Overcoming Cold Start Issues in Azure Functions
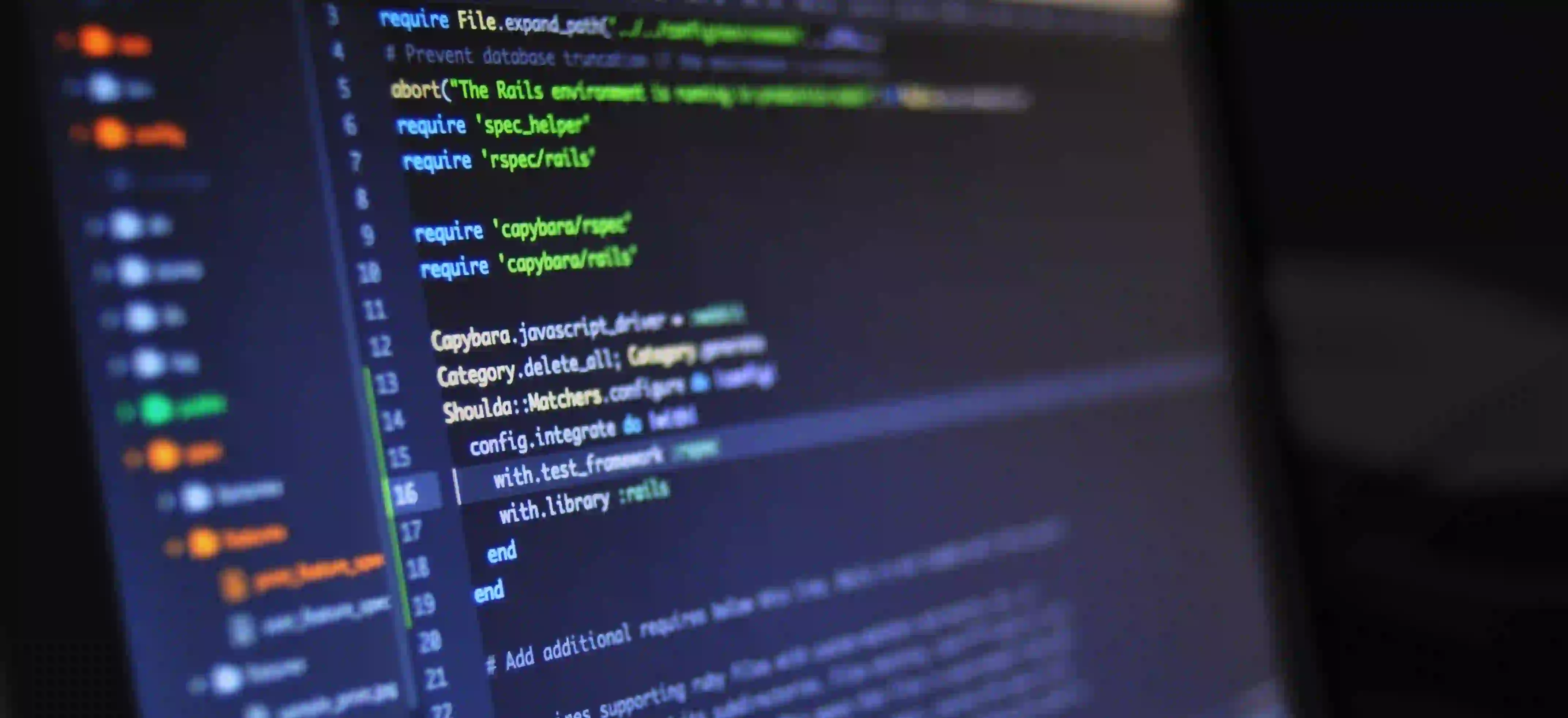
Overcoming Cold Start Issues in Azure Functions
As applications increasingly migrate to serverless architectures, Azure Functions has emerged as a popular platform for building event-driven applications. One of the key challenges that developers face in Azure Functions is the "cold start" problem. In this post, we will explore what cold start is, why it matters, and how you can mitigate its effects to ensure a more responsive application.
What is Cold Start?
Cold start occurs when an Azure Function is triggered after a period of inactivity. The Azure platform needs to allocate resources, load the functions runtime, and initialize your function code. This process introduces latency, resulting in longer response times, which can be detrimental for time-sensitive applications.
When a function experiences a cold start, you may notice delays of several seconds (or more) before execution begins. This delay can negatively impact user experience, especially in applications that require high responsiveness.
!Cold Start vs Warm Start
Why Cold Start Matters
- User Experience: For consumer-facing applications, delays can frustrate users, leading to reduced engagement or churn.
- Performance Metrics: Cold starts can skew performance metrics, making it harder to gauge the true efficiency of your application.
- Cost Implications: Understandably, higher latency can lead to more resources consumed, which may increase costs.
Understanding the Lifecycles
Azure Functions can operate in multiple hosting plans: Consumption plan, Premium plan, and App Service plan. Each of these plans handles cold starts differently:
- Consumption Plan: Functions scale automatically and may experience cold starts when idle.
- Premium Plan: Supports pre-warmed instances, providing better response times but at a higher cost.
- App Service Plan: Functions run continuously, keeping them warm but at a fixed cost regardless of usage.
Understanding these hosting options is crucial when architecting your serverless applications.
Mitigation Strategies
Here are several proven strategies to help you overcome cold start issues in Azure Functions:
1. Optimize Function Code
Optimize your function code to reduce the amount of time it takes to initialize. This can include minimizing package dependencies, which often add overhead during the bootstrapping phase.
Code Example: Minimizing Dependencies
using System.Collections.Generic;
public static class Function
{
[FunctionName("MyFunction")]
public static void Run([QueueTrigger("my-queue")] string queueMessage, ILogger log)
{
// Do the work here
// Keep code lean
var processedData = ProcessData(queueMessage);
log.LogInformation($"Processed data: {processedData}");
}
private static string ProcessData(string message)
{
// Simulating data processing
return message.ToUpper();
}
}
Why? In this example, the code is intentionally kept minimal, reducing the overhead involved with loading external libraries. Keeping initialization lightweight can expedite cold starts.
2. Choose the Right Hosting Plan
As mentioned, moving to a Premium Plan or an App Service Plan can significantly reduce the frequency of cold starts.
- Premium Plan provides options for pre-warmed instances. This means Azure keeps a specified instance of your function warm and ready to handle requests.
- App Service Plan runs continuously and prevents cold starts altogether but may incur a higher cost.
3. Use Durable Functions
Durable Functions allows you to write stateful functions in a serverless compute environment. Although they introduce some overhead, they can help manage workflows efficiently, which can mitigate cold start latency over time.
Code Example: Using Durable Functions
[FunctionName("OrchestratorFunction")]
public static async Task RunOrchestrator(
[OrchestrationTrigger] IDurableOrchestrationContext context)
{
var result = await context.CallActivityAsync<string>("ActivityFunction", null);
}
[FunctionName("ActivityFunction")]
public static string SayHello([ActivityTrigger] string name)
{
return "Hello, " + name + "!";
}
Why? Durable Functions allow you to manage complex processes and keep connections and states without frequently triggering cold starts for simpler execution paths.
4. Keep Functions Warm with Ping Requests
Implementing a warm-up routine using ping requests can be helpful to avoid cold starts. Be aware that this might result in unnecessary executions, which could lead to increased costs.
Code Example: Warm-Up Function
public static class WarmUpFunction
{
[FunctionName("WarmUp")]
public static async Task Run([TimerTrigger("0 */5 * * * *")] TimerInfo myTimer, ILogger log)
{
log.LogInformation("Warm-up function executed.");
// Dummy processing to keep the function "hot"
await Task.Delay(10);
}
}
Why? Scheduling periodic execution of a function ensures that the Azure Function remains "warm," reducing the chance of hitting cold starts.
5. Optimize Application Insights
Using Application Insights can help identify bottlenecks within your Azure Functions. Pinpointing what takes up most of the time during initialization can guide your optimization efforts.
- Look into "Function Execution Time" and "Cold Start Total Time" metrics.
- Review performance recommendations from Azure to further enhance your functions.
Measuring Impact
Once you've implemented strategies, it’s crucial to measure the impact of the changes you made. This can help validate your decisions and guide future optimizations.
- Benchmarking: Run your functions numerous times to track their response times under different conditions.
- Monitoring: Use Azure's monitoring tools to observe metrics over time, adjusting as necessary.
Final Considerations
Cold starts in Azure Functions can significantly impact the user experience and performance metrics of your application. However, with the right strategies in place—optimizing your function code, choosing the appropriate hosting plan, using Durable Functions, employing warm-up techniques, and leveraging Application Insights—you can effectively mitigate these issues.
For more resources on Azure Functions and serverless architecture, check out the official Microsoft Azure Documentation and consider exploring community forums to share experiences and strategies.
By tackling the cold start problem proactively, you not only improve the performance of your functions but also enhance the overall user experience of your applications. So, go ahead and implement these strategies to keep your functions warm and responsive. Happy coding!