Common Pitfalls in JUnit4 Parameterized Testing
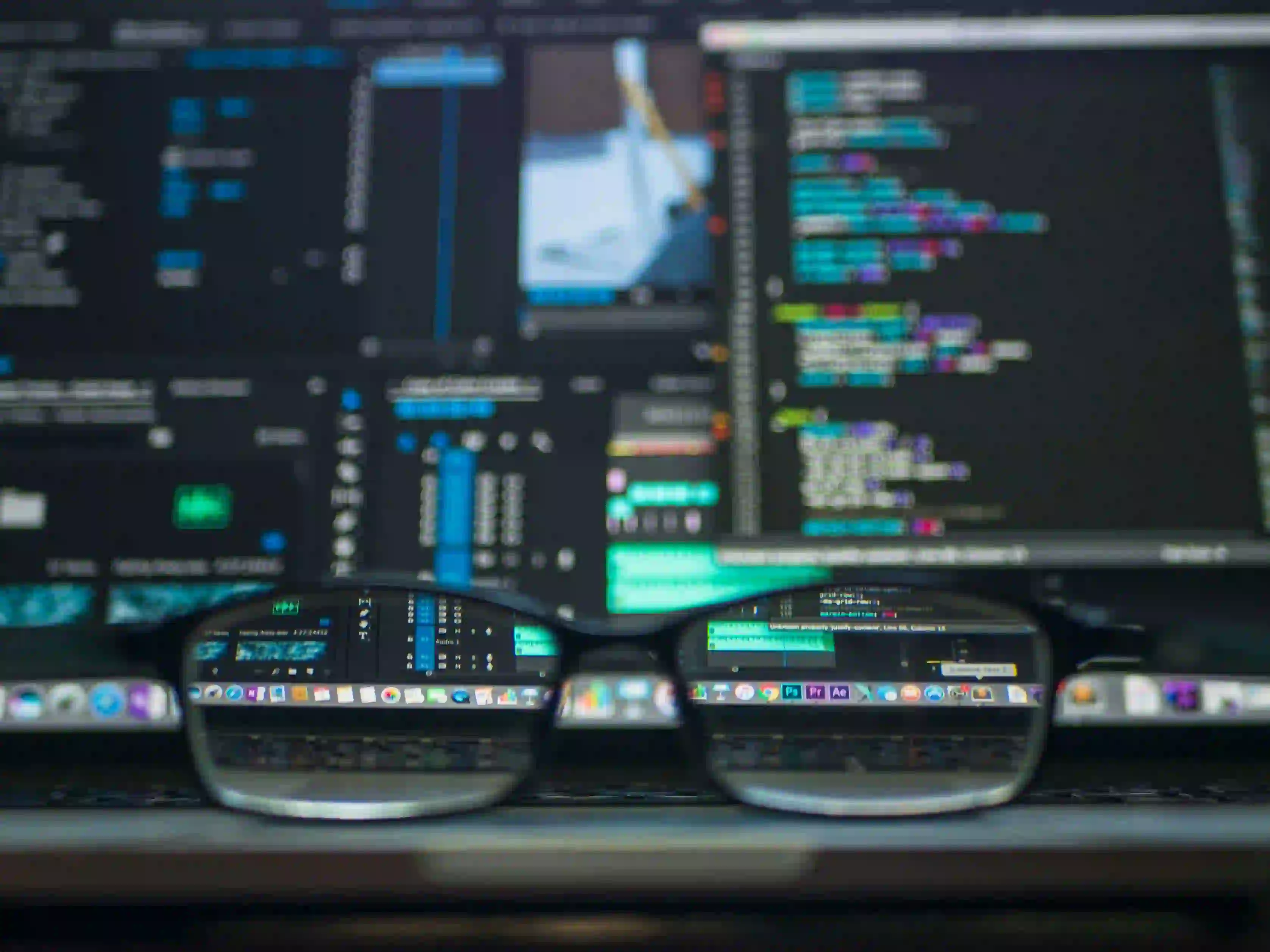
Common Pitfalls in JUnit4 Parameterized Testing
JUnit is an essential framework for unit testing in Java. It allows developers to write clean, efficient tests that can be run automatically. One of its powerful features is parameterized testing, which allows methods to be run multiple times with different parameters. However, like any powerful tool, it can also lead to common pitfalls if not used correctly. In this blog post, we will explore these pitfalls, provide code snippets, and offer tips on how to avoid them.
What is Parameterized Testing?
Parameterized testing is a methodology that allows you to run the same test with different inputs. Instead of repeating the same test code multiple times, parameterized testing promotes reuse and flexibility. In JUnit4, you can achieve this by using @RunWith(Parameterized.class)
.
Basic Example
Here's a simple example of how parameterized tests work in JUnit4:
import org.junit.Test;
import org.junit.runner.RunWith;
import org.junit.runners.Parameterized;
import java.util.Arrays;
import java.util.Collection;
@RunWith(Parameterized.class)
public class SimpleParameterizedTest {
private int input;
private int expected;
public SimpleParameterizedTest(int input, int expected) {
this.input = input;
this.expected = expected;
}
@Parameterized.Parameters
public static Collection<Object[]> data() {
return Arrays.asList(new Object[][]{
{1, 2}, // This means input 1 should produce output 2
{2, 3}, // This means input 2 should produce output 3
{3, 4} // This means input 3 should produce output 4
});
}
@Test
public void testIncrement() {
assertEquals(expected, input + 1);
}
}
Why Use Parameterized Testing?
- Code Reusability: Instead of writing several test methods, you can manage multiple test cases in one place.
- Improved Readability: It makes the tests easier to understand as you can see different scenarios with corresponding inputs and expected outputs collectively.
Common Pitfalls in JUnit4 Parameterized Testing
1. Ignoring Parameter Order
One of the most common pitfalls is assuming that the order of parameters will always be the same. JUnit runs tests in a way that may not maintain the expected order.
Recommendation: Always test with multiple sets of parameters to ensure that order does not matter.
2. Not Handling Edge Cases
When creating your parameterized tests, it is easy to overlook edge cases. For example, if you are testing a method that must handle negative numbers, include cases for negative inputs.
Example Code Snippet:
@Parameterized.Parameters
public static Collection<Object[]> data() {
return Arrays.asList(new Object[][]{
{1, 2}, // Valid case
{0, 1}, // Edge case
{-1, 0} // Edge case
});
}
3. Hard-Coding Parameter Values
Hard-coding parameter values directly into the test can lead to maintainability issues. If the logic changes, it might require extensive changes to your test cases.
Better Approach: Utilize constants or methods to generate these values.
Example Code Snippet:
public static int[] generateTestInputs() {
return new int[]{1, 2, 3};
}
@Parameterized.Parameters
public static Collection<Object[]> data() {
return Arrays.asList(new Object[][]{
{generateTestInputs()[0], 2},
{generateTestInputs()[1], 3},
{generateTestInputs()[2], 4}
});
}
4. Not Documenting Your Tests
While it might seem trivial, failing to document your tests can lead to confusion over what various parameter combinations represent. Documentation not only helps you but also assists anyone else who might work on your code in the future.
Recommendation: Use comment sections to explain each test case briefly, especially when testing complex scenarios.
5. Complex Test Logic
Your parameterized tests should be straightforward. While parameterized tests can be useful, should the logic inside them get too complex, it warrants a rethink. Complicated tests can hinder readability and debugging.
Keep It Simple: Maintain clarity by breaking down complex test cases into multiple simpler tests.
6. Neglecting the Impact of State
JUnit tests should ideally be stateless. If a parameterized test changes the state in such a way that affects subsequent tests, it becomes hard to track down failures.
Best Practice: Ensure each test is isolated. You can use setup methods annotated with @Before
to reset any shared state before tests run.
Example of State Management
private static int sharedState;
@Before
public void setUp() {
sharedState = 0; // Resetting shared state before each test
}
The Last Word
Using JUnit4's parameterized testing feature can greatly enhance your unit testing strategy. However, being aware of common pitfalls is crucial to ensure your tests remain clear, maintainable, and effective. Always strive to document your tests, manage shared state diligently, and focus on simplicity to avoid unnecessary complexity.
Further Resources
By understanding and embracing these guidelines, you can ensure that your parameterized tests serve their purpose—helping you deliver high-quality, bug-free Java applications.
Happy testing!