Mastering File Operations in Java 8: A Lambda Guide
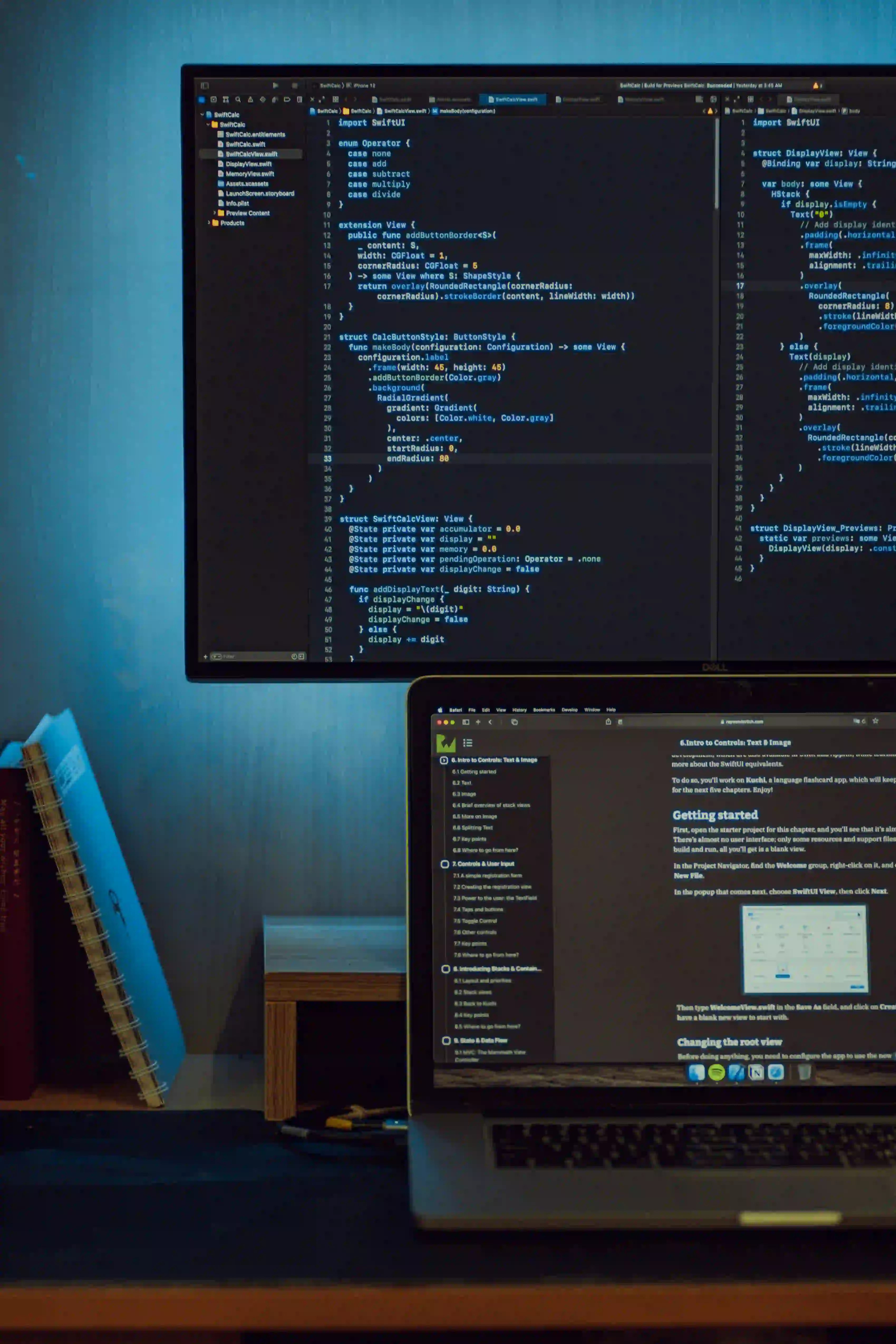
Mastering File Operations in Java 8: A Lambda Guide
Java 8 introduced several powerful features, most notably the Stream API and Lambda expressions. These innovations vastly changed how developers handle collections and file operations. This guide will focus on mastering file operations using Java 8's new capabilities, particularly Lambda expressions and Streams.
The Starting Line to File I/O in Java
Before diving into the core of our topic, let’s establish a basic understanding of file I/O (input/output) in Java. The traditional way to handle files involved using classes from the java.io
package, often requiring multiple lines of boilerplate code to read or write data. With the introduction of the java.nio.file
package, file operations became more efficient and expressive.
Why Use Java 8 File Operations?
- Conciseness: Lambda expressions reduce boilerplate code, making it easier to read and write.
- Stream API: It allows for functional-style operations on collections of data, and it can be applied to files.
- Better Performance: Efficient processing of large datasets.
With this groundwork laid, let's start exploring file operations in Java 8!
Reading Files Using Streams and Lambdas
The Files
class in java.nio.file
provides static methods to read from files. One effective way to read a file line by line and apply operations to each line is by using Streams.
Example: Reading a File Line by Line
Here’s how you can read a file line by line using Streams:
import java.nio.file.*;
import java.io.IOException;
import java.util.stream.Stream;
public class FileReaderExample {
public static void main(String[] args) {
String fileName = "example.txt"; // Specify your file name here
try (Stream<String> lines = Files.lines(Paths.get(fileName))) {
lines.filter(line -> !line.isEmpty()) // Filter out empty lines
.map(String::toUpperCase) // Convert lines to uppercase
.forEach(System.out::println); // Print each line
} catch (IOException e) {
e.printStackTrace();
}
}
}
Code Explanation:
- Paths.get(fileName): This method retrieves the path of the file you want to read.
- Files.lines(...): This creates a Stream of lines for the specified file.
- filter(): Filters out any empty lines, ensuring we only work with meaningful content.
- map(String::toUpperCase): Transforms each line to uppercase.
- forEach(System.out::println): Finally, prints each processed line.
This code succinctly demonstrates how Java 8's features can reduce complexity while engaging in file I/O.
Writing to Files with Java 8
In addition to reading files, Java 8 provides ways to write to files efficiently using the Files.write()
method. This method accepts a path, a list of strings (or bytes), and additional options.
Example: Writing to a File
Let’s see how to write data to a file:
import java.nio.file.*;
import java.io.IOException;
import java.util.Arrays;
import java.util.List;
public class FileWriteExample {
public static void main(String[] args) {
String fileName = "output.txt"; // Specify your output file
List<String> lines = Arrays.asList("Hello World", "Java 8 File Operations", "Lambda Expressions");
try {
Files.write(Paths.get(fileName), lines);
System.out.println("File written successfully!");
} catch (IOException e) {
e.printStackTrace();
}
}
}
Code Explanation:
- Arrays.asList(...): Creates a List of strings that we want to write to the file.
- Files.write(...): Takes care of writing the list to the specified file path.
- Handling Exceptions: It’s crucial to handle IOExceptions which can occur during file operations.
Using this method, you can quickly save a collection of strings to a file, all in a few lines of code, thanks to Java 8 capabilities.
Modifying Files with Streams
Beyond simply reading or writing files, you can also modify existing files by using Java 8's Streams.
Example: Appending Lines to a File
While Files.write()
creates or overwrites files, you can append data using the StandardOpenOption.APPEND
option.
import java.nio.file.*;
import java.io.IOException;
import java.util.List;
import java.util.Arrays;
public class FileAppendExample {
public static void main(String[] args) {
String fileName = "output.txt"; // The existing file to append to
List<String> newLines = Arrays.asList("New Line 1", "New Line 2");
try {
Files.write(Paths.get(fileName), newLines, StandardOpenOption.APPEND);
System.out.println("Lines appended successfully!");
} catch (IOException e) {
e.printStackTrace();
}
}
}
Code Explanation:
On top of the previous code, the key addition here is:
- StandardOpenOption.APPEND: This option ensures that new data is added at the end of the file rather than overwriting it.
This approach demonstrates how you can efficiently manage and modify your file content with minimal code.
Deleting Files with Java 8
In addition to reading, writing, and modifying files, you may often find yourself needing to delete files. Java 8 makes this straightforward as well.
Example: Deleting a File
To remove a file, you can use the Files.delete()
method.
import java.nio.file.*;
import java.io.IOException;
public class FileDeleteExample {
public static void main(String[] args) {
String fileName = "output.txt"; // Specify the file you want to delete
try {
Files.delete(Paths.get(fileName));
System.out.println("File deleted successfully!");
} catch (IOException e) {
System.out.println("An error occurred: " + e.getMessage());
}
}
}
Code Explanation:
- Files.delete(...): Takes the path of the file to delete. If the file does not exist, an exception is thrown.
- Exception Handling: It's essential to implement exception handling to manage scenarios when the specified file does not exist.
This snippet showcases the simplicity and efficiency of file deletion in Java 8.
Bringing It All Together
Java 8 revolutionized file operations with its Stream API and Lambda expressions. By leveraging these features, developers can write clean, concise, and efficient file-handling code. Whether you're reading, writing, appending, or deleting, mastering these techniques will enhance your Java programming skills.
Additional Resources:
- Java NIO for New I/O: Oracle's official tutorial on file I/O.
- Java 8 Streams Documentation: Explore more about Stream operations.
In summary, embrace the capabilities of Java 8 to enhance your file handling tasks and enjoy a smoother programming experience. Happy coding!