Common Pitfalls in Unit Testing Java Hadoop Jobs
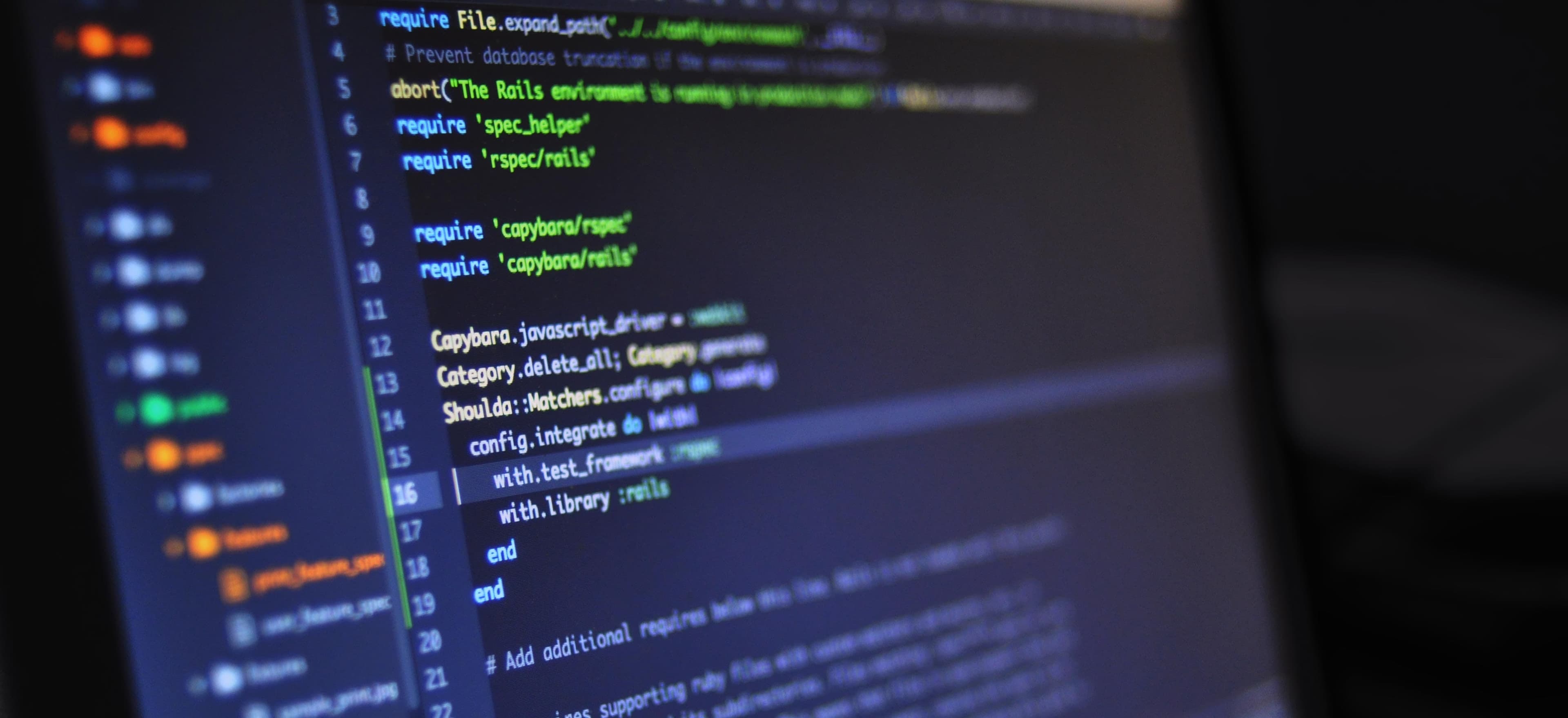
- Published on
Common Pitfalls in Unit Testing Java Hadoop Jobs
Unit testing is a crucial aspect of software development that ensures each component of your application behaves as expected. In a complex ecosystem like Hadoop, unit testing takes on an additional layer of complexity. Hadoop jobs, which often involve distributed data processing, can be challenging to test reliably. This post will explore common pitfalls in unit testing Java Hadoop jobs and how to navigate them effectively.
Understanding Hadoop Jobs
Before diving into unit testing, it's essential to comprehend what Hadoop jobs are. A Hadoop job typically refers to a process that uses the Hadoop framework for distributed computing and big data processing. It includes jobs like MapReduce tasks, which process vast amounts of data across distributed clusters.
In a typical Java Hadoop job, you may find classes like Mapper
, Reducer
, and Driver
. To ensure these components work seamlessly, unit testing is necessary. However, there are several pitfalls that can lead to ineffective testing.
Pitfall 1: Not Isolating Your Tests
One of the most significant mistakes in unit testing Hadoop jobs is not isolating tests properly. When tests depend on external systems or share states, they can lead to unpredictable results. Here is an example of an isolated unit test for a Mapper
class:
import org.apache.hadoop.io.IntWritable;
import org.apache.hadoop.io.Text;
import org.apache.hadoop.mrunit.mapreduce.MapDriver;
import org.junit.Before;
import org.junit.Test;
public class MyMapperTest {
private MapDriver<Text, IntWritable, Text, IntWritable> mapDriver;
@Before
public void setUp() {
MyMapper mapper = new MyMapper();
mapDriver = MapDriver.newMapDriver(mapper);
}
@Test
public void testMapper() {
mapDriver.withInput(new Text("key"), new IntWritable(1))
.withOutput(new Text("key"), new IntWritable(1))
.runTest();
}
}
Why Isolating Is Important
The above code demonstrates how to isolate the Mapper
test. It avoids dependencies on the Hadoop framework itself and uses MRUnit to directly test the Mapper logic. Isolation helps ensure that tests are repeatable and focus only on the functionality of the unit being tested.
Pitfall 2: Overlooking Data Input and Output
Another common oversight is not thoroughly testing different scenarios of data input and output. Often, tests only cover the happy path, leaving out edge cases that could lead to failures in production.
@Test
public void testEdgeCases() {
mapDriver.withInput(new Text(""), new IntWritable(0))
.withOutput(new Text(""), new IntWritable(0))
.runTest();
mapDriver.withInput(new Text("null"), new IntWritable(-1))
.withOutput(new Text("null"), new IntWritable(-1))
.runTest();
}
Why Cover Edge Cases
Covering edge cases is crucial for robustness and reliability. By testing various inputs, such as nulls or empty strings, you ensure your Hadoop job can handle unexpected situations gracefully.
Pitfall 3: Ignoring Local Unit Testing
In a big data context, it's easy to think that testing can only occur in a distributed environment. However, many aspects of Hadoop jobs can be tested locally on a smaller scale.
Local Testing with MiniCluster
Consider using a MiniCluster
to perform integration tests. It allows you to simulate a distributed environment locally and test how your job runs on Hadoop.
import org.apache.hadoop.hdron.test.MiniCluster;
import org.junit.After;
import org.junit.Before;
public class MyHadoopJobTest {
private MiniCluster miniCluster;
@Before
public void setUp() throws Exception {
miniCluster = new MiniCluster();
miniCluster.start();
}
@After
public void tearDown() {
miniCluster.stop();
}
}
Why Local Testing Matters
Local testing helps to identify issues early without deploying to a live cluster. It reduces the feedback loop, allowing for faster iterations and more reliable code before entering a production environment.
Pitfall 4: Neglecting Mocking Frameworks
Mocking frameworks are indispensable when unit testing, especially to avoid dependencies on complex external systems. Using frameworks like Mockito can help create mock objects that simulate interactions with the Hadoop framework.
import static org.mockito.Mockito.*;
import org.apache.hadoop.io.*;
@Test
public void testWithMocking() {
Context mockContext = mock(Context.class);
MyMapper mapper = new MyMapper();
// Set up Mock behavior
when(mockContext.write(any(Text.class), any(IntWritable.class))).thenAnswer(invocation -> {
return null;
});
// Test your mapper
mapper.map(new Text("key"), new IntWritable(1), mockContext);
verify(mockContext, times(1)).write(new Text("key"), new IntWritable(1));
}
Why Use Mocking
The example above demonstrates how to mock Context
to isolate the test. This approach allows you to simulate interactions without the overhead of running a full Hadoop job, resulting in faster unit tests.
Pitfall 5: Skipping Documentation and Comments
Documentation may feel tedious, especially in a tightly coupled system like Hadoop. However, providing comments and documentation in your tests is vital for future maintenance and understanding.
Example: Effective Commenting
@Test
public void testCorrectMapperOutput() {
// Test case where input is a regular key-value pair
mapDriver.withInput(new Text("inputKey"), new IntWritable(5))
.withOutput(new Text("expectedOutputKey"), new IntWritable(5))
.runTest(); // This should ensure our Mapper is producing the expected output.
}
Why Documentation Helps
Comments can clarify the purpose of a test, making it easier for others (or yourself in the future) to comprehend why a specific scenario was tested. Good documentation fosters collaboration and knowledge sharing.
Final Considerations
Testing Java Hadoop jobs is essential for ensuring robustness in data processing applications. However, it's easy to fall into common pitfalls that can lead to unreliable tests. By isolating tests, covering edge cases, utilizing mock frameworks, and documenting effectively, you can improve the reliability and maintainability of your Hadoop jobs.
For further reading on Hadoop best practices and testing methodologies, check out the official Apache Hadoop Documentation or MRUnit Documentation.
By being mindful of these pitfalls, you can enhance your unit testing strategy, ensuring your Hadoop applications run smoothly in production. Remember, a solid testing foundation paves the way for successful big data processing tasks. Happy coding!
Checkout our other articles