Overcoming Spring Boot Retry Limitations for Transient Errors
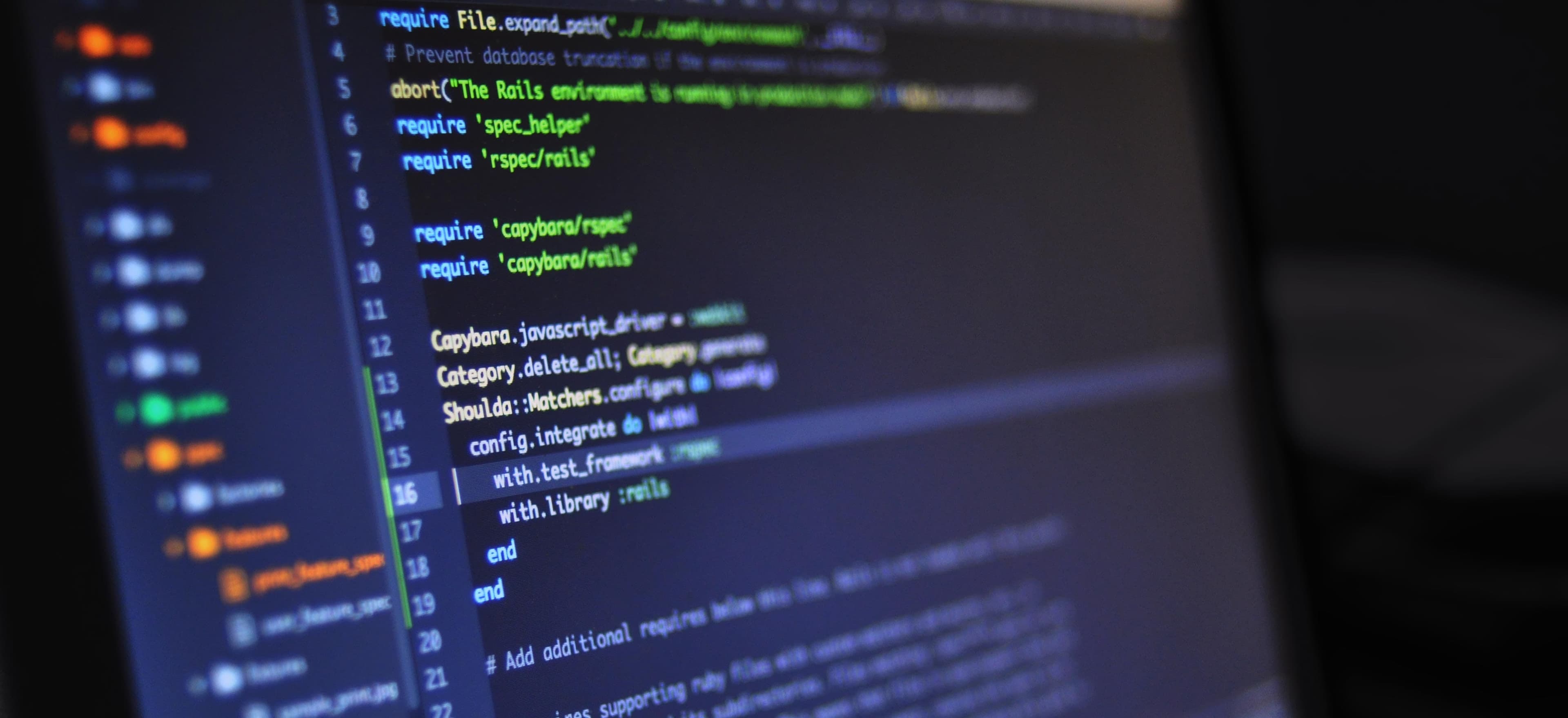
- Published on
Overcoming Spring Boot Retry Limitations for Transient Errors
When building resilient applications, especially microservices, handling transient errors gracefully is crucial. Spring Boot, a popular framework for building Java applications, provides a straightforward way to implement retries, yet it has its limitations. In this blog post, we will explore Spring Boot's retry capabilities, the limitations you may encounter, and practical solutions to overcome them.
Understanding Transient Errors
Before diving into the retry mechanism, let’s first clarify what transient errors are. Transient errors are temporary issues that resolve themselves after a short period. Examples include:
- Network timeouts
- Database connection failures
- Temporary unavailability of a service
The key characteristic of transient errors is that they are usually short-lived. Thus, implementing a retry mechanism can effectively mitigate their effects.
Spring Boot Retry Support
Spring Retry is a library that simplifies the implementation of retry logic. It allows you to retry a method call with configurable backoff strategies. With Spring Boot, you can easily add it to your project using Maven or Gradle.
Adding Spring Retry Dependency
To use Spring Retry in your application, you need to include the dependency. Here’s how to add it to your pom.xml
if you're using Maven:
<dependency>
<groupId>org.springframework.retry</groupId>
<artifactId>spring-retry</artifactId>
<version>2.4.2</version>
</dependency>
For Gradle users, include the following line in your build.gradle
:
implementation 'org.springframework.retry:spring-retry:2.4.2'
Basic Usage of Spring Retry
Once you add the dependency, you can start using Spring Retry. Let’s look at a simple example of a service calling an external API which might fail transiently.
import org.springframework.retry.annotation.Backoff;
import org.springframework.retry.annotation.EnableRetry;
import org.springframework.retry.annotation.Recovery;
import org.springframework.retry.annotation.Retryable;
import org.springframework.stereotype.Service;
import org.springframework.web.client.RestTemplate;
@Service
@EnableRetry
public class ApiService {
private final RestTemplate restTemplate;
public ApiService(RestTemplate restTemplate) {
this.restTemplate = restTemplate;
}
@Retryable(
value = { RuntimeException.class },
maxAttempts = 5,
backoff = @Backoff(delay = 2000))
public String callExternalService() {
// Simulating an external service call
return restTemplate.getForObject("http://external-service/api/data", String.class);
}
@Recovery
public String recovery(RuntimeException e) {
// This method is called when all retry attempts fail
return "Fallback response";
}
}
Analyzing the Code
-
@EnableRetry: This annotation enables retry functionality on the class level.
-
@Retryable: This annotation controls the retry behavior. You can specify:
value
: The exceptions that should trigger a retry. In this case, we retry forRuntimeException
.maxAttempts
: The maximum number of retry attempts. In our example, it retries up to 5 times.backoff
: This defines the delay between attempts. We set a delay of 2000 milliseconds.
-
Recovery Method: If all retry attempts fail, the recovery method is invoked. This can handle the error gracefully, providing a fallback response.
Limitations of Spring Retry
While Spring Retry provides basic functionality, some limitations exist, particularly when dealing with complex scenarios:
-
Limited Retry Strategies: Out of the box, Spring Retry may not support advanced retry strategies, like fixed delay, exponential backoff, or jitter.
-
No Context Propagation: If you have an existing context (like user session, transaction state, etc.), it won't be propagated to retries.
-
Granular Control: Different methods may require different retry logic, which can become cluttered in a single service class.
Overcoming Limitations with Custom Solutions
To address these limitations, you can implement custom retry logic. This allows for greater flexibility and adaptability in your application.
Implementing Custom Retry Logic
Our custom retry solution can be based on ExecutorService, which allows us to run retries in a non-blocking manner.
import java.util.concurrent.Callable;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
import java.util.concurrent.TimeUnit;
public class CustomRetry {
private final ExecutorService executorService = Executors.newSingleThreadExecutor();
public <T> T retry(Callable<T> task, int maxAttempts, long delay) throws Exception {
Exception lastException = null;
for (int attempt = 1; attempt <= maxAttempts; attempt++) {
try {
return task.call(); // Try the callable task
} catch (Exception e) {
lastException = e; // Catch the exception for the retry attempt
if (attempt == maxAttempts) {
throw e; // If max attempts reached, throw the last exception
}
TimeUnit.MILLISECONDS.sleep(delay); // Wait before the next attempt
}
}
throw lastException;
}
}
Usage Example
Here’s how you can use the custom retry logic with a service:
public String getDataWithRetry() {
CustomRetry customRetry = new CustomRetry();
try {
return customRetry.retry(() -> externalService.callExternalService(), 5, 2000);
} catch (Exception e) {
return "Fallback response after retries.";
}
}
Advantages of Custom Retry Logic
-
Flexibility: You can modify the retry behavior according to specific demands, such as implementing different backoff strategies for different services.
-
Context Awareness: With custom logic, it is easier to pass along context or maintain state across retries.
-
Handling Complex Scenarios: You can integrate custom logic for logging, metrics, or alerts when retries are triggered.
A Final Look
Handling transient errors effectively is crucial for creating resilient applications. While Spring Boot provides a solid foundation for implementing retry logic, it's essential to recognize its limitations. Custom retry logic allows for more flexibility, context awareness, and the ability to tailor retries to the specific needs of your application.
For more detailed information, check out the official Spring Retry Documentation, where you’ll find additional features and configurations.
In conclusion, combining Spring Retry's strengths with custom implementations can greatly improve error handling and fault tolerance. Implement these strategies in your projects to deliver robust Java applications that can gracefully manage transient failures.
Checkout our other articles