Mastering Particle Effects in Android Game Development
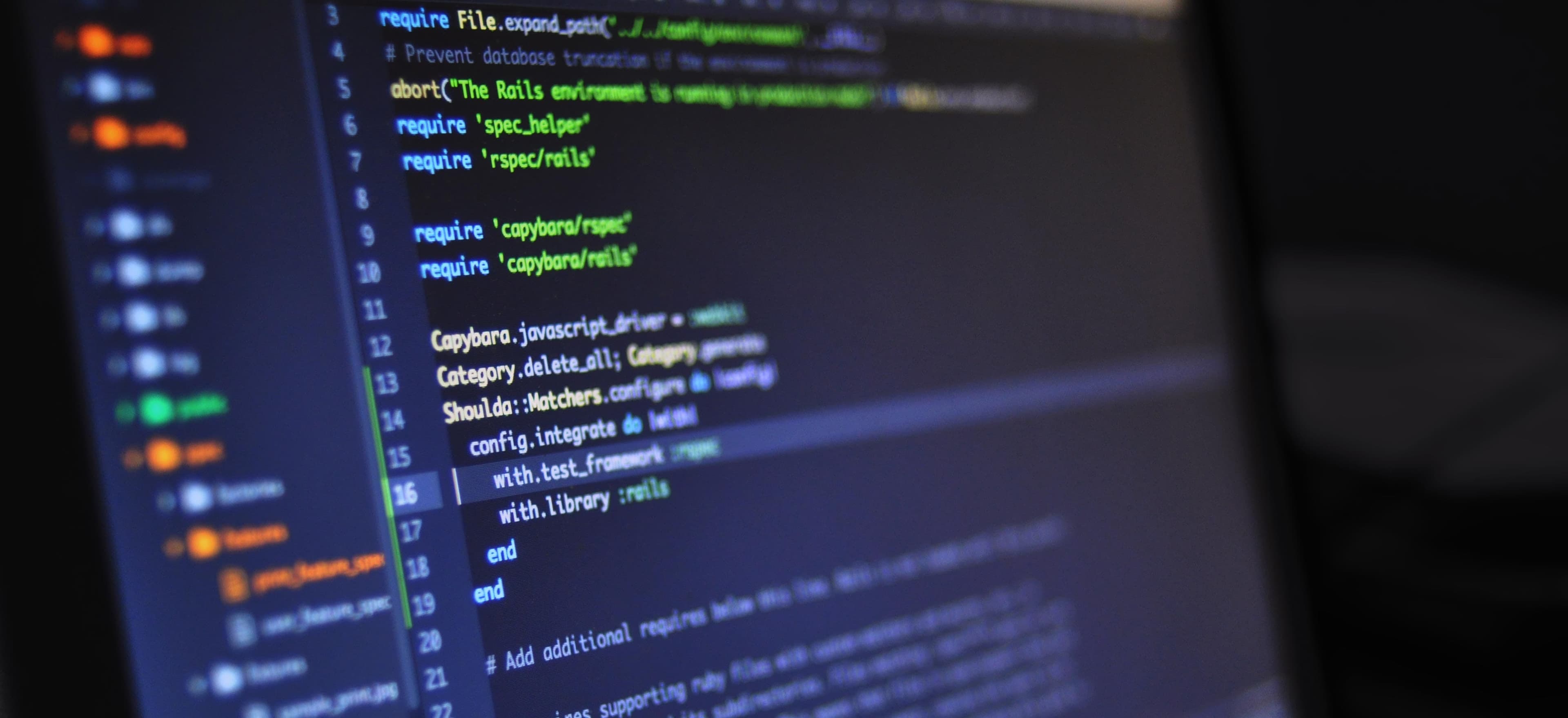
- Published on
Mastering Particle Effects in Android Game Development
Creating visually appealing games requires an understanding of various elements, one of which is particle effects. Particle systems can add realism and depth to your game, enhancing the player experience. This blog post will delve into the intricacies of implementing particle effects in Android game development using Java.
What are Particle Effects?
Before we dive into coding, let's clarify what particle effects are. Particle effects are techniques used to simulate fuzzy phenomena, which can include effects such as fire, smoke, rain, and explosions. They are not only visually stunning but can help in rendering complex systems like weather or magic systems dynamically.
Why Use Particle Effects?
- Visual Appeal: Enhances the graphics of the game, making it more immersive.
- Realism: Adds a layer of realism by simulating natural phenomena.
- Performance: Efficiently simulates complex effects without heavy performance costs.
Setting Up Your Android Game Development Environment
To get started with particle effects, ensure your environment is properly set up for game development. You can use LibGDX, a popular framework for developing Android games. It allows for robust graphics and is structured to take full advantage of Java.
Prerequisites
- Install JDK: Ensure you have the Java Development Kit (JDK) installed.
- Setup LibGDX: Follow the LibGDX setup guide.
Creating a Basic Particle System
Now, let's create a simple particle effect using LibGDX. The first step is to create a new project and set up the necessary dependencies.
Dependency Setup
In your build.gradle
file, make sure to include the following dependencies:
dependencies {
implementation "com.badlogicgames.gdx:gdx:1.9.10"
implementation "com.badlogicgames.gdx:gdx-box2d:1.9.10"
implementation "com.badlogicgames.gdx:gdx-box2d-platform:1.9.10:natives-windows"
implementation "com.badlogicgames.gdx:gdx-platform:1.9.10:natives-windows"
}
Make sure to replace version numbers with the latest ones from the LibGDX repository.
Particle Effect Implementation
Now let's delve into the code. Here, we’ll create a basic particle effect that simulates sparkling particles.
Code Snippet: Creating a Particle Effect
import com.badlogic.gdx.ApplicationAdapter;
import com.badlogic.gdx.Gdx;
import com.badlogic.gdx.graphics.GL20;
import com.badlogic.gdx.graphics.g2d.ParticleEffect;
public class ParticleDemo extends ApplicationAdapter {
private ParticleEffect particleEffect;
@Override
public void create() {
particleEffect = new ParticleEffect();
particleEffect.load(Gdx.files.internal("spark.p"), Gdx.files.internal("images"));
particleEffect.setPosition(400, 300);
}
@Override
public void render() {
Gdx.gl.glClearColor(0, 0, 0, 1);
Gdx.gl.glClear(GL20.GL_COLOR_BUFFER_BIT);
// Update and draw the particle effect
particleEffect.update(Gdx.graphics.getDeltaTime());
particleEffect.draw(Gdx.graphics.getBatch());
}
@Override
public void dispose() {
particleEffect.dispose();
}
}
Explanation of the Code
- Import Statements: Ensure you're importing the required libraries from LibGDX.
- ParticleEffect Initialization: The
ParticleEffect
object is created, where we load the particle effect configuration file (in.p
format). - Setting Position: The position of the particle effect is set using
setPosition
. - Render Method: Inside the render method, we clear the screen for every frame and then update and draw our particle effect.
Why Load a Particle Effect from a File?
Loading a particle effect from a file allows for greater flexibility and reusability. It means you can easily tweak the properties of the effect without changing the core code, which is essential for prototyping and adjusting game dynamics efficiently.
Creating the Particle Effect File
To create the particle effect file, you can use the Particle Editor. This tool lets you design your own particle effects visually and save them as .p
files.
Particle Effect Characteristics
When creating particle effects, pay attention to the following characteristics:
- Lifetime: How long each particle lives before disappearing.
- Initial Velocity: Speed of each particle when emitted.
- Gravity: Applies downward force, simulating gravity effects.
- Color: Gradients can be applied to create effects like fire or smoke.
Example of Fine-Tuning Particle Effects
Here’s an example of adjusting the gravity and velocity to simulate a more interesting effect.
// Set gravity to make particles fall down
particleEffect.getEmitters().first().setGravity(0, -200);
// Set initial velocity
particleEffect.getEmitters().first().setVelocity(100, 200);
Why Fine-Tune Particle Effects?
Fine-tuning particle effects allows creators to adjust the game feel and atmosphere. For example, in a fantasy game, you might want your magical sparkles to float gently, while in an action game, explosions need to pack a punch.
Adding Particle Effects to Entities
In many cases, you may want to associate particle effects with specific game actions, such as hitting an enemy or shooting a projectile. Below is a brief demonstration of how to do this.
Code Snippet: Associating Particle Effects with Actions
public class Player {
private ParticleEffect impactEffect;
public Player() {
impactEffect = new ParticleEffect();
impactEffect.load(Gdx.files.internal("impact.p"), Gdx.files.internal("images"));
}
public void onHit() {
impactEffect.setPosition(this.x, this.y);
impactEffect.start();
}
public void update() {
impactEffect.update(Gdx.graphics.getDeltaTime());
}
public void draw(SpriteBatch batch) {
impactEffect.draw(batch);
}
}
Explanation of Action Effects
- Impact Effect Initialization: Load the effect specific to the hit action.
- onHit Method: This method triggers the particle effect at the player’s current location.
- update Method: Updates the effect each frame.
- draw Method: Draws the effect on the screen.
Why Use Particle Effects for Actions?
Associating particle effects with actions enhances player feedback. When an action occurs—like a hit—it provides a visual confirmation to the player, enriching the gameplay experience.
To Wrap Things Up
Mastering particle effects is a significant step in creating immersive experiences in Android game development. Through this post, you've learned to set up a basic particle system using LibGDX, create effects, and associate them with gameplay actions.
By creatively utilizing particle effects, you can bring your games to life, capturing your audience’s attention. So, go ahead and experiment with different effects, styles, and implementations to make your game unique.
Further Resources
By understanding and mastering these techniques, you can elevate your game development skills and create captivating experiences for players. Happy coding!
Checkout our other articles