Common Pitfalls in Apache Camel for Microservices
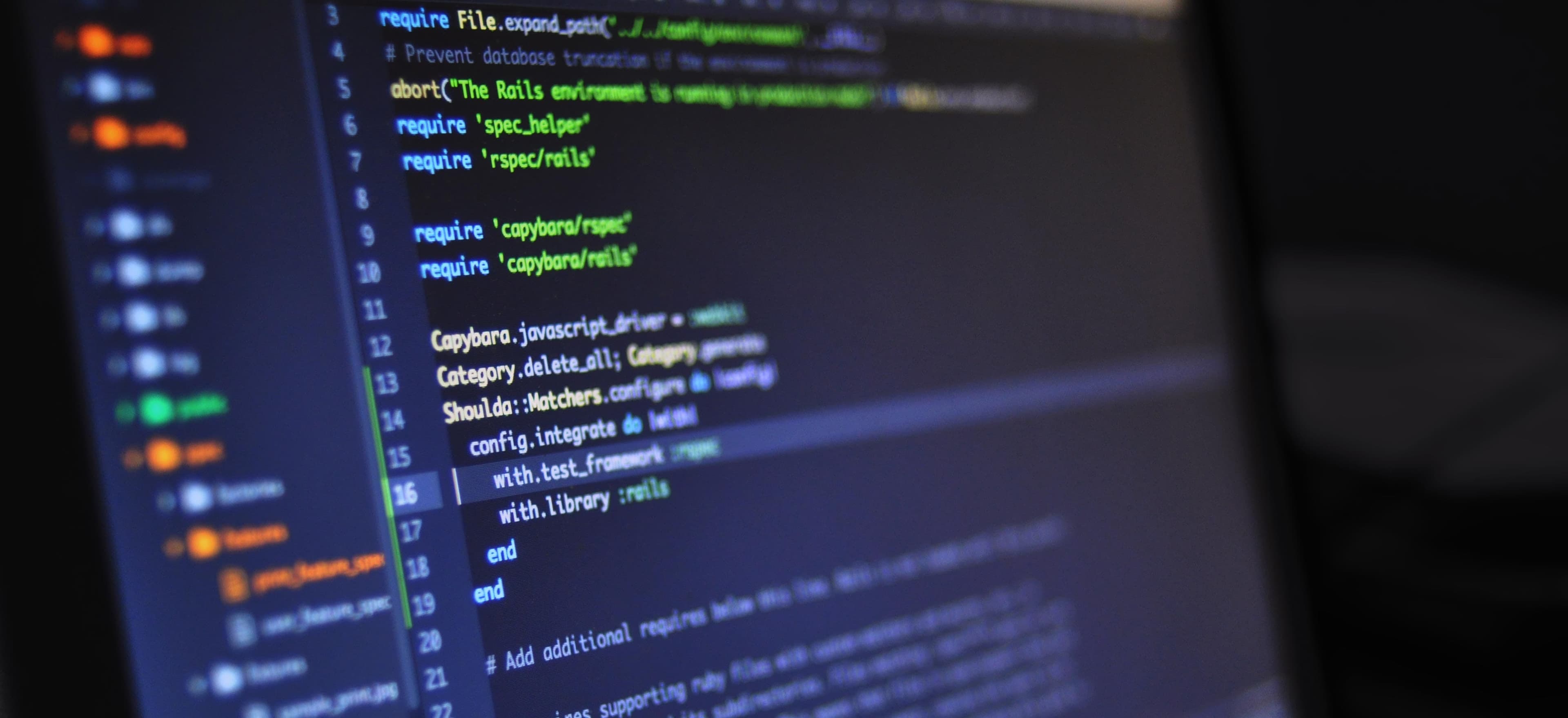
- Published on
Common Pitfalls in Apache Camel for Microservices
Apache Camel is a powerful integration framework that simplifies the process of integrating disparate systems, making it an ideal choice for building microservices. While developers often appreciate its versatility, there are some common pitfalls encountered during implementation. Understanding these pitfalls can ensure better design and implementation, leading to more efficient software architecture.
In this blog post, we will explore common pitfalls in Apache Camel for microservices, elucidating their implications and offering solutions.
1. Overusing Camel Components
The Issue
One of the most common pitfalls is overusing Apache Camel components without fully understanding their operational characteristics. Camel provides a vast variety of components like HTTP, JMS, and file components, among others. While it is tempting to utilize many components, this can lead to a complex system that becomes harder to maintain.
The Solution
The secret lies in selecting the right components that best suit your application needs. Choose a few components and create custom DSLs (Domain-Specific Languages) when necessary to avoid bloating your application with unnecessary complexity.
Example
from("direct:start")
.to("http://example.com/api");
In the above snippet, it’s crucial to evaluate if using a direct route to an external API is optimal or if you should design an internal service to handle such requests instead.
2. Ignoring Performance Implications
The Issue
Performance is often an afterthought in microservices architecture. Failing to consider how your Camel routes might handle load or scale can lead to bottlenecks. For example, the default configuration may suffice for development but can deteriorate in production scenarios.
The Solution
Always conduct performance testing early in the development cycle. Look at using Camel’s thread pool settings
, configuration options for route balancing
, and load testing tools like JMeter
.
Example
from("direct:start")
.threads()
.to("logger:myLogger");
By using threads explicitly, you can better manage how routes handle incoming requests, thus optimizing performance.
3. Improper Exception Management
The Issue
Exception handling in Apache Camel can be both a source of power and a potential pitfall. Many developers do not leverage the robust exception handling features provided by the framework, leading to unhandled exceptions and ultimately crashing the microservices.
The Solution
Utilize Camel's exception handling strategies effectively. Define error handlers to ensure that you gracefully handle exceptions, allowing for retries or redirections to alternative flows.
Example
onException(Exception.class)
.handled(true)
.log("Error occurred: ${exception.message}")
.to("mock:error");
With this code snippet, whenever an exception occurs, Camel will log the error and redirect it to an error funnel, making it easier to debug while keeping service operations running.
For detailed insights on error handling in Apache Camel, you can check Apache Camel Error Handling.
4. Misconfiguration of Routes
The Issue
Misconfigured routes in Apache Camel are a frequent cause of runtime errors. This can include incorrect URIs, missing components, or wrong options specified in routes. Such misconfigurations can lead to sluggish performance or, worse, service failures.
The Solution
Always validate and thoroughly test routes. Utilize Camel’s integration testing support to conduct tests on your routes before deploying them. This proactive approach will identify configuration issues early.
Example
from("timer:foo?period=1000")
.to("log:Bar?level=INFO");
The above code starts a timer that logs an informational message every second. If the timer's URI is incorrectly configured, the route may fail to start, causing interruptions in the service.
5. Not Utilizing Camel DSL Effectively
The Issue
Many developers are not fully leveraging Camel’s powerful DSL capabilities, sticking to the basic settings rather than developing the additional logic that Camel offers, such as EIP (Enterprise Integration Patterns).
The Solution
Familiarize yourself with the various DSL options available in Apache Camel, utilizing direct, fluent, or XML DSLs as per your needs. Leveraging DSL not only enhances readability and maintainability but also captures complex routing logic more succinctly.
Example
from("direct:input")
.choice()
.when(header("foo").isEqualTo("bar"))
.to("direct:bar")
.otherwise()
.to("direct:default");
In the example above, we've utilized a choice block to route messages based on certain header values, demonstrating conditional routing which is integral to many microservices architectures.
6. Overlooking Logging and Monitoring
The Issue
Logging and monitoring are often undervalued in the development phase, leading to significant issues in production environments. Understanding what occurs in system interactions can be critical for debugging and maintaining overall system health.
The Solution
Integrate robust logging and monitoring solutions from the start. Use Camel’s built-in logging capabilities alongside tools like Prometheus and Grafana for monitoring your microservices.
Example
from("direct:start")
.log("Received message: ${body}")
.to("http://example.com/api");
This log statement provides immediate visibility into what messages are being processed, invaluable for troubleshooting.
Key Takeaways
As you venture into the realm of microservices with Apache Camel, being aware of these common pitfalls can save time and resources. From route configuration to exception management, each aspect of implementation requires careful consideration. By following these guidelines and embracing the framework's best practices, you can ensure robust and resilient microservices.
For further information and resources, check out the Apache Camel official documentation to deepen your understanding and effectively leverage its capabilities.
By properly implementing Apache Camel, microservices development can be markedly more efficient, leading to an optimized end product that is easier to maintain and scale. Happy coding!