Unlocking JMX with Spring: Common Configuration Pitfalls
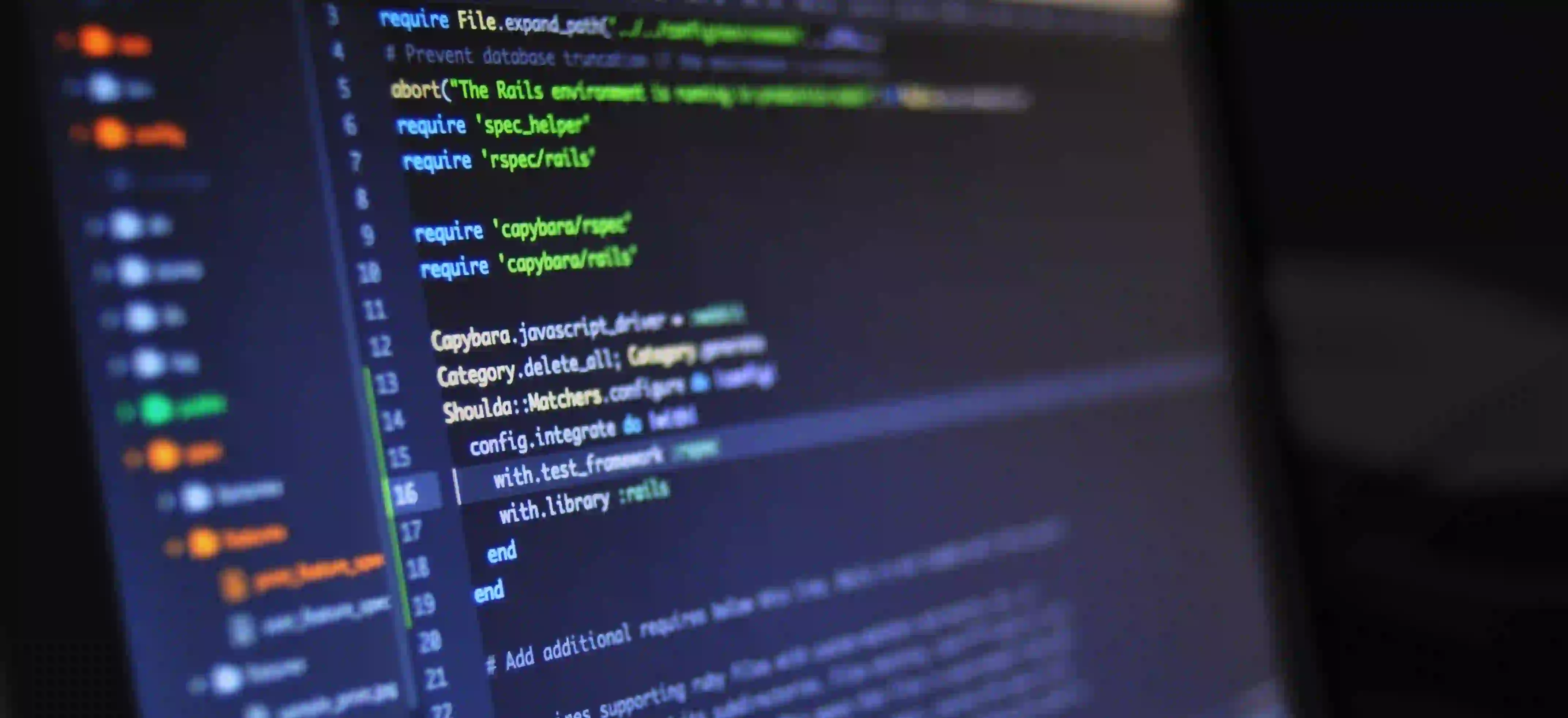
Unlocking JMX with Spring: Common Configuration Pitfalls
Java Management Extensions (JMX) is a powerful tool that allows developers to manage and monitor Java applications. When combined with the Spring framework, it offers a robust way to expose application metrics, control resources, and provide extensive insights into your app's performance. However, many developers encounter common pitfalls when configuring JMX in Spring applications. In this blog post, we will explore some of these pitfalls and provide actionable solutions to help you effectively unlock JMX in your Spring applications.
Understanding JMX Basics
Before diving into the pitfalls, let’s clarify what JMX is and why it’s essential.
JMX provides a way to manage Java resources through a set of objects called MBeans (Managed Beans). MBeans allow you to expose various metrics and operations of the application you may want to monitor, manage, or control.
Example of a Simple MBean
Here's a simple example of how to create an MBean in Java:
public interface HelloMBean {
void sayHello();
int getCacheSize();
}
public class Hello implements HelloMBean {
private int cacheSize;
public void sayHello() {
System.out.println("Hello, World!");
}
public int getCacheSize() {
return cacheSize;
}
public void setCacheSize(int size) {
this.cacheSize = size;
}
}
Why JMX?
Using JMX offers various benefits including:
- Real-time performance monitoring
- Easy interaction with the application to obtain metrics
- Ability to modify configurations dynamically
If you'd like to delve deeper into JMX, take a look at the Java JMX documentation.
Common Configuration Pitfalls
Now that we understand JMX, let’s explore some common pitfalls when setting it up with Spring.
1. Not Registering MBeans Properly
One common mistake is forgetting to register the MBeans with the MBeanServer. In a Spring application, it's essential to ensure that the MBeans are registered correctly during application startup.
Here’s how you can do this:
@Bean
public MBeanExporter mBeanExporter(Hello helloMBean) {
MBeanExporter exporter = new MBeanExporter();
exporter.setBeans(Collections.singletonMap("com.example:type=Hello", helloMBean));
return exporter;
}
Why This Matters
If MBeans are not registered correctly, you won’t be able to access them through JMX consoles or tools such as JConsole. Proper registration ensures your MBeans serve their purpose, exposing the necessary operations and attributes.
2. Misconfigured Object Names
Another frequent issue arises when the object names used for MBeans do not match what JMX clients expect. Object name conventions in JMX are strict.
For instance, the object name com.example:type=Hello
needs to follow the proper structure. It consists of domain and key-value pairs.
// Correct way to define object names
@Bean
public HelloMBean helloMBean() {
return new Hello();
}
@Bean
public MBeanExporter mBeanExporter() {
MBeanExporter exporter = new MBeanExporter();
exporter.setBeans(Collections.singletonMap("com.example:type=Hello", helloMBean()));
return exporter;
}
Why It’s Important
Using incorrect object names or failing to follow naming conventions could lead to mismatched expectations and make it hard to retrieve the desired MBeans.
3. Ignoring Security Considerations
When exposing JMX agents, neglecting security can be a major flaw. By default, JMX is not secure, potentially allowing unauthorized access to your beans.
To secure your JMX setup, consider the following configuration in your JVM options:
-Dcom.sun.management.jmxremote
-Dcom.sun.management.jmxremote.port=8080
-Dcom.sun.management.jmxremote.authenticate=true
-Dcom.sun.management.jmxremote.password.file=/path/to/jmxremote.password
-Dcom.sun.management.jmxremote.access.file=/path/to/jmxremote.access
Why Security Matters
Securing your JMX endpoint prevents unauthorized access to critical application metrics and configurations. A poorly secured JMX endpoint can expose sensitive data or allow malicious actors to manipulate your application.
4. Not Handling Exceptions Properly
Another pitfall developers encounter is not handling exceptions correctly when interacting with JMX. Operations performed on MBeans can throw various exceptions, and handling those poorly can lead to unexpected application behavior.
For example, in the MBean methods, make sure you encapsulate the logic in try-catch blocks.
public void sayHello() {
try {
System.out.println("Hello, World!");
} catch (Exception e) {
// Handle exception appropriately
System.err.println("An error occurred: " + e.getMessage());
}
}
Why Exception Handling Is Crucial
Proper exception handling within your MBeans ensures that one broken operation doesn't disrupt the entire monitoring and management experience. It also provides a more graceful failure mechanism.
5. Not Testing Your Configuration
Failing to test your JMX configuration can lead to surprises in production environments. Always test your MBean access using tools like JConsole or VisualVM to ensure the MBeans are accessible and behaving as expected.
Why Testing Is Key
Testing helps you spot issues early, ensuring that your JD does not lead to unmonitored application behavior. It also provides you with an opportunity to verify that your security settings are functioning as intended.
The Closing Argument
Configuring JMX in a Spring application can be straightforward if you avoid the common pitfalls we’ve discussed. By ensuring proper MBean registration, using the correct object names, securing your setup, handling exceptions, and thoroughly testing your configuration, you can unlock the full potential of JMX.
Remember, JMX is an invaluable resource for real-time insights into your application performance, so make sure to leverage it properly. For further reading on JMX and Spring integration, consider looking into the Spring documentation on Spring and JMX.
By implementing these practices, you will create a robust monitoring solution that enhances your application's performance and reliability. Happy coding!