Conquering Software Maintenance: Key Strategies to Succeed
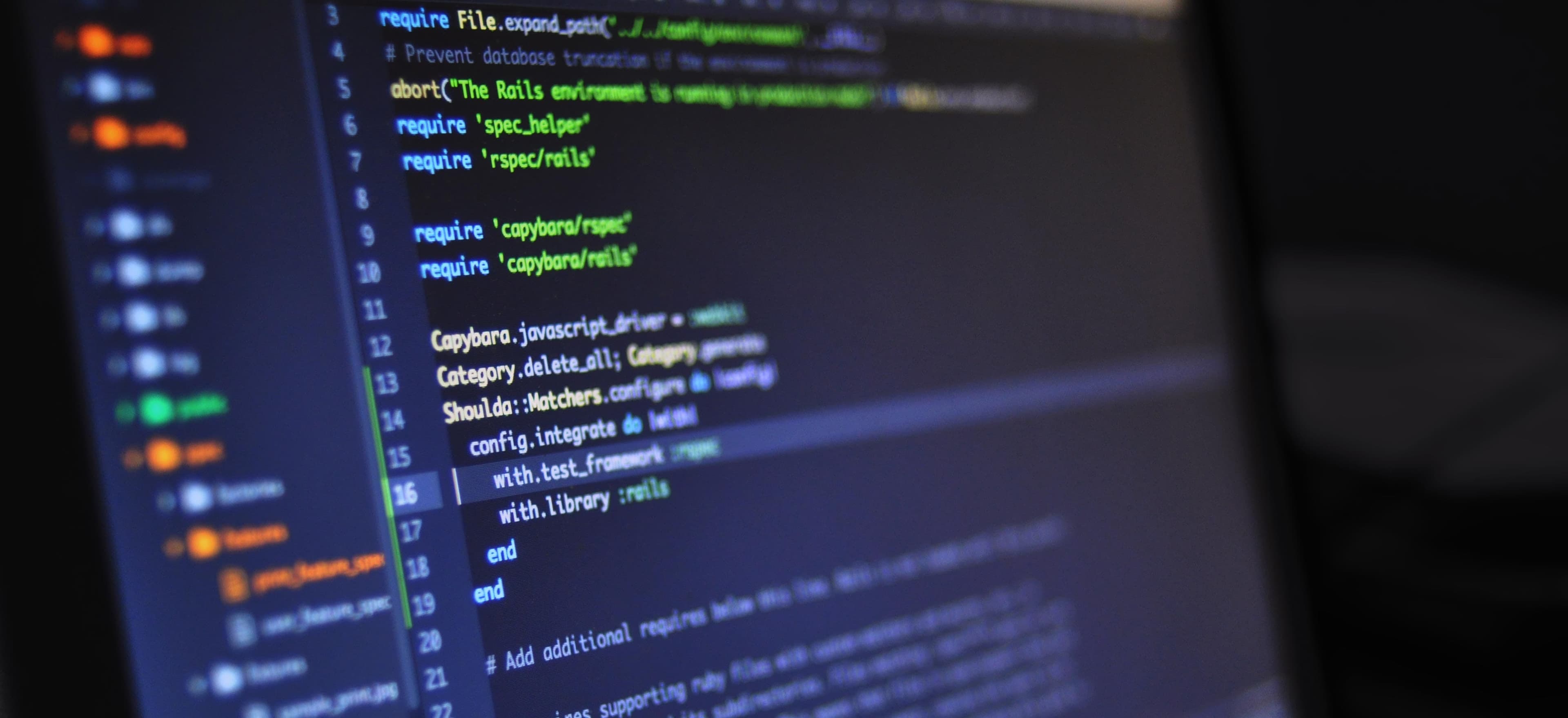
- Published on
Conquering Software Maintenance: Key Strategies to Succeed
Maintaining software is an essential yet often overlooked aspect of software development. While creating an application is challenging, ensuring its longevity and efficiency in the long run can be even tougher. This blog post dives deep into the complexities of software maintenance and outlines key strategies to succeed in this crucial area.
Understanding Software Maintenance
Software maintenance refers to the process of updating, enhancing, and fixing software applications after their initial development. This includes:
- Bug Fixes: Rectifying issues that impede functionality.
- Updates: Enhancing existing features or integration of new technologies.
- Performance Improvement: Ensuring the application runs efficiently as environments and user demands evolve.
Before delving into effective strategies, it’s essential to recognize the types of software maintenance:
- Corrective Maintenance: This involves fixing bugs or deficiencies.
- Adaptive Maintenance: Modifying software to accommodate changing environments.
- Perfective Maintenance: Improving software to enhance performance or other non-critical aspects.
- Preventive Maintenance: Implementing updates or changes to anticipate future issues.
Key Strategies for Successful Software Maintenance
1. Establish a Robust Documentation Process
Comprehensive documentation can be your best ally. It serves as a reference for developers and ensures that everyone understands the application’s intricacies. Good documentation includes:
- Technical Documentation: Details the software’s architecture, codebase, and design decisions.
- User Manuals: Guides for the end-users highlighting features and usability.
Why Documentation Matters
Without effective documentation, you risk knowledge loss. New developers might struggle to understand the software, leading to prolonged downtime and potential mishaps. Additionally, maintaining consistent documentation encourages adherence to coding standards and practices.
Example of Effective Documentation Structure:
# Project Overview
## Architecture
- Overview of system components
- Diagram and flow of data
## Codebase
- Directory structure
- Key modules and their responsibilities
## Known Issues
- Bugs found and their resolutions
2. Prioritize Code Quality
Maintaining high-quality code should be a priority in every development cycle. Implement code review processes and coding standards to ensure maintainability. Tools like SonarQube can help automate code quality analysis.
Why Quality Matters
Code that is clean, well-structured, and concise is easier to read and modify. Poor-quality code accumulates technical debt, making future changes more costly and labor-intensive.
Example of Improving Code Quality:
// Example of Code Review: Avoiding Long Methods
public class Example {
// Long Method
public void processOrder(Order order) {
// validation code...
// processing code...
// notification code...
}
}
// Improved by Splitting
public void processOrder(Order order) {
validateOrder(order);
processPayment(order);
notifyCustomer(order);
}
private void validateOrder(Order order) {
// validation code...
}
private void processPayment(Order order) {
// processing code...
}
private void notifyCustomer(Order order) {
// notification code...
}
3. Implement Automated Testing
Automated testing is a game-changer when it comes to software maintenance. Testing frameworks such as JUnit for Java create repeatable and reliable test cases that can catch bugs early.
Why Automation is Key
Automation significantly reduces the time needed for regression testing. When a developer makes changes, running automated tests ensures that existing functionalities remain intact. This leads to faster deployment and a much smoother workflow.
Example of a Simple JUnit Test:
import static org.junit.jupiter.api.Assertions.*;
import org.junit.jupiter.api.Test;
public class OrderProcessorTest {
@Test
void testOrderProcessing() {
OrderProcessor processor = new OrderProcessor();
Order order = new Order();
order.setAmount(100);
String result = processor.processOrder(order);
assertEquals("Processed", result);
}
}
4. Use Version Control Systems
Utilizing version control systems like Git allows for better collaboration among developers and helps keep track of changes to the codebase efficiently.
Why Version Control is Imperative
Version control systems enable team members to work concurrently without overwriting each other’s work. You can revert to previous versions of code if bugs are introduced, ensuring that you have a stable base to work from.
5. Regular Updates and Refactoring
Regularly updating and refactoring code can prevent obsolescence. Using the latest libraries and frameworks can lead to performance improvements.
Why Refactoring is Important
Refactoring is an ongoing process that allows you to improve the internal structure without changing its external behavior. This is key to reducing technical debt, ensuring that software remains manageable and less prone to bugs.
Example of Refactoring to Enhance Clarity:
// Before Refactoring
public double calculateDiscount(double price, int quantity) {
return price * quantity > 1000 ? price * 0.10 : 0;
}
// After Refactoring for Clarity
public double calculateBulkDiscount(double price, int quantity) {
if (isBulkOrder(price, quantity)) {
return price * 0.10;
}
return 0;
}
private boolean isBulkOrder(double price, int quantity) {
return price * quantity > 1000;
}
6. Establish a Maintenance Plan
A well-defined maintenance plan can delineate the steps and strategies involved in maintaining the software. This plan should include:
- Scheduling regular updates and testing cycles.
- Defining roles and responsibilities among team members.
- Establishing clear lines for user feedback.
Why Planning is Essential
If you fail to plan, you plan to fail. A structured approach fosters accountability while ensuring that users can expect timely support and updates.
7. Prioritize User Feedback
Regularly soliciting user feedback enables you to calibrate your maintenance efforts effectively. This can be through surveys, user interviews, or direct communication channels.
Why User Feedback is Vital
Users provide insights that the development team may overlook. They encounter real-world scenarios that reveal bugs, usability issues, and areas for improvement. Listening to your users is paramount to sustained software relevance.
Final Thoughts
Software maintenance is an ongoing commitment that requires diligence, regular updates, and a proactive approach. By implementing the strategies outlined above, organizations can better navigate the complexities of software maintenance and broaden the longevity and effectiveness of their applications.
Remember, maintaining your software is just as important as developing it. The strategies you apply today will yield returns in performance, user satisfaction, and reduced costs in the future. Always focus on quality, automation, documentation, and user interaction to ensure your applications are not just surviving but thriving.
For further reading, consider looking into resources like Martin Fowler's Refactoring or Code Complete by Steve McConnell for nuanced insights into software development and maintenance practices. Happy coding!
Checkout our other articles