Why Java's Legacy Struggles to Keep Up Today
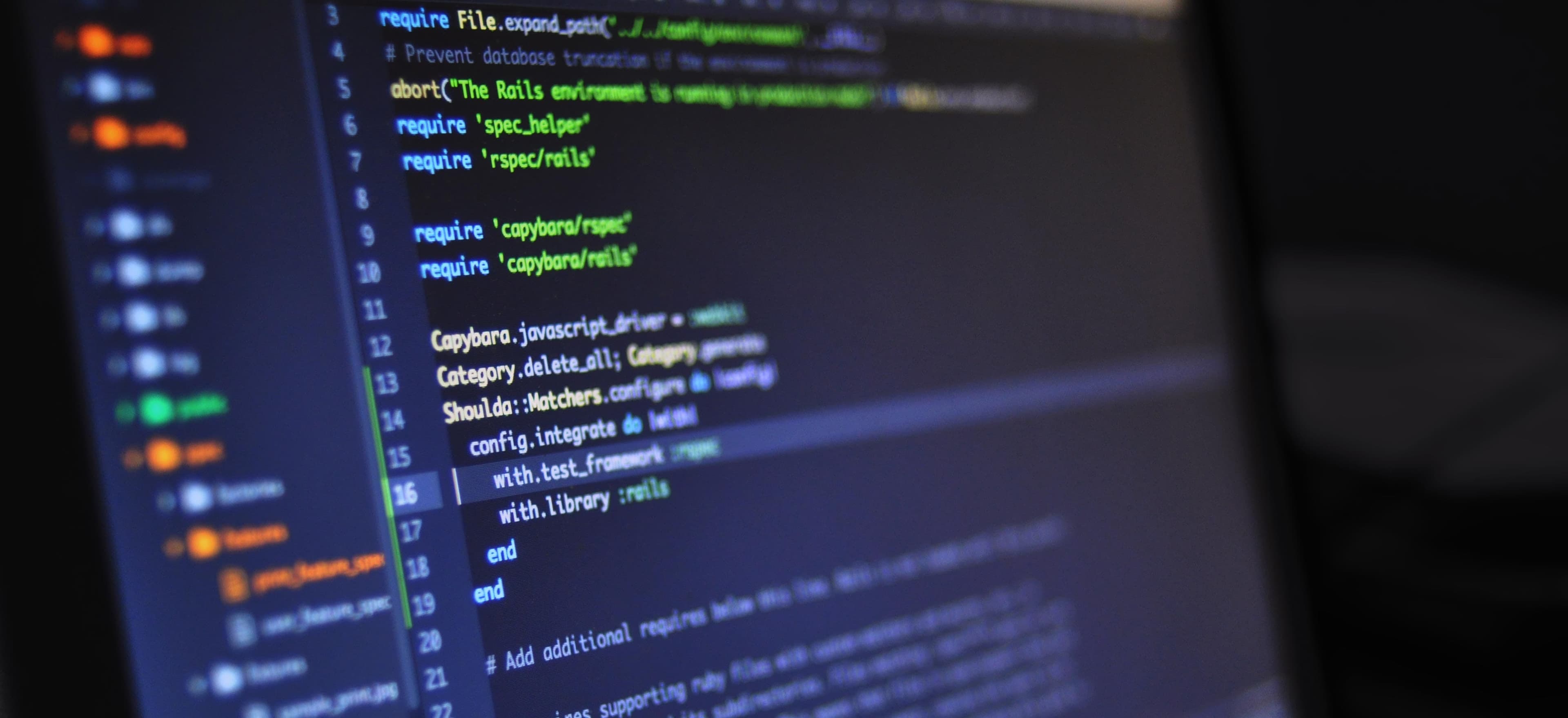
- Published on
Why Java's Legacy Struggles to Keep Up Today
Java is one of the most widely used programming languages, cherished for its portability, robustness, and extensive libraries. However, as technology rapidly evolves, Java faces challenges that make its legacy seem dated. This blog post explores why Java's legacy struggles to keep up with modern demands, while also considering its strengths and how it can adapt.
The Rise of New Programming Paradigms
Object-Oriented Programming vs. Functional Programming
Java primarily follows an object-oriented programming (OOP) paradigm. While OOP remains popular, there's been a significant shift towards functional programming (FP), especially with the rise of languages such as Scala and Kotlin.
// A classic approach in Java using OOP
class Calculator {
public int add(int a, int b) {
return a + b;
}
}
Here, we define a Calculator class with an add method. However, this doesn't showcase the conciseness offered by functional programming.
// Using Java's streams for a functional approach
import java.util.stream.Stream;
public class FunctionalExample {
public static void main(String[] args) {
int sum = Stream.of(1, 2, 3, 4, 5)
.reduce(0, Integer::sum);
System.out.println("Sum: " + sum);
}
}
In this example, Java features like streams allow a more declarative style. This shift towards functional paradigms offers developers better readability and maintainability, leading to the reduction of boilerplate code.
A Cluttered Language
Java is often criticized for its verbosity and boilerplate code requirements. Every class, for example, needs a full structure, leading to lengthy codebases that can be overwhelming.
Consider the following simple data class:
public class User {
private String name;
private int age;
public User(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
}
In contrast, Kotlin allows for more concise data class definitions:
data class User(val name: String, val age: Int)
The Kotlin version provides the same functionality with much less code. The competition forces Java to continuously evolve, but many developers are still drawn to the less verbose, more streamlined options.
Framework Saturation and Legacy Technologies
The Emergence of Alternatives
Java established a solid footing in the enterprise market with Spring and Hibernate. However, with the arrival of lightweight frameworks like Node.js, Django, and Ruby on Rails, many developers have turned away from Java.
These frameworks are often simpler to set up, providing quicker turnaround times for MVPs (Minimum Viable Products). Businesses seeking rapid development cycles are increasingly considering alternatives, which poses a challenge to Java’s dominance.
Kubernetes and Microservices
The burgeoning trend around microservices has also affected Java. While Java has been a staple in enterprise applications, the microservices architecture favors lightweight, non-blocking systems. Many Java applications are traditionally built using the server-centric model:
@RestController
public class HelloWorldController {
@RequestMapping("/hello")
public String hello() {
return "Hello World!";
}
}
While this is perfectly valid, languages like Go and Node.js offer asynchronous capabilities and better performance in resource-constrained environments:
const express = require('express');
const app = express();
app.get('/hello', (req, res) => {
res.send('Hello World!');
});
This non-blocking model allows services to handle more requests concurrently, a necessity in today’s cloud-based environment.
Performance and Scalability Concerns
Garbage Collection and Memory Management
Garbage collection in Java is robust yet adds overhead. For high-performance applications, this can lead to unpredictable pauses, which can affect processing speed—especially when compared to languages with more granular control over memory management like C++.
For instance, a real-time trading application might require ultra-low latency, making languages like C++ the better choice:
// A simple allocation in C++
int* arr = new int[100];
The explicit memory control in C++ is advantageous in situations where performance is critical and allows developers to optimize their applications more effectively than Java.
Optimization Challenges
Java applications can suffer from slower startup times and higher memory usage in comparison to their counterparts. Consider the startup time for a Java application:
java -jar myapplication.jar
In contrast, languages like Go compile to binaries, resulting in faster execution times and reduced resource footprint:
go run myapplication.go
Modern developers prioritize efficiency, particularly in cloud-native environments.
The Ecosystem and Community
Fragmentation and Compatibility
Java’s ecosystem suffers from fragmentation. With numerous versions (like Java 8 vs. Java 17), it can be challenging to maintain backward compatibility, posing risks to long-term support for applications built on older frameworks.
While tools like Java's Maven or Gradle work well, newer language ecosystems such as JavaScript's npm have established themselves with clearer dependency management. This streamlining is particularly appealing for new projects and developers seeking simplicity.
Stagnation in Innovation?
While Java has made strides with features like lambdas (Java 8) and pattern matching (Java 14), many developers perceive its incremental changes as insufficient. Community-driven languages like Rust and Kotlin enjoy enthusiastic backing and rapid growth, appealing to newer developers.
The Closing Argument
Java's legacy as a powerhouse in software development cannot be denied. However, as the landscape of programming languages shifts dramatically toward shorter, more efficient, and expressive paradigms, Java faces significant challenges.
The trends of increased focus on functional programming, performance needs, and lightweight frameworks underline the urgency for Java to evolve beyond its existing paradigms. While it remains relevant, especially in enterprise settings, the demand for innovation and adaptability to contemporary needs is critical.
Ultimately, Java can retain its place in the programming world through continued evolution and by embracing modern programming principles, perhaps by looking at the benefits seen in languages like Kotlin or Scala.
For further reading on Java's evolution, consider checking out the Java Documentation or the discussion on effective Java practices.
Embrace change. Keep coding!
Checkout our other articles