Overcoming Eager Evaluation in Neo4j Cypher Queries
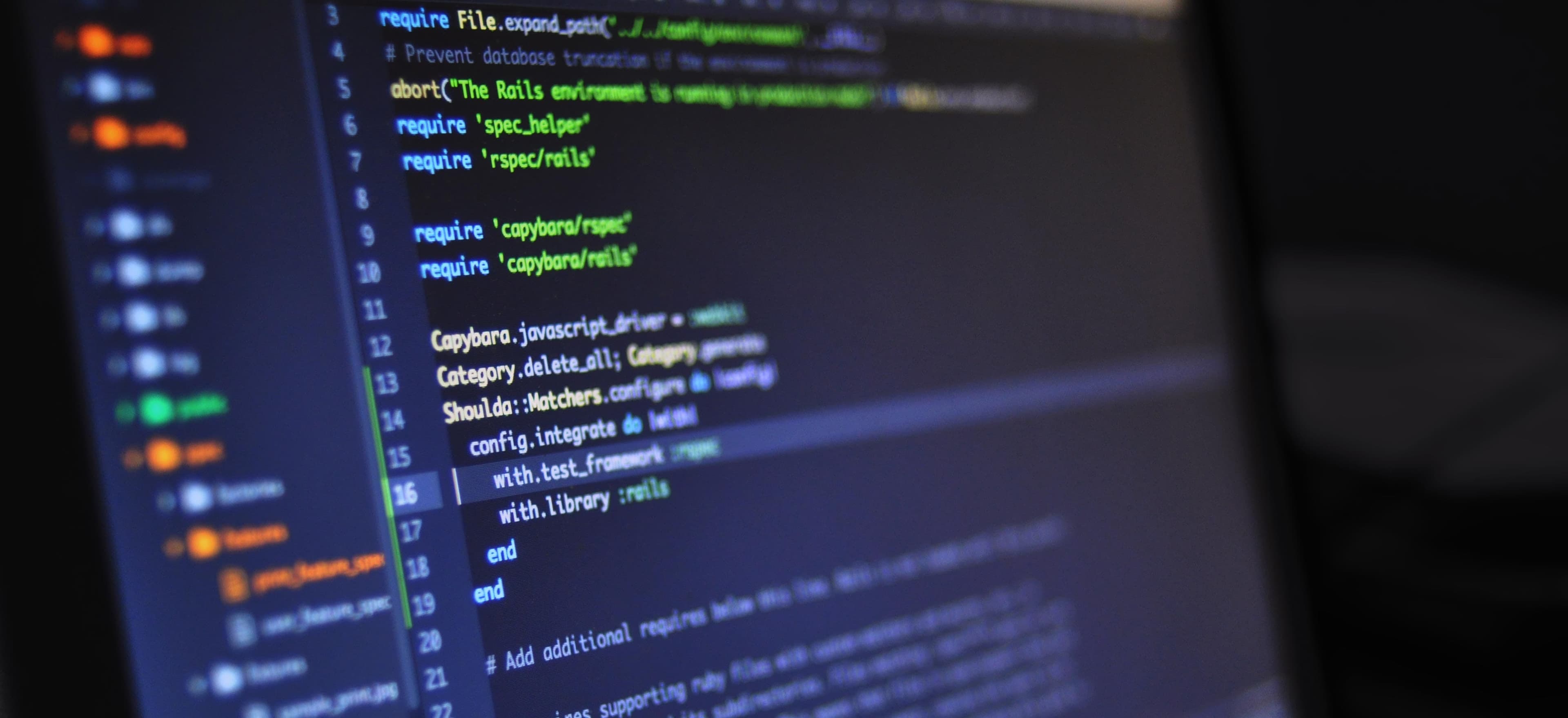
- Published on
Overcoming Eager Evaluation in Neo4j Cypher Queries
Neo4j is a powerful graph database that allows for the storage, retrieval, and manipulation of complex relationships. One of the most popular ways to interact with Neo4j is through Cypher, its declarative query language. However, one common challenge developers face is eager evaluation, which can lead to performance issues when querying large datasets. In this blog post, we'll explore what eager evaluation is, how it impacts your Cypher queries, and strategies to mitigate it.
Understanding Eager Evaluation
Eager evaluation means that a system computes all the values upfront instead of deferring computation until absolutely necessary. In Neo4j, this can occur when Cypher queries fetch data that is not subsequently needed or relevant for the returned results. This can lead to excessive memory consumption and slow performance, especially in large graphs.
Example of Eager Evaluation
Consider the following Cypher query:
MATCH (p:Person)-[:FRIENDS_WITH]->(f:Person)
RETURN p.name, f.name
In this query, we are looking for all people and their friends. However, if Person
nodes are linked to numerous other nodes that are not needed in the final result, Neo4j will compute all of those connections upfront, which can be inefficient.
The Implications of Eager Evaluation
-
Performance Degradation: When the database has to process and evaluate unnecessary data, it drains system resources, slows down the query, and can lead to higher latency.
-
Memory Issues: Large datasets can surpass memory limits when all related nodes and relationships are loaded into memory, causing the backend to crash or run inefficiently.
-
Increased Complexity: Maintaining complex queries, particularly when eager evaluation is at play, can lead to debugging challenges and code that is harder to read and maintain.
Dealing with Eager Evaluation
To effectively overcome eager evaluation in Cypher queries, we can adopt several strategies that enhance query performance without sacrificing the accuracy of results.
1. Use Optional Matches Wisely
The OPTIONAL MATCH
clause can be beneficial. Instead of loading all connections, we can conditionally load only the nodes we need.
Example:
MATCH (p:Person)
OPTIONAL MATCH (p)-[:FRIENDS_WITH]->(f:Person)
WHERE f.age < 30
RETURN p.name, f.name
In this query, we are only interested in friends who are under 30. By introducing conditions, we significantly reduce the number of nodes that Neo4j needs to evaluate.
2. Filter Early and Often
Using WHERE
clauses effectively can limit the amount of data that needs to be evaluated. Evaluate conditions as early in the query as possible to minimize the dataset.
Example:
MATCH (p:Person)
WHERE p.active = true
RETURN p.name
Here, we're filtering out inactive persons before processing data related to their relationships. This can drastically improve performance in a large dataset.
3. Reduce Returned Data
Instead of pulling back all properties from nodes, specify only what is needed. This reduces the load on the database and the amount of data transferred over the network.
Example:
MATCH (p:Person)-[:FRIENDS_WITH]->(f:Person)
RETURN p.name, f.name, f.age
Instead of returning all properties of the Person
nodes, we only return the name and age properties.
4. Use WITH
Clauses to Break Down Large Queries
Using WITH
clauses can help break a complex query into manageable parts, allowing Neo4j to process data in stages.
Example:
MATCH (p:Person)
WHERE p.city = 'New York'
WITH p
MATCH (p)-[:FRIENDS_WITH]->(f:Person)
RETURN p.name, f.name
By splitting queries, we reduce the evaluation scope of each step, optimizing performance.
5. Profiling Your Queries
Using the PROFILE
keyword before your query can provide insights into how Neo4j is executing it. Analyzing the query profile can reveal potential inefficiencies caused by eager evaluation.
PROFILE MATCH (p:Person)
RETURN p.name
The output will showcase how many nodes were accessed and how much time was spent on each part of the query.
The Bottom Line
Eager evaluation in Neo4j Cypher queries can be a significant barrier to performance, particularly as datasets grow in complexity and size. However, by employing strategies such as filtering early, using optional matches judiciously, reducing returned data, leveraging WITH
clauses, and profiling queries, you can mitigate its impact effectively.
To master your Cypher queries and enhance the performance of your Neo4j database, consider exploring more about Cypher query optimization and the nuances of graph databases. The key is balancing the depth of data retrieval with the efficiency of query execution, ensuring that your application runs optimally.
By following the tips laid out in this post, you can build more efficient, performance-oriented Cypher queries that minimize the challenges posed by eager evaluation, leading to faster data insights and a more responsive application overall. Happy querying!
Checkout our other articles