Common JPA Schema Generation Errors and How to Fix Them
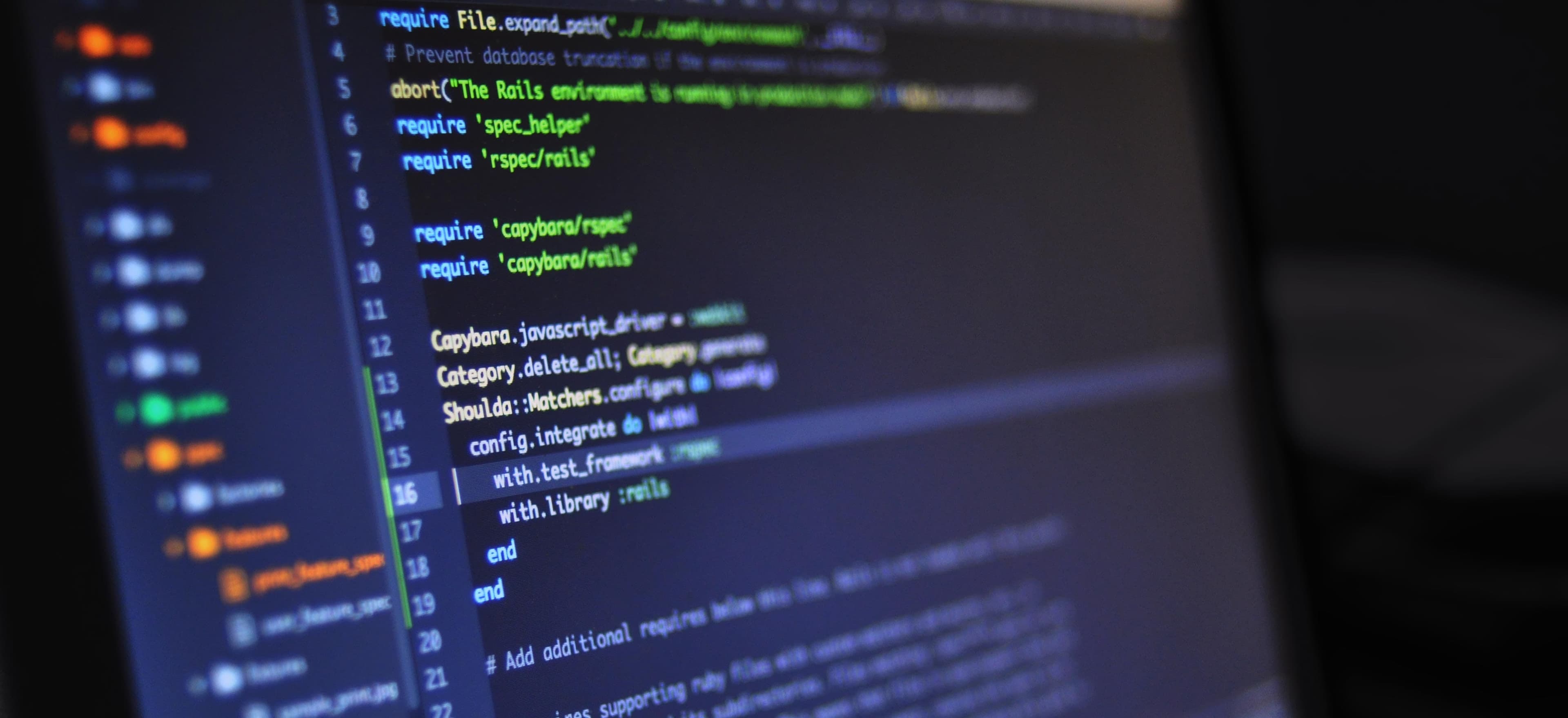
- Published on
Common JPA Schema Generation Errors and How to Fix Them
Java Persistence API (JPA) simplifies data access in Java applications that need to interact with relational databases. However, developers often encounter schema generation errors that can impede application development. This blog post addresses common JPA schema generation errors and provides solutions to fix them.
What is JPA Schema Generation?
Before delving into the errors, let's define what schema generation entails. JPA can automatically generate database schemas from entity classes during application startup. This feature is extremely useful for rapid development and testing. However, configuration issues and coding mistakes can lead to frustrating errors.
Common Errors and Their Solutions
1. Unable to locate the persistence provider
Error Description: This error arises when the application cannot find a valid persistence.xml
file or when it is improperly configured.
Solution: Ensure you have the persistence.xml
file located in the src/main/resources/META-INF/
directory. Here's how this file should look:
<persistence xmlns="http://xmlns.jcp.org/xml/ns/persistence"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/persistence
http://xmlns.jcp.org/xml/ns/persistence/persistence_2_1.xsd"
version="2.1">
<persistence-unit name="myPersistenceUnit">
<provider>org.hibernate.jpa.HibernatePersistenceProvider</provider>
<class>com.example.EntityClass</class>
<properties>
<property name="javax.persistence.jdbc.url" value="jdbc:h2:mem:testdb"/>
<property name="javax.persistence.jdbc.user" value="sa"/>
<property name="javax.persistence.jdbc.password" value=""/>
<property name="javax.persistence.jdbc.driver" value="org.h2.Driver"/>
<property name="hibernate.hbm2ddl.auto" value="update"/>
</properties>
</persistence-unit>
</persistence>
Make sure to adjust the class
entry to reference your actual entity classes.
2. Table already exists
Error Description: If you are using hibernate.hbm2ddl.auto
set to create
or create-drop
, you may encounter this error when trying to create a schema that already exists.
Solution: Change the hbm2ddl.auto
property to update
. This will alter the database schema to reflect your entity definitions without deleting existing data.
<property name="hibernate.hbm2ddl.auto" value="update"/>
For production environments, managing schemas through migration tools like Flyway or Liquibase is recommended.
3. Column [NAME] not found
Error Description: This error usually indicates that the entity is expected to map to a non-existent column in the database schema, which could be due to mismatched entity definitions or database schema design.
Solution: Check your entity class for mismatches between field names and column names. Use the @Column
annotation to specify the correct mapping, as shown below:
@Entity
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Column(name = "username") // Explicit column name
private String userName;
// getters and setters
}
4. No identifier specified for entity
Error Description: Every entity in JPA must have a primary key, and this error occurs when there's no identifier defined.
Solution: Make sure that your entity class has an @Id
annotation on one field. Here’s an example:
@Entity
public class Product {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long productId;
private String name;
// getters and setters
}
5. Invalid entity name
Error Description: This happens if the entity name specified in the @Entity
annotation conflicts with the database table name or is not found at all.
Solution: Make sure the names are consistent. Additionally, you can explicitly define the table name in the @Table
annotation if it differs from the entity name:
@Entity
@Table(name = "products_table")
public class Product {
...
}
6. The database schema creation failed
Error Description: This generic error can arise from various causes such as JDBC configuration issues, constraints violations, or network connectivity problems.
Solution: Review the stacktrace for more details regarding the cause. Common fixes include checking your JDBC URL for correctness, ensuring the database service is running, and verifying permissions.
<property name="javax.persistence.jdbc.url" value="jdbc:mysql://localhost:3306/mydatabase"/>
Debugging Tips
When encountering these issues, try these debugging strategies:
-
Review Logs: Always check your application logs. Most JPA providers, like Hibernate, provide detailed information about errors.
-
Enable SQL Logging: Configure your JPA provider to display SQL statements including schema generation statements. This can provide insight into what's happening behind the scenes.
<property name="hibernate.show_sql" value="true"/>
<property name="hibernate.format_sql" value="true"/>
- Check Database State: If errors persist, manually inspect the database state using a database management tool to ensure that it reflects the expected schema.
Lessons Learned
Working with JPA and schema generation can be overwhelming when errors arise, especially during the early stages of application development. This guide provides insight into common schema generation issues and how to remedy them.
For effective schema management, consider using tools like Hibernate for ORM and schema generation alongside database migration tools like Flyway or Liquibase to handle scenarios in production environments.
By understanding and applying these solutions, you can streamline your development process and minimize frustrations when working with JPA. Happy coding!