Overcoming Common J2Pay Setup Challenges
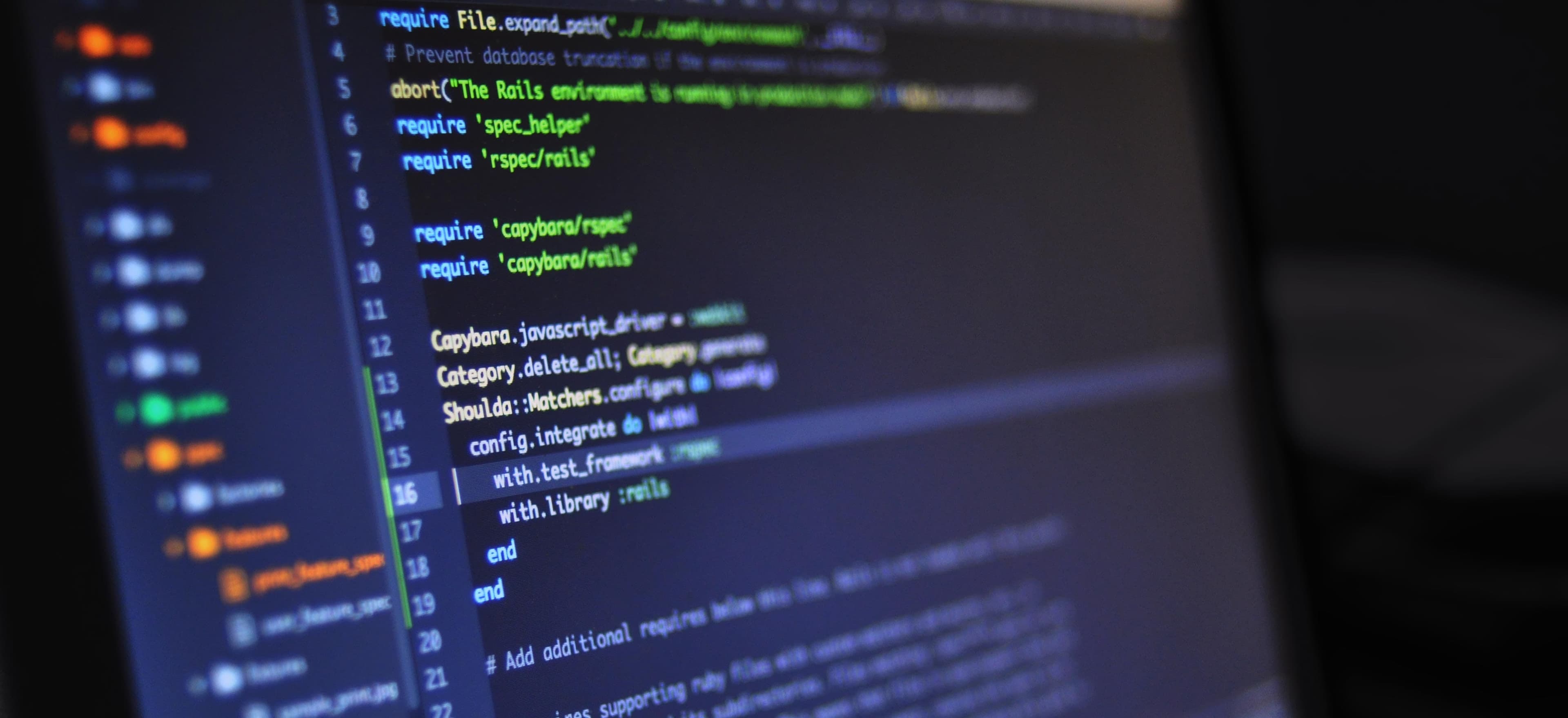
- Published on
Overcoming Common J2Pay Setup Challenges
When diving into the world of payment processing in Java applications, J2Pay emerges as a robust option. However, setting it up comes with its own set of challenges. This blog post outlines the common hurdles developers face while implementing J2Pay and provides practical solutions to overcome these issues. Whether you're a seasoned Java developer or just starting with payment systems, this guide will help you streamline your J2Pay setup.
What is J2Pay?
J2Pay is a Java payment processing library that allows developers to integrate various payment gateways into their applications. It’s designed to be flexible, easy to use, and powerful enough for production environments. Its scope includes handling payments, refunds, and transaction management seamlessly.
Key Features of J2Pay
- Multiple payment gateways support: J2Pay is compatible with multiple payment processors.
- Simple API: The library offers a straightforward API, making integration easier.
- Security features: J2Pay comes with built-in security mechanisms to safeguard data.
Common Setup Challenges
1. Dependency Management
One major challenge in setting up J2Pay is handling dependencies efficiently. New developers may find it overwhelming to resolve all library dependencies correctly.
Solution:
Using a build automation tool like Maven or Gradle can streamline your setup. For Maven users, you can declare dependencies in the pom.xml
configuration file.
Example: Maven Dependency Declaration
<dependency>
<groupId>com.j2pay</groupId>
<artifactId>j2pay</artifactId>
<version>1.0.0</version>
</dependency>
In Gradle, add the following line to your build.gradle
:
implementation 'com.j2pay:j2pay:1.0.0'
This ensures that all required libraries for J2Pay are included without manual downloads.
2. Environment Configuration
Another common challenge is configuring the correct environment variables or properties needed by J2Pay. Credentials, API keys, and payment endpoints can be tricky to set up.
Solution:
Create a configuration file, such as config.properties
, and store your environment-specific information there. This not only streamlines the setup but also secures sensitive data away from the codebase.
Example: Properties File
j2pay.apiKey=YOUR_API_KEY_HERE
j2pay.apiUrl=https://api.yourpaymentgateway.com
Load the properties in your Java application using the Properties
class:
import java.io.FileInputStream;
import java.io.IOException;
import java.util.Properties;
public class ConfigLoader {
public static Properties loadConfig(String filepath) throws IOException {
Properties properties = new Properties();
try (FileInputStream fileInputStream = new FileInputStream(filepath)) {
properties.load(fileInputStream);
}
return properties;
}
}
3. Limited Documentation
Sometimes, developers may struggle with the limited or confusing documentation associated with J2Pay. This can make it difficult to understand how to use specific features or troubleshoot problems.
Solution:
The first step is to visit the official J2Pay GitHub repository and review the provided examples. Also, consider contributing to a documentation improvement or creating a README in your project to clarify usage.
If you're having trouble, don’t hesitate to reach out to the community via forums or Stack Overflow. Many developers face the same issues, and solutions are often shared.
4. Handling Payment Failure Scenarios
Another significant challenge during setup is properly managing payment failure scenarios and ensuring that the application handles these gracefully.
Solution:
Implement robust error handling and notification systems to track payment failures. For example, consider using an interceptor or middleware to handle exceptions that occur during payment processing.
Example: Payment Processing
try {
PaymentResponse response = paymentService.processPayment(paymentRequest);
if (!response.isSuccessful()) {
// Handle failure
logError(response.getErrorMessage());
notifyUser(response.getErrorMessage());
}
} catch (PaymentException ex) {
logError("Payment processing error: " + ex.getMessage());
notifyAdmin("Payment processing error occurred.");
}
5. Testing Payment Integration
Testing payment gateways often introduces complexity, such as simulating different scenarios (successful transactions, payment declines, etc.).
Solution:
Use a sandbox environment provided by payment gateways. J2Pay typically gives developers access to sandbox credentials to test the integration without making real transactions.
Create test cases using automated testing frameworks like JUnit to ensure that all scenarios are covered. Here's a simple example:
Example: JUnit Test Case
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertTrue;
public class PaymentServiceTest {
@Test
public void testSuccessfulPayment() {
// Arrange
PaymentRequest request = new PaymentRequest(/* parameters */);
// Act
PaymentResponse response = paymentService.processPayment(request);
// Assert
assertTrue(response.isSuccessful());
}
}
6. Security Concerns
Security is paramount in payment processing, and integrating J2Pay requires a solid understanding of best security practices.
Solution:
Ensure that you are following secure coding practices. Implement secure SSL/TLS connections and consider encrypting sensitive data before storage.
Always keep your dependencies up to date as they may include security patches. Consider using tools like OWASP Dependency-Check to monitor vulnerabilities in your libraries.
Wrapping Up
Setting up J2Pay for your Java application can be challenging, but by following the solutions provided above, you can overcome these common hurdles. Remember to leverage community support, maintain strong security practices, and utilize robust testing methods to ensure a smooth payment processing experience in your application.
For more details on payment processing in Java, check out these resources:
By sharing knowledge and resources, developers can navigate the complexities of payment integration more smoothly and effectively. Happy coding!