Common Pitfalls in Java Design Patterns You Should Avoid
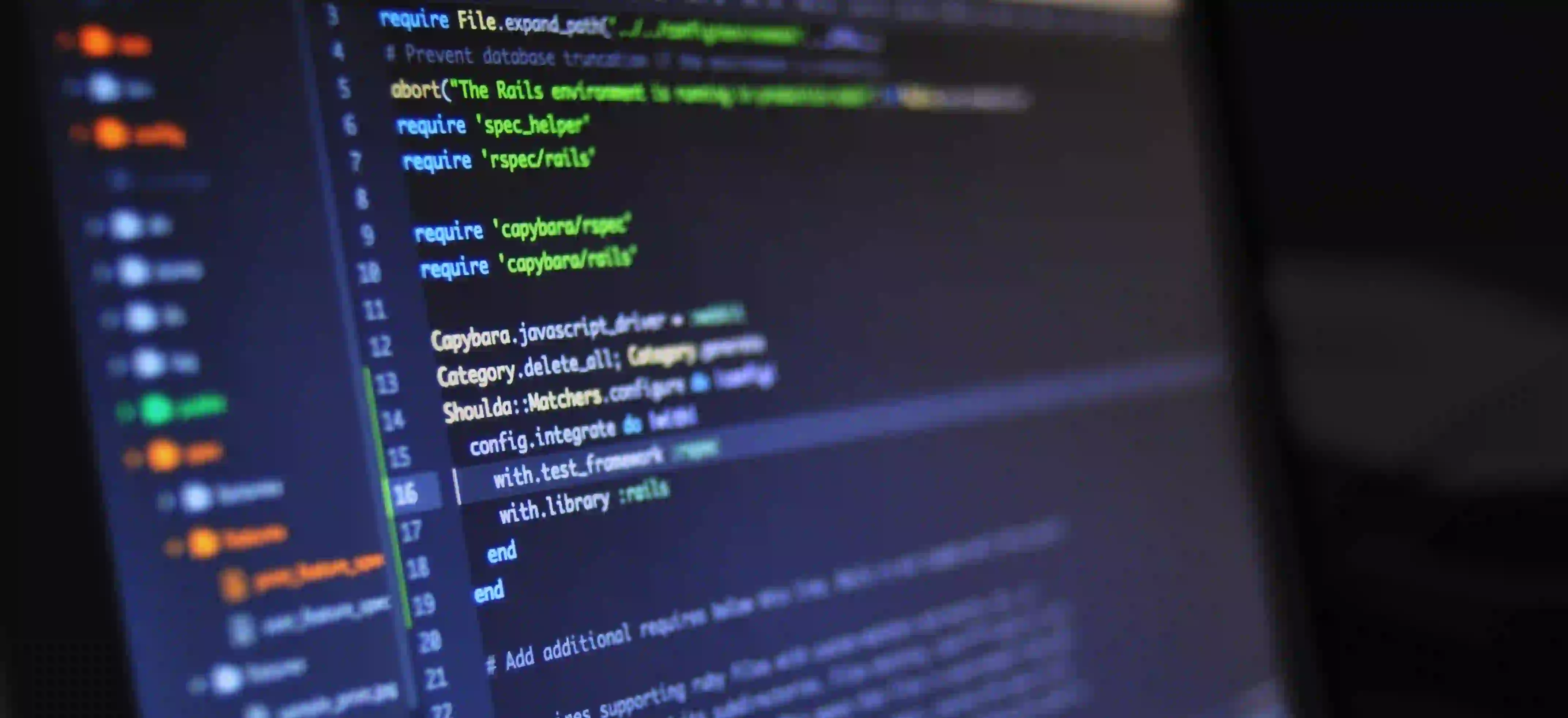
Common Pitfalls in Java Design Patterns You Should Avoid
Design patterns are proven solutions to recurring problems that many developers face. They provide a standardized way to tackle software design issues, optimize codebase maintenance, and improve collaboration within teams. However, using design patterns without a clear understanding can lead to challenges and excessive complexity within your code. In this blog post, we'll explore common pitfalls in Java design patterns that you should avoid.
What Are Design Patterns?
Before we delve into the pitfalls, let’s recap what design patterns are. They are categorized into three main types:
- Creational Patterns: Focus on object creation mechanisms.
- Structural Patterns: Deal with object composition.
- Behavioral Patterns: Focus on communication between objects.
For a deeper exploration, check out the GoF Design Patterns (Gang of Four).
1. Overusing Patterns
The Pitfall
One common mistake is applying design patterns unnecessarily. This can lead to code that is overly complex, making it difficult to read and maintain.
The Solution
Design patterns should be used when there’s a clear need. Sometimes a simple solution is more effective than layering multiple design patterns on top of each other.
// Bad Example - Unnecessary use of Builder Pattern
public class User {
private String name;
private String email;
private User(UserBuilder builder) {
this.name = builder.name;
this.email = builder.email;
}
public static class UserBuilder {
private String name;
private String email;
public UserBuilder setName(String name) {
this.name = name;
return this;
}
public UserBuilder setEmail(String email) {
this.email = email;
return this;
}
public User build() {
return new User(this);
}
}
}
// In this case, creating a User object could simply be done
User user = new User("John Doe", "john@example.com"); // Simpler Constructor
2. Ignoring the Context
The Pitfall
Design patterns are often context-sensitive, meaning their effectiveness depends on the scenario in which they are implemented. Ignoring this can result in a poor implementation.
The Solution
Before applying a design pattern, analyze the problem you are trying to solve. Are the benefits worth the overhead?
// Bad Example - Strategy Pattern Misuse
interface PaymentStrategy {
void pay(int amount);
}
class CreditCardPayment implements PaymentStrategy {
public void pay(int amount) {
// payment logic
}
}
class ShoppingCart {
private PaymentStrategy paymentStrategy;
public void setPaymentStrategy(PaymentStrategy paymentStrategy) {
this.paymentStrategy = paymentStrategy;
}
public void checkout(int amount) {
if (paymentStrategy == null) {
throw new IllegalArgumentException("Payment Strategy must be set");
}
paymentStrategy.pay(amount);
}
}
// Here, when the ShoppingCart does not need different payment strategies,
// direct payment processing can be simpler without the overhead of strategy pattern.
3. Poor Documentation and Comments
The Pitfall
Developers sometimes skip making proper documentation or commenting within code, especially when complex design patterns are involved. This can make the codebase less maintainable and harder for new team members to understand.
The Solution
Always document the purpose of each pattern choice clearly. Comments should explain the “why” behind the implementation, not just the “how.”
// Good Example - Using Observer Pattern
interface Observer {
void update();
}
class Subject {
private List<Observer> observers = new ArrayList<>();
public void registerObserver(Observer observer) {
observers.add(observer);
}
public void notifyObservers() {
for (Observer observer : observers) {
observer.update(); // Notify all registered observers
}
}
}
// Document:
// This Subject class is designed to keep track of observers
// that need updates. It's essential for implementing a publish-subscribe system.
4. Not Testing Your Patterns
The Pitfall
Failing to test when using design patterns can lead to bugs infiltrating your application. Patterns often introduce layers of abstraction, which can obscure issues.
The Solution
Testing your design patterns from multiple angles is essential to ensure reliability. Use unit tests and integration tests to validate interactions within your design.
// Testing the Singleton Pattern
public class Singleton {
private static Singleton instance;
private Singleton() {}
public static Singleton getInstance() {
if (instance == null) {
instance = new Singleton();
}
return instance;
}
}
// Test
public class SingletonTest {
@Test
public void testSingleton() {
Singleton instance1 = Singleton.getInstance();
Singleton instance2 = Singleton.getInstance();
// Assert that both instances are indeed the same
assertSame(instance1, instance2);
}
}
5. Not Adapting Patterns to Business Needs
The Pitfall
Design patterns are tools, not strict rules. Failing to adjust a pattern for specific business requirements could lead to inefficiencies or a subpar solution.
The Solution
Customize your design patterns according to the business logic and requirements. Modify patterns to create a hybrid that serves your needs better.
// A hybrid strategy
// Create a Context class that can dynamically choose the most fitting
// strategy based on business rules.
class Context {
private PaymentStrategy paymentStrategy;
public void setPaymentType(String type) {
if ("CreditCard".equals(type)) {
paymentStrategy = new CreditCardPayment();
} else if ("PayPal".equals(type)) {
paymentStrategy = new PayPalPayment();
}
}
public void pay(int amount) {
paymentStrategy.pay(amount); // Use the selected strategy
}
}
// Document:
// The Context class allows for dynamic selection of the payment method
// based on business rules rather than fixed payment types.
Final Considerations
Design patterns can significantly enhance your Java projects if used properly. Avoiding the pitfalls highlighted in this post is crucial to maintaining a clean, efficient, and adaptable codebase. Remember, patterns should simplify your coding efforts, not complicate them. Always consider the context of your development needs, adjust patterns to fit, and document your choices clearly.
If you're interested in further studying design patterns in Java, consider reading about Java Design Patterns from the official Oracle documentation.
By following these guidelines, you can harness the power of design patterns to create robust, scalable applications that meet both current and future business requirements. Happy coding!