Overcoming Communication Barriers in Pair Programming
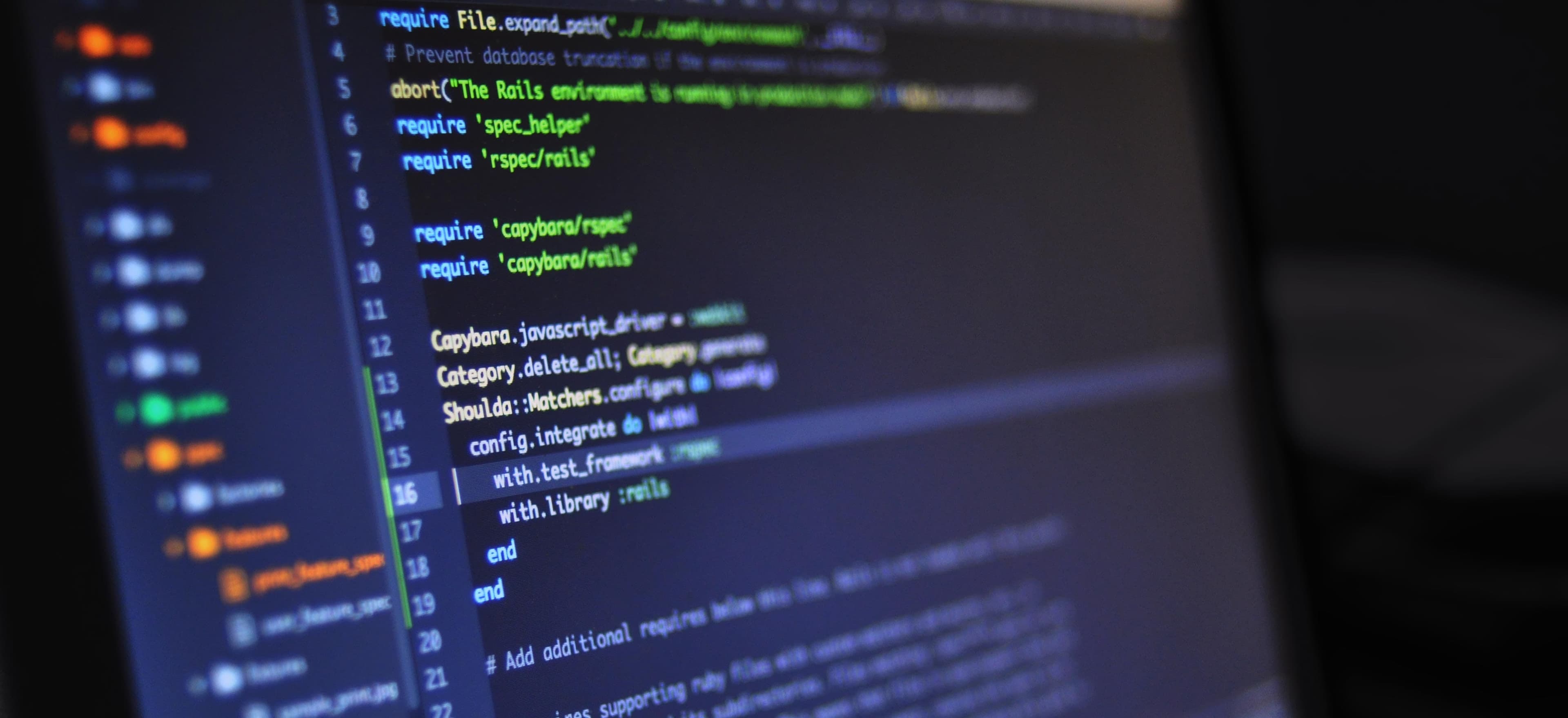
- Published on
Overcoming Communication Barriers in Pair Programming
Pair programming is a central practice in agile software development that emphasizes collaboration and shared knowledge. It involves two developers working together at one computer: one writing code (the "driver") and the other reviewing it (the "observer"). While this method can enhance productivity and improve code quality, it can also lead to communication barriers. In this article, we will discuss effective techniques to overcome these barriers, facilitating a smoother pair programming experience.
Understanding the Importance of Communication in Pair Programming
Effective communication is the backbone of successful pair programming. It encourages knowledge sharing, reduces errors, and improves overall productivity. When team members communicate well, they can:
- Quickly identify and fix issues.
- Collaborate effectively on problem-solving.
- Learn from each other, which can lead to skill development and team growth.
However, several factors can create communication barriers, including individual communication styles, cultural differences, and environmental factors. Recognizing and addressing these barriers is essential for making pair programming a productive endeavor.
Common Communication Barriers
-
Different Communication Styles: Every individual has a unique style of communication, which can lead to misunderstandings. Some may prefer direct feedback while others may be more subtle in their approach.
-
Cultural Differences: If a team consists of members from various cultural backgrounds, differences in norms and values can affect communication. This can result in misinterpretations or assumptions that hinder collaboration.
-
Environmental Factors: The physical workspace can also play a critical role. Noise levels, seating arrangements, and even computer setup can impact focus and the ability to communicate effectively.
Example: Communication Styles in Action
Consider two developers, Alice and Bob. Alice is straightforward and appreciates immediate feedback, while Bob is more reserved and prefers to consider feedback before responding. In a pair programming situation, Alice may feel frustrated if Bob does not respond quickly, while Bob might feel overwhelmed by Alice's rapid-fire questions.
Overcoming Different Communication Styles
To address these differences, it's crucial for team members to establish a mutual understanding of each other's preferences from the outset. Use the following strategies:
-
Set Guidelines for Communication: At the start of a project, discuss preferred communication styles. Ask team members what methods help them feel comfortable sharing their thoughts.
-
Adjust to Your Partner's Style: Similar to the Observer-Driver model, adjust your communication frequency and style to suit your partner's preferences.
Navigating Cultural Differences
Cultural sensitivity is vital in any team setting. Here are some ways to foster an inclusive atmosphere:
-
Promote Open Discussions: Encourage team members to share their cultural backgrounds and communication preferences. This practice not only fosters understanding but also allows everyone to feel valued.
-
Be Mindful of Language Nuances: Avoid idioms or jargon that may not translate well across cultures. Instead, opt for simple language that conveys your point clearly.
Example: Cultural Sensitivity in Practice
Imagine a pair of programmers, Jiro from Japan and Megan from the United States. Jiro may be accustomed to a more indirect way of communication, while Megan prefers direct feedback. It's important for Megan to understand Jiro's preference and vice versa.
Environmental Considerations
A conducive work environment can significantly enhance communication during pair programming sessions. Consider the following:
-
Optimize the Workspace: Ensure that the workspace is free from distractions. This could mean a quiet room or using noise-canceling headphones.
-
Utilize Technology Wisely: When working remotely, tools like Zoom, Slack, or Microsoft Teams can help close the communication gap. Familiarize yourself with these tools and choose the ones that best suit your team's needs.
Strategies for Effective Communication
1. Establish a Feedback Loop
Feedback is essential for continuous improvement. Establish a feedback loop early on:
-
Regular Check-ins: Schedule brief check-ins where team members can discuss any issues openly.
-
Constructive Criticism: Frame feedback in a way that's helpful and encourages growth. Focus on the code, not the coder.
Code Snippet: Implementing a Feedback Mechanism
Here's a simple way to implement a feedback loop in your code review practices using Java. Consider the following example where we rate code quality.
import java.util.HashMap;
import java.util.Map;
public class FeedbackMechanism {
private Map<String, Integer> feedbackScores;
// Constructor to initialize the feedback scores
public FeedbackMechanism() {
feedbackScores = new HashMap<>();
}
// Function to add feedback score
public void addFeedback(String feedbackType, int score) {
feedbackScores.put(feedbackType, score);
}
// Print feedback summary
public void printFeedback() {
System.out.println("Feedback Summary:");
for (Map.Entry<String, Integer> entry : feedbackScores.entrySet()) {
System.out.println(entry.getKey() + ": " + entry.getValue());
}
}
public static void main(String[] args) {
FeedbackMechanism feedback = new FeedbackMechanism();
feedback.addFeedback("Code Readability", 8);
feedback.addFeedback("Bug Prevention", 9);
feedback.printFeedback();
}
}
Why This Code Matters: This code snippet allows team members to rate various aspects of their pair programming sessions. By having a structured way of collecting feedback, you can foster a culture of openness and improvement.
2. Documentation and Shared Resources
Documentation plays a vital role in bridging communication gaps. Ensure that:
-
Code Comments: Write clear comments in code to explain complex logic. This helps both programmers understand the reasoning behind certain decisions.
-
Shared Documentation: Use tools like Confluence or Google Docs for storing meeting notes, coding standards, and other important information.
3. Develop Trust and Rapport
Trust is foundational for effective communication. To develop trust:
-
Be Respectful and Supportive: Encourage a culture where individuals feel secure in sharing their thoughts without judgment.
-
Engage in Team-Building Activities: Participate in team-building exercises that help foster relationships among team members.
Example: Building Rapport Through Team Activities
Consider setting aside regular team-building sessions. This could be coding challenges, social outings, or even virtual game hours. These activities help members understand each other better and lay the groundwork for effective communication.
Additional Resources
For further reading on effective pair programming strategies, consider checking out the Agile Alliance website. It's a valuable resource filled with insights on agile methodologies.
Another excellent resource is the book "Extreme Programming Explained" by Kent Beck, which delves deeper into the practices that can optimize pair programming. You can find this book on Amazon.
Bringing It All Together
Overcoming communication barriers in pair programming is essential for achieving effective collaboration and enhancing productivity. By being mindful of communication styles, cultural nuances, and environmental factors, teams can build a solid foundation for success. Implementing structured feedback, maintaining documentation, and developing trust among team members will only bolster this foundation.
By applying these strategies, you can transform pair programming into a powerful tool for software development, fostering a collaborative environment where creativity and innovation thrive. So, embrace the opportunity to learn and grow through this collaborative process, and watch your team's performance reach new heights.