Mastering Multitenancy in Java EE 7: Top Challenges Explained
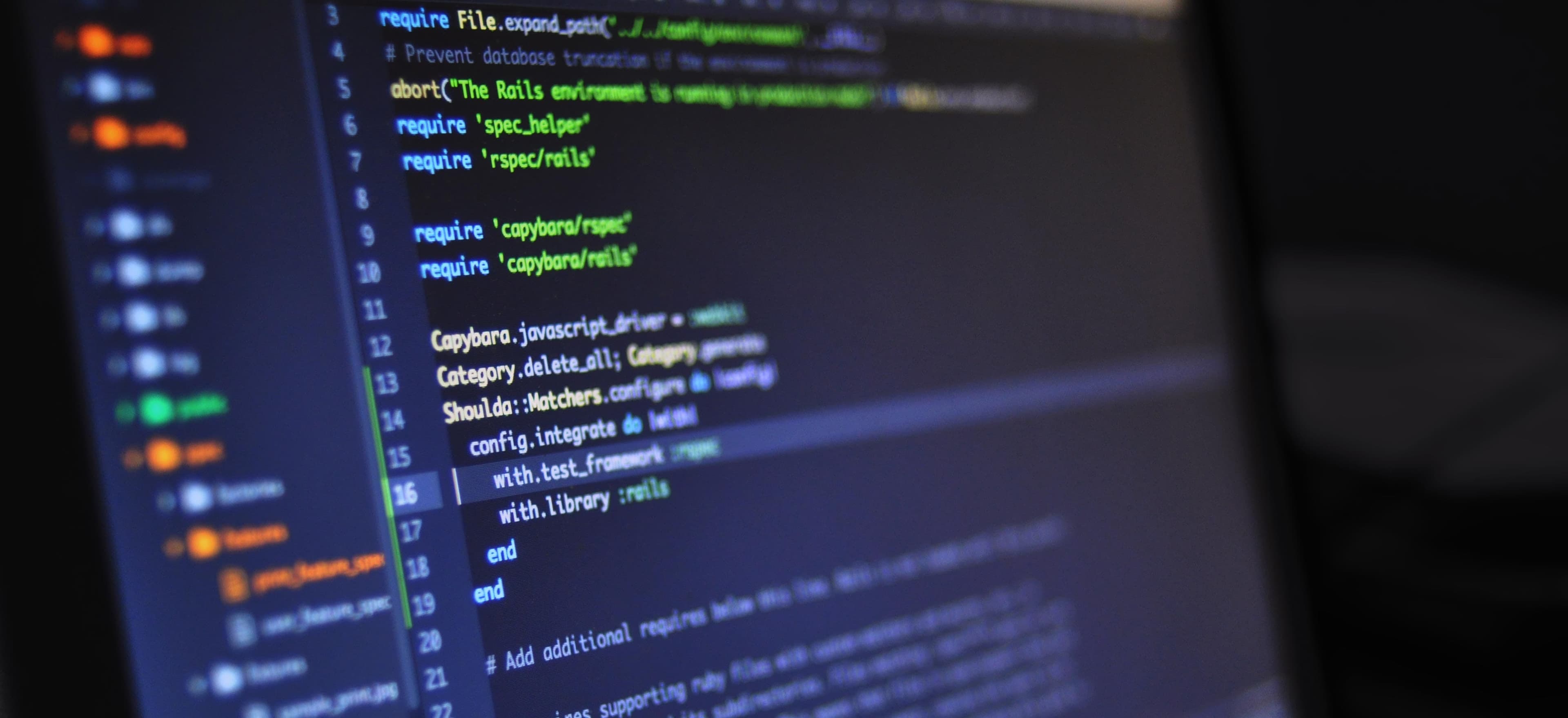
- Published on
Mastering Multitenancy in Java EE 7: Top Challenges Explained
In today's software landscape, multitenancy has become a pivotal design pattern for applications, especially for Software as a Service (SaaS) platforms. In Java EE 7, multitenancy enables a single application instance to serve multiple tenants, each with unique data and configurations. However, implementing a robust multitenant architecture presents several challenges. This post will delve into these challenges, highlight effective solutions, and provide code snippets to illustrate key concepts.
What is Multitenancy?
Before diving into the challenges, let's clarify what multitenancy is. In its essence, multitenancy involves a single instance of an application serving multiple customers (tenants). Each tenant's data remains isolated and secure, ensuring privacy and customization capabilities.
Types of Multitenancy
- Database-per-tenant: Each tenant has their own database. This approach offers maximum isolation but can be resource-intensive.
- Schema-per-tenant: All tenants share a single database, but each tenant has a dedicated schema. This provides a middle ground between performance and isolation.
- Table-per-tenant: Tenants share the same tables, distinguished by a tenant ID. This is the most resource-efficient but can complicate queries and security.
Each strategy comes with pros and cons in terms of complexity, isolation, and performance.
Challenges of Implementing Multitenancy in Java EE 7
1. Data Isolation
Challenge: Ensuring that tenants cannot access each other's data is paramount. Any breach could lead to significant liability issues.
Solution: Implement a robust security model that enforces tenant identification at the database layer. Using JPA (Java Persistence API), tenant information can be included in every query.
Code Snippet: Implementing Tenant ID in JPA
@Entity
@Table(name = "users")
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
// Tenant ID to isolate data
@Column(name = "tenant_id")
private String tenantId;
// Getters and Setters
}
Commentary: Here, we introduce a tenantId
field in the User entity. Every query using this entity should filter by tenantId
, ensuring that users can only access their own data.
2. Configuration Management
Challenge: Different tenants may require different configurations, such as feature sets or UI design elements.
Solution: Utilize a centralized configuration management system and load tenant configurations dynamically.
Code Snippet: Loading Tenant Configurations
@Singleton
public class TenantConfigLoader {
private final Map<String, TenantConfig> configs = new HashMap<>();
@PostConstruct
public void init() {
// Load configurations for each tenant from a file or database
}
public TenantConfig getConfig(String tenantId) {
return configs.get(tenantId);
}
}
Commentary: This singleton service loads tenant configurations at startup. By achieving this separation, applications can cater to diverse tenant needs without hard-coding configurations.
3. Performance Optimization
Challenge: As the number of tenants increases, so does the complexity of managing queries, caching, and connections.
Solution: Employ connection pooling and optimize queries by indexing tenant-specific columns.
Code Snippet: Connection Pooling with HikariCP
<dependency>
<groupId>com.zaxxer</groupId>
<artifactId>HikariCP</artifactId>
<version>5.0.0</version>
</dependency>
Commentary: HikariCP is a fast connection pool. Ensure to configure it properly in persistence.xml
to maximize connection use efficiency:
<persistence-unit name="myPersistenceUnit">
<properties>
<property name="javax.persistence.jdbc.url" value="jdbc:mysql://localhost:3306/mydb"/>
<property name="javax.persistence.jdbc.user" value="user"/>
<property name="javax.persistence.jdbc.password" value="password"/>
<property name="hibernate.hbm2ddl.auto" value="update"/>
<property name="hibernate.show_sql" value="true"/>
<property name="hibernate.hikari.maximumPoolSize" value="10"/>
</properties>
</persistence-unit>
With pooled connections, your application remains responsive, even with multiple tenants.
4. Tenant Provisioning and De-provisioning
Challenge: Onboarding and offboarding tenants efficiently while maintaining data integrity is crucial.
Solution: Develop an automated provisioning system that can create, delete, and update tenant information seamlessly.
Code Snippet: Simple Tenant Provisioning Service
@Stateless
public class TenantProvisioningService {
@PersistenceContext
private EntityManager em;
public void provisionTenant(Tenant tenant) {
em.persist(tenant);
// Additional logic to provision resources
}
public void deProvisionTenant(String tenantId) {
Tenant tenant = em.find(Tenant.class, tenantId);
if (tenant != null) {
em.remove(tenant);
// Additional logic for resource cleanup
}
}
}
Commentary: This stateless session bean manages the lifecycle of tenant entities, ensuring they are provisioned and deprovisioned without manual intervention.
5. Reporting and Analytics
Challenge: Aggregating data for reporting while maintaining data isolation can be challenging.
Solution: Implement a dedicated analytics database, where tenant data can be mirrored for analysis without compromising security.
Code Snippet: Reporting Query
public List<User> getUsersByTenant(String tenantId) {
TypedQuery<User> query = em.createQuery("SELECT u FROM User u WHERE u.tenantId = :tenantId", User.class);
query.setParameter("tenantId", tenantId);
return query.getResultList();
}
Commentary: This query ensures that only users from the specified tenant are returned, safeguarding against data leakage during analytics operations.
The Closing Argument
Multitenancy is a powerful paradigm that allows Java EE applications to efficiently serve multiple clients without compromising isolation, security, or performance. While the implementation of a multitenant architecture presents various challenges, understanding these obstacles and employing the right strategies, such as utilizing JPA effectively, managing tenant configurations, and optimizing performance, can lead to a successful multitenant solution.
For further reading on multitenancy in Java applications, check out articles on Baeldung and JPA. Familiarizing yourself with the best practices and common pitfalls can give you an edge in implementing a robust multitenant solution.
Remember, the journey to mastering multitenancy is ongoing. Embrace the challenges, learn continuously, and adapt your approaches as necessary. Your tenancy management system will thrive as you put these principles into practice. Happy coding!