Common Pitfalls in Building Reactive Systems with JavaFX
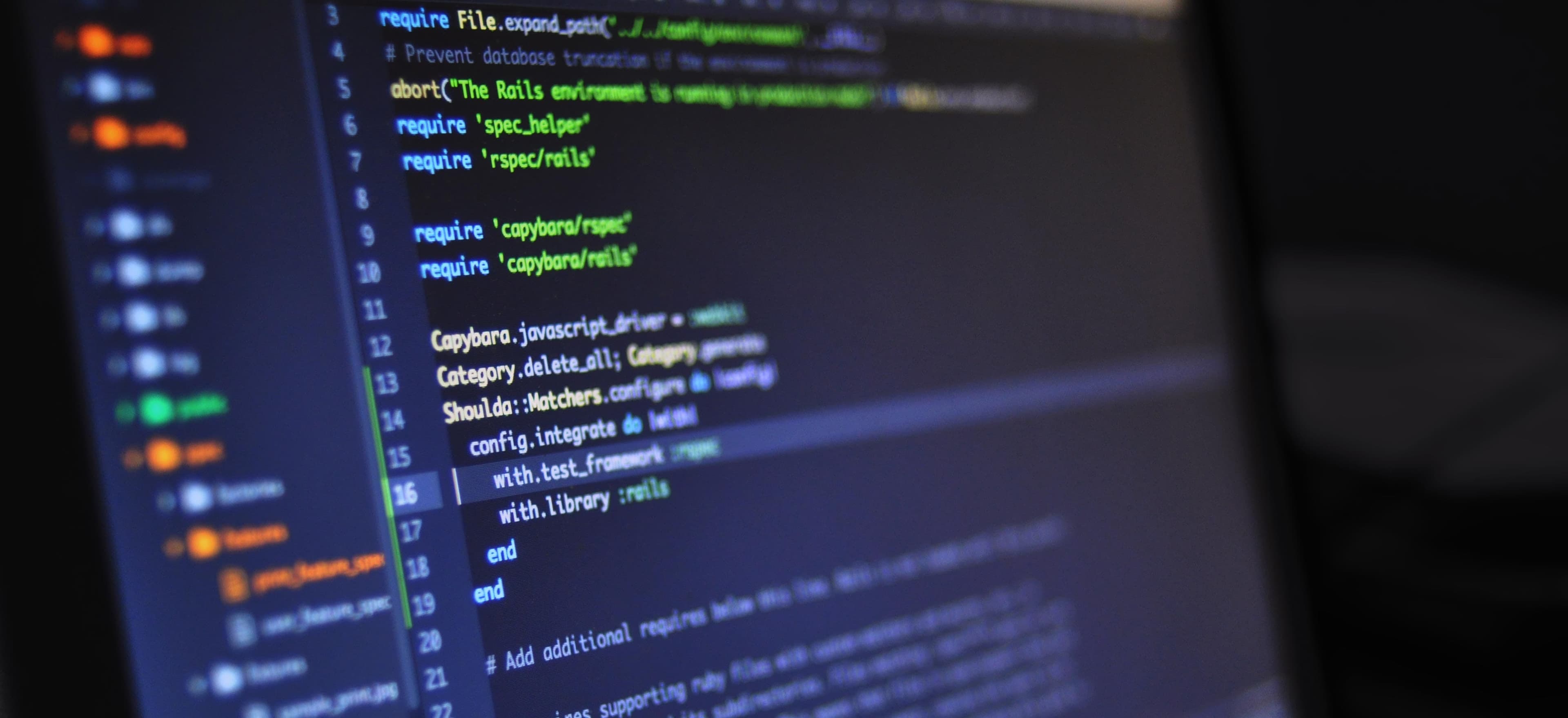
- Published on
Common Pitfalls in Building Reactive Systems with JavaFX
Building reactive systems with JavaFX can be a rewarding but complex endeavor. The reactive programming paradigm emphasizes asynchronous data streams and the propagation of change, which suits responsive GUIs well. However, developers often encounter pitfalls along the way. In this article, we will explore common challenges and how to avoid them, along with best practices for developing effective reactive systems using JavaFX.
Understanding Reactive Systems
Before diving into specific pitfalls, it’s essential to grasp what reactive programming is. Reactive programming is a programming paradigm focused on the data streams and the propagation of change. In JavaFX, reactive programming helps you build applications that respond asynchronously to user actions, external events, or other data changes.
The core principles of reactive programming align well with the JavaFX framework:
- Asynchronous: Non-blocking code execution.
- Event-driven: Responds to changes or events rather than pushing changes.
- Composition: Composes complex functionalities using simple parts.
Now, let’s discuss some common pitfalls that developers face when building reactive systems with JavaFX.
Pitfall #1: Blocking the UI Thread
One of the most common mistakes when developing JavaFX applications is blocking the UI thread. Since all JavaFX UI updates must occur on the JavaFX Application Thread, performing long-running tasks in this thread can freeze the UI, leading to a poor user experience.
Example
public void performLongRunningTask() {
// This should NOT be done on the JavaFX Application Thread
System.out.println("Processing...");
try {
Thread.sleep(10000); // Simulating a long task
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println("Task finished.");
}
Solution
Use Java's concurrency utilities, such as Task
, to perform long-running operations in a background thread. JavaFX’s Task
can also be used to update the UI once the task completes.
Correct Implementation
import javafx.concurrent.Task;
public void startTask() {
Task<Void> task = new Task<Void>() {
@Override
protected Void call() throws Exception {
// Perform a long-running task here
for (int i = 0; i < 10; i++) {
Thread.sleep(1000); // Simulating work
updateMessage("Step " + (i + 1) + " completed");
}
return null;
}
@Override
protected void succeeded() {
System.out.println("Task succeeded.");
}
};
// Bind task output to UI components
task.messageProperty().addListener((observable, oldValue, newValue) -> {
System.out.println(newValue);
// Update a label or UI component here
});
// Start the task in a background thread
new Thread(task).start();
}
This code demonstrates how to avoid blocking the UI thread by creating a Task
that runs in the background.
Pitfall #2: Ignoring Error Handling
Reactive programming inherently involves dealing with asynchronous operations, and with it often comes the risk of encountering errors that can lead to hard-to-track bugs.
Example
Imagine if a network request fails and you don't catch the exception; this can result in unhandled exceptions propagating, leading to an application crash.
Solution
Always include comprehensive error handling in your reactive systems. With JavaFX's Task
, you can override the failed
method to handle exceptions gracefully.
Correct Implementation
@Override
protected void failed() {
Throwable ex = getException(); // Get the underlying exception
System.err.println("Error occurred: " + ex.getMessage());
// Display error message to the UI or log it
}
Make sure to log errors and notify users or developers about failures appropriately.
Pitfall #3: Bad Data Binding Practices
JavaFX heavily relies on properties and bindings. Misusing these can lead to performance issues or data inconsistencies.
Example
When you bind two properties inappropriately, it can lead to unnecessary updates, resulting in heavy processing.
Solution
Use bindings judiciously. Understand the lifecycle of your properties and avoid redundant bindings where possible.
Correct Implementation
IntegerProperty ageProperty = new SimpleIntegerProperty();
StringProperty eligibilityProperty = new SimpleStringProperty();
eligibilityProperty.bind(Bindings.createStringBinding(() -> {
if (ageProperty.get() < 18) {
return "Not Eligible";
} else {
return "Eligible";
}
}, ageProperty));
In this code snippet, eligibilityProperty
is reactive to changes in ageProperty
, which is a clear and efficient use of bindings.
Pitfall #4: Overcomplicating State Management
In larger applications, developers may start adding layers of complexity to reactively manage state. While state management is crucial, overly complicated state logic can bloat your codebase and make maintenance harder.
Solution
- Keep it Simple: Use JavaFX’s built-in properties whenever possible.
- Consider using external libraries: Libraries like RxJava can help manage state and data streams effectively without reinventing the wheel.
Pitfall #5: Not Leveraging JavaFX Features
JavaFX comes with multiple components designed for reactive programming, such as EventHandler
, ChangeListener
, fires and observers.
Example
Instead of handling everything manually, you can use JavaFX's listener model effectively.
Solution
Listen for changes in properties and handle them appropriately. Always prefer built-in functionality first.
label.textProperty().bind(myStringProperty);
In this example, whenever myStringProperty
changes, label
will automatically update its text without extra code.
Wrapping Up
Building reactive systems with JavaFX can be a complex undertaking, but recognizing and avoiding these common pitfalls will help you create robust, efficient applications. Always remember to keep the UI responsive by offloading lengthy computations to background threads, handle errors gracefully, use bindings wisely, keep your state management simple, and utilize JavaFX's rich set of features.
For more about JavaFX and reactive programming, consider checking out Javadocs for JavaFX and ReactiveX documentation.
With practice and awareness of these pitfalls, you can create highly responsive and user-friendly applications that capitalize on the strengths of the JavaFX framework. Happy coding!
Checkout our other articles