Unveiling the 97% of Java Logged Errors: Causes Explained
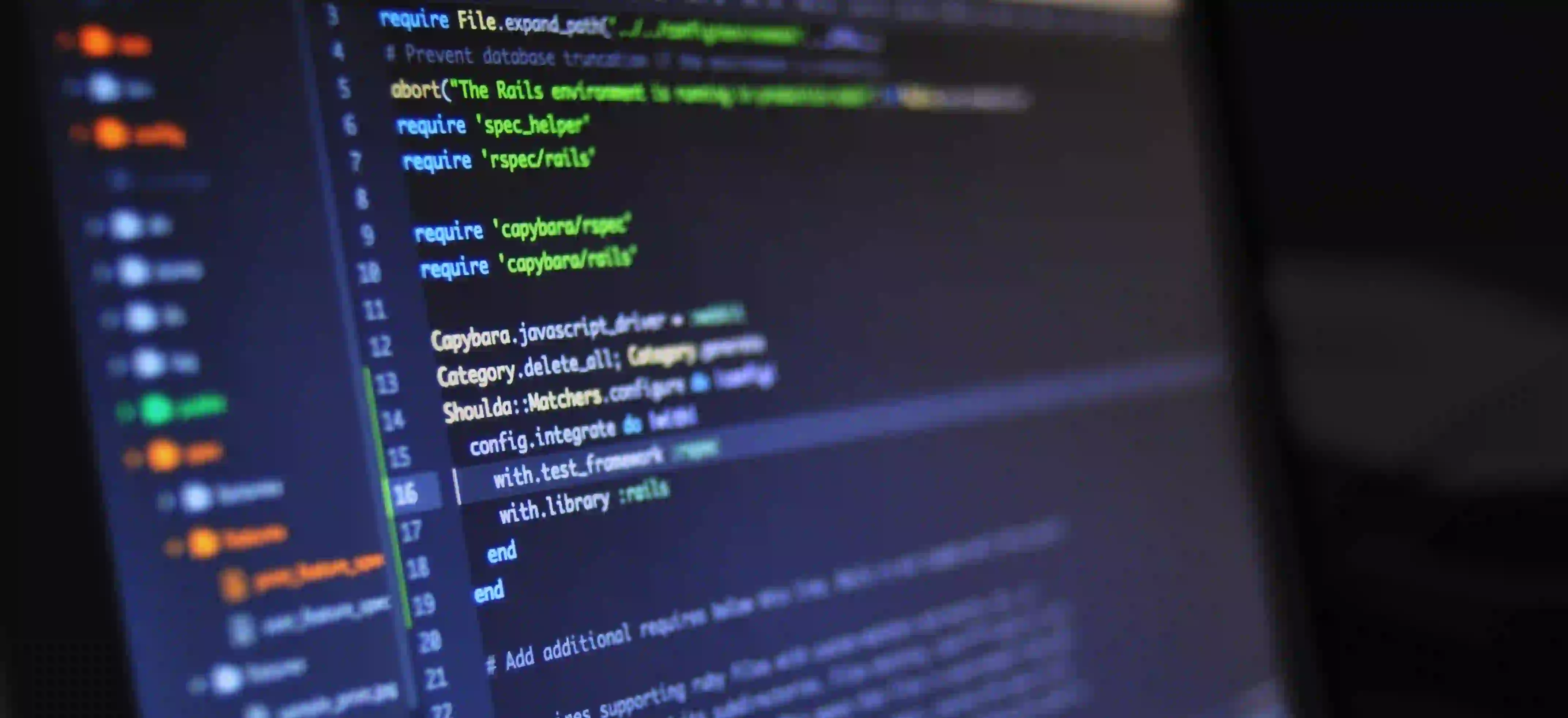
Unveiling the 97% of Java Logged Errors: Causes Explained
Java, a charismatic and widely-used programming language, has transformed the landscape of software development. With its robust capabilities, it’s employed in everything from web applications to Android development. However, despite its strengths, Java applications are not immune to errors. An astounding 97% of logged errors in Java can often be traced back to a few common causes. This blog post will explore these causes in detail, providing you with the tools to diagnose and rectify these issues effectively.
Table of Contents
- Understanding Java Error Types
- Common Causes of Logged Errors
- Best Practices for Error Handling
- Utilizing Logging Effectively
- Conclusion
Understanding Java Error Types
Before diving into the specifics, let's clarify various Java errors. Broadly speaking, Java categorizes errors into exceptions and errors.
-
Exceptions: These are events that disrupt the normal flow of a program. They are usually recoverable. For instance, handling a user input error.
-
Errors: These are more severe issues indicating problems with the Java runtime environment. They are not usually recoverable, such as
OutOfMemoryError
.
In this discussion, we will primarily focus on exceptions, given that they make up a significant chunk of logged errors.
Common Causes of Logged Errors
1. NullPointerException
The infamous NullPointerException
(NPE) is one of the most common exceptions in Java. It occurs when the Java Virtual Machine (JVM) attempts to use an object reference that has not been initialized (i.e., it points to null).
public class Example {
private String message;
public void printMessage() {
System.out.println(message.length()); // This will throw NPE if message is null
}
}
Why This Happens: If the message
variable has not been assigned any value, attempting to call .length()
results in a runtime exception.
Preventive Measures: Use proper null checks before using an object. You could implement:
if (message != null) {
System.out.println(message.length());
} else {
System.out.println("Message is null");
}
2. ArrayIndexOutOfBoundsException
As the name suggests, an ArrayIndexOutOfBoundsException
occurs when an attempt is made to access an array with an index that does not exist.
public class ArrayExample {
public static void main(String[] args) {
int[] numbers = {1, 2, 3};
System.out.println(numbers[3]); // This will cause an ArrayIndexOutOfBoundsException
}
}
Why This Happens: In the above example, the valid indices for numbers
are 0, 1, and 2. Accessing index 3 throws the exception.
Preventive Measures: Ensure that you verify indices before accessing arrays. A robust approach would be:
if (index >= 0 && index < numbers.length) {
System.out.println(numbers[index]);
} else {
System.out.println("Index out of bounds");
}
3. ClassCastException
A ClassCastException
occurs when you attempt to cast an object of one type to another type that is not compatible.
public class CastExample {
public static void main(String[] args) {
Object obj = new Integer(1);
String str = (String) obj; // This will throw ClassCastException
}
}
Why This Happens: The object obj
holds an integer value, and trying to cast it to a String
results in a ClassCastException.
Preventive Measures: Use the instanceof
keyword to check the object's type before casting.
if (obj instanceof String) {
String str = (String) obj;
} else {
System.out.println("Object is not a String");
}
4. SQLException
SQLException
is commonly encountered when dealing with database connectivity. It can arise due to a variety of reasons, like syntax errors in SQL queries or issues with accessing a database.
public void executeQuery() {
Connection conn = null;
Statement stmt = null;
try {
conn = DriverManager.getConnection("jdbc:mysql://localhost/test", "user", "password");
stmt = conn.createStatement();
String sql = "SELECT * FROM non_existing_table"; // This query will throw SQLException
stmt.executeQuery(sql);
} catch (SQLException e) {
e.printStackTrace();
} finally {
// Ensure the connection and statement are closed
}
}
Why This Happens: The SQL query is attempting to access a non-existing entity, which triggers the exception.
Preventive Measures: Validate SQL syntax carefully before execution, and utilize prepared statements to reduce the risk of SQL injection.
5. ConcurrentModificationException
This exception arises when an object is modified concurrently while iterating over it:
import java.util.ArrayList;
import java.util.List;
public class ConcurrentModificationExample {
public static void main(String[] args) {
List<Integer> list = new ArrayList<>();
list.add(1);
list.add(2);
for (Integer number : list) {
list.remove(number); // This will throw ConcurrentModificationException
}
}
}
Why This Happens: The list is altered while being iterated, leading to an inconsistency.
Preventive Measures: Use an explicit iterator to safely remove elements from a collection.
Iterator<Integer> iterator = list.iterator();
while (iterator.hasNext()) {
Integer number = iterator.next();
if (number.equals(1)) {
iterator.remove(); // Safe removal
}
}
Best Practices for Error Handling
-
Use Try-Catch Blocks Judiciously: Enclose code sections that may throw exceptions within try-catch blocks. Avoid wrapping large code segments as it can lead to ambiguous error handling.
-
Log Meaningful Messages: Always log an exception's message or a custom description to facilitate easier debugging. Utilize logging frameworks like Log4j and SLF4J for better logging capabilities.
-
Graceful Degradation: Design your application to handle errors gracefully, providing fallback mechanisms instead of crashing.
-
Keep Exceptions Localized: Only catch exceptions where you can handle them meaningfully. Let others propagate if you cannot do anything useful.
Utilizing Logging Effectively
Logging plays a pivotal role in diagnosing issues. Here are effective practices:
-
Choose the Right Logger Level: Use levels such as
DEBUG
,INFO
,WARN
,ERROR
, andFATAL
appropriately. This helps in filtering logs based on severity. -
Log in Context: Provide relevant context to the logs such as user actions, input values, or state changes.
-
Analyze Logs Regularly: Invest time in analyzing the logs to identify recurring issues and potential enhancements.
The Last Word
Java's robustness often leads to intricate applications that can run into operational issues, resulting in logged errors. Understanding common exceptions like NullPointerException
, ArrayIndexOutOfBoundsException
, and others can empower developers to craft more resilient applications.
By adhering to best practices for error handling and making good use of logging, you can significantly reduce the number of logged errors and improve the overall health of your Java applications.
For further reading on exception handling in Java, you might find the following resources useful:
Embrace these insights, apply them diligently, and elevate your Java programming craft to new heights!