Maximizing ROI in Your Enterprise Testing Unit
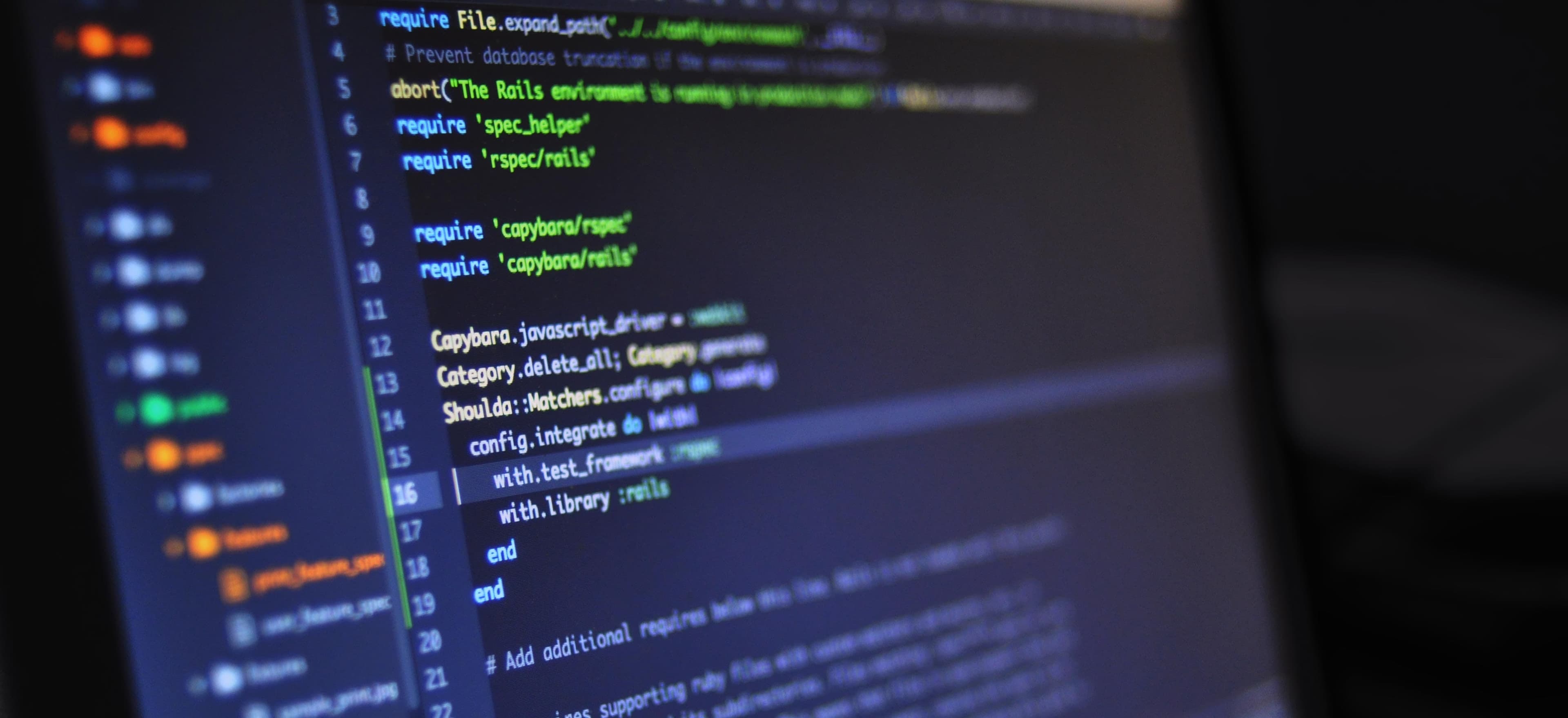
- Published on
Maximizing ROI in Your Enterprise Testing Unit
In today's fast-paced digital landscape, software quality is paramount. Enterprises invest heavily in testing units to ensure their applications run smoothly and efficiently. However, the challenge remains: how do we maximize the return on investment (ROI) from these testing units? In this post, we will explore various strategies and best practices that can help you achieve higher ROI from your enterprise testing efforts.
Understanding ROI in Testing
ROI in testing can be defined as the measure of the profitability of your testing investments relative to the benefits gained. This includes reduced defects, faster time to market, and improved customer satisfaction. To effectively maximize ROI, it’s crucial to understand what factors contribute to high-performing testing units.
Key Metrics in Testing ROI
-
Defect Density: This is the number of defects identified in a given size of the software (for example, per 1,000 lines of code). A lower defect density typically indicates better quality.
-
Test Coverage: This metric assesses the percentage of your code that is tested. Higher test coverage generally leads to better quality software.
-
Cost of Defects: The earlier a defect is found, the cheaper it is to fix. Tracking the cost associated with defects can highlight testing unit effectiveness.
-
Time to Market: The efficiency of your testing unit can significantly impact how quickly you can deliver products to market.
By tracking these metrics, you can assess whether your testing efforts are yielding a solid return.
Best Practices for Maximizing Test ROI
1. Implement Test Automation
Automation can drastically enhance the efficiency of your testing processes. Automated tests can run continuously and at scale, increasing test coverage and reducing manual testing effort.
// Sample Selenium WebDriver test case for testing a login functionality
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
public class LoginTest {
public static void main(String[] args) {
// Set the path for the WebDriver
System.setProperty("webdriver.chrome.driver", "path/to/chromedriver");
WebDriver driver = new ChromeDriver();
// Open the application
driver.get("http://example.com/login");
// Find username and password fields and send keys
driver.findElement(By.name("username")).sendKeys("myusername");
driver.findElement(By.name("password")).sendKeys("mypassword");
// Submit the form
driver.findElement(By.id("loginButton")).click();
// Check for success message
String message = driver.findElement(By.id("successMessage")).getText();
System.out.println(message); // Output the success message
// Close browser
driver.quit();
}
}
Why Automate? Automation increases accuracy and speeds up regression testing, which is crucial in agile environments. It allows you to run a suite of tests as often as necessary without the need for extensive manual resources.
2. Adopt Agile Testing Practices
Agile methodologies emphasize flexibility and responsiveness to change. By incorporating Agile Testing practices, you ensure that testing is integrated early in the development process.
- Implement cross-functional teams.
- Engage testers in sprint planning.
- Use continuous feedback to refine testing strategies.
3. Leverage Tooling and Technologies
Utilizing the right tools can immensely optimize your testing unit's efficiency. Consider tools for:
- Automated Testing: Selenium, TestNG, or JUnit for unit testing.
- Performance Testing: JMeter or Gatling.
- Continuous Integration: Tools like Jenkins can automate deployments and testing processes.
4. Increase Test Coverage Strategically
While 100% test coverage is ideal, it may not be practical. Focus on covering critical paths and high-risk areas of your application.
// Example of a JUnit unit test for a simple Calculator class
import static org.junit.Assert.assertEquals;
import org.junit.Test;
public class CalculatorTest {
@Test
public void testAdd() {
Calculator calculator = new Calculator();
assertEquals(5, calculator.add(2, 3)); // Test addition
}
@Test
public void testSubtract() {
Calculator calculator = new Calculator();
assertEquals(1, calculator.subtract(3, 2)); // Test subtraction
}
}
Why Focus on Coverage? Strategic test coverage ensures that high-impact areas are tested adequately, ultimately reducing the likelihood of critical failures in production.
5. Continuously Monitor and Evaluate
To maximize ROI, regular evaluation of your testing process is vital. Use feedback from both testers and business stakeholders to refine your testing strategies continually.
- Set up regular review meetings.
- Adjust test plans based on feedback.
The Bottom Line
Maximizing ROI in your enterprise testing unit is not merely about spending less; it’s about spending strategically. By employing automation, adopting agile practices, leveraging the right tools, increasing test coverage, and continuously evaluating your processes, you can significantly enhance the effectiveness of your testing unit.
For more insights on enhancing testing processes, consider checking out articles on Test Automation Best Practices and The Benefits of Agile Testing.
Empower your enterprise by optimizing your testing unit, and you will see positive results not just in ROI, but in improved software quality and customer satisfaction.
Happy Testing!
Checkout our other articles