Navigating the Pitfalls of Java Dependency Injection
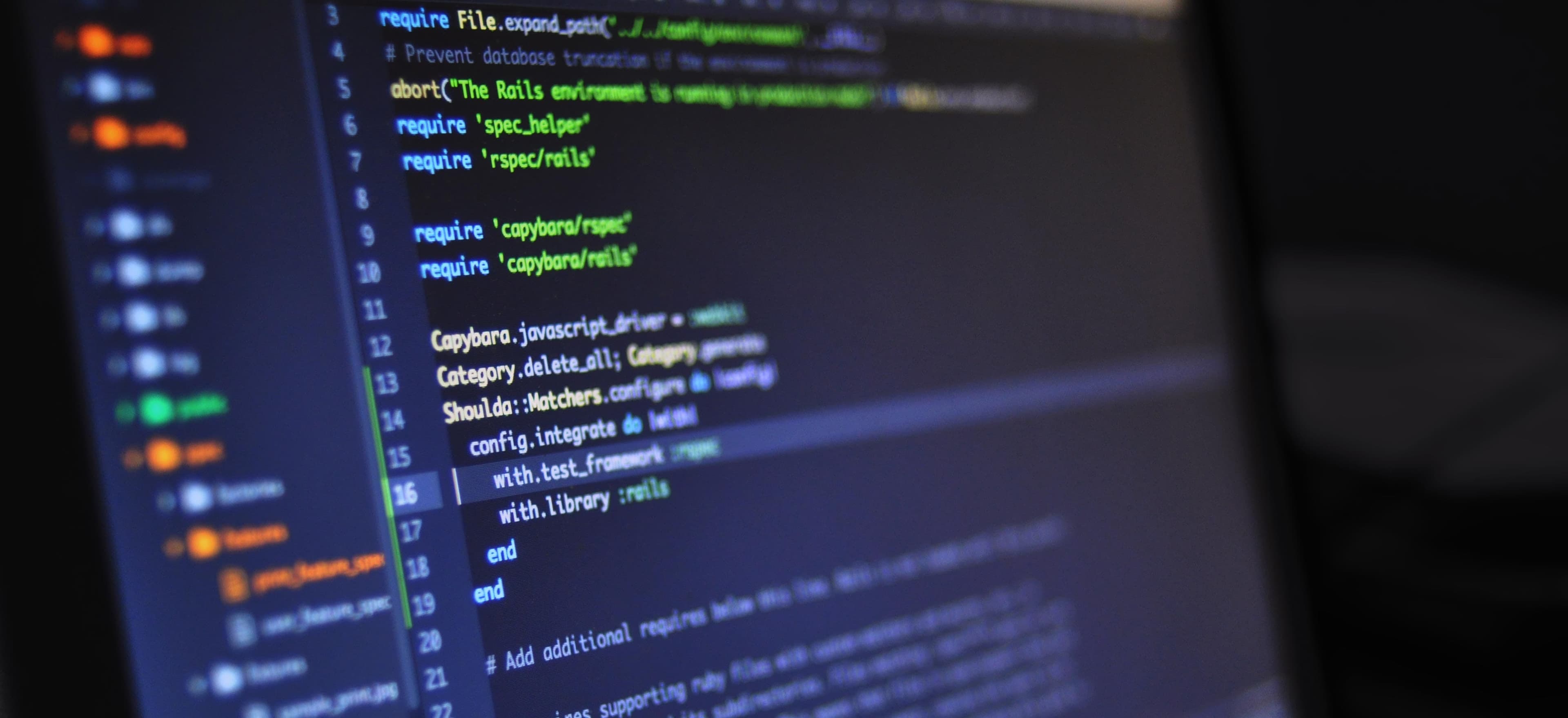
- Published on
Navigating the Pitfalls of Java Dependency Injection
Dependency Injection (DI) is a fundamental principle in object-oriented programming that promotes loose coupling, making your application more modular, testable, and maintainable. Despite its advantages, if not implemented correctly, DI can lead to problems that can hamper productivity and increase complexity. In this blog post, we'll explore the pitfalls of Java Dependency Injection, how to navigate them, and best practices to ensure you harness the true power of DI in your Java applications.
What is Dependency Injection?
Before diving into the pitfalls of DI, it's essential to understand the concept itself. Dependency Injection is a design pattern that involves passing dependencies (objects or services) to a class rather than the class creating them directly. This approach allows for better separation of concerns, easier testing, and improved code reusability.
Here's a basic example in Java:
public interface Service {
void execute();
}
public class RealService implements Service {
public void execute() {
System.out.println("Executing real service.");
}
}
public class Client {
private Service service;
// Dependency injection through the constructor
public Client(Service service) {
this.service = service;
}
public void performAction() {
service.execute();
}
}
// Usage
public class Main {
public static void main(String[] args) {
Service service = new RealService();
Client client = new Client(service);
client.performAction(); // Outputs: Executing real service.
}
}
In this example, Client
does not instantiate Service
directly; instead, it receives it as a parameter in the constructor. This approach enhances flexibility and enables easy swapping of implementations.
Common Pitfalls of Dependency Injection
1. Increased Complexity
One of the most significant drawbacks when using DI is the added complexity it can introduce. New developers might find DI frameworks (like Spring or Guice) overwhelming.
Solution: Educate your team on the basics of the DI principle before introducing complex frameworks. Start with simple constructor-based injection before gradually moving to advanced DI tools.
2. Overuse of DI Frameworks
Using a DI framework can sometimes lead to over-engineering. It's easy to inject far too many dependencies, leading to bloated classes and making the codebase harder to follow.
Solution: Apply the Single Responsibility Principle. Only inject what is necessary for a class's primary purpose.
Example of over-injection:
public class OverInjectedClient {
private Service service1;
private Service service2;
private Service service3;
// Constructor overload
public OverInjectedClient(Service service1, Service service2, Service service3) {
this.service1 = service1;
this.service2 = service2;
this.service3 = service3;
}
}
Here, OverInjectedClient
is complicated unnecessarily. Instead, consider using composition or grouping related services together.
3. Lifecycle Management
DI frameworks often manage the lifecycle of injected dependencies. A misunderstanding of how scopes and lifecycles work can lead to issues like memory leaks or unintentional singleton behavior.
Solution: Familiarize yourself with the lifecycles of your services in your chosen DI framework. For instance, in Spring, services can be defined as singleton, prototype, request, etc.
Example of defining a singleton bean in Spring:
@Configuration
public class AppConfig {
@Bean
public Service myService() {
return new RealService();
}
}
4. Testing Challenges
While DI generally facilitates testing, misuse can lead to difficulties. For example, if external dependencies are injected during tests instead of mocks, it can lead to slow and flaky tests.
Solution: Use mocking frameworks like Mockito to insert mocks of dependencies when running your tests.
Example of unit testing with Mockito:
import static org.mockito.Mockito.*;
public class ClientTest {
@Test
public void testPerformAction() {
Service mockService = mock(Service.class);
Client client = new Client(mockService);
client.performAction();
verify(mockService).execute(); // Verify if the service's execute method is called
}
}
5. Framework Dependency
Often, applications become tightly coupled to a specific DI framework. This can lead to challenges if you want to migrate to another framework or revert to traditional instantiation methods.
Solution: Favor interfaces and abstract classes for your services instead of concrete implementations tied to a specific framework. This practice enhances flexibility.
Best Practices for Java Dependency Injection
1. Favor Constructor Injection
Constructor injection is generally preferred as it makes the dependencies explicit and easier to manage.
public class Client {
private final Service service;
// Constructor injection
public Client(Service service) {
this.service = service;
}
}
2. Limit Injected Dependencies
As mentioned previously, adhere to the Single Responsibility Principle. Try to limit the number of injected dependencies to enhance readability and maintainability.
3. Use DI Framework Wisely
Leverage DI frameworks to manage configuration and lifecycle, but ensure you are not overly dependent on them. Decide what makes sense for your application and avoid unnecessary complexity.
4. Document Your Code
Use JavaDoc to document classes, their dependencies, and the rationale for their design. This documentation aids developers in understanding the interactions between components.
5. Continuously Review and Refactor
Regularly review your code to ensure that it adheres to DI best practices. Refactoring should be a continuous part of your development cycle.
A Final Look
Java Dependency Injection is a powerful tool for building maintainable and testable applications. However, as with any powerful tool, it comes with potential pitfalls that can lead to increased complexity and confusion if not handled correctly.
By understanding the common pitfalls, applying best practices, and continuously educating your team, you can successfully navigate the challenges of Dependency Injection and develop high-quality Java applications.
For further resources, check out Spring Framework Documentation and Guice User Guide. Happy coding!