You can automate caching control using a cleanup script that runs at specified intervals to remove old caches:
#!/bin/bash
CACHE_DIR="/path/to/cache"
# Clean up cached files older than 30 days
find $CACHE_DIR -type f -mtime +30 -exec rm -f {} \;
Integrating such scripts into your pipeline jobs can significantly improve cache management.
5. Lack of Documentation
Documentation regarding your caching strategy is essential. Without it, your team may struggle down the line when dealing with caching-related issues.
Solution: Centralized Documentation
Maintain a centralized README file that outlines your caching protocols, dependencies, and configurations. This practice will keep your team on the same page.
Strategies to Improve Caching in Concourse CI
1. Leverage Resource Caching
Utilize Concourse build resources to cache build outputs, making them available for future builds. This reduces the need to rebuild every dependency.
2. Optimize Local Maven Repository Caching
Take advantage of the local .m2
directory for caching Maven dependencies:
plan:
- get: maven-repo
params: {path: ".m2"}
Configuring your pipeline to cache the local Maven repository can save significant time.
3. Use Containerized Builds
By using containers, you can encapsulate your build environment, ensuring consistency across builds and allowing caching mechanisms to work seamlessly.
4. Use Code Caching Strategies
Implement Gradle’s buildCache
or Maven's caching features to minimize rebuilds for Java applications.
Example of Gradle caching
Gradle can store build outputs in a cache directory configured in your settings.gradle
:
buildCache {
local {
enabled = true
}
}
Enabling local build cache allows Gradle to cache outputs on disk for future builds.
Final Thoughts
Caching is a critical component of optimizing Java builds within Concourse CI. By addressing issues like ineffective dependency caching, inconsistent environments, cache invalidation, and manual management, you can significantly enhance your build process. Solid practices, such as proper resource management and consistent documentation, lay the groundwork for a streamlined CI/CD pipeline.
For further reading on caching strategies in CI/CD, check out the official Concourse CI documentation or explore Maven's official site for more on dependency management.
With the right approach, you can ensure your Java builds run smoothly, saving both time and resources. Happy coding!