Overcoming Common Challenges of Quarkus Remote Dev in Docker
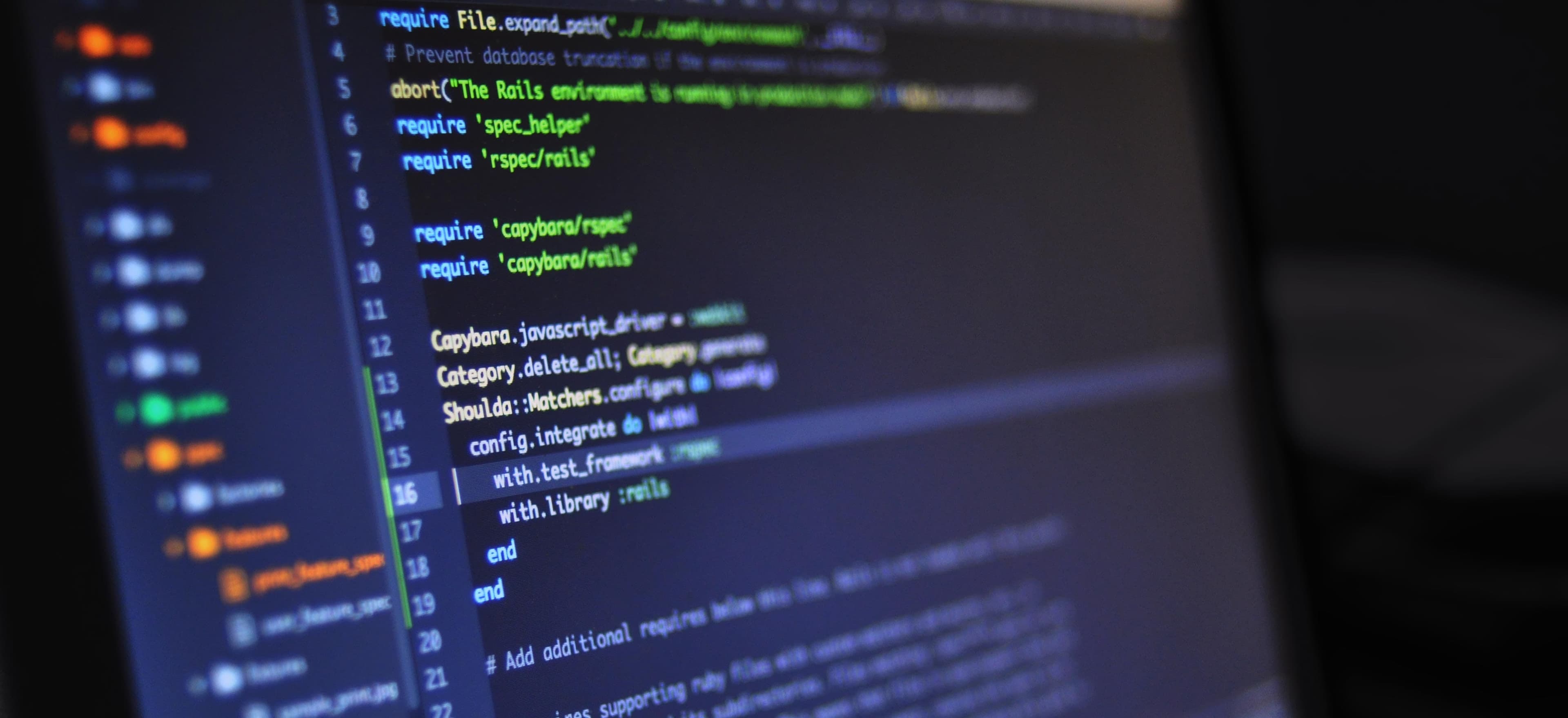
- Published on
Overcoming Common Challenges of Quarkus Remote Development in Docker
Quarkus is a powerful framework that empowers Java developers to create cloud-native applications. By integrating with Docker, Quarkus enables smooth remote development, but challenges can arise. In this blog post, we will delve into some common challenges faced during Quarkus remote development in Docker and how to overcome them.
Understanding Quarkus and Docker Integration
Before diving into the challenges, it's essential to understand how Quarkus and Docker work together. Quarkus optimizes Java applications for Kubernetes and provides out-of-the-box support for containerization. Docker, on the other hand, makes it easy to package applications and their dependencies in a single unit.
The synergy between the two allows developers to run their Java applications seamlessly in different environments. However, this process can sometimes invoke issues that need addressing.
Common Challenges and Solutions
1. Performance Issues with Remote Development
Problem
One of the most common challenges developers face when using Quarkus with Docker is performance. Remote development can lead to slow response times, especially when the code is being executed within a Docker container.
Solution
To combat performance issues, developers can streamline their Docker build processes and leverage Quarkus's dev mode effectively. Consider using the following command to run your application:
./mvnw quarkus:dev -Dquarkus.remote-dev
This command activates remote development mode, which enables hot-reloading. Changes made to your source files will automatically sync with the running application, reducing build times and improving feedback loops.
2. Network Configuration Problems
Problem
When running a Quarkus application in Docker, network configuration can pose substantial challenges. Containers need to communicate over the network, but sometimes they cannot find each other, leading to frustrating debugging sessions.
Solution
Make sure you set up the Docker network correctly. You can create a dedicated network and attach your containers to this network, which will facilitate communication. For example:
docker network create my-quarkus-network
Then, run your Docker container with the --network
option:
docker run --network my-quarkus-network -p 8080:8080 my-quarkus-app
Utilizing a dedicated network simplifies communication between your application and other services, resolving many connectivity issues.
3. Dependency Management
Problem
Dependency management can become complicated when dealing with Docker. Sometimes an updated package might conflict with others, or dependencies may not work correctly with the Quarkus version in use.
Solution
To solve dependency issues, ensure your pom.xml
is up to date. Also, utilize the Quarkus BOM (Bill of Materials), which conforms to a consistent versioning strategy:
<dependencyManagement>
<dependencies>
<dependency>
<groupId>io.quarkus</groupId>
<artifactId>quarkus-bom</artifactId>
<version>${quarkus.version}</version>
<type>pom</type>
<scope>import</scope>
</dependency>
</dependencies>
</dependencyManagement>
By doing this, you will keep your dependencies aligned with the Quarkus framework, ensuring compatibility and reducing potential conflicts.
4. Docker Image Size
Problem
Docker images can grow excessively large, making deployments slow and cumbersome. A bloated image can also lead to increased network transfer time.
Solution
To minimize Docker image size, you can take advantage of Quarkus’s native image feature if your use case allows it. Using a native GraalVM image significantly reduces the size of the Docker image.
You can create a native image with the following command:
./mvnw package -Pnative
Additionally, consider using multi-stage builds in your Dockerfile. This practice allows you to compile your application in one stage and copy only the necessary artifacts to the final image.
Example:
# Build stage
FROM registry.access.redhat.com/ubi8/openjdk-11 as build
COPY ./target/my-app-1.0.0-SNAPSHOT-runner /my-app
# Final image
FROM registry.access.redhat.com/ubi8/ubi-minimal
COPY --from=build /my-app /my-app
ENTRYPOINT ["/my-app"]
This approach minimizes the size of your final image while ensuring that all necessary components are included.
5. Debugging Complications
Problem
Debugging a remote Quarkus application running in Docker can be challenging due to the lack of direct familiarity with the environment.
Solution
Using remote debugging can provide a clear pathway to identify issues in your application. Quarkus supports remote debugging out of the box. To enable remote debugging, you must add JVM options in your Dockerfile:
ENV JAVA_TOOL_OPTIONS="-agentlib:jdwp=transport=dt_socket,server=y,suspend=n,address=*:5005"
Then, when running your Docker container, bind the debug port:
docker run -p 8080:8080 -p 5005:5005 my-quarkus-app
Now, you can connect your IDE to the specified debug port, allowing you to examine stack traces and evaluate variables live.
6. Managing Environment Variables
Problem
The configuration of environment variables in Docker can be a source of confusion, especially when trying to manage different settings for various environments (development, testing, production).
Solution
Use a .env
file to manage your environment variables effectively. Docker-compose works seamlessly with these files.
Example .env
file:
# .env file
DB_USER=myuser
DB_PASSWORD=mypassword
Then, in your docker-compose.yml
, you can refer to these variables:
version: '3'
services:
my-quarkus-app:
image: my-quarkus-app
env_file:
- .env
This approach keeps your sensitive data secure and maintains consistency across different environments.
Final Considerations
While there are common challenges associated with Quarkus remote development in Docker, implementing these strategies can significantly mitigate those issues. By optimizing performance, managing dependencies, and using Docker best practices, you can enhance your development experience.
Embracing these solutions not only streamlines your workflow but also enhances the overall reliability of your applications. To continue learning, consider referring to the official Quarkus Docker documentation and the Docker official documentation.
With the right tools and strategies at your disposal, Quarkus will not only simplify your development process but also empower your applications to perform at their best. Happy coding!